Library for uVGAIII
Embed:
(wiki syntax)
Show/hide line numbers
uVGAIII_Touch.cpp
00001 // 00002 // uVGAIII is a class to drive 4D Systems TFT touch screens 00003 // 00004 // Copyright (C) <2010> Stephane ROCHON <stephane.rochon at free.fr> 00005 // 00006 // uVGAIII is free software: you can redistribute it and/or modify 00007 // it under the terms of the GNU General Public License as published by 00008 // the Free Software Foundation, either version 3 of the License, or 00009 // (at your option) any later version. 00010 // 00011 // uVGAIII is distributed in the hope that it will be useful, 00012 // but WITHOUT ANY WARRANTY; without even the implied warranty of 00013 // MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 // GNU General Public License for more details. 00015 // 00016 // You should have received a copy of the GNU General Public License 00017 // along with uVGAIII. If not, see <http://www.gnu.org/licenses/>. 00018 00019 #include "mbed.h" 00020 #include "uVGAIII.h" 00021 00022 //****************************************************************************************************** 00023 int uVGAIII :: touch_status(void) { // Get the touch screen status 00024 char command[4] = ""; 00025 00026 command[0] = (TOUCHGET >> 8) & 0xFF; 00027 command[1] = TOUCHGET & 0xFF; 00028 00029 command[2] = (STATUS >> 8) & 0xFF; 00030 command[3] = STATUS & 0xFF; 00031 00032 return getSTATUS(command, 4); 00033 } 00034 00035 //****************************************************************************************************** 00036 void uVGAIII :: touch_get_x(int *x) { // Get X coordinates 00037 char command[4] = ""; 00038 00039 command[0] = (TOUCHGET >> 8) & 0xFF; 00040 command[1] = TOUCHGET & 0xFF; 00041 00042 command[2] = 0; 00043 command[3] = GETXPOSITION; 00044 00045 getTOUCH(command, 4, x); 00046 00047 #if DEBUGMODE 00048 pc.printf("Get X coordinates completed.\n"); 00049 #endif 00050 } 00051 00052 //****************************************************************************************************** 00053 void uVGAIII :: touch_get_y(int *y) { // Get Y coordinates 00054 char command[4] = ""; 00055 00056 command[0] = (TOUCHGET >> 8) & 0xFF; 00057 command[1] = TOUCHGET & 0xFF; 00058 00059 command[2] = 0; 00060 command[3] = GETYPOSITION; 00061 00062 getTOUCH(command, 4, y); 00063 00064 #if DEBUGMODE 00065 pc.printf("Get Y coordinates completed.\n"); 00066 #endif 00067 } 00068 00069 //****************************************************************************************************** 00070 void uVGAIII :: touch_set(char mode) { // Sets various Touch Screen related parameters 00071 00072 char command[4]= ""; 00073 00074 command[0] = (TOUCHSET >>8) & 0xFF; 00075 command[1] = TOUCHSET & 0xFF; 00076 00077 command[2] = 0; 00078 command[3] = mode; 00079 00080 writeCOMMAND(command, 4, 1); 00081 00082 #if DEBUGMODE 00083 pc.printf("Touch set completed.\n"); 00084 #endif 00085 } 00086 00087 //****************************************************************************************************** 00088 void uVGAIII :: detect_touch_region(int x1, int y1, int x2, int y2) { // Specificies a new touch detect region on the screen 00089 char command[10] = ""; 00090 00091 command[0] = (TOUCHDETECT >> 8) & 0xFF; 00092 command[1] = TOUCHDETECT & 0xFF; 00093 00094 command[2] = (x1 >> 8) & 0xFF; 00095 command[3] = x1 & 0xFF; 00096 00097 command[4] = (y1 >> 8) & 0xFF; 00098 command[5] = y1 & 0xFF; 00099 00100 command[6] = (x2 >> 8) & 0xFF; 00101 command[7] = x2 & 0xFF; 00102 00103 command[8] = (y2 >> 8) & 0xFF; 00104 command[9] = y2 & 0xFF; 00105 00106 writeCOMMAND(command, 10, 1); 00107 00108 #if DEBUGMODE 00109 pc.printf("Detect touch region completed.\n"); 00110 #endif 00111 }
Generated on Tue Jul 12 2022 17:04:14 by
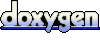