Library for uVGAIII
Embed:
(wiki syntax)
Show/hide line numbers
uVGAIII.h
00001 // 00002 // uVGAIII is a class to drive 4D Systems TFT touch screens 00003 // 00004 // Copyright (C) <2010> Stephane ROCHON <stephane.rochon at free.fr> 00005 // 00006 // uVGAIII is free software: you can redistribute it and/or modify 00007 // it under the terms of the GNU General Public License as published by 00008 // the Free Software Foundation, either version 3 of the License, or 00009 // (at your option) any later version. 00010 // 00011 // uVGAIII is distributed in the hope that it will be useful, 00012 // but WITHOUT ANY WARRANTY; without even the implied warranty of 00013 // MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 // GNU General Public License for more details. 00015 // 00016 // You should have received a copy of the GNU General Public License 00017 // along with uVGAIII. If not, see <http://www.gnu.org/licenses/>. 00018 00019 // @author Stephane Rochon 00020 00021 #include "mbed.h" 00022 00023 // Debug Verbose on terminal enabled 00024 #ifndef DEBUGMODE 00025 #define DEBUGMODE 1 00026 #endif 00027 00028 // Common WAIT value in millisecond 00029 #define TEMPO 5 00030 00031 // 4DGL Functions values 00032 // Graphic Commands values 00033 #define CLS 0xFFCD 00034 #define BCKGDCOLOR 0xFFA4 00035 #define SCREENMODE 0xFF9E 00036 #define CIRCLE 0xFFC3 00037 #define CIRCLE_F 0xFFC2 00038 #define TRIANGLE 0xFFBF 00039 #define TRIANGLE_F 0xFFA9 00040 #define LINE 0xFFC8 00041 #define RECTANGLE 0xFFC5 00042 #define RECTANGLE_F 0xFFC4 00043 #define ELLIPSE 0xFFB2 00044 #define ELLIPSE_F 0xFFB1 00045 #define PUTPIXEL 0xFFC1 00046 #define READPIXEL 0xFFC0 00047 #define SCREENCOPY 0xFFAD 00048 #define CLIPPING 0xFFA2 00049 #define SETCLIPWIN 0xFFB5 00050 #define EXTCLIPREG 0xFFB3 00051 #define BUTTON 0x0011 00052 #define PANEL 0xFFAF 00053 #define SLIDER 0xFFAE 00054 #define CHANGECOLOR 0xFFB4 00055 #define MOVEORIGIN 0xFFCC 00056 #define LINEPATTERN 0xFF9B 00057 #define OUTLINECOLOR 0xFF9D 00058 #define TRANSPARENCY 0xFFA0 00059 #define TRANSPCOLOR 0xFFA1 00060 #define PARAMETERS 0xFFCE 00061 00062 // System Commands 00063 #define BAUDRATE 0x0026 00064 #define SPEVERSION 0x001B 00065 #define PMMCVERSION 0x001C 00066 #define SETVOLUME 0xFF00 00067 00068 // Text and String Commands 00069 #define MOVECURSOR 0xFFE9 00070 #define PUTCHAR 0xFFFE 00071 #define SETFONT 0xFFE5 00072 #define TEXTOPACITY 0xFFDF 00073 #define TEXTFGCOLOR 0xFFE7 00074 #define TEXTBGCOLOR 0xFFE6 00075 #define TEXTWIDTH 0xFFE4 00076 #define TEXTHEIGHT 0xFFE3 00077 #define TEXTXGAP 0xFFE2 00078 #define TEXTYGAP 0xFFE1 00079 #define TEXTBOLD 0xFFDE 00080 #define TEXTINVERSE 0xFFDC 00081 #define TEXTITALIC 0xFFDD 00082 #define TEXTUNDLINE 0xFFDB 00083 #define TEXTATTRIBU 0xFFDA 00084 #define PUTSTRING 0x0018 00085 #define CHARWIDTH 0x001E 00086 #define CHARHEIGHT 0x001D 00087 #define GETTOUCH '\x6F' 00088 #define WAITTOUCH '\x77' 00089 #define SETTOUCH '\x75' 00090 00091 // Touch Screen Commands 00092 #define TOUCHSET 0xFF38 00093 #define TOUCHGET 0xFF37 00094 #define TOUCHDETECT 0xFF39 00095 00096 // Slider 00097 #define INTENDED '\x00' 00098 #define S_RAISED '\x01' 00099 #define HIDDEN '\x02' 00100 00101 // Button 00102 #define DEPRESSED '\x00' 00103 #define B_RAISED '\x01' 00104 00105 // Panel 00106 #define RECESSED '\x00' 00107 #define P_RAISED '\x01' 00108 00109 // Screen answers 00110 #define ACK '\x06' 00111 #define NAK '\x15' 00112 00113 // Screen states 00114 #define OFF '\x00' 00115 #define ON '\x01' 00116 00117 // Graphics parameters 00118 #define OBJECTCOLOR 18 00119 #define RESOLUTION 32 00120 #define PGDISPLAY 33 00121 #define PGREAD 34 00122 #define PGWRITE 35 00123 00124 // Graphics modes 00125 #define SOLID '\x00' 00126 #define WIREFRAME '\x01' 00127 00128 // Text modes 00129 #define TRANSPARENT '\x00' 00130 #define OPAQUE '\x01' 00131 00132 // Fonts Sizes 00133 #define FONT1 '\x00' // 7X8 00134 #define FONT2 '\x01' // 8X8 00135 #define FONT3 '\x02' // 8X12 00136 00137 // Touch Values 00138 #define MOVE '\x03' 00139 #define STATUS '\x00' 00140 #define GETXPOSITION '\x01' 00141 #define GETYPOSITION '\x02' 00142 00143 // Data speed 00144 #define BAUD_110 0x0000 00145 #define BAUD_300 0x0001 00146 #define BAUD_600 0x0002 00147 #define BAUD_1200 0x0003 00148 #define BAUD_2400 0x0004 00149 #define BAUD_4800 0x0005 00150 #define BAUD_9600 0x0006 00151 #define BAUD_14400 0x0007 00152 #define BAUD_19200 0x0008 00153 #define BAUD_31250 0x0009 00154 #define BAUD_38400 0x000A 00155 #define BAUD_56000 0x000B 00156 #define BAUD_57600 0x000C 00157 #define BAUD_115200 0x000D 00158 #define BAUD_128000 0x000E 00159 #define BAUD_256000 0x000F 00160 00161 // Defined Colors 00162 #define BLACK 0x000000 00163 #define WHITE 0xFFFFFF 00164 #define LGREY 0xBFBFBF 00165 #define DGREY 0x5F5F5F 00166 #define RED 0xFF0000 00167 #define LIME 0x00FF00 00168 #define BLUE 0x0000FF 00169 #define YELLOW 0xFFFF00 00170 #define CYAN 0x00FFFF 00171 #define MAGENTA 0xFF00FF 00172 #define SILVER 0xC0C0C0 00173 #define GRAY 0x808080 00174 #define MAROON 0x800000 00175 #define OLIVE 0x808000 00176 #define GREEN 0x008000 00177 #define PURPLE 0x800080 00178 #define TEAL 0x008080 00179 #define NAVY 0x000080 00180 00181 // Mode data 00182 #define BACKLIGHT '\x00' 00183 #define DISPLAY '\x01' 00184 #define CONTRAST '\x02' 00185 #define POWER '\x03' 00186 #define ORIENTATION '\x04' 00187 #define TOUCH_CTRL '\x05' 00188 #define IMAGE_FORMAT '\x06' 00189 #define PROTECT_FAT '\x08' 00190 00191 // change this to your specific screen (newer versions) if needed 00192 // Startup orientation is PORTRAIT so SIZE_X must be lesser than SIZE_Y 00193 #define SIZE_X 480 00194 #define SIZE_Y 800 00195 00196 #define IS_LANDSCAPE 0 00197 #define IS_PORTRAIT 1 00198 00199 // Screen orientation 00200 #define LANDSCAPE '\x00' 00201 #define LANDSCAPE_R '\x01' 00202 #define PORTRAIT '\x02' 00203 #define PORTRAIT_R '\x03' 00204 00205 /** 00206 * This is a class for uVGAIII 00207 */ 00208 class uVGAIII : public Stream 00209 { 00210 00211 public : 00212 00213 uVGAIII(PinName tx, PinName rx, PinName rst); 00214 00215 // General Commands ******************************************************************************* 00216 00217 /** Clear the entire screen using the current background colour */ 00218 void cls(); 00219 00220 /** Reset screen */ 00221 void reset(); 00222 00223 /** Set serial Baud rate (both sides : screen and mbed) 00224 * @param Speed Correct BAUD value (see uVGAIII.h) 00225 */ 00226 void baudrate(int speed); 00227 00228 /** Set internal speaker to specified value 00229 * @param value Correct range is 8 - 127 00230 */ 00231 void set_volume(char value); 00232 00233 // Graphics Commands 00234 /** Set the graphics orientation 00235 * @param mode LANDSCAPE, LANDSCAPE_R, PORTRAIT, PORTRAIT_R 00236 */ 00237 void screen_mode(char mode); 00238 00239 /** 00240 * Set background color of the screen 00241 * @param color 24 bits in total with the most significant byte representing the red part and the middle byte representing the green part and the least significant byte representing the blue part 00242 */ 00243 void background_color(int color); 00244 /** 00245 * Draw a circle 00246 * @param x x-coordinate of the center 00247 * @param y y-coordinate of the center 00248 * @param radius radius of the circle 00249 * @param color color of the outline of the circle 00250 */ 00251 void circle(int x , int y , int radius, int color); 00252 /** 00253 * Draw a filled circle 00254 * @param x x-coordinate of the center 00255 * @param y y-coordinate of the center 00256 * @param radius radius of the circle 00257 * @param color color of the circle 00258 */ 00259 void filled_circle(int x, int y, int radius, int color); 00260 /** 00261 * Draw a triangle 00262 * @param x1 x-coordinate of the first vertice 00263 * @param y1 y-coordinate of the first vertice 00264 * @param x2 x-coordinate of the second vertice 00265 * @param y2 y-coordinate of the second vertice 00266 * @param x3 x-coordinate of the third vertice 00267 * @param y3 y-coordinate of the third vertice 00268 * @param color color of the outline of the triangle 00269 */ 00270 void triangle(int x1, int y1, int x2, int y2, int x3, int y3, int color); 00271 /** 00272 * Draw a filled triangle 00273 * @param x1 x-coordinate of the first vertice 00274 * @param y1 y-coordinate of the first vertice 00275 * @param x2 x-coordinate of the second vertice 00276 * @param y2 y-coordinate of the second vertice 00277 * @param x3 x-coordinate of the third vertice 00278 * @param y3 y-coordinate of the third vertice 00279 * @param color color of the triangle 00280 */ 00281 void filled_triangle(int x1, int y1, int x2, int y2, int x3, int y3, int color); 00282 /** 00283 * Draw a line 00284 * @param x1 x-coordinate of the first vertice 00285 * @param y1 y-coordinate of the first vertice 00286 * @param x2 x-coordinate of the second vertice 00287 * @param y2 y-coordinate of the second vertice 00288 * @param color color of the line 00289 */ 00290 void line(int x1, int y1 , int x2, int y2, int color); 00291 /** 00292 * Draw a rectangle 00293 * @param x1 x-coordinate of the first vertice 00294 * @param y1 y-coordinate of the first vertice 00295 * @param x2 x-coordinate of the second vertice 00296 * @param y2 y-coordinate of the second vertice 00297 * @param color color of the outline of the rectangle 00298 */ 00299 void rectangle(int x1, int y1 , int x2, int y2, int color); 00300 /** 00301 * Draw a filled rectangle 00302 * @param x1 x-coordinate of the first vertice 00303 * @param y1 y-coordinate of the first vertice 00304 * @param x2 x-coordinate of the second vertice 00305 * @param y2 y-coordinate of the second vertice 00306 * @param color color of the rectangle 00307 */ 00308 void filled_rectangle(int, int, int, int, int); 00309 /** 00310 * Draw a ellipse 00311 * @param x x-coordinate of the center 00312 * @param y y-coordinate of the center 00313 * @param radius_x x-radius of the ellipse 00314 * @param redius_y y-radius of the ellipse 00315 * @param color color of the outline of the rectangle 00316 */ 00317 void ellipse(int x, int y , int radius_x, int radius_y, int color); 00318 /** 00319 * Draw a filled ellipse 00320 * @param x x-coordinate of the center 00321 * @param y y-coordinate of the center 00322 * @param radius_x x-radius of the ellipse 00323 * @param redius_y y-radius of the ellipse 00324 * @param color color of the rectangle 00325 */ 00326 void filled_ellipse(int x, int y , int radius_x, int radius_y, int color); 00327 /** 00328 * Draw a button 00329 * @param state appearance of button, 0 = Button depressed; 1 = Button raised 00330 * @param x x-coordinate of the top left corner of the button 00331 * @param y y-coordinate of the top left corner of the button 00332 * @param buttoncolor color of the button 00333 * @param txtcolor color of the text 00334 * @param font Specifies the Font ID 00335 * @param txtWidth Set the width of the text 00336 * @param txtHeight Set the height of the text 00337 * @param text Specifies the text string 00338 */ 00339 void button(int state, int x, int y, int buttoncolor, int txtcolor, int font, int txtWidth, int txtHeight, char * text); 00340 /** 00341 * Draw a panel 00342 * @param state appearance of panel, 0 = recessed; 1 = raised 00343 * @param x x-coordinate of the top left corner of the button 00344 * @param y y-coordinate of the top left corner of the button 00345 * @param Width Set the width of the panel 00346 * @param Height Set the height of the panel 00347 * @param color Set the color of the panel 00348 */ 00349 void panel(int state, int x, int y, int Width, int Height, int color); 00350 /** 00351 * Draw a slider 00352 * @param mode Mode = 0: Slider indented, mode = 1: Slider raised, mode 2: Slider hidden 00353 * @param x1 x-coordinate of the top left position of the slider 00354 * @param y1 y-coordinate of the top left position of the slider 00355 * @param x2 x-coordinate of the bottom right position of the slider 00356 * @param y2 y-coordinate of the bottom right position of the slider 00357 * @param color Set the color of slider 00358 * @param scale Set the full scale range of the slider for the thumb from 0 to n 00359 * @param value If value positive, sets the relative position of the thumb on the slider bar, else set thumb to ABS position of the negative number 00360 */ 00361 void slider(char mode, int x1, int y1, int x2, int y2, int color, int scale, int value); 00362 /** 00363 * Draw a pixel 00364 * @param x x-coordinate of the pixel 00365 * @param y y-coordinate of the pixel 00366 * @param color Set the color of pixel 00367 */ 00368 void put_pixel(int x, int y, int color); 00369 /** 00370 * Read the color value of the pixel 00371 * @param x x-coordinate of the pixel 00372 * @param y y-coordinate of the pixel 00373 * @return the color value of the pixel 00374 */ 00375 int read_pixel(int x, int y); 00376 /** 00377 * Copy an area of a screen 00378 * @param xs x-coordinate of the top left corner of the area to be copied 00379 * @param ys y-coordinate of the top left corner of the area to be copied 00380 * @param xd x-coordinate of the top left corner of the area to be pasted 00381 * @param yd y-coordinate of the top left corner of the area to be pasted 00382 * @param width Set the width of the copied area 00383 * @param width Set the height of the copied area 00384 */ 00385 void screen_copy(int xs, int ys , int xd, int yd , int width, int height); 00386 /** 00387 * Enable or disable the ability for Clipping to be used 00388 * @param value 0 = Clipping Disabled, 1 = Clipping Enabled 00389 */ 00390 void clipping(char value); 00391 /** 00392 * Specifiy the clipping window region on the screen 00393 * @param x1 x-coordinate of the top left corner of the clipping window 00394 * @param y1 y-coordinate of the top left corner of the clipping window 00395 * @param x2 x-coordinate of the bottom right corner of the clipping window 00396 * @param y2 y-coordinate of the bottom right corner of the clipping window 00397 */ 00398 void set_clipping_win(int x1, int y1, int x2, int y2); 00399 /** 00400 * Force the clip region to the extent of the last text 00401 * 00402 */ 00403 void extend_clip_region(); 00404 /** 00405 * Change all old color pixels to new color within the clipping window area 00406 * @param oldColor Sample color to be changed within the clipping window 00407 * @param newColor New color to change all occurrences of old color within the clipping window 00408 */ 00409 void change_color(int oldColor, int newColor); 00410 /** 00411 * Move the origin to a new position 00412 * @param xpos x-coordinate of the new origin 00413 * @param ypos y-coordinate of the new origin 00414 */ 00415 void move_origin(int xpos, int ypos); 00416 /** 00417 * Set the line pattern 00418 * @param pattern Correct value 0 to 65535 = number of bits in the line are turned off to form a pattern 00419 */ 00420 void line_pattern(int pattern); 00421 /** 00422 * Set the outline color for rectangles and circles 00423 * @param color 24 bits in total of the color value 00424 */ 00425 void outline_color(int color); 00426 /** 00427 * Set whether to enable the transparency 00428 * @param mode 0 = Transparency OFF, 1 = Transparency ON 00429 */ 00430 void transparency(char mode); 00431 /** 00432 * Set the color to be transparent 00433 * @param color Color to be set to be transparent 00434 */ 00435 void transparent_color(int color); 00436 /** 00437 * Set graphics parameters 00438 * @param function 18 = Object Color, 32 = Screen Resolution, 33 = Page Display, 34 = Page Read, 35 = Page Write 00439 * @param value different values to be applied according to different functions 00440 */ 00441 void graphics_parameters(int function, int value); 00442 00443 // Texts Commands 00444 /** 00445 * Set font mode 00446 * @param mode 0 for FONT1 = system font; 1 for FONT2; 2 for FONT3 = Default font 00447 */ 00448 void set_font(char mode); 00449 /** 00450 * Move teh text cursor to a screen postion 00451 * @param line Line position 00452 * @param column Column position 00453 */ 00454 void move_cursor(int line, int column); 00455 /** 00456 * Set whether or not the 'background' pixels are drawn 00457 * @param mode 1 for ON(opaque), 0 for OFF(transparent) 00458 */ 00459 void text_opacity(char mode); 00460 /** 00461 * Set the text width multiplier between 1 and 16 00462 * @param multiplier Width multiplier 1 to 16 00463 */ 00464 void text_width(int multiplier); 00465 /** 00466 * Set the text height multiplier between 1 and 16 00467 * @param multiplier Height multiplier 1 to 16 00468 */ 00469 void text_height(int multiplier); 00470 /** 00471 * Set the pixel gap between characters(x-axis) 00472 * @param pixelcount 0 to 32 00473 */ 00474 void text_x_gap(int pixelcount); 00475 /** 00476 * Set the pixel gap between characters(y-axis) 00477 * @param pixelcount 0 to 32 00478 */ 00479 void text_y_gap(int pixelcount); 00480 /** 00481 * Set the bold attribute for the text 00482 * @param mode 1 for ON, 0 for OFF 00483 */ 00484 void text_bold(char mode); 00485 /** 00486 * Inverse the background and foreground color of the text 00487 * @param mode 1 for ON, 0 for OFF 00488 */ 00489 void text_inverse(char mode); 00490 /** 00491 * Set the text to italic 00492 * @param mode 1 for ON, 0 for OFF 00493 */ 00494 void text_italic(char mode); 00495 /** 00496 * Set the text to underlined 00497 * @param mode 1 for ON, 0 for OFF 00498 */ 00499 void text_underline(char mode); 00500 /** 00501 * Control several functions grouped, Text Bold, Text Italic, Text Inverse, Text Underlined 00502 * @param value DEC 16 for BOLD, DEC 32 for ITALIC, DEC 64 for INVERSE, DEC 128 for UNDERLINED 00503 */ 00504 void text_attributes(int value); 00505 /** 00506 * Print a single character on the display 00507 * @param c Character to be print on the screen 00508 */ 00509 void put_char(char c); 00510 /** 00511 * Print a string on the display 00512 * @param s A Null terminated string 00513 */ 00514 void put_string(char *s); 00515 /** 00516 * Set the text foreground color 00517 * @param color Specify the color to be set 00518 */ 00519 void text_fgd_color(int color); 00520 /** 00521 * Set the text background color 00522 * @param color Specify the color to be set 00523 */ 00524 void text_bgd_color(int color); 00525 /** 00526 * calculate the width in pixel units for a character 00527 * @param c Character for the width calculation 00528 */ 00529 void char_width(char c); 00530 /** 00531 * calculate the height in pixel units for a character 00532 * @param c Character for the height calculation 00533 */ 00534 void char_height(char c); 00535 /** place char at current cursor position 00536 * used by virtual printf function _putc 00537 * @param c Character to be printed out 00538 */ 00539 void putc(char c); 00540 /** place string at current cursor position 00541 * @param s String to be printed out 00542 */ 00543 void puts(char *s); 00544 00545 // Touch Command 00546 /** Specify a new touch detect region on the screen 00547 * @param x1 x-coordinate of the top left corner of the region 00548 * @param y1 y-coordinate of the top left corner of the region 00549 * @param x2 x-coordinate of the bottom right corner of the region 00550 * @param y2 y-coordinate of the bottom rigth corner of the region 00551 */ 00552 void detect_touch_region(int x1, int y1, int x2, int y2); 00553 /** Get X coordinates of the touch 00554 * @param x X coordinate of the touch 00555 */ 00556 void touch_get_x(int *x); 00557 /** Get Y coordinates of the touch 00558 * @param y Y coordinate of the touch 00559 */ 00560 void touch_get_y(int *y); 00561 /** Set various touch screen related parameters 00562 * @param mode Mode = 0: enable and initialise touch screen hardware; mode = 1: disable the touch screen; mode = 2: reset the current active region to default which is the full screen area 00563 */ 00564 void touch_set(char mode); 00565 /** Get the status of the screen 00566 * @return Status of the screen: 0 = INVALID/NOTOUCH, 1 = PRESS, 2 = RELEASE, 3 = MOVING 00567 */ 00568 int touch_status(void); 00569 00570 // Screen Version Data 00571 int version; 00572 int SPEversion; 00573 int PmmCversion; 00574 00575 // Text data 00576 char current_col; 00577 char current_row; 00578 int current_color; 00579 char current_font; 00580 char current_orientation; 00581 char max_col; 00582 char max_row; 00583 int current_w, current_h; 00584 int current_fx, current_fy; 00585 00586 protected : 00587 00588 Serial _cmd; 00589 DigitalOut _rst; 00590 00591 //used by printf 00592 virtual int _putc(int c) { 00593 putc(c); 00594 return 0; 00595 }; 00596 virtual int _getc() { 00597 return -1; 00598 } 00599 00600 void freeBUFFER (void); 00601 void writeBYTE (char); 00602 int writeCOMMAND(char *, int, int); 00603 int readVERSION (char *, int); 00604 void getTOUCH (char *, int, int *); 00605 int getSTATUS (char *, int); 00606 void speversion (void); 00607 void pmmcversion (void); 00608 #if DEBUGMODE 00609 Serial pc; 00610 #endif // DEBUGMODE 00611 }; 00612 00613 typedef unsigned char BYTE; 00614
Generated on Tue Jul 12 2022 17:04:14 by
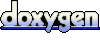