RTOS enabled i2c-driver based on the official i2c-C-api.
Fork of mbed-RtosI2cDriver by
I2CMasterRtos.h
00001 #ifndef I2CMASTERRTOS_H 00002 #define I2CMASTERRTOS_H 00003 00004 #include "I2CDriver.h" 00005 00006 namespace mbed 00007 { 00008 00009 /// I2C master interface to the RTOS-I2CDriver. 00010 /// The interface is compatible to the original mbed I2C class. 00011 /// Provides an additonal "read from register"-function. 00012 class I2CMasterRtos 00013 { 00014 I2CDriver m_drv; 00015 00016 public: 00017 /** Create an I2C Master interface, connected to the specified pins 00018 * 00019 * @param sda I2C data line pin 00020 * @param scl I2C clock line pin 00021 * 00022 * @note Has to be created in a thread context, i.e. within the main or some other function. A global delaration does not work 00023 */ 00024 I2CMasterRtos(PinName sda, PinName scl, int freq=100000):m_drv(sda,scl,freq) {} 00025 00026 /** Set the frequency of the I2C interface 00027 * 00028 * @param hz The bus frequency in hertz 00029 */ 00030 void frequency(int hz) { 00031 m_drv.frequency(hz); 00032 } 00033 00034 /** Read from an I2C slave 00035 * 00036 * Performs a complete read transaction. The bottom bit of 00037 * the address is forced to 1 to indicate a read. 00038 * 00039 * @param address 8-bit I2C slave address [ addr | 1 ] 00040 * @param data Pointer to the byte-array to read data in to 00041 * @param length Number of bytes to read 00042 * @param repeated Repeated start, true - don't send stop at end 00043 * 00044 * @returns 00045 * 0 on success (ack), 00046 * non-0 on failure (nack) 00047 */ 00048 int read(int address, char *data, int length, bool repeated = false) { 00049 return m_drv.readMaster( address, data, length, repeated); 00050 } 00051 00052 /** Read from a given I2C slave register 00053 * 00054 * Performs a complete write-register-read-data-transaction. The bottom bit of 00055 * the address is forced to 1 to indicate a read. 00056 * 00057 * @param address 8-bit I2C slave address [ addr | 1 ] 00058 * @param _register 8-bit regster address 00059 * @param data Pointer to the byte-array to read data in to 00060 * @param length Number of bytes to read 00061 * @param repeated Repeated start, true - don't send stop at end 00062 * 00063 * @returns 00064 * 0 on success (ack), 00065 * non-0 on failure (nack) 00066 */ 00067 int read(int address, uint8_t _register, char* data, int length, bool repeated = false) { 00068 return m_drv.readMaster( address, _register, data, length, repeated); 00069 } 00070 00071 /** Read a single byte from the I2C bus 00072 * 00073 * @param ack indicates if the byte is to be acknowledged (1 = acknowledge) 00074 * 00075 * @returns 00076 * the byte read 00077 */ 00078 int read(int ack) { 00079 return m_drv.readMaster(ack); 00080 } 00081 00082 /** Write to an I2C slave 00083 * 00084 * Performs a complete write transaction. The bottom bit of 00085 * the address is forced to 0 to indicate a write. 00086 * 00087 * @param address 8-bit I2C slave address [ addr | 0 ] 00088 * @param data Pointer to the byte-array data to send 00089 * @param length Number of bytes to send 00090 * @param repeated Repeated start, true - do not send stop at end 00091 * 00092 * @returns 00093 * 0 on success (ack), 00094 * non-0 on failure (nack) 00095 */ 00096 int write(int address, const char *data, int length, bool repeated = false) { 00097 return m_drv.writeMaster(address, data, length, repeated); 00098 } 00099 00100 /** Write single byte out on the I2C bus 00101 * 00102 * @param data data to write out on bus 00103 * 00104 * @returns 00105 * '1' if an ACK was received, 00106 * '0' otherwise 00107 */ 00108 int write(int data) { 00109 return m_drv.writeMaster(data); 00110 } 00111 00112 /** Creates a start condition on the I2C bus 00113 */ 00114 00115 void start(void) { 00116 m_drv.startMaster(); 00117 } 00118 00119 /// Creates a stop condition on the I2C bus 00120 /// If unsccessful because someone on the bus holds the scl line down it returns "false" after 23µs 00121 /// In normal operation the stop shouldn't take longer than 12µs @ 100kHz and 3-4µs @ 400kHz. 00122 bool stop(void) { 00123 return m_drv.stopMaster(); 00124 } 00125 00126 /// Wait until the interface becomes available. 00127 /// 00128 /// Useful if you want to run a sequence of command without interrution by another thread. 00129 /// There's no need to call this function for running single request, because all driver functions 00130 /// will lock the device for exclusive access automatically. 00131 void lock() { 00132 m_drv.lock(); 00133 } 00134 00135 /// Unlock the interface that has previously been locked by the same thread. 00136 void unlock() { 00137 m_drv.unlock(); 00138 } 00139 00140 }; 00141 } 00142 00143 00144 #endif
Generated on Wed Jul 13 2022 17:20:05 by
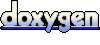