RTOS enabled i2c-driver based on the official i2c-C-api.
Fork of mbed-RtosI2cDriver by
I2CMasterRtos Class Reference
I2C master interface to the RTOS-I2CDriver. More...
#include <I2CMasterRtos.h>
Public Member Functions | |
I2CMasterRtos (PinName sda, PinName scl, int freq=100000) | |
Create an I2C Master interface, connected to the specified pins. | |
void | frequency (int hz) |
Set the frequency of the I2C interface. | |
int | read (int address, char *data, int length, bool repeated=false) |
Read from an I2C slave. | |
int | read (int address, uint8_t _register, char *data, int length, bool repeated=false) |
Read from a given I2C slave register. | |
int | read (int ack) |
Read a single byte from the I2C bus. | |
int | write (int address, const char *data, int length, bool repeated=false) |
Write to an I2C slave. | |
int | write (int data) |
Write single byte out on the I2C bus. | |
void | start (void) |
Creates a start condition on the I2C bus. | |
bool | stop (void) |
Creates a stop condition on the I2C bus If unsccessful because someone on the bus holds the scl line down it returns "false" after 23µs In normal operation the stop shouldn't take longer than 12µs @ 100kHz and 3-4µs @ 400kHz. | |
void | lock () |
Wait until the interface becomes available. | |
void | unlock () |
Unlock the interface that has previously been locked by the same thread. |
Detailed Description
I2C master interface to the RTOS-I2CDriver.
The interface is compatible to the original mbed I2C class. Provides an additonal "read from register"-function.
Definition at line 12 of file I2CMasterRtos.h.
Constructor & Destructor Documentation
I2CMasterRtos | ( | PinName | sda, |
PinName | scl, | ||
int | freq = 100000 |
||
) |
Create an I2C Master interface, connected to the specified pins.
- Parameters:
-
sda I2C data line pin scl I2C clock line pin
- Note:
- Has to be created in a thread context, i.e. within the main or some other function. A global delaration does not work
Definition at line 24 of file I2CMasterRtos.h.
Member Function Documentation
void frequency | ( | int | hz ) |
Set the frequency of the I2C interface.
- Parameters:
-
hz The bus frequency in hertz
Definition at line 30 of file I2CMasterRtos.h.
void lock | ( | ) |
Wait until the interface becomes available.
Useful if you want to run a sequence of command without interrution by another thread. There's no need to call this function for running single request, because all driver functions will lock the device for exclusive access automatically.
Definition at line 131 of file I2CMasterRtos.h.
int read | ( | int | address, |
char * | data, | ||
int | length, | ||
bool | repeated = false |
||
) |
Read from an I2C slave.
Performs a complete read transaction. The bottom bit of the address is forced to 1 to indicate a read.
- Parameters:
-
address 8-bit I2C slave address [ addr | 1 ] data Pointer to the byte-array to read data in to length Number of bytes to read repeated Repeated start, true - don't send stop at end
- Returns:
- 0 on success (ack), non-0 on failure (nack)
Definition at line 48 of file I2CMasterRtos.h.
int read | ( | int | ack ) |
Read a single byte from the I2C bus.
- Parameters:
-
ack indicates if the byte is to be acknowledged (1 = acknowledge)
- Returns:
- the byte read
Definition at line 78 of file I2CMasterRtos.h.
int read | ( | int | address, |
uint8_t | _register, | ||
char * | data, | ||
int | length, | ||
bool | repeated = false |
||
) |
Read from a given I2C slave register.
Performs a complete write-register-read-data-transaction. The bottom bit of the address is forced to 1 to indicate a read.
- Parameters:
-
address 8-bit I2C slave address [ addr | 1 ] _register 8-bit regster address data Pointer to the byte-array to read data in to length Number of bytes to read repeated Repeated start, true - don't send stop at end
- Returns:
- 0 on success (ack), non-0 on failure (nack)
Definition at line 67 of file I2CMasterRtos.h.
void start | ( | void | ) |
Creates a start condition on the I2C bus.
Definition at line 115 of file I2CMasterRtos.h.
bool stop | ( | void | ) |
Creates a stop condition on the I2C bus If unsccessful because someone on the bus holds the scl line down it returns "false" after 23µs In normal operation the stop shouldn't take longer than 12µs @ 100kHz and 3-4µs @ 400kHz.
Definition at line 122 of file I2CMasterRtos.h.
void unlock | ( | ) |
Unlock the interface that has previously been locked by the same thread.
Definition at line 136 of file I2CMasterRtos.h.
int write | ( | int | data ) |
Write single byte out on the I2C bus.
- Parameters:
-
data data to write out on bus
- Returns:
- '1' if an ACK was received, '0' otherwise
Definition at line 108 of file I2CMasterRtos.h.
int write | ( | int | address, |
const char * | data, | ||
int | length, | ||
bool | repeated = false |
||
) |
Write to an I2C slave.
Performs a complete write transaction. The bottom bit of the address is forced to 0 to indicate a write.
- Parameters:
-
address 8-bit I2C slave address [ addr | 0 ] data Pointer to the byte-array data to send length Number of bytes to send repeated Repeated start, true - do not send stop at end
- Returns:
- 0 on success (ack), non-0 on failure (nack)
Definition at line 96 of file I2CMasterRtos.h.
Generated on Wed Jul 13 2022 17:20:05 by
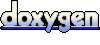