
X-CUBE-SPN1-20150128 example source code for one motor compiled under mbed. Tested OK on Nucleo F401. l6474.cpp is modified from original with defines in l6474_target_config.h to select the original behaviour (motor de-energised when halted), or new mode to continue powering with a (reduced) current in the coils (braking/position hold capability). On F401 avoid using mbed's InterruptIn on pins 10-15 (any port). Beware of other conflicts! L0 & F0 are included but untested.
motorcontrol.h
00001 /** 00002 ****************************************************************************** 00003 * @file motorcontrol.h 00004 * @author IPC Rennes 00005 * @version V1.0.0 00006 * @date 12-November-2014 00007 * @brief This file provides common definitions for motor control 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2014 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 00039 /* Define to prevent recursive inclusion -------------------------------------*/ 00040 #ifndef __MOTOR_CONTROL_H 00041 #define __MOTOR_CONTROL_H 00042 00043 #ifdef __cplusplus 00044 extern "C" { 00045 #endif 00046 00047 /* Includes ------------------------------------------------------------------*/ 00048 #include "motor.h" 00049 00050 /** @addtogroup BSP 00051 * @{ 00052 */ 00053 00054 /** @addtogroup MOTOR_CONTROL 00055 * @{ 00056 */ 00057 00058 /** @defgroup MOTOR_CONTROL_Exported_Types 00059 * @{ 00060 */ 00061 00062 00063 00064 /** 00065 * @} 00066 */ 00067 00068 /** @defgroup MOTOR_CONTROL_Exported_Constants 00069 * @{ 00070 */ 00071 ///Motor control board id for L6474 00072 #define BSP_MOTOR_CONTROL_BOARD_ID_L6474 (6474) 00073 ///Motor control board id for Powerstep01 00074 #define BSP_MOTOR_CONTROL_BOARD_ID_POWERSTEP01 (0001) 00075 00076 /** 00077 * @} 00078 */ 00079 00080 00081 /** @defgroup MOTOR_CONTROL_Exported_Macros 00082 * @{ 00083 */ 00084 #if defined ( __GNUC__ ) 00085 #ifndef __weak 00086 #define __weak __attribute__((weak)) 00087 #endif /* __weak */ 00088 #endif /* __GNUC__ */ 00089 /** 00090 * @} 00091 */ 00092 00093 /** @defgroup MOTOR_CONTROL_Weak_Function_Prototypes 00094 * @{ 00095 */ 00096 __weak motorDrv_t* L6474_GetMotorHandle(void); 00097 __weak motorDrv_t* Powerstep01_GetMotorHandle(void); 00098 /** 00099 * @} 00100 */ 00101 00102 /** @defgroup MOTOR_CONTROL_Exported_Functions 00103 * @{ 00104 */ 00105 void BSP_MotorControl_AttachErrorHandler(void (*callback)(uint16_t)); 00106 void BSP_MotorControl_AttachFlagInterrupt(void (*callback)(void)); 00107 void BSP_MotorControl_AttachBusyInterrupt(void (*callback)(void)); 00108 void BSP_MotorControl_ErrorHandler(uint16_t error); 00109 void BSP_MotorControl_Init(uint16_t id, uint8_t nbDevices); 00110 void BSP_MotorControl_FlagInterruptHandler(void); 00111 uint16_t BSP_MotorControl_GetAcceleration(uint8_t deviceId); 00112 uint16_t BSP_MotorControl_GetBoardId(void); 00113 uint16_t BSP_MotorControl_GetCurrentSpeed(uint8_t deviceId); 00114 uint16_t BSP_MotorControl_GetDeceleration(uint8_t deviceId); 00115 motorState_t BSP_MotorControl_GetDeviceState(uint8_t deviceId); 00116 uint8_t BSP_MotorControl_GetFwVersion(void); 00117 int32_t BSP_MotorControl_GetMark(uint8_t deviceId); 00118 uint16_t BSP_MotorControl_GetMaxSpeed(uint8_t deviceId); 00119 uint16_t BSP_MotorControl_GetMinSpeed(uint8_t deviceId); 00120 int32_t BSP_MotorControl_GetPosition(uint8_t deviceId); 00121 void BSP_MotorControl_GoHome(uint8_t deviceId); 00122 void BSP_MotorControl_GoMark(uint8_t deviceId); 00123 void BSP_MotorControl_GoTo(uint8_t deviceId, int32_t targetPosition); 00124 void BSP_MotorControl_HardStop(uint8_t deviceId); 00125 void BSP_MotorControl_Move(uint8_t deviceId, motorDir_t direction, uint32_t stepCount); 00126 void BSP_MotorControl_ResetAllDevices(void); 00127 void BSP_MotorControl_Run(uint8_t deviceId, motorDir_t direction); 00128 bool BSP_MotorControl_SetAcceleration(uint8_t deviceId,uint16_t newAcc); 00129 bool BSP_MotorControl_SetDeceleration(uint8_t deviceId, uint16_t newDec); 00130 void BSP_MotorControl_SetHome(uint8_t deviceId); 00131 void BSP_MotorControl_SetMark(uint8_t deviceId); 00132 bool BSP_MotorControl_SetMaxSpeed(uint8_t deviceId, uint16_t newMaxSpeed); 00133 bool BSP_MotorControl_SetMinSpeed(uint8_t deviceId, uint16_t newMinSpeed); 00134 bool BSP_MotorControl_SoftStop(uint8_t deviceId); 00135 void BSP_MotorControl_StepClockHandler(uint8_t deviceId); 00136 void BSP_MotorControl_WaitWhileActive(uint8_t deviceId); 00137 void BSP_MotorControl_CmdDisable(uint8_t deviceId); 00138 void BSP_MotorControl_CmdEnable(uint8_t deviceId); 00139 uint32_t BSP_MotorControl_CmdGetParam(uint8_t deviceId, uint32_t param); 00140 uint16_t BSP_MotorControl_CmdGetStatus(uint8_t deviceId); 00141 void BSP_MotorControl_CmdNop(uint8_t deviceId); 00142 void BSP_MotorControl_CmdSetParam(uint8_t deviceId, uint32_t param, uint32_t value); 00143 uint16_t BSP_MotorControl_ReadStatusRegister(uint8_t deviceId); 00144 void BSP_MotorControl_ReleaseReset(void) ; 00145 void BSP_MotorControl_Reset(void); 00146 void BSP_MotorControl_SelectStepMode(uint8_t deviceId, motorStepMode_t stepMod); 00147 void BSP_MotorControl_SetDirection(uint8_t deviceId, motorDir_t dir); 00148 void BSP_MotorControl_CmdGoToDir(uint8_t deviceId, motorDir_t dir, int32_t abs_pos); 00149 uint8_t BSP_MotorControl_CheckBusyHw(void); 00150 uint8_t BSP_MotorControl_CheckStatusHw(void); 00151 void BSP_MotorControl_CmdGoUntil(uint8_t deviceId, motorAction_t action, motorDir_t dir, uint32_t speed); 00152 void BSP_MotorControl_CmdHardHiZ(uint8_t deviceId); 00153 void BSP_MotorControl_CmdReleaseSw(uint8_t deviceId, motorAction_t action, motorDir_t dir); 00154 void BSP_MotorControl_CmdResetDevice(uint8_t deviceId); 00155 void BSP_MotorControl_CmdResetPos(uint8_t deviceId); 00156 void BSP_MotorControl_CmdRun(uint8_t deviceId, motorDir_t dir, uint32_t speed); 00157 void BSP_MotorControl_CmdSoftHiZ(uint8_t deviceId); 00158 void BSP_MotorControl_CmdStepClock(uint8_t deviceId, motorDir_t dir); 00159 void BSP_MotorControl_FetchAndClearAllStatus(void); 00160 uint16_t BSP_MotorControl_GetFetchedStatus(uint8_t deviceId); 00161 uint8_t BSP_MotorControl_GetNbDevices(void); 00162 bool BSP_MotorControl_IsDeviceBusy(uint8_t deviceId); 00163 void BSP_MotorControl_SendQueuedCommands(void); 00164 void BSP_MotorControl_QueueCommands(uint8_t deviceId, uint8_t param, uint32_t value); 00165 void BSP_MotorControl_WaitForAllDevicesNotBusy(void); 00166 void BSP_MotorControl_BusyInterruptHandler(void); 00167 void BSP_MotorControl_CmdSoftStop(uint8_t deviceId); 00168 /** 00169 * @} 00170 */ 00171 00172 /** 00173 * @} 00174 */ 00175 00176 /** 00177 * @} 00178 */ 00179 00180 #ifdef __cplusplus 00181 } 00182 #endif 00183 00184 #endif /* __MOTOR_CONTROL_H */ 00185 00186 00187 00188 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jul 12 2022 22:53:31 by
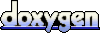