KL25Z Comparator library
Dependents: ComparatorIn_demo TEMT6200_demo 05_comparator_demo 05_comparator_demo ... more
ComparatorIn Class Reference
Class to use KL25Z Comparator. More...
#include <ComparatorIn.h>
Public Member Functions | |
ComparatorIn (PinName pinP, PinName pinM) | |
Create a ComparatorIn, connected to the specified pins. | |
void | FilterCount (unsigned char fico) |
Set the number of consecutive threshold samples. | |
void | hysteresis (unsigned char hyst) |
Set the hysteresis. | |
void | SampleMode (unsigned char samp_en) |
Sampling mode control. | |
void | WindowMode (unsigned char win_en) |
Windowing mode control. | |
void | TrigMode (unsigned char trig_en) |
Trigger mode control. | |
void | PowerMode (unsigned char pmode) |
Power mode control. | |
void | invert (unsigned char inv) |
Invert mode control. | |
void | OutputSelect (unsigned char cos) |
Comparator Output Select. | |
void | OutputPin (PinName ope) |
Connect the comparator Output Pin to an external pin. | |
void | enable (unsigned char en) |
Comparator Module control. | |
void | FilterPeriod (unsigned char fipe) |
Set the filter sample period. | |
void | dma (unsigned char dmaen) |
DMA Control. | |
unsigned char | status (void) |
Analog Comparator Output. | |
void | dac (unsigned char den) |
DAC Control. | |
void | RefSource (unsigned char res) |
Supply Voltage Reference Source Select. | |
void | treshold (float vo_pct) |
Set the detection threshold level (DAC Output Voltage Select). | |
void | PassThrough (unsigned char ptm) |
Pass Through Mode Control. | |
void | SwitchPlus (unsigned char pinP) |
Plus Input Mux Control. | |
void | SwitchMin (unsigned char pinM) |
Minus Input Mux Control. | |
void | rising (void(*fptr)(void)) |
Comparator rising interrupt callback. | |
void | falling (void(*fptr)(void)) |
Comparator falling interrupt callback. |
Detailed Description
Class to use KL25Z Comparator.
Definition at line 52 of file ComparatorIn.h.
Constructor & Destructor Documentation
ComparatorIn | ( | PinName | pinP, |
PinName | pinM | ||
) |
Create a ComparatorIn, connected to the specified pins.
- Parameters:
-
pinP = positive ComparatorIn pin to connect to pinM = negative ComparatorIn pin to connect to
- Note:
- Valid values for pinP/pinM:
PTC6, PTC7, PTC8, PTC9, PTE30, PTE29, NC
Special cases:
NC the corresponding input is connected to the internal 6-bit DAC0
PTE30 PTE30 is set as 12-bit DAC0 output and connected to IN4
- Returns:
- none
Definition at line 27 of file ComparatorIn.cpp.
Member Function Documentation
void dac | ( | unsigned char | den ) |
DAC Control.
Used to switch the internal DAC on/off. When the DAC is disabled, it is powered down to conserve power.
0 DAC is disabled.
1 DAC is enabled.
- Parameters:
-
input Unsigned char - 0 or 1
- Returns:
- none
Definition at line 163 of file ComparatorIn.cpp.
void dma | ( | unsigned char | dmaen ) |
DMA Control.
Used to switch the DMA transfer triggered from the CMP module on/off.
When this field is set, a DMA request is asserted when CFR or CFF is set.
0 DMA is disabled.
1 DMA is enabled.
- Parameters:
-
input Unsigned char - (0 = disable, 1 = enable)
- Returns:
- none
Definition at line 152 of file ComparatorIn.cpp.
void enable | ( | unsigned char | en ) |
Comparator Module control.
Used to switch the Analog Comparator module on/off. When the module is not enabled,
it remains in the off state, and consumes no power. When the user selects the same
input from analog mux to the positive and negative port, the comparator is disabled automatically.
0 Analog Comparator is disabled.
1 Analog Comparator is enabled.
- Parameters:
-
input Unsigned char - (0 = disable, 1 = enable)
- Returns:
- none
Definition at line 141 of file ComparatorIn.cpp.
void falling | ( | void(*)(void) | fptr ) |
Comparator falling interrupt callback.
- Parameters:
-
pointer to the user function to execute after IRQ assertion NULL to disable the interrupt
- Returns:
- none
- Note:
- The interrupt is automatically enabled when a valid pointer is used.
The interrupt is automatically disabled when both risng and falling are set to NULL.
Definition at line 232 of file ComparatorIn.cpp.
void FilterCount | ( | unsigned char | fico ) |
Set the number of consecutive threshold samples.
Represents the number of consecutive samples that must agree
prior to the comparator ouput filter accepting a new output state.
- Parameters:
-
input Unsigned char - range : 1..7
- Returns:
- none
Definition at line 59 of file ComparatorIn.cpp.
void FilterPeriod | ( | unsigned char | fipe ) |
Set the filter sample period.
Specifies the sampling period, in bus clock cycles, of the comparator
output filter, when CR1[SE]=0. Setting FILT_PER to 0x0 disables the filter.
- Parameters:
-
input Unsigned char - range : 0..255
- Returns:
- none
Definition at line 147 of file ComparatorIn.cpp.
void hysteresis | ( | unsigned char | hyst ) |
Set the hysteresis.
0 : 5mV
1 : 10mV
2 : 20mV
3 : 30mV
- Parameters:
-
input Unsigned char
- Returns:
- none
Definition at line 69 of file ComparatorIn.cpp.
void invert | ( | unsigned char | inv ) |
Invert mode control.
Allows selection of the polarity of the analog comparator function.
It is also driven to the COUT output, on both the device pin and as SCR[COUT], when OPE=0.
0 Does not invert the comparator output.
1 Inverts the comparator output.
- Parameters:
-
input Unsigned char - (0 = not inverted, 1 = inverted)
- Returns:
- none
Definition at line 109 of file ComparatorIn.cpp.
void OutputPin | ( | PinName | ope ) |
Connect the comparator Output Pin to an external pin.
Only one pin can be connected at any time.
Each time this function is called, the last active pin will be disabled before the new pin is enabled.
- Parameters:
-
input NC disconnect CMPO from the associated CMPO output pin. input PTC0 connect CMPO to PTC0. input PTC5 connect CMPO to PTC5. input PTE0 connect CMPO to PTE0.
Definition at line 121 of file ComparatorIn.cpp.
void OutputSelect | ( | unsigned char | cos ) |
Comparator Output Select.
0 Set the filtered comparator output (CMPO) to equal COUT.
1 Set the unfiltered comparator output (CMPO) to equal COUTA.
- Parameters:
-
input Unsigned char - (0 : CMPO = COUT, 1 : CMPO = COUTA)
- Returns:
- none
Definition at line 115 of file ComparatorIn.cpp.
void PassThrough | ( | unsigned char | ptm ) |
Pass Through Mode Control.
Set the MUX pass through mode. Pass through mode is always available, but for
some devices, this feature must be always disabled due to the lack of package pins.
0 Pass Through Mode is disabled.
1 Pass Through Mode is enabled.
- Parameters:
-
input Unsigned char - (0 = disable, 1 = enable)
- Returns:
- none
Definition at line 191 of file ComparatorIn.cpp.
void PowerMode | ( | unsigned char | pmode ) |
Power mode control.
0 Low-Speed (LS) Comparison mode selected. In this mode, CMP has
slower output propagation delay and lower current consumption.
1 High-Speed (HS) Comparison mode selected. In this mode, CMP has
faster output propagation delay and higher current consumption.
- Parameters:
-
input Unsigned char - (0 = Low-Speed, 1 = high-Speed)
- Returns:
- none
Definition at line 103 of file ComparatorIn.cpp.
void RefSource | ( | unsigned char | res ) |
Supply Voltage Reference Source Select.
0 - V is selected as resistor ladder network supply reference Vin1 = VREFH
1 - V is selected as resistor ladder network supply reference Vin2 = VDD
(Use this option for the best ADC operation).
- Parameters:
-
input Unsigned char - 0 or 1
- Returns:
- none
Definition at line 169 of file ComparatorIn.cpp.
void rising | ( | void(*)(void) | fptr ) |
Comparator rising interrupt callback.
- Parameters:
-
pointer to the user function to execute after IRQ assertion NULL to disable the interrupt
- Returns:
- none
- Note:
- The interrupt is automatically enabled when a valid pointer is used.
The interrupt is automatically disabled when both risng and falling are set to NULL.
Definition at line 215 of file ComparatorIn.cpp.
void SampleMode | ( | unsigned char | samp_en ) |
Sampling mode control.
This mode cannot be set when windowing mode is enabled.
- Parameters:
-
input Unsigned char (0 = disable, 1 = enable)
- Returns:
- none
Definition at line 79 of file ComparatorIn.cpp.
unsigned char status | ( | void | ) |
Analog Comparator Output.
Returns the current value of the Analog Comparator output.
The field is reset to 0 and will read as CR1[INV] when the Analog Comparator
module is disabled, that is, when CR1[EN] = 0. Writes to this field are ignored.
- Parameters:
-
none
- Returns:
- comparator status (unsigned char)
Definition at line 158 of file ComparatorIn.cpp.
void SwitchMin | ( | unsigned char | pinM ) |
Minus Input Mux Control.
Determines which input is selected for the plus input of the comparator.
For INx inputs, see CMP, DAC, and ANMUX block diagrams.
0 : IN0 PTC6 CMP0_IN0
1 : IN1 PTC7 CMP0_IN1
2 : IN2 PTC8 CMP0_IN2
3 : IN3 PTC9 CMP0_IN3
4 : IN4 PTE30 CMP0_IN4 12-bit DAC0
5 : IN5 PTE29 CMP0_IN5
6 : IN6 - Bandgap reference (1V)
7 : IN7 - Internal 6-bit DAC0
- Note:
- When an inappropriate operation selects the same input for both muxes, the comparator
automatically shuts down to prevent itself from becoming a noise generator.
- When using the PMC bandgap 1V reference voltage as CMP input, ensure that you enable the bandgap buffer by setting the PMC_REGSC[BGBE] bit.
- Parameters:
-
input Unsigned char - range 0..7
- Returns:
- none
Definition at line 201 of file ComparatorIn.cpp.
void SwitchPlus | ( | unsigned char | pinP ) |
Plus Input Mux Control.
Determines which input is selected for the plus input of the comparator.
For INx inputs, see CMP, DAC, and ANMUX block diagrams.
0 : IN0 PTC6 CMP0_IN0
1 : IN1 PTC7 CMP0_IN1
2 : IN2 PTC8 CMP0_IN2
3 : IN3 PTC9 CMP0_IN3
4 : IN4 PTE30 CMP0_IN4 12-bit DAC0
5 : IN5 PTE29 CMP0_IN5
6 : IN6 - Bandgap reference (1V)
7 : IN7 - Internal 6-bit DAC0
- Note:
- When an inappropriate operation selects the same input for both muxes, the comparator
automatically shuts down to prevent itself from becoming a noise generator.
-
When using the PMC bandgap 1V reference voltage as CMP input, ensure that
enable the bandgap buffer by setting the PMC_REGSC[BGBE] bit.
- Parameters:
-
input Unsigned char - range 0..7
- Returns:
- none
Definition at line 197 of file ComparatorIn.cpp.
void treshold | ( | float | vo_pct ) |
Set the detection threshold level (DAC Output Voltage Select).
Sets The 6-bit or 12-bit DAC output voltage, depending on which DAC is selected on init.
6-bit DACO range is from Vin/64 to Vin.
12-bit DACO range is from Vin/4096 to Vin.
- Parameters:
-
input float - range 0.0 .. 1.0
- Returns:
- none
Definition at line 175 of file ComparatorIn.cpp.
void TrigMode | ( | unsigned char | trig_en ) |
Trigger mode control.
CMP and DAC are configured to CMP Trigger mode when CMP_CR1[TRIGM] is set to 1.
In addition, the CMP should be enabled. If the DAC is to be used as a reference
to the CMP, it should also be enabled.
CMP Trigger mode depends on an external timer resource to periodically enable the
CMP and 6-bit DAC in order to generate a triggered compare. Upon setting TRIGM,
the CMP and DAC are placed in a standby state until an external timer resource
trigger is received.
- Parameters:
-
input Unsigned char (0 = disable, 1 = enable)
- Returns:
- none
Definition at line 97 of file ComparatorIn.cpp.
void WindowMode | ( | unsigned char | win_en ) |
Windowing mode control.
This mode cannot be set when sampling mode is enabled.
- Parameters:
-
input Unsigned char (0 = disable, 1 = enable)
- Returns:
- none
Definition at line 88 of file ComparatorIn.cpp.
Generated on Tue Jul 12 2022 21:37:49 by
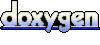