
X-CUBE-SPN1-20150128 example source code for one motor compiled under mbed. Tested OK on Nucleo F401. l6474.cpp is modified from original with defines in l6474_target_config.h to select the original behaviour (motor de-energised when halted), or new mode to continue powering with a (reduced) current in the coils (braking/position hold capability). On F401 avoid using mbed's InterruptIn on pins 10-15 (any port). Beware of other conflicts! L0 & F0 are included but untested.
BSP_MotorControl_Control_Functions
[MOTOR_CONTROL]
Functions | |
void | BSP_MotorControl_CmdDisable (uint8_t deviceId) |
Issue the Disable command to the motor driver of the specified device. | |
void | BSP_MotorControl_CmdEnable (uint8_t deviceId) |
Issues the Enable command to the motor driver of the specified device. | |
uint32_t | BSP_MotorControl_CmdGetParam (uint8_t deviceId, uint32_t param) |
Issues the GetParam command to the motor driver of the specified device. | |
uint16_t | BSP_MotorControl_CmdGetStatus (uint8_t deviceId) |
Issues the GetStatus command to the motor driver of the specified device. | |
void | BSP_MotorControl_CmdNop (uint8_t deviceId) |
Issues the Nop command to the motor driver of the specified device. | |
void | BSP_MotorControl_CmdSetParam (uint8_t deviceId, uint32_t param, uint32_t value) |
Issues the SetParam command to the motor driver of the specified device. | |
uint16_t | BSP_MotorControl_ReadStatusRegister (uint8_t deviceId) |
Reads the Status Register value. | |
void | BSP_MotorControl_ReleaseReset (void) |
Releases the motor driver (pin set to High) of all devices. | |
void | BSP_MotorControl_Reset (void) |
Resets the motor driver (reset pin set to low) of all devices. | |
void | BSP_MotorControl_SelectStepMode (uint8_t deviceId, motorStepMode_t stepMod) |
Set the stepping mode. | |
void | BSP_MotorControl_SetDirection (uint8_t deviceId, motorDir_t dir) |
Specifies the direction. | |
void | BSP_MotorControl_CmdGoToDir (uint8_t deviceId, motorDir_t dir, int32_t abs_pos) |
Issues Go To Dir command. | |
uint8_t | BSP_MotorControl_CheckBusyHw (void) |
Checks if at least one device is busy by checking busy pin position. | |
uint8_t | BSP_MotorControl_CheckStatusHw (void) |
Checks if at least one device has an alarm flag set by reading flag pin position. | |
void | BSP_MotorControl_CmdGoUntil (uint8_t deviceId, motorAction_t action, motorDir_t dir, uint32_t speed) |
Issues Go Until command. | |
void | BSP_MotorControl_CmdHardHiZ (uint8_t deviceId) |
Issues Hard HiZ command. | |
void | BSP_MotorControl_CmdReleaseSw (uint8_t deviceId, motorAction_t action, motorDir_t dir) |
Issues Release SW command. | |
void | BSP_MotorControl_CmdResetDevice (uint8_t deviceId) |
Issues Reset Device command. | |
void | BSP_MotorControl_CmdResetPos (uint8_t deviceId) |
Issues Reset Pos command. | |
void | BSP_MotorControl_CmdRun (uint8_t deviceId, motorDir_t dir, uint32_t speed) |
Issues Run command. | |
void | BSP_MotorControl_CmdSoftHiZ (uint8_t deviceId) |
Issues Soft HiZ command. | |
void | BSP_MotorControl_CmdStepClock (uint8_t deviceId, motorDir_t dir) |
Issues Step Clock command. | |
void | BSP_MotorControl_FetchAndClearAllStatus (void) |
Fetch and clear status flags of all devices by issuing a GET_STATUS command simultaneously to all devices. | |
uint16_t | BSP_MotorControl_GetFetchedStatus (uint8_t deviceId) |
Get the value of the STATUS register which was fetched by using BSP_MotorControl_FetchAndClearAllStatus. | |
uint8_t | BSP_MotorControl_GetNbDevices (void) |
Return the number of devices in the daisy chain. | |
bool | BSP_MotorControl_IsDeviceBusy (uint8_t deviceId) |
Checks if the specified device is busy by reading the Busy flag bit ot its status Register. | |
void | BSP_MotorControl_SendQueuedCommands (void) |
Sends commands stored in the queue by previously Powerstep01_QueueCommands. | |
void | BSP_MotorControl_QueueCommands (uint8_t deviceId, uint8_t param, uint32_t value) |
Put commands in queue before synchronous sending done by calling BSP_MotorControl_SendQueuedCommands. | |
void | BSP_MotorControl_WaitForAllDevicesNotBusy (void) |
Locks until all devices become not busy. | |
void | BSP_MotorControl_BusyInterruptHandler (void) |
Handler of the busy interrupt which calls the user callback (if defined) | |
void | BSP_MotorControl_CmdSoftStop (uint8_t deviceId) |
Issues PowerStep01 Soft Stop command. |
Function Documentation
void BSP_MotorControl_BusyInterruptHandler | ( | void | ) |
Handler of the busy interrupt which calls the user callback (if defined)
- Parameters:
-
None
- Return values:
-
None
Definition at line 1298 of file motorcontrol.cpp.
uint8_t BSP_MotorControl_CheckBusyHw | ( | void | ) |
Checks if at least one device is busy by checking busy pin position.
The busy pin is shared between all devices.
- Parameters:
-
None
- Return values:
-
One if at least one device is busy, otherwise zero
Definition at line 968 of file motorcontrol.cpp.
uint8_t BSP_MotorControl_CheckStatusHw | ( | void | ) |
Checks if at least one device has an alarm flag set by reading flag pin position.
The flag pin is shared between all devices.
- Parameters:
-
None
- Return values:
-
One if at least one device has an alarm flag set , otherwise zero
Definition at line 990 of file motorcontrol.cpp.
void BSP_MotorControl_CmdDisable | ( | uint8_t | deviceId ) |
Issue the Disable command to the motor driver of the specified device.
- Parameters:
-
[in] deviceId (from 0 to 2)
- Return values:
-
None
Definition at line 739 of file motorcontrol.cpp.
void BSP_MotorControl_CmdEnable | ( | uint8_t | deviceId ) |
Issues the Enable command to the motor driver of the specified device.
- Parameters:
-
[in] deviceId (from 0 to 2)
- Return values:
-
None
Definition at line 756 of file motorcontrol.cpp.
uint32_t BSP_MotorControl_CmdGetParam | ( | uint8_t | deviceId, |
uint32_t | param | ||
) |
Issues the GetParam command to the motor driver of the specified device.
- Parameters:
-
[in] deviceId (from 0 to 2) [in] param Register adress (BSP_MotorControl_ABS_POS, BSP_MotorControl_MARK,...)
- Return values:
-
Register value
Definition at line 774 of file motorcontrol.cpp.
uint16_t BSP_MotorControl_CmdGetStatus | ( | uint8_t | deviceId ) |
Issues the GetStatus command to the motor driver of the specified device.
- Parameters:
-
[in] deviceId (from 0 to 2)
- Return values:
-
Status Register value
- Note:
- Once the GetStatus command is performed, the flags of the status register are reset. This is not the case when the status register is read with the GetParam command (via the functions ReadStatusRegister or CmdGetParam).
Definition at line 797 of file motorcontrol.cpp.
void BSP_MotorControl_CmdGoToDir | ( | uint8_t | deviceId, |
motorDir_t | dir, | ||
int32_t | abs_pos | ||
) |
Issues Go To Dir command.
- Parameters:
-
[in] deviceId (from 0 to MAX_NUMBER_OF_DEVICES-1 ) [in] dir movement direction [in] abs_pos absolute position where requested to move
- Return values:
-
None
Definition at line 949 of file motorcontrol.cpp.
void BSP_MotorControl_CmdGoUntil | ( | uint8_t | deviceId, |
motorAction_t | action, | ||
motorDir_t | dir, | ||
uint32_t | speed | ||
) |
Issues Go Until command.
- Parameters:
-
[in] deviceId (from 0 to MAX_NUMBER_OF_DEVICES-1 ) [in] action ACTION_RESET or ACTION_COPY [in] dir movement direction [in] speed
- Return values:
-
None
Definition at line 1012 of file motorcontrol.cpp.
void BSP_MotorControl_CmdHardHiZ | ( | uint8_t | deviceId ) |
Issues Hard HiZ command.
- Parameters:
-
[in] deviceId (from 0 to MAX_NUMBER_OF_DEVICES-1 )
- Return values:
-
None
Definition at line 1029 of file motorcontrol.cpp.
void BSP_MotorControl_CmdNop | ( | uint8_t | deviceId ) |
Issues the Nop command to the motor driver of the specified device.
- Parameters:
-
[in] deviceId (from 0 to 2)
- Return values:
-
None
Definition at line 816 of file motorcontrol.cpp.
void BSP_MotorControl_CmdReleaseSw | ( | uint8_t | deviceId, |
motorAction_t | action, | ||
motorDir_t | dir | ||
) |
Issues Release SW command.
- Parameters:
-
[in] deviceId (from 0 to MAX_NUMBER_OF_DEVICES-1 ) [in] action [in] dir movement direction
- Return values:
-
None
Definition at line 1048 of file motorcontrol.cpp.
void BSP_MotorControl_CmdResetDevice | ( | uint8_t | deviceId ) |
Issues Reset Device command.
- Parameters:
-
[in] deviceId (from 0 to MAX_NUMBER_OF_DEVICES-1 )
- Return values:
-
None
Definition at line 1065 of file motorcontrol.cpp.
void BSP_MotorControl_CmdResetPos | ( | uint8_t | deviceId ) |
Issues Reset Pos command.
- Parameters:
-
[in] deviceId (from 0 to MAX_NUMBER_OF_DEVICES-1 )
- Return values:
-
None
Definition at line 1082 of file motorcontrol.cpp.
void BSP_MotorControl_CmdRun | ( | uint8_t | deviceId, |
motorDir_t | dir, | ||
uint32_t | speed | ||
) |
Issues Run command.
- Parameters:
-
[in] deviceId (from 0 to MAX_NUMBER_OF_DEVICES-1 ) [in] dir Movement direction (FORWARD, BACKWARD) [in] speed in steps/s
- Return values:
-
None
Definition at line 1101 of file motorcontrol.cpp.
void BSP_MotorControl_CmdSetParam | ( | uint8_t | deviceId, |
uint32_t | param, | ||
uint32_t | value | ||
) |
Issues the SetParam command to the motor driver of the specified device.
- Parameters:
-
[in] deviceId (from 0 to 2) [in] param Register adress (BSP_MotorControl_ABS_POS, BSP_MotorControl_MARK,...) [in] value Value to set in the register
- Return values:
-
None
Definition at line 835 of file motorcontrol.cpp.
void BSP_MotorControl_CmdSoftHiZ | ( | uint8_t | deviceId ) |
Issues Soft HiZ command.
- Parameters:
-
[in] deviceId (from 0 to MAX_NUMBER_OF_DEVICES-1 )
- Return values:
-
None
Definition at line 1118 of file motorcontrol.cpp.
void BSP_MotorControl_CmdSoftStop | ( | uint8_t | deviceId ) |
Issues PowerStep01 Soft Stop command.
- Parameters:
-
[in] deviceId (from 0 to MAX_NUMBER_OF_DEVICES-1 )
- Return values:
-
None
Definition at line 1315 of file motorcontrol.cpp.
void BSP_MotorControl_CmdStepClock | ( | uint8_t | deviceId, |
motorDir_t | dir | ||
) |
Issues Step Clock command.
- Parameters:
-
[in] deviceId (from 0 to MAX_NUMBER_OF_DEVICES-1 ) [in] dir Movement direction (FORWARD, BACKWARD)
- Return values:
-
None
Definition at line 1136 of file motorcontrol.cpp.
void BSP_MotorControl_FetchAndClearAllStatus | ( | void | ) |
Fetch and clear status flags of all devices by issuing a GET_STATUS command simultaneously to all devices.
Then, the fetched status of each device can be retrieved by using the BSP_MotorControl_GetFetchedStatus function provided there is no other calls to functions which use the SPI in between.
- Parameters:
-
None
- Return values:
-
None
Definition at line 1159 of file motorcontrol.cpp.
uint16_t BSP_MotorControl_GetFetchedStatus | ( | uint8_t | deviceId ) |
Get the value of the STATUS register which was fetched by using BSP_MotorControl_FetchAndClearAllStatus.
The fetched values are available as long as there no other calls to functions which use the SPI.
- Parameters:
-
[in] deviceId (from 0 to MAX_NUMBER_OF_DEVICES-1 )
- Return values:
-
Last fetched value of the STATUS register
Definition at line 1179 of file motorcontrol.cpp.
uint8_t BSP_MotorControl_GetNbDevices | ( | void | ) |
Return the number of devices in the daisy chain.
- Parameters:
-
None
- Return values:
-
number of devices from 1 to MAX_NUMBER_OF_DEVICES
Definition at line 1198 of file motorcontrol.cpp.
bool BSP_MotorControl_IsDeviceBusy | ( | uint8_t | deviceId ) |
Checks if the specified device is busy by reading the Busy flag bit ot its status Register.
- Parameters:
-
[in] deviceId (from 0 to MAX_NUMBER_OF_DEVICES-1 )
- Return values:
-
true if device is busy, false zero
Definition at line 1218 of file motorcontrol.cpp.
void BSP_MotorControl_QueueCommands | ( | uint8_t | deviceId, |
uint8_t | param, | ||
uint32_t | value | ||
) |
Put commands in queue before synchronous sending done by calling BSP_MotorControl_SendQueuedCommands.
Any call to functions that use the SPI between the calls of BSP_MotorControl_QueueCommands and BSP_MotorControl_SendQueuedCommands will corrupt the queue. A command for each device of the daisy chain must be specified before calling BSP_MotorControl_SendQueuedCommands.
- Parameters:
-
[in] deviceId deviceId (from 0 to MAX_NUMBER_OF_DEVICES-1 ) [in] param Command to queue (all BSP_MotorControl commmands except SET_PARAM, GET_PARAM, GET_STATUS) [in] value argument of the command to queue
- Return values:
-
None
Definition at line 1264 of file motorcontrol.cpp.
uint16_t BSP_MotorControl_ReadStatusRegister | ( | uint8_t | deviceId ) |
Reads the Status Register value.
- Parameters:
-
[in] deviceId (from 0 to 2)
- Return values:
-
Status register valued
- Note:
- The status register flags are not cleared at the difference with CmdGetStatus()
Definition at line 856 of file motorcontrol.cpp.
void BSP_MotorControl_ReleaseReset | ( | void | ) |
Releases the motor driver (pin set to High) of all devices.
- Parameters:
-
None
- Return values:
-
None
Definition at line 875 of file motorcontrol.cpp.
void BSP_MotorControl_Reset | ( | void | ) |
Resets the motor driver (reset pin set to low) of all devices.
- Parameters:
-
None
- Return values:
-
None
Definition at line 892 of file motorcontrol.cpp.
void BSP_MotorControl_SelectStepMode | ( | uint8_t | deviceId, |
motorStepMode_t | stepMod | ||
) |
Set the stepping mode.
- Parameters:
-
[in] deviceId (from 0 to 2) [in] stepMod from full step to 1/16 microstep as specified in enum BSP_MotorControl_STEP_SEL_t
- Return values:
-
None
Definition at line 910 of file motorcontrol.cpp.
void BSP_MotorControl_SendQueuedCommands | ( | void | ) |
Sends commands stored in the queue by previously Powerstep01_QueueCommands.
- Parameters:
-
None
- Return values:
-
None
Definition at line 1238 of file motorcontrol.cpp.
void BSP_MotorControl_SetDirection | ( | uint8_t | deviceId, |
motorDir_t | dir | ||
) |
Specifies the direction.
- Parameters:
-
[in] deviceId (from 0 to 2) [in] dir FORWARD or BACKWARD
- Note:
- The direction change is only applied if the device is in INACTIVE state
- Return values:
-
None
Definition at line 930 of file motorcontrol.cpp.
void BSP_MotorControl_WaitForAllDevicesNotBusy | ( | void | ) |
Locks until all devices become not busy.
- Parameters:
-
None
- Return values:
-
None
Definition at line 1281 of file motorcontrol.cpp.
Generated on Tue Jul 12 2022 22:53:31 by
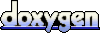