
X-CUBE-SPN1-20150128 example source code for one motor compiled under mbed. Tested OK on Nucleo F401. l6474.cpp is modified from original with defines in l6474_target_config.h to select the original behaviour (motor de-energised when halted), or new mode to continue powering with a (reduced) current in the coils (braking/position hold capability). On F401 avoid using mbed's InterruptIn on pins 10-15 (any port). Beware of other conflicts! L0 & F0 are included but untested.
Functions | |
void | L6474_AttachErrorHandler (void(*callback)(uint16_t)) |
Attaches a user callback to the error Handler. | |
void | L6474_AttachFlagInterrupt (void(*callback)(void)) |
Attaches a user callback to the flag Interrupt The call back will be then called each time the status flag pin will be pulled down due to the occurrence of a programmed alarms ( OCD, thermal pre-warning or shutdown, UVLO, wrong command, non-performable command) | |
void | L6474_Init (uint8_t nbDevices) |
Starts the L6474 library. | |
uint16_t | L6474_GetAcceleration (uint8_t deviceId) |
Returns the acceleration of the specified device. | |
uint16_t | L6474_GetCurrentSpeed (uint8_t deviceId) |
Returns the current speed of the specified device. | |
uint16_t | L6474_GetDeceleration (uint8_t deviceId) |
Returns the deceleration of the specified device. | |
motorState_t | L6474_GetDeviceState (uint8_t deviceId) |
Returns the device state. | |
uint8_t | L6474_GetFwVersion (void) |
Returns the FW version of the library. | |
motorDrv_t * | L6474_GetMotorHandle (void) |
Return motor handle (pointer to the L6474 motor driver structure) | |
int32_t | L6474_GetMark (uint8_t deviceId) |
Returns the mark position of the specified device. | |
uint16_t | L6474_GetMaxSpeed (uint8_t deviceId) |
Returns the max speed of the specified device. | |
uint16_t | L6474_GetMinSpeed (uint8_t deviceId) |
Returns the min speed of the specified device. | |
int32_t | L6474_GetPosition (uint8_t deviceId) |
Returns the ABS_POSITION of the specified device. | |
void | L6474_GoHome (uint8_t deviceId) |
Requests the motor to move to the home position (ABS_POSITION = 0) | |
void | L6474_GoMark (uint8_t deviceId) |
Requests the motor to move to the mark position. | |
void | L6474_GoTo (uint8_t deviceId, int32_t targetPosition) |
Requests the motor to move to the specified position. | |
void | L6474_HardStop (uint8_t deviceId) |
Immediatly stops the motor and disable the power bridge. | |
void | L6474_Move (uint8_t deviceId, motorDir_t direction, uint32_t stepCount) |
Moves the motor of the specified number of steps. | |
uint16_t | L6474_ReadId (void) |
Read id. | |
void | L6474_ResetAllDevices (void) |
Resets all L6474 devices. | |
void | L6474_Run (uint8_t deviceId, motorDir_t direction) |
Runs the motor. | |
bool | L6474_SetAcceleration (uint8_t deviceId, uint16_t newAcc) |
Changes the acceleration of the specified device. | |
bool | L6474_SetDeceleration (uint8_t deviceId, uint16_t newDec) |
Changes the deceleration of the specified device. | |
void | L6474_SetHome (uint8_t deviceId) |
Set current position to be the Home position (ABS pos set to 0) | |
void | L6474_SetMark (uint8_t deviceId) |
Sets current position to be the Mark position. | |
bool | L6474_SetMaxSpeed (uint8_t deviceId, uint16_t newMaxSpeed) |
Changes the max speed of the specified device. | |
bool | L6474_SetMinSpeed (uint8_t deviceId, uint16_t newMinSpeed) |
Changes the min speed of the specified device. | |
bool | L6474_SoftStop (uint8_t deviceId) |
Stops the motor by using the device deceleration. | |
void | L6474_WaitWhileActive (uint8_t deviceId) |
Locks until the device state becomes Inactive. |
Function Documentation
void L6474_AttachErrorHandler | ( | void(*)(uint16_t) | callback ) |
void L6474_AttachFlagInterrupt | ( | void(*)(void) | callback ) |
Attaches a user callback to the flag Interrupt The call back will be then called each time the status flag pin will be pulled down due to the occurrence of a programmed alarms ( OCD, thermal pre-warning or shutdown, UVLO, wrong command, non-performable command)
- Parameters:
-
[in] callback Name of the callback to attach to the Flag Interrupt
- Return values:
-
None
uint16_t L6474_GetAcceleration | ( | uint8_t | deviceId ) |
uint16_t L6474_GetCurrentSpeed | ( | uint8_t | deviceId ) |
uint16_t L6474_GetDeceleration | ( | uint8_t | deviceId ) |
motorState_t L6474_GetDeviceState | ( | uint8_t | deviceId ) |
uint8_t L6474_GetFwVersion | ( | void | ) |
int32_t L6474_GetMark | ( | uint8_t | deviceId ) |
uint16_t L6474_GetMaxSpeed | ( | uint8_t | deviceId ) |
uint16_t L6474_GetMinSpeed | ( | uint8_t | deviceId ) |
motorDrv_t* L6474_GetMotorHandle | ( | void | ) |
int32_t L6474_GetPosition | ( | uint8_t | deviceId ) |
void L6474_GoHome | ( | uint8_t | deviceId ) |
void L6474_GoMark | ( | uint8_t | deviceId ) |
void L6474_GoTo | ( | uint8_t | deviceId, |
int32_t | targetPosition | ||
) |
void L6474_HardStop | ( | uint8_t | deviceId ) |
void L6474_Init | ( | uint8_t | nbDevices ) |
void L6474_Move | ( | uint8_t | deviceId, |
motorDir_t | direction, | ||
uint32_t | stepCount | ||
) |
uint16_t L6474_ReadId | ( | void | ) |
void L6474_ResetAllDevices | ( | void | ) |
void L6474_Run | ( | uint8_t | deviceId, |
motorDir_t | direction | ||
) |
bool L6474_SetAcceleration | ( | uint8_t | deviceId, |
uint16_t | newAcc | ||
) |
Changes the acceleration of the specified device.
- Parameters:
-
[in] deviceId (from 0 to 2) [in] newAcc New acceleration to apply in pps^2
- Return values:
-
true if the command is successfully executed, else false
- Note:
- The command is not performed is the device is executing a MOVE or GOTO command (but it can be used during a RUN command)
bool L6474_SetDeceleration | ( | uint8_t | deviceId, |
uint16_t | newDec | ||
) |
Changes the deceleration of the specified device.
- Parameters:
-
[in] deviceId (from 0 to 2) [in] newDec New deceleration to apply in pps^2
- Return values:
-
true if the command is successfully executed, else false
- Note:
- The command is not performed is the device is executing a MOVE or GOTO command (but it can be used during a RUN command)
void L6474_SetHome | ( | uint8_t | deviceId ) |
void L6474_SetMark | ( | uint8_t | deviceId ) |
bool L6474_SetMaxSpeed | ( | uint8_t | deviceId, |
uint16_t | newMaxSpeed | ||
) |
Changes the max speed of the specified device.
- Parameters:
-
[in] deviceId (from 0 to 2) [in] newMaxSpeed New max speed to apply in pps
- Return values:
-
true if the command is successfully executed, else false
- Note:
- The command is not performed is the device is executing a MOVE or GOTO command (but it can be used during a RUN command).
bool L6474_SetMinSpeed | ( | uint8_t | deviceId, |
uint16_t | newMinSpeed | ||
) |
Changes the min speed of the specified device.
- Parameters:
-
[in] deviceId (from 0 to 2) [in] newMinSpeed New min speed to apply in pps
- Return values:
-
true if the command is successfully executed, else false
- Note:
- The command is not performed is the device is executing a MOVE or GOTO command (but it can be used during a RUN command).
bool L6474_SoftStop | ( | uint8_t | deviceId ) |
Generated on Tue Jul 12 2022 22:53:31 by
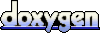