KL25Z Comparator library
Dependents: ComparatorIn_demo TEMT6200_demo 05_comparator_demo 05_comparator_demo ... more
ComparatorIn.h
00001 /************************************************************************************************** 00002 ***** ***** 00003 ***** Name: ComparatorIn.h ***** 00004 ***** Date: 05/06/2013 ***** 00005 ***** Auth: Frank Vannieuwkerke ***** 00006 ***** Func: library for KL25Z Comparator ***** 00007 ***** ***** 00008 **************************************************************************************************/ 00009 00010 #ifndef COMPARATORIN_H 00011 #define COMPARATORIN_H 00012 00013 /* 00014 * Includes 00015 */ 00016 #include "mbed.h" 00017 #include "pinmap.h" 00018 00019 #ifndef TARGET_KL25Z 00020 #error "Target not supported" 00021 #endif 00022 00023 /** ComparatorIn library 00024 * 00025 * INP/INM connection selection : 00026 * PTC6 (IN0) CMP0_IN0 00027 * PTC7 (IN1) CMP0_IN1 00028 * PTC8 (IN2) CMP0_IN2 00029 * PTC9 (IN3) CMP0_IN3 00030 * PTE30 (IN4) CMP0_IN4 (External 12-bit DAC0, auto connect to IN4) 00031 * PTE29 (IN5) CMP0_IN5 00032 * Internal 6-bit DAC reference voltage = VDD (3.3V) 00033 * Hysteresis, filter count and sample period are initialised to these values when ComparatorIn is initialized: 00034 * CMP0->CR0 = 0x00; // Filter and digital hysteresis disabled 00035 * CMP0->CR1 = 0x17; // Continuous mode, high-speed compare, unfiltered output, output pin disabled 00036 * CMP0->FPR = 0x00; // Filter disabled 00037 * CMP0->SCR = 0x06; // Disable all interrupts and clear flags (flags are cleared by this write) 00038 * CMP0->DACCR = 0xC4; // DAC enabled, Vdd is 6bit reference, threshold set to 1/16 of full-scale (0.2V) 00039 */ 00040 00041 typedef enum { 00042 CMP0_IN0 = 0, 00043 CMP0_IN1 = 1, 00044 CMP0_IN2 = 2, 00045 CMP0_IN3 = 3, 00046 CMP0_IN4 = 4, 00047 CMP0_IN5 = 5, 00048 } CMPName; 00049 00050 /** Class to use KL25Z Comparator 00051 */ 00052 class ComparatorIn { 00053 00054 public: 00055 00056 /** Create a ComparatorIn, connected to the specified pins. 00057 * @param pinP = positive ComparatorIn pin to connect to 00058 * @param pinM = negative ComparatorIn pin to connect to\n 00059 * @note Valid values for pinP/pinM:\n 00060 * PTC6, PTC7, PTC8, PTC9, PTE30, PTE29, NC\n 00061 * Special cases:\n 00062 * NC the corresponding input is connected to the internal 6-bit DAC0\n 00063 * PTE30 PTE30 is set as 12-bit DAC0 output and connected to IN4\n 00064 * @return none 00065 */ 00066 ComparatorIn(PinName pinP,PinName pinM); 00067 00068 /** Set the number of consecutive threshold samples. 00069 * Represents the number of consecutive samples that must agree\n 00070 * prior to the comparator ouput filter accepting a new output state.\n 00071 * @param input Unsigned char - range : 1..7 00072 * @return none 00073 */ 00074 void FilterCount(unsigned char fico); 00075 00076 /** Set the hysteresis. 00077 * 0 : 5mV\n 00078 * 1 : 10mV\n 00079 * 2 : 20mV\n 00080 * 3 : 30mV\n 00081 * @param input Unsigned char 00082 * @return none 00083 */ 00084 void hysteresis(unsigned char hyst); 00085 00086 /** Sampling mode control. 00087 * This mode cannot be set when windowing mode is enabled. 00088 * @param input Unsigned char (0 = disable, 1 = enable) 00089 * @return none 00090 */ 00091 void SampleMode(unsigned char samp_en); 00092 00093 /** Windowing mode control. 00094 * This mode cannot be set when sampling mode is enabled. 00095 * @param input Unsigned char (0 = disable, 1 = enable) 00096 * @return none 00097 */ 00098 void WindowMode(unsigned char win_en); 00099 00100 /** Trigger mode control. 00101 * CMP and DAC are configured to CMP Trigger mode when CMP_CR1[TRIGM] is set to 1.\n 00102 * In addition, the CMP should be enabled. If the DAC is to be used as a reference\n 00103 * to the CMP, it should also be enabled.\n 00104 * CMP Trigger mode depends on an external timer resource to periodically enable the\n 00105 * CMP and 6-bit DAC in order to generate a triggered compare. Upon setting TRIGM,\n 00106 * the CMP and DAC are placed in a standby state until an external timer resource\n 00107 * trigger is received.\n 00108 * @param input Unsigned char (0 = disable, 1 = enable) 00109 * @return none 00110 */ 00111 void TrigMode(unsigned char trig_en); 00112 00113 /** Power mode control. 00114 * 0 Low-Speed (LS) Comparison mode selected. In this mode, CMP has\n 00115 * slower output propagation delay and lower current consumption.\n 00116 * 1 High-Speed (HS) Comparison mode selected. In this mode, CMP has\n 00117 * faster output propagation delay and higher current consumption.\n 00118 * @param input Unsigned char - (0 = Low-Speed, 1 = high-Speed) 00119 * @return none 00120 */ 00121 void PowerMode(unsigned char pmode); 00122 00123 /** Invert mode control. 00124 * Allows selection of the polarity of the analog comparator function.\n 00125 * It is also driven to the COUT output, on both the device pin and as SCR[COUT], when OPE=0.\n 00126 * 0 Does not invert the comparator output.\n 00127 * 1 Inverts the comparator output.\n 00128 * @param input Unsigned char - (0 = not inverted, 1 = inverted) 00129 * @return none 00130 */ 00131 void invert(unsigned char inv); 00132 00133 /** Comparator Output Select. 00134 * 0 Set the filtered comparator output (CMPO) to equal COUT.\n 00135 * 1 Set the unfiltered comparator output (CMPO) to equal COUTA.\n 00136 * @param input Unsigned char - (0 : CMPO = COUT, 1 : CMPO = COUTA) 00137 * @return none 00138 */ 00139 void OutputSelect(unsigned char cos); 00140 00141 /** Connect the comparator Output Pin to an external pin. 00142 * Only one pin can be connected at any time.\n 00143 * Each time this function is called, the last active pin will be disabled before the new pin is enabled.\n 00144 * @param input NC disconnect CMPO from the associated CMPO output pin. 00145 * @param input PTC0 connect CMPO to PTC0. 00146 * @param input PTC5 connect CMPO to PTC5. 00147 * @param input PTE0 connect CMPO to PTE0. 00148 */ 00149 void OutputPin(PinName ope); 00150 00151 /** Comparator Module control. 00152 * Used to switch the Analog Comparator module on/off. When the module is not enabled,\n 00153 * it remains in the off state, and consumes no power. When the user selects the same\n 00154 * input from analog mux to the positive and negative port, the comparator is disabled automatically.\n 00155 * 0 Analog Comparator is disabled.\n 00156 * 1 Analog Comparator is enabled.\n 00157 * @param input Unsigned char - (0 = disable, 1 = enable) 00158 * @return none 00159 */ 00160 void enable(unsigned char en); 00161 00162 /** Set the filter sample period. 00163 * Specifies the sampling period, in bus clock cycles, of the comparator\n 00164 * output filter, when CR1[SE]=0. Setting FILT_PER to 0x0 disables the filter.\n 00165 * @param input Unsigned char - range : 0..255 00166 * @return none 00167 */ 00168 void FilterPeriod(unsigned char fipe); 00169 00170 /** DMA Control. 00171 * Used to switch the DMA transfer triggered from the CMP module on/off.\n 00172 * When this field is set, a DMA request is asserted when CFR or CFF is set.\n 00173 * 0 DMA is disabled.\n 00174 * 1 DMA is enabled.\n 00175 * @param input Unsigned char - (0 = disable, 1 = enable) 00176 * @return none 00177 */ 00178 void dma(unsigned char dmaen); 00179 00180 /** Analog Comparator Output. 00181 * Returns the current value of the Analog Comparator output.\n 00182 * The field is reset to 0 and will read as CR1[INV] when the Analog Comparator\n 00183 * module is disabled, that is, when CR1[EN] = 0. Writes to this field are ignored.\n 00184 * @param none 00185 * @return comparator status (unsigned char) 00186 */ 00187 unsigned char status(void); 00188 00189 /** DAC Control. 00190 * Used to switch the internal DAC on/off. When the DAC is disabled, it is powered down to conserve power.\n 00191 * 0 DAC is disabled.\n 00192 * 1 DAC is enabled.\n 00193 * @param input Unsigned char - 0 or 1 00194 * @return none 00195 */ 00196 void dac(unsigned char den); 00197 00198 /** Supply Voltage Reference Source Select. 00199 * 0 - V is selected as resistor ladder network supply reference Vin1 = VREFH\n 00200 * 1 - V is selected as resistor ladder network supply reference Vin2 = VDD\n 00201 * (Use this option for the best ADC operation).\n 00202 * @param input Unsigned char - 0 or 1 00203 * @return none 00204 */ 00205 void RefSource(unsigned char res); 00206 00207 /** Set the detection threshold level (DAC Output Voltage Select). 00208 * Sets The 6-bit or 12-bit DAC output voltage, depending on which DAC is selected on init.\n 00209 * 6-bit DACO range is from Vin/64 to Vin.\n 00210 * 12-bit DACO range is from Vin/4096 to Vin.\n 00211 * @param input float - range 0.0 .. 1.0 00212 * @return none 00213 */ 00214 void treshold(float vo_pct); 00215 00216 /** Pass Through Mode Control. 00217 * Set the MUX pass through mode. Pass through mode is always available, but for\n 00218 * some devices, this feature must be always disabled due to the lack of package pins.\n 00219 * 0 Pass Through Mode is disabled.\n 00220 * 1 Pass Through Mode is enabled.\n 00221 * @param input Unsigned char - (0 = disable, 1 = enable) 00222 * @return none 00223 */ 00224 void PassThrough(unsigned char ptm); 00225 00226 /** Plus Input Mux Control. 00227 * Determines which input is selected for the plus input of the comparator.\n 00228 * For INx inputs, see CMP, DAC, and ANMUX block diagrams.\n 00229 * 0 : IN0 PTC6 CMP0_IN0\n 00230 * 1 : IN1 PTC7 CMP0_IN1\n 00231 * 2 : IN2 PTC8 CMP0_IN2\n 00232 * 3 : IN3 PTC9 CMP0_IN3\n 00233 * 4 : IN4 PTE30 CMP0_IN4 12-bit DAC0\n 00234 * 5 : IN5 PTE29 CMP0_IN5\n 00235 * 6 : IN6 - Bandgap reference (1V)\n 00236 * 7 : IN7 - Internal 6-bit DAC0\n 00237 * @note When an inappropriate operation selects the same input for both muxes, the comparator\n 00238 * automatically shuts down to prevent itself from becoming a noise generator.\n 00239 * @note When using the PMC bandgap 1V reference voltage as CMP input, ensure that\n 00240 * @you enable the bandgap buffer by setting the PMC_REGSC[BGBE] bit.\n 00241 * @param input Unsigned char - range 0..7 00242 * @return none 00243 */ 00244 void SwitchPlus(unsigned char pinP); 00245 00246 /** Minus Input Mux Control. 00247 * Determines which input is selected for the plus input of the comparator.\n 00248 * For INx inputs, see CMP, DAC, and ANMUX block diagrams.\n 00249 * 0 : IN0 PTC6 CMP0_IN0\n 00250 * 1 : IN1 PTC7 CMP0_IN1\n 00251 * 2 : IN2 PTC8 CMP0_IN2\n 00252 * 3 : IN3 PTC9 CMP0_IN3\n 00253 * 4 : IN4 PTE30 CMP0_IN4 12-bit DAC0\n 00254 * 5 : IN5 PTE29 CMP0_IN5\n 00255 * 6 : IN6 - Bandgap reference (1V)\n 00256 * 7 : IN7 - Internal 6-bit DAC0\n 00257 * @note When an inappropriate operation selects the same input for both muxes, the comparator\n 00258 * automatically shuts down to prevent itself from becoming a noise generator.\n 00259 * @note When using the PMC bandgap 1V reference voltage as CMP input, ensure that 00260 * you enable the bandgap buffer by setting the PMC_REGSC[BGBE] bit. 00261 * @param input Unsigned char - range 0..7 00262 * @return none 00263 */ 00264 void SwitchMin(unsigned char pinM); 00265 00266 /** Comparator rising interrupt callback. 00267 * @param pointer to the user function to execute after IRQ assertion 00268 * @param NULL to disable the interrupt 00269 * @return none 00270 * @note The interrupt is automatically enabled when a valid pointer is used.\n 00271 * The interrupt is automatically disabled when both risng and falling are set to NULL. 00272 */ 00273 void rising(void(*fptr)(void)); 00274 00275 /** Comparator falling interrupt callback 00276 * @param pointer to the user function to execute after IRQ assertion 00277 * @param NULL to disable the interrupt 00278 * @return none 00279 * @note The interrupt is automatically enabled when a valid pointer is used.\n 00280 * The interrupt is automatically disabled when both risng and falling are set to NULL. 00281 */ 00282 void falling(void(*fptr)(void)); 00283 00284 private: 00285 static const PinMap PinMap_CMP[7]; 00286 static void _cmpISR(void); 00287 void hscmp_clear(void); 00288 PinName op_status(void); 00289 void op_enable(PinName pen, PinName pstat); 00290 void op_disable(PinName pdi); 00291 void dac6_write(unsigned int value); 00292 char CMPnumberP, CMPnumberM; 00293 }; 00294 00295 #endif 00296 00297 00298
Generated on Tue Jul 12 2022 21:37:49 by
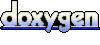