Library for uVGAIII
Embed:
(wiki syntax)
Show/hide line numbers
uVGAIII_Graphics.cpp
00001 // 00002 // uVGAIII is a class to drive 4D Systems TFT touch screens 00003 // 00004 // Copyright (C) <2010> Stephane ROCHON <stephane.rochon at free.fr> 00005 // 00006 // uVGAIII is free software: you can redistribute it and/or modify 00007 // it under the terms of the GNU General Public License as published by 00008 // the Free Software Foundation, either version 3 of the License, or 00009 // (at your option) any later version. 00010 // 00011 // uVGAIII is distributed in the hope that it will be useful, 00012 // but WITHOUT ANY WARRANTY; without even the implied warranty of 00013 // MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 // GNU General Public License for more details. 00015 // 00016 // You should have received a copy of the GNU General Public License 00017 // along with uVGAIII. If not, see <http://www.gnu.org/licenses/>. 00018 00019 #include "mbed.h" 00020 #include "uVGAIII.h" 00021 00022 #define ARRAY_SIZE(X) sizeof(X)/sizeof(X[0]) 00023 00024 //************************************************************************** 00025 void uVGAIII :: cls() { // clear screen 00026 char command[2] = ""; 00027 command[0] = ( CLS >> 8 ) & 0xFF; 00028 command[1] = CLS & 0xFF; 00029 writeCOMMAND(command, 2, 1); 00030 00031 #if DEBUGMODE 00032 pc.printf("Clear screen completed.\n"); 00033 #endif 00034 } 00035 00036 //**************************************************************************************************** 00037 void uVGAIII :: change_color(int oldColor, int newColor) { // change all old color pixels to new color within the clipping window area 00038 char command[6] = ""; 00039 00040 command[0] = (CHANGECOLOR >> 8) & 0xFF; 00041 command[1] = CHANGECOLOR & 0xFF; 00042 00043 int red5 = (oldColor >> (16 + 3)) & 0x1F; // get red on 5 bits 00044 int green6 = (oldColor >> (8 + 2)) & 0x3F; // get green on 6 bits 00045 int blue5 = (oldColor >> (0 + 3)) & 0x1F; // get blue on 5 bits 00046 00047 command[2] = ((red5 << 3) + (green6 >> 3)) & 0xFF; // first part of 16 bits color 00048 command[3] = ((green6 << 5) + (blue5 >> 0)) & 0xFF; // second part of 16 bits color 00049 00050 red5 = (newColor >> (16 + 3)) & 0x1F; // get red on 5 bits 00051 green6 = (newColor >> (8 + 2)) & 0x3F; // get green on 6 bits 00052 blue5 = (newColor >> (0 + 3)) & 0x1F; // get blue on 5 bits 00053 00054 command[4] = ((red5 << 3) + (green6 >> 3)) & 0xFF; // first part of 16 bits color 00055 command[5] = ((green6 << 5) + (blue5 >> 0)) & 0xFF; // second part of 16 bits color 00056 00057 writeCOMMAND(command, 6, 1); 00058 00059 #if DEBUGMODE 00060 pc.printf("Change color completed.\n"); 00061 #endif 00062 } 00063 00064 //**************************************************************************************************** 00065 void uVGAIII :: background_color(int color) { // set screen background color 00066 00067 char command[4]= ""; // input color is in 24bits like 0xRRGGBB 00068 00069 command[0] = ( BCKGDCOLOR >> 8 ) & 0xFF; 00070 command[1] = BCKGDCOLOR & 0xFF; 00071 00072 int red5 = (color >> (16 + 3)) & 0x1F; // get red on 5 bits 00073 int green6 = (color >> (8 + 2)) & 0x3F; // get green on 6 bits 00074 int blue5 = (color >> (0 + 3)) & 0x1F; // get blue on 5 bits 00075 00076 command[2] = ((red5 << 3) + (green6 >> 3)) & 0xFF; // first part of 16 bits color 00077 command[3] = ((green6 << 5) + (blue5 >> 0)) & 0xFF; // second part of 16 bits color 00078 00079 //command[2] = 0x00; 00080 //command[3] = 0x10; 00081 00082 writeCOMMAND(command, 4, 3); 00083 00084 #if DEBUGMODE 00085 pc.printf("Set background color completed.\n"); 00086 #endif 00087 } 00088 00089 //**************************************************************************************************** 00090 void uVGAIII :: screen_mode(char mode) { // set the graphics orientation LANDSCAPE, LANDSCAPE_R, PORTRAIT, PORTRAIT_R 00091 char command[4]= ""; 00092 00093 command[0] = (SCREENMODE >> 8) & 0xFF; 00094 command[1] = SCREENMODE & 0xFF; 00095 command[2] = 0x00; 00096 command[3] = mode; 00097 00098 switch (mode) { 00099 case LANDSCAPE : 00100 current_orientation = IS_LANDSCAPE; 00101 break; 00102 case LANDSCAPE_R : 00103 current_orientation = IS_LANDSCAPE; 00104 break; 00105 case PORTRAIT : 00106 current_orientation = IS_PORTRAIT; 00107 break; 00108 case PORTRAIT_R : 00109 current_orientation = IS_PORTRAIT; 00110 break; 00111 } 00112 00113 writeCOMMAND(command, 4, 3); 00114 00115 #if DEBUGMODE 00116 pc.printf("Screen mode completed.\n"); 00117 #endif 00118 00119 set_font(current_font); 00120 } 00121 00122 //**************************************************************************************************** 00123 void uVGAIII :: circle(int x, int y , int radius, int color) { // draw a circle in (x,y) 00124 char command[10]= ""; 00125 00126 command[0] = (CIRCLE >> 8) & 0xFF; 00127 command[1] = CIRCLE & 0xFF; 00128 00129 command[2] = (x >> 8) & 0xFF; 00130 command[3] = x & 0xFF; 00131 00132 command[4] = (y >> 8) & 0xFF; 00133 command[5] = y & 0xFF; 00134 00135 command[6] = (radius >> 8) & 0xFF; 00136 command[7] = radius & 0xFF; 00137 00138 int red5 = (color >> (16 + 3)) & 0x1F; // get red on 5 bits 00139 int green6 = (color >> (8 + 2)) & 0x3F; // get green on 6 bits 00140 int blue5 = (color >> (0 + 3)) & 0x1F; // get blue on 5 bits 00141 00142 command[8] = ((red5 << 3) + (green6 >> 3)) & 0xFF; // first part of 16 bits color 00143 command[9] = ((green6 << 5) + (blue5 >> 0)) & 0xFF; // second part of 16 bits color 00144 00145 writeCOMMAND(command, 10, 1); 00146 00147 #if DEBUGMODE 00148 pc.printf("Draw circle completed.\n"); 00149 #endif 00150 } 00151 00152 //**************************************************************************************************** 00153 void uVGAIII :: filled_circle(int x, int y , int radius, int color) { // draw a filled circle in (x,y) 00154 char command[10]= ""; 00155 00156 command[0] = (CIRCLE_F >> 8) & 0xFF; 00157 command[1] = CIRCLE_F & 0xFF; 00158 00159 command[2] = (x >> 8) & 0xFF; 00160 command[3] = x & 0xFF; 00161 00162 command[4] = (y >> 8) & 0xFF; 00163 command[5] = y & 0xFF; 00164 00165 command[6] = (radius >> 8) & 0xFF; 00166 command[7] = radius & 0xFF; 00167 00168 int red5 = (color >> (16 + 3)) & 0x1F; // get red on 5 bits 00169 int green6 = (color >> (8 + 2)) & 0x3F; // get green on 6 bits 00170 int blue5 = (color >> (0 + 3)) & 0x1F; // get blue on 5 bits 00171 00172 command[8] = ((red5 << 3) + (green6 >> 3)) & 0xFF; // first part of 16 bits color 00173 command[9] = ((green6 << 5) + (blue5 >> 0)) & 0xFF; // second part of 16 bits color 00174 00175 writeCOMMAND(command, 10, 1); 00176 00177 #if DEBUGMODE 00178 pc.printf("Draw filled circle completed.\n"); 00179 #endif 00180 } 00181 00182 //**************************************************************************************************** 00183 void uVGAIII :: triangle(int x1, int y1 , int x2, int y2, int x3, int y3, int color) { // draw a traingle 00184 char command[16]= ""; 00185 00186 command[0] = (TRIANGLE >> 8) & 0xFF; 00187 command[1] = TRIANGLE & 0xFF; 00188 00189 command[2] = (x1 >> 8) & 0xFF; 00190 command[3] = x1 & 0xFF; 00191 00192 command[4] = (y1 >> 8) & 0xFF; 00193 command[5] = y1 & 0xFF; 00194 00195 command[6] = (x2 >> 8) & 0xFF; 00196 command[7] = x2 & 0xFF; 00197 00198 command[8] = (y2 >> 8) & 0xFF; 00199 command[9] = y2 & 0xFF; 00200 00201 command[10] = (x3 >> 8) & 0xFF; 00202 command[11] = x3 & 0xFF; 00203 00204 command[12] = (y3 >> 8) & 0xFF; 00205 command[13] = y3 & 0xFF; 00206 00207 int red5 = (color >> (16 + 3)) & 0x1F; // get red on 5 bits 00208 int green6 = (color >> (8 + 2)) & 0x3F; // get green on 6 bits 00209 int blue5 = (color >> (0 + 3)) & 0x1F; // get blue on 5 bits 00210 00211 command[14] = ((red5 << 3) + (green6 >> 3)) & 0xFF; // first part of 16 bits color 00212 command[15] = ((green6 << 5) + (blue5 >> 0)) & 0xFF; // second part of 16 bits color 00213 00214 writeCOMMAND(command, 16, 1); 00215 00216 #if DEBUGMODE 00217 pc.printf("Draw triangle completed.\n"); 00218 #endif 00219 } 00220 00221 //**************************************************************************************************** 00222 void uVGAIII :: filled_triangle(int x1, int y1 , int x2, int y2, int x3, int y3, int color) { // draw a filled traingle 00223 char command[16]= ""; 00224 00225 command[0] = (TRIANGLE_F >> 8) & 0xFF; 00226 command[1] = TRIANGLE_F & 0xFF; 00227 00228 command[2] = (x1 >> 8) & 0xFF; 00229 command[3] = x1 & 0xFF; 00230 00231 command[4] = (y1 >> 8) & 0xFF; 00232 command[5] = y1 & 0xFF; 00233 00234 command[6] = (x2 >> 8) & 0xFF; 00235 command[7] = x2 & 0xFF; 00236 00237 command[8] = (y2 >> 8) & 0xFF; 00238 command[9] = y2 & 0xFF; 00239 00240 command[10] = (x3 >> 8) & 0xFF; 00241 command[11] = x3 & 0xFF; 00242 00243 command[12] = (y3 >> 8) & 0xFF; 00244 command[13] = y3 & 0xFF; 00245 00246 int red5 = (color >> (16 + 3)) & 0x1F; // get red on 5 bits 00247 int green6 = (color >> (8 + 2)) & 0x3F; // get green on 6 bits 00248 int blue5 = (color >> (0 + 3)) & 0x1F; // get blue on 5 bits 00249 00250 command[14] = ((red5 << 3) + (green6 >> 3)) & 0xFF; // first part of 16 bits color 00251 command[15] = ((green6 << 5) + (blue5 >> 0)) & 0xFF; // second part of 16 bits color 00252 00253 writeCOMMAND(command, 16, 1); 00254 00255 #if DEBUGMODE 00256 pc.printf("Draw filled triangle completed.\n"); 00257 #endif 00258 } 00259 00260 //**************************************************************************************************** 00261 void uVGAIII :: line(int x1, int y1 , int x2, int y2, int color) { // draw a line 00262 char command[12]= ""; 00263 00264 command[0] = (LINE >> 8) & 0xFF; 00265 command[1] = LINE & 0xFF; 00266 00267 command[2] = (x1 >> 8) & 0xFF; 00268 command[3] = x1 & 0xFF; 00269 00270 command[4] = (y1 >> 8) & 0xFF; 00271 command[5] = y1 & 0xFF; 00272 00273 command[6] = (x2 >> 8) & 0xFF; 00274 command[7] = x2 & 0xFF; 00275 00276 command[8] = (y2 >> 8) & 0xFF; 00277 command[9] = y2 & 0xFF; 00278 00279 int red5 = (color >> (16 + 3)) & 0x1F; // get red on 5 bits 00280 int green6 = (color >> (8 + 2)) & 0x3F; // get green on 6 bits 00281 int blue5 = (color >> (0 + 3)) & 0x1F; // get blue on 5 bits 00282 00283 command[10] = ((red5 << 3) + (green6 >> 3)) & 0xFF; // first part of 16 bits color 00284 command[11] = ((green6 << 5) + (blue5 >> 0)) & 0xFF; // second part of 16 bits color 00285 00286 writeCOMMAND(command, 12, 1); 00287 00288 #if DEBUGMODE 00289 pc.printf("Draw line completed.\n"); 00290 #endif 00291 } 00292 00293 //**************************************************************************************************** 00294 void uVGAIII :: rectangle(int x1, int y1 , int x2, int y2, int color) { // draw a rectangle 00295 char command[12]= ""; 00296 00297 command[0] = (RECTANGLE >> 8) & 0xFF; 00298 command[1] = RECTANGLE & 0xFF; 00299 00300 command[2] = (x1 >> 8) & 0xFF; 00301 command[3] = x1 & 0xFF; 00302 00303 command[4] = (y1 >> 8) & 0xFF; 00304 command[5] = y1 & 0xFF; 00305 00306 command[6] = (x2 >> 8) & 0xFF; 00307 command[7] = x2 & 0xFF; 00308 00309 command[8] = (y2 >> 8) & 0xFF; 00310 command[9] = y2 & 0xFF; 00311 00312 int red5 = (color >> (16 + 3)) & 0x1F; // get red on 5 bits 00313 int green6 = (color >> (8 + 2)) & 0x3F; // get green on 6 bits 00314 int blue5 = (color >> (0 + 3)) & 0x1F; // get blue on 5 bits 00315 00316 command[10] = ((red5 << 3) + (green6 >> 3)) & 0xFF; // first part of 16 bits color 00317 command[11] = ((green6 << 5) + (blue5 >> 0)) & 0xFF; // second part of 16 bits color 00318 00319 writeCOMMAND(command, 12, 1); 00320 00321 #if DEBUGMODE 00322 pc.printf("Draw rectangle completed.\n"); 00323 #endif 00324 } 00325 00326 //**************************************************************************************************** 00327 void uVGAIII :: filled_rectangle(int x1, int y1 , int x2, int y2, int color) { // draw a filled rectangle 00328 char command[12]= ""; 00329 00330 command[0] = (RECTANGLE_F >> 8) & 0xFF; 00331 command[1] = RECTANGLE_F & 0xFF; 00332 00333 command[2] = (x1 >> 8) & 0xFF; 00334 command[3] = x1 & 0xFF; 00335 00336 command[4] = (y1 >> 8) & 0xFF; 00337 command[5] = y1 & 0xFF; 00338 00339 command[6] = (x2 >> 8) & 0xFF; 00340 command[7] = x2 & 0xFF; 00341 00342 command[8] = (y2 >> 8) & 0xFF; 00343 command[9] = y2 & 0xFF; 00344 00345 int red5 = (color >> (16 + 3)) & 0x1F; // get red on 5 bits 00346 int green6 = (color >> (8 + 2)) & 0x3F; // get green on 6 bits 00347 int blue5 = (color >> (0 + 3)) & 0x1F; // get blue on 5 bits 00348 00349 command[10] = ((red5 << 3) + (green6 >> 3)) & 0xFF; // first part of 16 bits color 00350 command[11] = ((green6 << 5) + (blue5 >> 0)) & 0xFF; // second part of 16 bits color 00351 00352 writeCOMMAND(command, 12, 1); 00353 00354 #if DEBUGMODE 00355 pc.printf("Draw filled rectangle completed.\n"); 00356 #endif 00357 } 00358 00359 //**************************************************************************************************** 00360 void uVGAIII :: ellipse(int x, int y , int radius_x, int radius_y, int color) { // draw an ellipse 00361 char command[12]= ""; 00362 00363 command[0] = (ELLIPSE >> 8) & 0xFF; 00364 command[1] = ELLIPSE & 0xFF; 00365 00366 command[2] = (x >> 8) & 0xFF; 00367 command[3] = x & 0xFF; 00368 00369 command[4] = (y >> 8) & 0xFF; 00370 command[5] = y & 0xFF; 00371 00372 command[6] = (radius_x >> 8) & 0xFF; 00373 command[7] = radius_x & 0xFF; 00374 00375 command[8] = (radius_y >> 8) & 0xFF; 00376 command[9] = radius_y & 0xFF; 00377 00378 int red5 = (color >> (16 + 3)) & 0x1F; // get red on 5 bits 00379 int green6 = (color >> (8 + 2)) & 0x3F; // get green on 6 bits 00380 int blue5 = (color >> (0 + 3)) & 0x1F; // get blue on 5 bits 00381 00382 command[10] = ((red5 << 3) + (green6 >> 3)) & 0xFF; // first part of 16 bits color 00383 command[11] = ((green6 << 5) + (blue5 >> 0)) & 0xFF; // second part of 16 bits color 00384 00385 writeCOMMAND(command, 12, 1); 00386 00387 #if DEBUGMODE 00388 pc.printf("Draw ellipse completed.\n"); 00389 #endif 00390 } 00391 00392 //**************************************************************************************************** 00393 void uVGAIII :: filled_ellipse(int x, int y , int radius_x, int radius_y, int color) { // draw a filled ellipse 00394 char command[12] = ""; 00395 00396 command[0] = (ELLIPSE_F >> 8) & 0xFF; 00397 command[1] = ELLIPSE_F & 0xFF; 00398 00399 command[2] = (x >> 8) & 0xFF; 00400 command[3] = x & 0xFF; 00401 00402 command[4] = (y >> 8) & 0xFF; 00403 command[5] = y & 0xFF; 00404 00405 command[6] = (radius_x >> 8) & 0xFF; 00406 command[7] = radius_x & 0xFF; 00407 00408 command[8] = (radius_y >> 8) & 0xFF; 00409 command[9] = radius_y & 0xFF; 00410 00411 int red5 = (color >> (16 + 3)) & 0x1F; // get red on 5 bits 00412 int green6 = (color >> (8 + 2)) & 0x3F; // get green on 6 bits 00413 int blue5 = (color >> (0 + 3)) & 0x1F; // get blue on 5 bits 00414 00415 command[10] = ((red5 << 3) + (green6 >> 3)) & 0xFF; // first part of 16 bits color 00416 command[11] = ((green6 << 5) + (blue5 >> 0)) & 0xFF; // second part of 16 bits color 00417 00418 writeCOMMAND(command, 12, 1); 00419 00420 #if DEBUGMODE 00421 pc.printf("Draw filled ellipse completed.\n"); 00422 #endif 00423 } 00424 00425 //**************************************************************************************************** 00426 void uVGAIII :: button(int state, int x, int y, int buttoncolor, int txtcolor, int font, int txtWidth, int txtHeight, char * text) { // draw a button 00427 char command[1000] = ""; 00428 int size = strlen(text); 00429 int i = 0; 00430 00431 command[0] = (BUTTON >> 8) & 0xFF; 00432 command[1] = BUTTON & 0xFF; 00433 00434 command[2] = (state >> 8) & 0xFF; 00435 command[3] = state & 0xFF; 00436 00437 command[4] = (x >> 8) & 0xFF; 00438 command[5] = x & 0xFF; 00439 00440 command[6] = (y >> 8) & 0xFF; 00441 command[7] = y & 0xFF; 00442 00443 int red5 = (buttoncolor >> (16 + 3)) & 0x1F; // get red on 5 bits 00444 int green6 = (buttoncolor >> (8 + 2)) & 0x3F; // get green on 6 bits 00445 int blue5 = (buttoncolor >> (0 + 3)) & 0x1F; // get blue on 5 bits 00446 00447 command[8] = ((red5 << 3) + (green6 >> 3)) & 0xFF; 00448 command[9] = ((green6 << 5) + (blue5 >> 0)) & 0xFF; 00449 00450 red5 = (txtcolor >> (16 + 3)) & 0x1F; // get red on 5 bits 00451 green6 = (txtcolor >> (8 + 2)) & 0x3F; // get green on 6 bits 00452 blue5 = (txtcolor >> (0 + 3)) & 0x1F; // get blue on 5 bits 00453 00454 command[10] = ((red5 << 3) + (green6 >> 3)) & 0xFF; 00455 command[11] = ((green6 << 5) + (blue5 >> 0)) & 0xFF; 00456 00457 command[12] = (font >> 8) & 0xFF; 00458 command[13] = font & 0xFF; 00459 00460 command[14] = (txtWidth >> 8) & 0xFF; 00461 command[15] = txtWidth & 0xFF; 00462 00463 command[16] = (txtHeight >> 8) & 0xFF; 00464 command[17] = txtHeight & 0xFF; 00465 00466 for (i=0; i<size; i++) command[18+i] = text[i]; 00467 00468 command[18+size] = 0; 00469 00470 writeCOMMAND(command, 19 + size, 1); 00471 00472 #if DEBUGMODE 00473 pc.printf("Draw button completed.\n"); 00474 #endif 00475 } 00476 00477 //**************************************************************************************************** 00478 void uVGAIII :: panel(int state, int x, int y, int Width, int Height, int color) { // draw a panel 00479 char command[14] = ""; 00480 00481 command[0] = (PANEL >> 8) & 0xFF; 00482 command[1] = PANEL & 0xFF; 00483 00484 command[2] = (state >> 8) & 0xFF; 00485 command[3] = state & 0xFF; 00486 00487 command[4] = (x >> 8) & 0xFF; 00488 command[5] = x & 0xFF; 00489 00490 command[6] = (y >> 8) & 0xFF; 00491 command[7] = y & 0xFF; 00492 00493 command[8] = (Width >> 8) & 0xFF; 00494 command[9] = Width & 0xFF; 00495 00496 command[10] = (Height >> 8) & 0xFF; 00497 command[11] = Height & 0xFF; 00498 00499 int red5 = (color >> (16 + 3)) & 0x1F; // get red on 5 bits 00500 int green6 = (color >> (8 + 2)) & 0x3F; // get green on 6 bits 00501 int blue5 = (color >> (0 + 3)) & 0x1F; // get blue on 5 bits 00502 00503 command[12] = ((red5 << 3) + (green6 >> 3)) & 0xFF; 00504 command[13] = ((green6 << 5) + (blue5 >> 0)) & 0xFF; 00505 00506 writeCOMMAND(command, 14, 1); 00507 00508 #if DEBUGMODE 00509 pc.printf("Draw panel completed.\n"); 00510 #endif 00511 } 00512 00513 //**************************************************************************************************** 00514 void uVGAIII :: slider(char mode, int x1, int y1, int x2, int y2, int color, int scale, int value) { // draw a slider 00515 char command[18] = ""; 00516 00517 command[0] = (SLIDER >> 8) & 0xFF; 00518 command[1] = SLIDER & 0xFF; 00519 00520 command[2] = 0x00; 00521 command[3] = mode & 0xFF; 00522 00523 command[4] = (x1 >> 8) & 0xFF; 00524 command[5] = x1 & 0xFF; 00525 00526 command[6] = (y1 >> 8) & 0xFF; 00527 command[7] = y1 & 0xFF; 00528 00529 command[8] = (x2 >> 8) & 0xFF; 00530 command[9] = x2 & 0xFF; 00531 00532 command[10] = (y2 >> 8) & 0xFF; 00533 command[11] = y2 & 0xFF; 00534 00535 int red5 = (color >> (16 + 3)) & 0x1F; // get red on 5 bits 00536 int green6 = (color >> (8 + 2)) & 0x3F; // get green on 6 bits 00537 int blue5 = (color >> (0 + 3)) & 0x1F; // get blue on 5 bits 00538 00539 command[12] = ((red5 << 3) + (green6 >> 3)) & 0xFF; 00540 command[13] = ((green6 << 5) + (blue5 >> 0)) & 0xFF; 00541 00542 command[14] = (scale >> 8) & 0xFF; 00543 command[15] = scale & 0xFF; 00544 00545 command[16] = (value) & 0xFF; 00546 command[17] = value & 0xFF; 00547 00548 writeCOMMAND(command, 18, 1); 00549 00550 #if DEBUGMODE 00551 pc.printf("Draw slider completed.\n"); 00552 #endif 00553 } 00554 00555 //**************************************************************************************************** 00556 void uVGAIII :: put_pixel(int x, int y, int color) { // draw a pixel 00557 char command[8] = ""; 00558 00559 command[0] = (PUTPIXEL >> 8) & 0xFF; 00560 command[1] = PUTPIXEL & 0xFF; 00561 00562 command[2] = (x >> 8) & 0xFF; 00563 command[3] = x & 0xFF; 00564 00565 command[4] = (y >> 8) & 0xFF; 00566 command[5] = y & 0xFF; 00567 00568 int red5 = (color >> (16 + 3)) & 0x1F; // get red on 5 bits 00569 int green6 = (color >> (8 + 2)) & 0x3F; // get green on 6 bits 00570 int blue5 = (color >> (0 + 3)) & 0x1F; // get blue on 5 bits 00571 00572 command[6] = ((red5 << 3) + (green6 >> 3)) & 0xFF; // first part of 16 bits color 00573 command[7] = ((green6 << 5) + (blue5 >> 0)) & 0xFF; // second part of 16 bits color 00574 00575 writeCOMMAND(command, 8, 1); 00576 00577 #if DEBUGMODE 00578 pc.printf("Put pixel completed.\n"); 00579 #endif 00580 } 00581 00582 //****************************************************************************************************** 00583 int uVGAIII :: read_pixel(int x, int y) { // read screen info and populate data 00584 00585 char command[6]= ""; 00586 00587 command[0] = (READPIXEL >> 8) & 0xFF; 00588 command[1] = READPIXEL & 0xFF; 00589 00590 command[2] = (x >> 8) & 0xFF; 00591 command[3] = x & 0xFF; 00592 00593 command[4] = (y >> 8) & 0xFF; 00594 command[5] = y & 0xFF; 00595 00596 int i, temp = 0, color = 0, resp = 0; 00597 char response[3] = ""; 00598 00599 freeBUFFER(); 00600 00601 #if DEBUGMODE 00602 pc.printf("\n"); 00603 pc.printf("New COMMAND : 0x%02X%02X\n", command[0], command[1]); 00604 #endif 00605 00606 for (i = 0; i < 6; i++) { // send all chars to serial port 00607 writeBYTE(command[i]); 00608 } 00609 00610 while (!_cmd.readable()) wait_ms(TEMPO); // wait for screen answer 00611 00612 while (_cmd.readable() && resp < ARRAY_SIZE(response)) { 00613 temp = _cmd.getc(); 00614 #if DEBUGMODE 00615 pc.printf("Response = 0x%02X\n",int(temp)); 00616 #endif 00617 response[resp++] = (char)temp; 00618 } 00619 00620 color = ((response[1] << 8) + response[2]); 00621 00622 #if DEBUGMODE 00623 pc.printf("Read pixel completed.\n"); 00624 #endif 00625 00626 return color; // WARNING : this is 16bits color, not 24bits... need to be fixed 00627 } 00628 00629 //****************************************************************************************************** 00630 void uVGAIII :: screen_copy(int xs, int ys , int xd, int yd , int width, int height) { // copy an area of a screen from xs, ys of size given by width and height parameters and pastes it to another location determined by xd, yd 00631 00632 char command[14]= ""; 00633 00634 command[0] = (SCREENCOPY >> 8) & 0xFF; 00635 command[1] = SCREENCOPY & 0xFF; 00636 00637 command[2] = (xs >> 8) & 0xFF; 00638 command[3] = xs & 0xFF; 00639 00640 command[4] = (ys >> 8) & 0xFF; 00641 command[5] = ys & 0xFF; 00642 00643 command[6] = (xd >> 8) & 0xFF; 00644 command[7] = xd & 0xFF; 00645 00646 command[8] = (yd >> 8) & 0xFF; 00647 command[9] = yd & 0xFF; 00648 00649 command[10] = (width >> 8) & 0xFF; 00650 command[11] = width & 0xFF; 00651 00652 command[12] = (height >> 8) & 0xFF; 00653 command[13] = height & 0xFF; 00654 00655 writeCOMMAND(command, 14, 1); 00656 00657 #if DEBUGMODE 00658 pc.printf("Screen copy completed.\n"); 00659 #endif 00660 } 00661 00662 //****************************************************************************************************** 00663 void uVGAIII :: clipping(char value) { // To enable or disable the ability for Clippling to be used 00664 char command[4] = ""; 00665 00666 command[0] = (CLIPPING >> 8) & 0xFF; 00667 command[1] = CLIPPING & 0xFF; 00668 00669 command[2] = 0x00; 00670 command[3] = value & 0xFF; 00671 00672 writeCOMMAND(command, 4, 1); 00673 00674 #if DEBUGMODE 00675 pc.printf("Clipping completed.\n"); 00676 #endif 00677 } 00678 00679 //****************************************************************************************************** 00680 void uVGAIII :: set_clipping_win(int x1, int y1, int x2, int y2) { // Set the clipping window region 00681 char command[10] = ""; 00682 00683 command[0] = (SETCLIPWIN >> 8) & 0xFF; 00684 command[1] = SETCLIPWIN & 0xFF; 00685 00686 command[2] = (x1 >> 8) & 0xFF; 00687 command[3] = x1 & 0xFF; 00688 00689 command[4] = (y1 >> 8) & 0xFF; 00690 command[5] = y1 & 0xFF; 00691 00692 command[6] = (x2 >> 8) & 0xFF; 00693 command[7] = x2 & 0xFF; 00694 00695 command[8] = (y2 >> 8) & 0xFF; 00696 command[9] = y2 & 0xFF; 00697 00698 writeCOMMAND(command, 10, 1); 00699 00700 #if DEBUGMODE 00701 pc.printf("Set clipping window completed.\n"); 00702 #endif 00703 } 00704 00705 //****************************************************************************************************** 00706 void uVGAIII :: extend_clip_region() { // to force the clip region to the extent of the last text that was printed, or the last image that was shown 00707 char command[2] = ""; 00708 00709 command[0] = (EXTCLIPREG) & 0xFF; 00710 command[1] = EXTCLIPREG & 0xFF; 00711 00712 writeCOMMAND(command, 2, 1); 00713 00714 #if DEBUGMODE 00715 pc.printf("Extend clip region completed.\n"); 00716 #endif 00717 } 00718 00719 //****************************************************************************************************** 00720 void uVGAIII :: move_origin(int xpos, int ypos) { // Move the origin to a new position 00721 char command[6] = ""; 00722 00723 command[0] = (MOVEORIGIN) & 0xFF; 00724 command[1] = MOVEORIGIN & 0xFF; 00725 00726 command[2] = (xpos >> 8) & 0xFF; 00727 command[3] = xpos & 0xFF; 00728 00729 command[4] = (ypos >> 8) & 0xFF; 00730 command[5] = ypos & 0xFF; 00731 00732 writeCOMMAND(command, 6, 1); 00733 00734 #if DEBUGMODE 00735 pc.printf("Move origin completed.\n"); 00736 #endif 00737 } 00738 00739 //****************************************************************************************************** 00740 void uVGAIII :: line_pattern(int pattern) { // Set the line pattern 00741 char command[4] = ""; // If set to zero means lines are solid, else each '1' bit represents a pixel that is turned off 00742 00743 command[0] = (LINEPATTERN) & 0xFF; 00744 command[1] = LINEPATTERN & 0xFF; 00745 00746 command[2] = (pattern >> 8) & 0xFF; 00747 command[3] = pattern & 0xFF; 00748 00749 writeCOMMAND(command, 4, 3); 00750 00751 #if DEBUGMODE 00752 pc.printf("Line pattern completed.\n"); 00753 #endif 00754 } 00755 00756 //****************************************************************************************************** 00757 void uVGAIII :: transparency(char mode) { // set whether to enable the transparency 00758 char command[4] = ""; 00759 00760 command[0] = (TRANSPARENCY) & 0xFF; 00761 command[1] = TRANSPARENCY & 0xFF; 00762 00763 command[2] = 0x00; 00764 command[3] = mode & 0xFF; 00765 00766 writeCOMMAND(command, 4, 3); 00767 00768 #if DEBUGMODE 00769 pc.printf("Transparency completed.\n"); 00770 #endif 00771 } 00772 00773 //****************************************************************************************************** 00774 void uVGAIII :: outline_color(int color) { // set the outline color for rectangles and circles 00775 char command[4] = ""; 00776 00777 command[0] = (OUTLINECOLOR) & 0xFF; 00778 command[1] = OUTLINECOLOR & 0xFF; 00779 00780 int red5 = (color >> (16 + 3)) & 0x1F; // get red on 5 bits 00781 int green6 = (color >> (8 + 2)) & 0x3F; // get green on 6 bits 00782 int blue5 = (color >> (0 + 3)) & 0x1F; // get blue on 5 bits 00783 00784 command[2] = ((red5 << 3) + (green6 >> 3)) & 0xFF; 00785 command[3] = ((green6 << 5) + (blue5 >> 0)) & 0xFF; 00786 00787 writeCOMMAND(command, 4, 3); 00788 00789 #if DEBUGMODE 00790 pc.printf("Outline color completed.\n"); 00791 #endif 00792 } 00793 00794 //****************************************************************************************************** 00795 void uVGAIII :: transparent_color(int color) { // Set the color to be transparent 00796 char command[4] = ""; 00797 00798 command[0] = (TRANSPCOLOR) & 0xFF; 00799 command[1] = TRANSPCOLOR & 0xFF; 00800 00801 int red5 = (color >> (16 + 3)) & 0x1F; // get red on 5 bits 00802 int green6 = (color >> (8 + 2)) & 0x3F; // get green on 6 bits 00803 int blue5 = (color >> (0 + 3)) & 0x1F; // get blue on 5 bits 00804 00805 command[2] = ((red5 << 3) + (green6 >> 3)) & 0xFF; 00806 command[3] = ((green6 << 5) + (blue5 >> 0)) & 0xFF; 00807 00808 writeCOMMAND(command, 4, 3); 00809 00810 #if DEBUGMODE 00811 pc.printf("Transparent color completed.\n"); 00812 #endif 00813 } 00814 00815 //****************************************************************************************************** 00816 void uVGAIII :: graphics_parameters(int function, int value) { //Set graphics parameters 00817 char command[6] = ""; 00818 00819 command[0] = (PARAMETERS >> 8) & 0xFF; 00820 command[1] = PARAMETERS & 0xFF; 00821 00822 command[2] = (function >> 8) & 0xFF; 00823 command[3] = function & 0xFF; 00824 00825 command[4] = (value >> 8) & 0xFF; 00826 command[5] = value & 0xFF; 00827 00828 writeCOMMAND(command, 6, 1); 00829 00830 #if DEBUGMODE 00831 pc.printf("Graphics parameters completed.\n"); 00832 #endif 00833 }
Generated on Tue Jul 12 2022 17:04:14 by
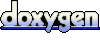