
X-CUBE-SPN1-20150128 example source code for one motor compiled under mbed. Tested OK on Nucleo F401. l6474.cpp is modified from original with defines in l6474_target_config.h to select the original behaviour (motor de-energised when halted), or new mode to continue powering with a (reduced) current in the coils (braking/position hold capability). On F401 avoid using mbed's InterruptIn on pins 10-15 (any port). Beware of other conflicts! L0 & F0 are included but untested.
MOTOR_CONTROL_Private_Functions
[MOTOR_CONTROL]
Functions | |
void | BSP_MotorControl_AttachErrorHandler (void(*callback)(uint16_t)) |
Attaches a user callback to the error Handler. | |
void | BSP_MotorControl_AttachFlagInterrupt (void(*callback)(void)) |
Attaches a user callback to the Flag interrupt Handler. | |
void | BSP_MotorControl_AttachBusyInterrupt (void(*callback)(void)) |
Attaches a user callback to the Busy interrupt Handler. | |
void | BSP_MotorControl_ErrorHandler (uint16_t error) |
Motor control error handler. | |
void | BSP_MotorControl_Init (uint16_t id, uint8_t nbDevices) |
Initialises the motor driver. | |
void | BSP_MotorControl_FlagInterruptHandler (void) |
Handlers of the flag interrupt which calls the user callback (if defined) | |
uint16_t | BSP_MotorControl_GetAcceleration (uint8_t deviceId) |
Returns the acceleration of the specified device. | |
uint16_t | BSP_MotorControl_GetBoardId (void) |
Get board Id the motor driver. | |
uint16_t | BSP_MotorControl_GetCurrentSpeed (uint8_t deviceId) |
Returns the current speed of the specified device. | |
uint16_t | BSP_MotorControl_GetDeceleration (uint8_t deviceId) |
Returns the deceleration of the specified device. | |
motorState_t | BSP_MotorControl_GetDeviceState (uint8_t deviceId) |
Returns the device state. | |
uint8_t | BSP_MotorControl_GetFwVersion (void) |
Returns the FW version of the library. | |
int32_t | BSP_MotorControl_GetMark (uint8_t deviceId) |
Returns the mark position of the specified device. | |
uint16_t | BSP_MotorControl_GetMaxSpeed (uint8_t deviceId) |
Returns the max speed of the specified device. | |
uint16_t | BSP_MotorControl_GetMinSpeed (uint8_t deviceId) |
Returns the min speed of the specified device. | |
int32_t | BSP_MotorControl_GetPosition (uint8_t deviceId) |
Returns the ABS_POSITION of the specified device. | |
void | BSP_MotorControl_GoHome (uint8_t deviceId) |
Requests the motor to move to the home position (ABS_POSITION = 0) | |
void | BSP_MotorControl_GoMark (uint8_t deviceId) |
Requests the motor to move to the mark position. | |
void | BSP_MotorControl_GoTo (uint8_t deviceId, int32_t targetPosition) |
Requests the motor to move to the specified position. | |
void | BSP_MotorControl_HardStop (uint8_t deviceId) |
Immediatly stops the motor and disable the power bridge. | |
void | BSP_MotorControl_Move (uint8_t deviceId, motorDir_t direction, uint32_t stepCount) |
Moves the motor of the specified number of steps. | |
void | BSP_MotorControl_ResetAllDevices (void) |
Resets all motor driver devices. | |
void | BSP_MotorControl_Run (uint8_t deviceId, motorDir_t direction) |
Runs the motor. | |
bool | BSP_MotorControl_SetAcceleration (uint8_t deviceId, uint16_t newAcc) |
Changes the acceleration of the specified device. | |
bool | BSP_MotorControl_SetDeceleration (uint8_t deviceId, uint16_t newDec) |
Changes the deceleration of the specified device. | |
void | BSP_MotorControl_SetHome (uint8_t deviceId) |
Set current position to be the Home position (ABS pos set to 0) | |
void | BSP_MotorControl_SetMark (uint8_t deviceId) |
Sets current position to be the Mark position. | |
bool | BSP_MotorControl_SetMaxSpeed (uint8_t deviceId, uint16_t newMaxSpeed) |
Changes the max speed of the specified device. | |
bool | BSP_MotorControl_SetMinSpeed (uint8_t deviceId, uint16_t newMinSpeed) |
Changes the min speed of the specified device. | |
bool | BSP_MotorControl_SoftStop (uint8_t deviceId) |
Stops the motor by using the device deceleration. | |
void | BSP_MotorControl_StepClockHandler (uint8_t deviceId) |
Handles the device state machine at each ste. | |
void | BSP_MotorControl_WaitWhileActive (uint8_t deviceId) |
Locks until the device state becomes Inactive. |
Function Documentation
void BSP_MotorControl_AttachBusyInterrupt | ( | void(*)(void) | callback ) |
Attaches a user callback to the Busy interrupt Handler.
The call back will be then called each time the library detects a BUSY signal falling edge.
- Parameters:
-
[in] callback Name of the callback to attach to the Busy interrupt Hanlder
- Return values:
-
None
Definition at line 159 of file motorcontrol.cpp.
void BSP_MotorControl_AttachErrorHandler | ( | void(*)(uint16_t) | callback ) |
Attaches a user callback to the error Handler.
The call back will be then called each time the library detects an error
- Parameters:
-
[in] callback Name of the callback to attach to the error Hanlder
- Return values:
-
None
Definition at line 119 of file motorcontrol.cpp.
void BSP_MotorControl_AttachFlagInterrupt | ( | void(*)(void) | callback ) |
Attaches a user callback to the Flag interrupt Handler.
The call back will be then called each time the library detects a FLAG signal falling edge.
- Parameters:
-
[in] callback Name of the callback to attach to the Flag interrupt Hanlder
- Return values:
-
None
Definition at line 139 of file motorcontrol.cpp.
void BSP_MotorControl_ErrorHandler | ( | uint16_t | error ) |
Motor control error handler.
- Parameters:
-
[in] error number of the error
- Return values:
-
None
Definition at line 176 of file motorcontrol.cpp.
void BSP_MotorControl_FlagInterruptHandler | ( | void | ) |
Handlers of the flag interrupt which calls the user callback (if defined)
- Parameters:
-
None
- Return values:
-
None
Definition at line 228 of file motorcontrol.cpp.
uint16_t BSP_MotorControl_GetAcceleration | ( | uint8_t | deviceId ) |
Returns the acceleration of the specified device.
- Parameters:
-
[in] deviceId (from 0 to 2)
- Return values:
-
Acceleration in pps^2
Definition at line 244 of file motorcontrol.cpp.
uint16_t BSP_MotorControl_GetBoardId | ( | void | ) |
Get board Id the motor driver.
- Parameters:
-
None
- Return values:
-
Motor control board Id
Definition at line 264 of file motorcontrol.cpp.
uint16_t BSP_MotorControl_GetCurrentSpeed | ( | uint8_t | deviceId ) |
Returns the current speed of the specified device.
- Parameters:
-
[in] deviceId (from 0 to 2)
- Return values:
-
Speed in pps
Definition at line 273 of file motorcontrol.cpp.
uint16_t BSP_MotorControl_GetDeceleration | ( | uint8_t | deviceId ) |
Returns the deceleration of the specified device.
- Parameters:
-
[in] deviceId (from 0 to 2)
- Return values:
-
Deceleration in pps^2
Definition at line 292 of file motorcontrol.cpp.
motorState_t BSP_MotorControl_GetDeviceState | ( | uint8_t | deviceId ) |
Returns the device state.
- Parameters:
-
[in] deviceId (from 0 to 2)
- Return values:
-
State (ACCELERATING, DECELERATING, STEADY or INACTIVE)
Definition at line 312 of file motorcontrol.cpp.
uint8_t BSP_MotorControl_GetFwVersion | ( | void | ) |
Returns the FW version of the library.
- Parameters:
-
None
- Return values:
-
BSP_MotorControl_FW_VERSION
Definition at line 332 of file motorcontrol.cpp.
int32_t BSP_MotorControl_GetMark | ( | uint8_t | deviceId ) |
Returns the mark position of the specified device.
- Parameters:
-
[in] deviceId (from 0 to 2)
- Return values:
-
Mark register value converted in a 32b signed integer
Definition at line 352 of file motorcontrol.cpp.
uint16_t BSP_MotorControl_GetMaxSpeed | ( | uint8_t | deviceId ) |
Returns the max speed of the specified device.
- Parameters:
-
[in] deviceId (from 0 to 2)
- Return values:
-
maxSpeed in pps
Definition at line 372 of file motorcontrol.cpp.
uint16_t BSP_MotorControl_GetMinSpeed | ( | uint8_t | deviceId ) |
Returns the min speed of the specified device.
- Parameters:
-
[in] deviceId (from 0 to 2)
- Return values:
-
minSpeed in pps
Definition at line 391 of file motorcontrol.cpp.
int32_t BSP_MotorControl_GetPosition | ( | uint8_t | deviceId ) |
Returns the ABS_POSITION of the specified device.
- Parameters:
-
[in] deviceId (from 0 to 2)
- Return values:
-
ABS_POSITION register value converted in a 32b signed integer
Definition at line 411 of file motorcontrol.cpp.
void BSP_MotorControl_GoHome | ( | uint8_t | deviceId ) |
Requests the motor to move to the home position (ABS_POSITION = 0)
- Parameters:
-
[in] deviceId (from 0 to 2)
- Return values:
-
None
Definition at line 431 of file motorcontrol.cpp.
void BSP_MotorControl_GoMark | ( | uint8_t | deviceId ) |
Requests the motor to move to the mark position.
- Parameters:
-
[in] deviceId (from 0 to 2)
- Return values:
-
None
Definition at line 448 of file motorcontrol.cpp.
void BSP_MotorControl_GoTo | ( | uint8_t | deviceId, |
int32_t | targetPosition | ||
) |
Requests the motor to move to the specified position.
- Parameters:
-
[in] deviceId (from 0 to 2) [in] targetPosition absolute position in steps
- Return values:
-
None
Definition at line 466 of file motorcontrol.cpp.
void BSP_MotorControl_HardStop | ( | uint8_t | deviceId ) |
Immediatly stops the motor and disable the power bridge.
- Parameters:
-
[in] deviceId (from 0 to 2)
- Return values:
-
None
Definition at line 483 of file motorcontrol.cpp.
void BSP_MotorControl_Init | ( | uint16_t | id, |
uint8_t | nbDevices | ||
) |
Initialises the motor driver.
- Parameters:
-
[in] id Component Id (L6474, Powerstep01,...) [in] nbDevices Number of motor devices to use (from 1 to 3)
- Return values:
-
None
Definition at line 196 of file motorcontrol.cpp.
void BSP_MotorControl_Move | ( | uint8_t | deviceId, |
motorDir_t | direction, | ||
uint32_t | stepCount | ||
) |
Moves the motor of the specified number of steps.
- Parameters:
-
[in] deviceId (from 0 to 2) [in] direction FORWARD or BACKWARD [in] stepCount Number of steps to perform
- Return values:
-
None
Definition at line 502 of file motorcontrol.cpp.
void BSP_MotorControl_ResetAllDevices | ( | void | ) |
Resets all motor driver devices.
- Parameters:
-
None
- Return values:
-
None
Definition at line 519 of file motorcontrol.cpp.
void BSP_MotorControl_Run | ( | uint8_t | deviceId, |
motorDir_t | direction | ||
) |
Runs the motor.
It will accelerate from the min speed up to the max speed by using the device acceleration.
- Parameters:
-
[in] deviceId (from 0 to 2) [in] direction FORWARD or BACKWARD
- Return values:
-
None
Definition at line 538 of file motorcontrol.cpp.
bool BSP_MotorControl_SetAcceleration | ( | uint8_t | deviceId, |
uint16_t | newAcc | ||
) |
Changes the acceleration of the specified device.
- Parameters:
-
[in] deviceId (from 0 to 2) [in] newAcc New acceleration to apply in pps^2
- Return values:
-
true if the command is successfully executed, else false
- Note:
- The command is not performed is the device is executing a MOVE or GOTO command (but it can be used during a RUN command)
Definition at line 557 of file motorcontrol.cpp.
bool BSP_MotorControl_SetDeceleration | ( | uint8_t | deviceId, |
uint16_t | newDec | ||
) |
Changes the deceleration of the specified device.
- Parameters:
-
[in] deviceId (from 0 to 2) [in] newDec New deceleration to apply in pps^2
- Return values:
-
true if the command is successfully executed, else false
- Note:
- The command is not performed is the device is executing a MOVE or GOTO command (but it can be used during a RUN command)
Definition at line 579 of file motorcontrol.cpp.
void BSP_MotorControl_SetHome | ( | uint8_t | deviceId ) |
Set current position to be the Home position (ABS pos set to 0)
- Parameters:
-
[in] deviceId (from 0 to 2)
- Return values:
-
None
Definition at line 598 of file motorcontrol.cpp.
void BSP_MotorControl_SetMark | ( | uint8_t | deviceId ) |
Sets current position to be the Mark position.
- Parameters:
-
[in] deviceId (from 0 to 2)
- Return values:
-
None
Definition at line 615 of file motorcontrol.cpp.
bool BSP_MotorControl_SetMaxSpeed | ( | uint8_t | deviceId, |
uint16_t | newMaxSpeed | ||
) |
Changes the max speed of the specified device.
- Parameters:
-
[in] deviceId (from 0 to 2) [in] newMaxSpeed New max speed to apply in pps
- Return values:
-
true if the command is successfully executed, else false
- Note:
- The command is not performed is the device is executing a MOVE or GOTO command (but it can be used during a RUN command).
Definition at line 635 of file motorcontrol.cpp.
bool BSP_MotorControl_SetMinSpeed | ( | uint8_t | deviceId, |
uint16_t | newMinSpeed | ||
) |
Changes the min speed of the specified device.
- Parameters:
-
[in] deviceId (from 0 to 2) [in] newMinSpeed New min speed to apply in pps
- Return values:
-
true if the command is successfully executed, else false
- Note:
- The command is not performed is the device is executing a MOVE or GOTO command (but it can be used during a RUN command).
Definition at line 657 of file motorcontrol.cpp.
bool BSP_MotorControl_SoftStop | ( | uint8_t | deviceId ) |
Stops the motor by using the device deceleration.
- Parameters:
-
[in] deviceId (from 0 to 2)
- Return values:
-
true if the command is successfully executed, else false
- Note:
- The command is not performed is the device is in INACTIVE state.
Definition at line 678 of file motorcontrol.cpp.
void BSP_MotorControl_StepClockHandler | ( | uint8_t | deviceId ) |
Handles the device state machine at each ste.
- Parameters:
-
[in] deviceId (from 0 to 2)
- Return values:
-
None
- Note:
- Must only be called by the timer ISR
Definition at line 698 of file motorcontrol.cpp.
void BSP_MotorControl_WaitWhileActive | ( | uint8_t | deviceId ) |
Locks until the device state becomes Inactive.
- Parameters:
-
[in] deviceId (from 0 to 2)
- Return values:
-
None
Definition at line 714 of file motorcontrol.cpp.
Generated on Tue Jul 12 2022 22:53:31 by
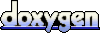