mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
ssl.h
00001 /** 00002 * \file ssl.h 00003 * 00004 * \brief SSL/TLS functions. 00005 * 00006 * Copyright (C) 2006-2014, ARM Limited, All Rights Reserved 00007 * 00008 * This file is part of mbed TLS (https://tls.mbed.org) 00009 * 00010 * This program is free software; you can redistribute it and/or modify 00011 * it under the terms of the GNU General Public License as published by 00012 * the Free Software Foundation; either version 2 of the License, or 00013 * (at your option) any later version. 00014 * 00015 * This program is distributed in the hope that it will be useful, 00016 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00017 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00018 * GNU General Public License for more details. 00019 * 00020 * You should have received a copy of the GNU General Public License along 00021 * with this program; if not, write to the Free Software Foundation, Inc., 00022 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00023 */ 00024 #ifndef POLARSSL_SSL_H 00025 #define POLARSSL_SSL_H 00026 00027 #if !defined(POLARSSL_CONFIG_FILE) 00028 #include "config.h" 00029 #else 00030 #include POLARSSL_CONFIG_FILE 00031 #endif 00032 00033 /* Temporary compatibility trick for the current stable branch */ 00034 #if !defined(POLARSSL_SSL_DISABLE_RENEGOTIATION) 00035 #define POLARSSL_SSL_RENEGOTIATION 00036 #endif 00037 00038 #include "net.h" 00039 #include "bignum.h" 00040 #include "ecp.h" 00041 00042 #include "ssl_ciphersuites.h" 00043 00044 #if defined(POLARSSL_MD5_C) 00045 #include "md5.h" 00046 #endif 00047 00048 #if defined(POLARSSL_SHA1_C) 00049 #include "sha1.h" 00050 #endif 00051 00052 #if defined(POLARSSL_SHA256_C) 00053 #include "sha256.h" 00054 #endif 00055 00056 #if defined(POLARSSL_SHA512_C) 00057 #include "sha512.h" 00058 #endif 00059 00060 // for session tickets 00061 #if defined(POLARSSL_AES_C) 00062 #include "aes.h" 00063 #endif 00064 00065 #if defined(POLARSSL_X509_CRT_PARSE_C) 00066 #include "x509_crt.h" 00067 #include "x509_crl.h" 00068 #endif 00069 00070 #if defined(POLARSSL_DHM_C) 00071 #include "dhm.h" 00072 #endif 00073 00074 #if defined(POLARSSL_ECDH_C) 00075 #include "ecdh.h" 00076 #endif 00077 00078 #if defined(POLARSSL_ZLIB_SUPPORT) 00079 #include "zlib.h" 00080 #endif 00081 00082 #if defined(POLARSSL_HAVE_TIME) 00083 #include <time.h> 00084 #endif 00085 00086 /* For convenience below and in programs */ 00087 #if defined(POLARSSL_KEY_EXCHANGE_PSK_ENABLED) || \ 00088 defined(POLARSSL_KEY_EXCHANGE_RSA_PSK_ENABLED) || \ 00089 defined(POLARSSL_KEY_EXCHANGE_DHE_PSK_ENABLED) || \ 00090 defined(POLARSSL_KEY_EXCHANGE_ECDHE_PSK_ENABLED) 00091 #define POLARSSL_KEY_EXCHANGE__SOME__PSK_ENABLED 00092 #endif 00093 00094 #if defined(POLARSSL_KEY_EXCHANGE_ECDHE_RSA_ENABLED) || \ 00095 defined(POLARSSL_KEY_EXCHANGE_ECDHE_ECDSA_ENABLED) || \ 00096 defined(POLARSSL_KEY_EXCHANGE_ECDHE_PSK_ENABLED) 00097 #define POLARSSL_KEY_EXCHANGE__SOME__ECDHE_ENABLED 00098 #endif 00099 00100 #if defined(_MSC_VER) && !defined(inline) 00101 #define inline _inline 00102 #else 00103 #if defined(__ARMCC_VERSION) && !defined(inline) 00104 #define inline __inline 00105 #endif /* __ARMCC_VERSION */ 00106 #endif /*_MSC_VER */ 00107 00108 /* 00109 * SSL Error codes 00110 */ 00111 #define POLARSSL_ERR_SSL_FEATURE_UNAVAILABLE -0x7080 /**< The requested feature is not available. */ 00112 #define POLARSSL_ERR_SSL_BAD_INPUT_DATA -0x7100 /**< Bad input parameters to function. */ 00113 #define POLARSSL_ERR_SSL_INVALID_MAC -0x7180 /**< Verification of the message MAC failed. */ 00114 #define POLARSSL_ERR_SSL_INVALID_RECORD -0x7200 /**< An invalid SSL record was received. */ 00115 #define POLARSSL_ERR_SSL_CONN_EOF -0x7280 /**< The connection indicated an EOF. */ 00116 #define POLARSSL_ERR_SSL_UNKNOWN_CIPHER -0x7300 /**< An unknown cipher was received. */ 00117 #define POLARSSL_ERR_SSL_NO_CIPHER_CHOSEN -0x7380 /**< The server has no ciphersuites in common with the client. */ 00118 #define POLARSSL_ERR_SSL_NO_RNG -0x7400 /**< No RNG was provided to the SSL module. */ 00119 #define POLARSSL_ERR_SSL_NO_CLIENT_CERTIFICATE -0x7480 /**< No client certification received from the client, but required by the authentication mode. */ 00120 #define POLARSSL_ERR_SSL_CERTIFICATE_TOO_LARGE -0x7500 /**< Our own certificate(s) is/are too large to send in an SSL message. */ 00121 #define POLARSSL_ERR_SSL_CERTIFICATE_REQUIRED -0x7580 /**< The own certificate is not set, but needed by the server. */ 00122 #define POLARSSL_ERR_SSL_PRIVATE_KEY_REQUIRED -0x7600 /**< The own private key or pre-shared key is not set, but needed. */ 00123 #define POLARSSL_ERR_SSL_CA_CHAIN_REQUIRED -0x7680 /**< No CA Chain is set, but required to operate. */ 00124 #define POLARSSL_ERR_SSL_UNEXPECTED_MESSAGE -0x7700 /**< An unexpected message was received from our peer. */ 00125 #define POLARSSL_ERR_SSL_FATAL_ALERT_MESSAGE -0x7780 /**< A fatal alert message was received from our peer. */ 00126 #define POLARSSL_ERR_SSL_PEER_VERIFY_FAILED -0x7800 /**< Verification of our peer failed. */ 00127 #define POLARSSL_ERR_SSL_PEER_CLOSE_NOTIFY -0x7880 /**< The peer notified us that the connection is going to be closed. */ 00128 #define POLARSSL_ERR_SSL_BAD_HS_CLIENT_HELLO -0x7900 /**< Processing of the ClientHello handshake message failed. */ 00129 #define POLARSSL_ERR_SSL_BAD_HS_SERVER_HELLO -0x7980 /**< Processing of the ServerHello handshake message failed. */ 00130 #define POLARSSL_ERR_SSL_BAD_HS_CERTIFICATE -0x7A00 /**< Processing of the Certificate handshake message failed. */ 00131 #define POLARSSL_ERR_SSL_BAD_HS_CERTIFICATE_REQUEST -0x7A80 /**< Processing of the CertificateRequest handshake message failed. */ 00132 #define POLARSSL_ERR_SSL_BAD_HS_SERVER_KEY_EXCHANGE -0x7B00 /**< Processing of the ServerKeyExchange handshake message failed. */ 00133 #define POLARSSL_ERR_SSL_BAD_HS_SERVER_HELLO_DONE -0x7B80 /**< Processing of the ServerHelloDone handshake message failed. */ 00134 #define POLARSSL_ERR_SSL_BAD_HS_CLIENT_KEY_EXCHANGE -0x7C00 /**< Processing of the ClientKeyExchange handshake message failed. */ 00135 #define POLARSSL_ERR_SSL_BAD_HS_CLIENT_KEY_EXCHANGE_RP -0x7C80 /**< Processing of the ClientKeyExchange handshake message failed in DHM / ECDH Read Public. */ 00136 #define POLARSSL_ERR_SSL_BAD_HS_CLIENT_KEY_EXCHANGE_CS -0x7D00 /**< Processing of the ClientKeyExchange handshake message failed in DHM / ECDH Calculate Secret. */ 00137 #define POLARSSL_ERR_SSL_BAD_HS_CERTIFICATE_VERIFY -0x7D80 /**< Processing of the CertificateVerify handshake message failed. */ 00138 #define POLARSSL_ERR_SSL_BAD_HS_CHANGE_CIPHER_SPEC -0x7E00 /**< Processing of the ChangeCipherSpec handshake message failed. */ 00139 #define POLARSSL_ERR_SSL_BAD_HS_FINISHED -0x7E80 /**< Processing of the Finished handshake message failed. */ 00140 #define POLARSSL_ERR_SSL_MALLOC_FAILED -0x7F00 /**< Memory allocation failed */ 00141 #define POLARSSL_ERR_SSL_HW_ACCEL_FAILED -0x7F80 /**< Hardware acceleration function returned with error */ 00142 #define POLARSSL_ERR_SSL_HW_ACCEL_FALLTHROUGH -0x6F80 /**< Hardware acceleration function skipped / left alone data */ 00143 #define POLARSSL_ERR_SSL_COMPRESSION_FAILED -0x6F00 /**< Processing of the compression / decompression failed */ 00144 #define POLARSSL_ERR_SSL_BAD_HS_PROTOCOL_VERSION -0x6E80 /**< Handshake protocol not within min/max boundaries */ 00145 #define POLARSSL_ERR_SSL_BAD_HS_NEW_SESSION_TICKET -0x6E00 /**< Processing of the NewSessionTicket handshake message failed. */ 00146 #define POLARSSL_ERR_SSL_SESSION_TICKET_EXPIRED -0x6D80 /**< Session ticket has expired. */ 00147 #define POLARSSL_ERR_SSL_PK_TYPE_MISMATCH -0x6D00 /**< Public key type mismatch (eg, asked for RSA key exchange and presented EC key) */ 00148 #define POLARSSL_ERR_SSL_UNKNOWN_IDENTITY -0x6C80 /**< Unknown identity received (eg, PSK identity) */ 00149 #define POLARSSL_ERR_SSL_INTERNAL_ERROR -0x6C00 /**< Internal error (eg, unexpected failure in lower-level module) */ 00150 #define POLARSSL_ERR_SSL_COUNTER_WRAPPING -0x6B80 /**< A counter would wrap (eg, too many messages exchanged). */ 00151 #define POLARSSL_ERR_SSL_WAITING_SERVER_HELLO_RENEGO -0x6B00 /**< Unexpected message at ServerHello in renegotiation. */ 00152 #define POLARSSL_ERR_SSL_NO_USABLE_CIPHERSUITE -0x6A80 /**< None of the common ciphersuites is usable (eg, no suitable certificate, see debug messages). */ 00153 00154 /* 00155 * Various constants 00156 */ 00157 #define SSL_MAJOR_VERSION_3 3 00158 #define SSL_MINOR_VERSION_0 0 /*!< SSL v3.0 */ 00159 #define SSL_MINOR_VERSION_1 1 /*!< TLS v1.0 */ 00160 #define SSL_MINOR_VERSION_2 2 /*!< TLS v1.1 */ 00161 #define SSL_MINOR_VERSION_3 3 /*!< TLS v1.2 */ 00162 00163 /* Determine minimum supported version */ 00164 #define SSL_MIN_MAJOR_VERSION SSL_MAJOR_VERSION_3 00165 00166 #if defined(POLARSSL_SSL_PROTO_SSL3) 00167 #define SSL_MIN_MINOR_VERSION SSL_MINOR_VERSION_0 00168 #else 00169 #if defined(POLARSSL_SSL_PROTO_TLS1) 00170 #define SSL_MIN_MINOR_VERSION SSL_MINOR_VERSION_1 00171 #else 00172 #if defined(POLARSSL_SSL_PROTO_TLS1_1) 00173 #define SSL_MIN_MINOR_VERSION SSL_MINOR_VERSION_2 00174 #else 00175 #if defined(POLARSSL_SSL_PROTO_TLS1_2) 00176 #define SSL_MIN_MINOR_VERSION SSL_MINOR_VERSION_3 00177 #endif /* POLARSSL_SSL_PROTO_TLS1_2 */ 00178 #endif /* POLARSSL_SSL_PROTO_TLS1_1 */ 00179 #endif /* POLARSSL_SSL_PROTO_TLS1 */ 00180 #endif /* POLARSSL_SSL_PROTO_SSL3 */ 00181 00182 /* Determine maximum supported version */ 00183 #define SSL_MAX_MAJOR_VERSION SSL_MAJOR_VERSION_3 00184 00185 #if defined(POLARSSL_SSL_PROTO_TLS1_2) 00186 #define SSL_MAX_MINOR_VERSION SSL_MINOR_VERSION_3 00187 #else 00188 #if defined(POLARSSL_SSL_PROTO_TLS1_1) 00189 #define SSL_MAX_MINOR_VERSION SSL_MINOR_VERSION_2 00190 #else 00191 #if defined(POLARSSL_SSL_PROTO_TLS1) 00192 #define SSL_MAX_MINOR_VERSION SSL_MINOR_VERSION_1 00193 #else 00194 #if defined(POLARSSL_SSL_PROTO_SSL3) 00195 #define SSL_MAX_MINOR_VERSION SSL_MINOR_VERSION_0 00196 #endif /* POLARSSL_SSL_PROTO_SSL3 */ 00197 #endif /* POLARSSL_SSL_PROTO_TLS1 */ 00198 #endif /* POLARSSL_SSL_PROTO_TLS1_1 */ 00199 #endif /* POLARSSL_SSL_PROTO_TLS1_2 */ 00200 00201 /* RFC 6066 section 4, see also mfl_code_to_length in ssl_tls.c 00202 * NONE must be zero so that memset()ing structure to zero works */ 00203 #define SSL_MAX_FRAG_LEN_NONE 0 /*!< don't use this extension */ 00204 #define SSL_MAX_FRAG_LEN_512 1 /*!< MaxFragmentLength 2^9 */ 00205 #define SSL_MAX_FRAG_LEN_1024 2 /*!< MaxFragmentLength 2^10 */ 00206 #define SSL_MAX_FRAG_LEN_2048 3 /*!< MaxFragmentLength 2^11 */ 00207 #define SSL_MAX_FRAG_LEN_4096 4 /*!< MaxFragmentLength 2^12 */ 00208 #define SSL_MAX_FRAG_LEN_INVALID 5 /*!< first invalid value */ 00209 00210 #define SSL_IS_CLIENT 0 00211 #define SSL_IS_SERVER 1 00212 00213 #define SSL_IS_NOT_FALLBACK 0 00214 #define SSL_IS_FALLBACK 1 00215 00216 #define SSL_EXTENDED_MS_DISABLED 0 00217 #define SSL_EXTENDED_MS_ENABLED 1 00218 00219 #define SSL_ETM_DISABLED 0 00220 #define SSL_ETM_ENABLED 1 00221 00222 #define SSL_COMPRESS_NULL 0 00223 #define SSL_COMPRESS_DEFLATE 1 00224 00225 #define SSL_VERIFY_NONE 0 00226 #define SSL_VERIFY_OPTIONAL 1 00227 #define SSL_VERIFY_REQUIRED 2 00228 00229 #define SSL_INITIAL_HANDSHAKE 0 00230 #define SSL_RENEGOTIATION 1 /* In progress */ 00231 #define SSL_RENEGOTIATION_DONE 2 /* Done */ 00232 #define SSL_RENEGOTIATION_PENDING 3 /* Requested (server only) */ 00233 00234 #define SSL_LEGACY_RENEGOTIATION 0 00235 #define SSL_SECURE_RENEGOTIATION 1 00236 00237 #define SSL_RENEGOTIATION_DISABLED 0 00238 #define SSL_RENEGOTIATION_ENABLED 1 00239 00240 #define SSL_RENEGOTIATION_NOT_ENFORCED -1 00241 #define SSL_RENEGO_MAX_RECORDS_DEFAULT 16 00242 00243 #define SSL_LEGACY_NO_RENEGOTIATION 0 00244 #define SSL_LEGACY_ALLOW_RENEGOTIATION 1 00245 #define SSL_LEGACY_BREAK_HANDSHAKE 2 00246 00247 #define SSL_TRUNC_HMAC_DISABLED 0 00248 #define SSL_TRUNC_HMAC_ENABLED 1 00249 #define SSL_TRUNCATED_HMAC_LEN 10 /* 80 bits, rfc 6066 section 7 */ 00250 00251 #define SSL_SESSION_TICKETS_DISABLED 0 00252 #define SSL_SESSION_TICKETS_ENABLED 1 00253 00254 #define SSL_CBC_RECORD_SPLITTING_DISABLED -1 00255 #define SSL_CBC_RECORD_SPLITTING_ENABLED 0 00256 00257 #define SSL_ARC4_ENABLED 0 00258 #define SSL_ARC4_DISABLED 1 00259 00260 /** 00261 * \name SECTION: Module settings 00262 * 00263 * The configuration options you can set for this module are in this section. 00264 * Either change them in config.h or define them on the compiler command line. 00265 * \{ 00266 */ 00267 00268 #if !defined(SSL_DEFAULT_TICKET_LIFETIME) 00269 #define SSL_DEFAULT_TICKET_LIFETIME 86400 /**< Lifetime of session tickets (if enabled) */ 00270 #endif 00271 00272 /* 00273 * Size of the input / output buffer. 00274 * Note: the RFC defines the default size of SSL / TLS messages. If you 00275 * change the value here, other clients / servers may not be able to 00276 * communicate with you anymore. Only change this value if you control 00277 * both sides of the connection and have it reduced at both sides, or 00278 * if you're using the Max Fragment Length extension and you know all your 00279 * peers are using it too! 00280 */ 00281 #if !defined(SSL_MAX_CONTENT_LEN) 00282 #define SSL_MAX_CONTENT_LEN 16384 /**< Size of the input / output buffer */ 00283 #endif 00284 00285 /* \} name SECTION: Module settings */ 00286 00287 /* 00288 * Allow extra bytes for record, authentication and encryption overhead: 00289 * counter (8) + header (5) + IV(16) + MAC (16-48) + padding (0-256) 00290 * and allow for a maximum of 1024 of compression expansion if 00291 * enabled. 00292 */ 00293 #if defined(POLARSSL_ZLIB_SUPPORT) 00294 #define SSL_COMPRESSION_ADD 1024 00295 #else 00296 #define SSL_COMPRESSION_ADD 0 00297 #endif 00298 00299 #if defined(POLARSSL_RC4_C) || defined(POLARSSL_CIPHER_MODE_CBC) 00300 /* Ciphersuites using HMAC */ 00301 #if defined(POLARSSL_SHA512_C) 00302 #define SSL_MAC_ADD 48 /* SHA-384 used for HMAC */ 00303 #elif defined(POLARSSL_SHA256_C) 00304 #define SSL_MAC_ADD 32 /* SHA-256 used for HMAC */ 00305 #else 00306 #define SSL_MAC_ADD 20 /* SHA-1 used for HMAC */ 00307 #endif 00308 #else 00309 /* AEAD ciphersuites: GCM and CCM use a 128 bits tag */ 00310 #define SSL_MAC_ADD 16 00311 #endif 00312 00313 #if defined(POLARSSL_CIPHER_MODE_CBC) 00314 #define SSL_PADDING_ADD 256 00315 #else 00316 #define SSL_PADDING_ADD 0 00317 #endif 00318 00319 #define SSL_BUFFER_LEN ( SSL_MAX_CONTENT_LEN \ 00320 + SSL_COMPRESSION_ADD \ 00321 + 29 /* counter + header + IV */ \ 00322 + SSL_MAC_ADD \ 00323 + SSL_PADDING_ADD \ 00324 ) 00325 00326 /* 00327 * Length of the verify data for secure renegotiation 00328 */ 00329 #if defined(POLARSSL_SSL_PROTO_SSL3) 00330 #define SSL_VERIFY_DATA_MAX_LEN 36 00331 #else 00332 #define SSL_VERIFY_DATA_MAX_LEN 12 00333 #endif 00334 00335 /* 00336 * Signaling ciphersuite values (SCSV) 00337 */ 00338 #define SSL_EMPTY_RENEGOTIATION_INFO 0xFF /**< renegotiation info ext */ 00339 #define SSL_FALLBACK_SCSV 0x5600 /**< draft-ietf-tls-downgrade-scsv-00 */ 00340 00341 /* 00342 * Supported Signature and Hash algorithms (For TLS 1.2) 00343 * RFC 5246 section 7.4.1.4.1 00344 */ 00345 #define SSL_HASH_NONE 0 00346 #define SSL_HASH_MD5 1 00347 #define SSL_HASH_SHA1 2 00348 #define SSL_HASH_SHA224 3 00349 #define SSL_HASH_SHA256 4 00350 #define SSL_HASH_SHA384 5 00351 #define SSL_HASH_SHA512 6 00352 00353 #define SSL_SIG_ANON 0 00354 #define SSL_SIG_RSA 1 00355 #define SSL_SIG_ECDSA 3 00356 00357 /* 00358 * Client Certificate Types 00359 * RFC 5246 section 7.4.4 plus RFC 4492 section 5.5 00360 */ 00361 #define SSL_CERT_TYPE_RSA_SIGN 1 00362 #define SSL_CERT_TYPE_ECDSA_SIGN 64 00363 00364 /* 00365 * Message, alert and handshake types 00366 */ 00367 #define SSL_MSG_CHANGE_CIPHER_SPEC 20 00368 #define SSL_MSG_ALERT 21 00369 #define SSL_MSG_HANDSHAKE 22 00370 #define SSL_MSG_APPLICATION_DATA 23 00371 00372 #define SSL_ALERT_LEVEL_WARNING 1 00373 #define SSL_ALERT_LEVEL_FATAL 2 00374 00375 #define SSL_ALERT_MSG_CLOSE_NOTIFY 0 /* 0x00 */ 00376 #define SSL_ALERT_MSG_UNEXPECTED_MESSAGE 10 /* 0x0A */ 00377 #define SSL_ALERT_MSG_BAD_RECORD_MAC 20 /* 0x14 */ 00378 #define SSL_ALERT_MSG_DECRYPTION_FAILED 21 /* 0x15 */ 00379 #define SSL_ALERT_MSG_RECORD_OVERFLOW 22 /* 0x16 */ 00380 #define SSL_ALERT_MSG_DECOMPRESSION_FAILURE 30 /* 0x1E */ 00381 #define SSL_ALERT_MSG_HANDSHAKE_FAILURE 40 /* 0x28 */ 00382 #define SSL_ALERT_MSG_NO_CERT 41 /* 0x29 */ 00383 #define SSL_ALERT_MSG_BAD_CERT 42 /* 0x2A */ 00384 #define SSL_ALERT_MSG_UNSUPPORTED_CERT 43 /* 0x2B */ 00385 #define SSL_ALERT_MSG_CERT_REVOKED 44 /* 0x2C */ 00386 #define SSL_ALERT_MSG_CERT_EXPIRED 45 /* 0x2D */ 00387 #define SSL_ALERT_MSG_CERT_UNKNOWN 46 /* 0x2E */ 00388 #define SSL_ALERT_MSG_ILLEGAL_PARAMETER 47 /* 0x2F */ 00389 #define SSL_ALERT_MSG_UNKNOWN_CA 48 /* 0x30 */ 00390 #define SSL_ALERT_MSG_ACCESS_DENIED 49 /* 0x31 */ 00391 #define SSL_ALERT_MSG_DECODE_ERROR 50 /* 0x32 */ 00392 #define SSL_ALERT_MSG_DECRYPT_ERROR 51 /* 0x33 */ 00393 #define SSL_ALERT_MSG_EXPORT_RESTRICTION 60 /* 0x3C */ 00394 #define SSL_ALERT_MSG_PROTOCOL_VERSION 70 /* 0x46 */ 00395 #define SSL_ALERT_MSG_INSUFFICIENT_SECURITY 71 /* 0x47 */ 00396 #define SSL_ALERT_MSG_INTERNAL_ERROR 80 /* 0x50 */ 00397 #define SSL_ALERT_MSG_INAPROPRIATE_FALLBACK 86 /* 0x56 */ 00398 #define SSL_ALERT_MSG_USER_CANCELED 90 /* 0x5A */ 00399 #define SSL_ALERT_MSG_NO_RENEGOTIATION 100 /* 0x64 */ 00400 #define SSL_ALERT_MSG_UNSUPPORTED_EXT 110 /* 0x6E */ 00401 #define SSL_ALERT_MSG_UNRECOGNIZED_NAME 112 /* 0x70 */ 00402 #define SSL_ALERT_MSG_UNKNOWN_PSK_IDENTITY 115 /* 0x73 */ 00403 #define SSL_ALERT_MSG_NO_APPLICATION_PROTOCOL 120 /* 0x78 */ 00404 00405 #define SSL_HS_HELLO_REQUEST 0 00406 #define SSL_HS_CLIENT_HELLO 1 00407 #define SSL_HS_SERVER_HELLO 2 00408 #define SSL_HS_NEW_SESSION_TICKET 4 00409 #define SSL_HS_CERTIFICATE 11 00410 #define SSL_HS_SERVER_KEY_EXCHANGE 12 00411 #define SSL_HS_CERTIFICATE_REQUEST 13 00412 #define SSL_HS_SERVER_HELLO_DONE 14 00413 #define SSL_HS_CERTIFICATE_VERIFY 15 00414 #define SSL_HS_CLIENT_KEY_EXCHANGE 16 00415 #define SSL_HS_FINISHED 20 00416 00417 /* 00418 * TLS extensions 00419 */ 00420 #define TLS_EXT_SERVERNAME 0 00421 #define TLS_EXT_SERVERNAME_HOSTNAME 0 00422 00423 #define TLS_EXT_MAX_FRAGMENT_LENGTH 1 00424 00425 #define TLS_EXT_TRUNCATED_HMAC 4 00426 00427 #define TLS_EXT_SUPPORTED_ELLIPTIC_CURVES 10 00428 #define TLS_EXT_SUPPORTED_POINT_FORMATS 11 00429 00430 #define TLS_EXT_SIG_ALG 13 00431 00432 #define TLS_EXT_ALPN 16 00433 00434 #define TLS_EXT_ENCRYPT_THEN_MAC 22 /* 0x16 */ 00435 #define TLS_EXT_EXTENDED_MASTER_SECRET 0x0017 /* 23 */ 00436 00437 #define TLS_EXT_SESSION_TICKET 35 00438 00439 #define TLS_EXT_RENEGOTIATION_INFO 0xFF01 00440 00441 /* 00442 * TLS extension flags (for extensions with outgoing ServerHello content 00443 * that need it (e.g. for RENEGOTIATION_INFO the server already knows because 00444 * of state of the renegotiation flag, so no indicator is required) 00445 */ 00446 #define TLS_EXT_SUPPORTED_POINT_FORMATS_PRESENT (1 << 0) 00447 00448 /* 00449 * Size defines 00450 */ 00451 #if !defined(POLARSSL_PSK_MAX_LEN) 00452 #define POLARSSL_PSK_MAX_LEN 32 /* 256 bits */ 00453 #endif 00454 00455 /* Dummy type used only for its size */ 00456 union _ssl_premaster_secret 00457 { 00458 #if defined(POLARSSL_KEY_EXCHANGE_RSA_ENABLED) 00459 unsigned char _pms_rsa[48]; /* RFC 5246 8.1.1 */ 00460 #endif 00461 #if defined(POLARSSL_KEY_EXCHANGE_DHE_RSA_ENABLED) 00462 unsigned char _pms_dhm[POLARSSL_MPI_MAX_SIZE]; /* RFC 5246 8.1.2 */ 00463 #endif 00464 #if defined(POLARSSL_KEY_EXCHANGE_ECDHE_RSA_ENABLED) || \ 00465 defined(POLARSSL_KEY_EXCHANGE_ECDHE_ECDSA_ENABLED) || \ 00466 defined(POLARSSL_KEY_EXCHANGE_ECDH_RSA_ENABLED) || \ 00467 defined(POLARSSL_KEY_EXCHANGE_ECDH_ECDSA_ENABLED) 00468 unsigned char _pms_ecdh[POLARSSL_ECP_MAX_BYTES]; /* RFC 4492 5.10 */ 00469 #endif 00470 #if defined(POLARSSL_KEY_EXCHANGE_PSK_ENABLED) 00471 unsigned char _pms_psk[4 + 2 * POLARSSL_PSK_MAX_LEN]; /* RFC 4279 2 */ 00472 #endif 00473 #if defined(POLARSSL_KEY_EXCHANGE_DHE_PSK_ENABLED) 00474 unsigned char _pms_dhe_psk[4 + POLARSSL_MPI_MAX_SIZE 00475 + POLARSSL_PSK_MAX_LEN]; /* RFC 4279 3 */ 00476 #endif 00477 #if defined(POLARSSL_KEY_EXCHANGE_RSA_PSK_ENABLED) 00478 unsigned char _pms_rsa_psk[52 + POLARSSL_PSK_MAX_LEN]; /* RFC 4279 4 */ 00479 #endif 00480 #if defined(POLARSSL_KEY_EXCHANGE_ECDHE_PSK_ENABLED) 00481 unsigned char _pms_ecdhe_psk[4 + POLARSSL_ECP_MAX_BYTES 00482 + POLARSSL_PSK_MAX_LEN]; /* RFC 5489 2 */ 00483 #endif 00484 }; 00485 00486 #define POLARSSL_PREMASTER_SIZE sizeof( union _ssl_premaster_secret ) 00487 00488 #ifdef __cplusplus 00489 extern "C" { 00490 #endif 00491 00492 /* 00493 * Generic function pointers for allowing external RSA private key 00494 * implementations. 00495 */ 00496 typedef int (*rsa_decrypt_func)( void *ctx, int mode, size_t *olen, 00497 const unsigned char *input, unsigned char *output, 00498 size_t output_max_len ); 00499 typedef int (*rsa_sign_func)( void *ctx, 00500 int (*f_rng)(void *, unsigned char *, size_t), void *p_rng, 00501 int mode, md_type_t md_alg, unsigned int hashlen, 00502 const unsigned char *hash, unsigned char *sig ); 00503 typedef size_t (*rsa_key_len_func)( void *ctx ); 00504 00505 /* 00506 * SSL state machine 00507 */ 00508 typedef enum 00509 { 00510 SSL_HELLO_REQUEST, 00511 SSL_CLIENT_HELLO, 00512 SSL_SERVER_HELLO, 00513 SSL_SERVER_CERTIFICATE, 00514 SSL_SERVER_KEY_EXCHANGE, 00515 SSL_CERTIFICATE_REQUEST, 00516 SSL_SERVER_HELLO_DONE, 00517 SSL_CLIENT_CERTIFICATE, 00518 SSL_CLIENT_KEY_EXCHANGE, 00519 SSL_CERTIFICATE_VERIFY, 00520 SSL_CLIENT_CHANGE_CIPHER_SPEC, 00521 SSL_CLIENT_FINISHED, 00522 SSL_SERVER_CHANGE_CIPHER_SPEC, 00523 SSL_SERVER_FINISHED, 00524 SSL_FLUSH_BUFFERS, 00525 SSL_HANDSHAKE_WRAPUP, 00526 SSL_HANDSHAKE_OVER, 00527 SSL_SERVER_NEW_SESSION_TICKET, 00528 } 00529 ssl_states; 00530 00531 typedef struct _ssl_session ssl_session; 00532 typedef struct _ssl_context ssl_context; 00533 typedef struct _ssl_transform ssl_transform; 00534 typedef struct _ssl_handshake_params ssl_handshake_params; 00535 #if defined(POLARSSL_SSL_SESSION_TICKETS) 00536 typedef struct _ssl_ticket_keys ssl_ticket_keys; 00537 #endif 00538 #if defined(POLARSSL_X509_CRT_PARSE_C) 00539 typedef struct _ssl_key_cert ssl_key_cert; 00540 #endif 00541 00542 /* 00543 * This structure is used for storing current session data. 00544 */ 00545 struct _ssl_session 00546 { 00547 #if defined(POLARSSL_HAVE_TIME) 00548 time_t start; /*!< starting time */ 00549 #endif 00550 int ciphersuite; /*!< chosen ciphersuite */ 00551 int compression; /*!< chosen compression */ 00552 size_t length; /*!< session id length */ 00553 unsigned char id[32]; /*!< session identifier */ 00554 unsigned char master[48]; /*!< the master secret */ 00555 00556 #if defined(POLARSSL_X509_CRT_PARSE_C) 00557 x509_crt *peer_cert; /*!< peer X.509 cert chain */ 00558 #endif /* POLARSSL_X509_CRT_PARSE_C */ 00559 int verify_result; /*!< verification result */ 00560 00561 #if defined(POLARSSL_SSL_SESSION_TICKETS) 00562 unsigned char *ticket; /*!< RFC 5077 session ticket */ 00563 size_t ticket_len; /*!< session ticket length */ 00564 uint32_t ticket_lifetime; /*!< ticket lifetime hint */ 00565 #endif /* POLARSSL_SSL_SESSION_TICKETS */ 00566 00567 #if defined(POLARSSL_SSL_MAX_FRAGMENT_LENGTH) 00568 unsigned char mfl_code; /*!< MaxFragmentLength negotiated by peer */ 00569 #endif /* POLARSSL_SSL_MAX_FRAGMENT_LENGTH */ 00570 00571 #if defined(POLARSSL_SSL_TRUNCATED_HMAC) 00572 int trunc_hmac; /*!< flag for truncated hmac activation */ 00573 #endif /* POLARSSL_SSL_TRUNCATED_HMAC */ 00574 00575 #if defined(POLARSSL_SSL_ENCRYPT_THEN_MAC) 00576 int encrypt_then_mac; /*!< flag for EtM activation */ 00577 #endif 00578 }; 00579 00580 /* 00581 * This structure contains a full set of runtime transform parameters 00582 * either in negotiation or active. 00583 */ 00584 struct _ssl_transform 00585 { 00586 /* 00587 * Session specific crypto layer 00588 */ 00589 const ssl_ciphersuite_t *ciphersuite_info; 00590 /*!< Chosen cipersuite_info */ 00591 unsigned int keylen; /*!< symmetric key length */ 00592 size_t minlen; /*!< min. ciphertext length */ 00593 size_t ivlen; /*!< IV length */ 00594 size_t fixed_ivlen; /*!< Fixed part of IV (AEAD) */ 00595 size_t maclen; /*!< MAC length */ 00596 00597 unsigned char iv_enc[16]; /*!< IV (encryption) */ 00598 unsigned char iv_dec[16]; /*!< IV (decryption) */ 00599 00600 #if defined(POLARSSL_SSL_PROTO_SSL3) 00601 /* Needed only for SSL v3.0 secret */ 00602 unsigned char mac_enc[20]; /*!< SSL v3.0 secret (enc) */ 00603 unsigned char mac_dec[20]; /*!< SSL v3.0 secret (dec) */ 00604 #endif /* POLARSSL_SSL_PROTO_SSL3 */ 00605 00606 md_context_t md_ctx_enc; /*!< MAC (encryption) */ 00607 md_context_t md_ctx_dec; /*!< MAC (decryption) */ 00608 00609 cipher_context_t cipher_ctx_enc; /*!< encryption context */ 00610 cipher_context_t cipher_ctx_dec; /*!< decryption context */ 00611 00612 /* 00613 * Session specific compression layer 00614 */ 00615 #if defined(POLARSSL_ZLIB_SUPPORT) 00616 z_stream ctx_deflate; /*!< compression context */ 00617 z_stream ctx_inflate; /*!< decompression context */ 00618 #endif 00619 }; 00620 00621 /* 00622 * This structure contains the parameters only needed during handshake. 00623 */ 00624 struct _ssl_handshake_params 00625 { 00626 /* 00627 * Handshake specific crypto variables 00628 */ 00629 int sig_alg; /*!< Hash algorithm for signature */ 00630 int cert_type; /*!< Requested cert type */ 00631 int verify_sig_alg; /*!< Signature algorithm for verify */ 00632 #if defined(POLARSSL_DHM_C) 00633 dhm_context dhm_ctx; /*!< DHM key exchange */ 00634 #endif 00635 #if defined(POLARSSL_ECDH_C) 00636 ecdh_context ecdh_ctx; /*!< ECDH key exchange */ 00637 #endif 00638 #if defined(POLARSSL_ECDH_C) || defined(POLARSSL_ECDSA_C) 00639 const ecp_curve_info **curves; /*!< Supported elliptic curves */ 00640 #endif 00641 #if defined(POLARSSL_X509_CRT_PARSE_C) 00642 /** 00643 * Current key/cert or key/cert list. 00644 * On client: pointer to ssl->key_cert, only the first entry used. 00645 * On server: starts as a pointer to ssl->key_cert, then becomes 00646 * a pointer to the chosen key from this list or the SNI list. 00647 */ 00648 ssl_key_cert *key_cert; 00649 #if defined(POLARSSL_SSL_SERVER_NAME_INDICATION) 00650 ssl_key_cert *sni_key_cert; /*!< key/cert list from SNI */ 00651 #endif 00652 #endif /* POLARSSL_X509_CRT_PARSE_C */ 00653 00654 /* 00655 * Checksum contexts 00656 */ 00657 #if defined(POLARSSL_SSL_PROTO_SSL3) || defined(POLARSSL_SSL_PROTO_TLS1) || \ 00658 defined(POLARSSL_SSL_PROTO_TLS1_1) 00659 md5_context fin_md5; 00660 sha1_context fin_sha1; 00661 #endif 00662 #if defined(POLARSSL_SSL_PROTO_TLS1_2) 00663 #if defined(POLARSSL_SHA256_C) 00664 sha256_context fin_sha256; 00665 #endif 00666 #if defined(POLARSSL_SHA512_C) 00667 sha512_context fin_sha512; 00668 #endif 00669 #endif /* POLARSSL_SSL_PROTO_TLS1_2 */ 00670 00671 void (*update_checksum)(ssl_context *, const unsigned char *, size_t); 00672 void (*calc_verify)(ssl_context *, unsigned char *); 00673 void (*calc_finished)(ssl_context *, unsigned char *, int); 00674 int (*tls_prf)(const unsigned char *, size_t, const char *, 00675 const unsigned char *, size_t, 00676 unsigned char *, size_t); 00677 00678 size_t pmslen; /*!< premaster length */ 00679 00680 unsigned char randbytes[64]; /*!< random bytes */ 00681 unsigned char premaster[POLARSSL_PREMASTER_SIZE]; 00682 /*!< premaster secret */ 00683 00684 int resume; /*!< session resume indicator*/ 00685 int max_major_ver; /*!< max. major version client*/ 00686 int max_minor_ver; /*!< max. minor version client*/ 00687 int cli_exts; /*!< client extension presence*/ 00688 00689 #if defined(POLARSSL_SSL_SESSION_TICKETS) 00690 int new_session_ticket; /*!< use NewSessionTicket? */ 00691 #endif /* POLARSSL_SSL_SESSION_TICKETS */ 00692 #if defined(POLARSSL_SSL_EXTENDED_MASTER_SECRET) 00693 int extended_ms; /*!< use Extended Master Secret? */ 00694 #endif 00695 }; 00696 00697 #if defined(POLARSSL_SSL_SESSION_TICKETS) 00698 /* 00699 * Parameters needed to secure session tickets 00700 */ 00701 struct _ssl_ticket_keys 00702 { 00703 unsigned char key_name[16]; /*!< name to quickly discard bad tickets */ 00704 aes_context enc; /*!< encryption context */ 00705 aes_context dec; /*!< decryption context */ 00706 unsigned char mac_key[16]; /*!< authentication key */ 00707 }; 00708 #endif /* POLARSSL_SSL_SESSION_TICKETS */ 00709 00710 #if defined(POLARSSL_X509_CRT_PARSE_C) 00711 /* 00712 * List of certificate + private key pairs 00713 */ 00714 struct _ssl_key_cert 00715 { 00716 x509_crt *cert; /*!< cert */ 00717 pk_context *key; /*!< private key */ 00718 int key_own_alloc; /*!< did we allocate key? */ 00719 ssl_key_cert *next; /*!< next key/cert pair */ 00720 }; 00721 #endif /* POLARSSL_X509_CRT_PARSE_C */ 00722 00723 struct _ssl_context 00724 { 00725 /* 00726 * Miscellaneous 00727 */ 00728 int state; /*!< SSL handshake: current state */ 00729 int renegotiation; /*!< Initial or renegotiation */ 00730 #if defined(POLARSSL_SSL_RENEGOTIATION) 00731 int renego_records_seen; /*!< Records since renego request */ 00732 #endif 00733 00734 int major_ver; /*!< equal to SSL_MAJOR_VERSION_3 */ 00735 int minor_ver; /*!< either 0 (SSL3) or 1 (TLS1.0) */ 00736 00737 int max_major_ver; /*!< max. major version used */ 00738 int max_minor_ver; /*!< max. minor version used */ 00739 int min_major_ver; /*!< min. major version used */ 00740 int min_minor_ver; /*!< min. minor version used */ 00741 00742 #if defined(POLARSSL_SSL_FALLBACK_SCSV) && defined(POLARSSL_SSL_CLI_C) 00743 char fallback; /*!< flag for fallback connections */ 00744 #endif 00745 #if defined(POLARSSL_SSL_ENCRYPT_THEN_MAC) 00746 char encrypt_then_mac; /*!< flag for encrypt-then-mac */ 00747 #endif 00748 #if defined(POLARSSL_SSL_EXTENDED_MASTER_SECRET) 00749 char extended_ms; /*!< flag for extended master secret */ 00750 #endif 00751 char arc4_disabled; /*!< flag for disabling RC4 */ 00752 00753 /* 00754 * Callbacks (RNG, debug, I/O, verification) 00755 */ 00756 int (*f_rng)(void *, unsigned char *, size_t); 00757 void (*f_dbg)(void *, int, const char *); 00758 int (*f_recv)(void *, unsigned char *, size_t); 00759 int (*f_send)(void *, const unsigned char *, size_t); 00760 int (*f_get_cache)(void *, ssl_session *); 00761 int (*f_set_cache)(void *, const ssl_session *); 00762 00763 void *p_rng; /*!< context for the RNG function */ 00764 void *p_dbg; /*!< context for the debug function */ 00765 void *p_recv; /*!< context for reading operations */ 00766 void *p_send; /*!< context for writing operations */ 00767 void *p_get_cache; /*!< context for cache retrieval */ 00768 void *p_set_cache; /*!< context for cache store */ 00769 void *p_hw_data; /*!< context for HW acceleration */ 00770 00771 #if defined(POLARSSL_SSL_SERVER_NAME_INDICATION) 00772 int (*f_sni)(void *, ssl_context *, const unsigned char *, size_t); 00773 void *p_sni; /*!< context for SNI extension */ 00774 #endif 00775 00776 #if defined(POLARSSL_X509_CRT_PARSE_C) 00777 int (*f_vrfy)(void *, x509_crt *, int, int *); 00778 void *p_vrfy; /*!< context for verification */ 00779 #endif 00780 00781 #if defined(POLARSSL_KEY_EXCHANGE__SOME__PSK_ENABLED) 00782 int (*f_psk)(void *, ssl_context *, const unsigned char *, size_t); 00783 void *p_psk; /*!< context for PSK retrieval */ 00784 #endif 00785 00786 /* 00787 * Session layer 00788 */ 00789 ssl_session *session_in; /*!< current session data (in) */ 00790 ssl_session *session_out; /*!< current session data (out) */ 00791 ssl_session *session; /*!< negotiated session data */ 00792 ssl_session *session_negotiate; /*!< session data in negotiation */ 00793 00794 ssl_handshake_params *handshake; /*!< params required only during 00795 the handshake process */ 00796 00797 /* 00798 * Record layer transformations 00799 */ 00800 ssl_transform *transform_in; /*!< current transform params (in) */ 00801 ssl_transform *transform_out; /*!< current transform params (in) */ 00802 ssl_transform *transform; /*!< negotiated transform params */ 00803 ssl_transform *transform_negotiate; /*!< transform params in negotiation */ 00804 00805 /* 00806 * Record layer (incoming data) 00807 */ 00808 unsigned char *in_ctr; /*!< 64-bit incoming message counter */ 00809 unsigned char *in_hdr; /*!< 5-byte record header (in_ctr+8) */ 00810 unsigned char *in_iv; /*!< ivlen-byte IV (in_hdr+5) */ 00811 unsigned char *in_msg; /*!< message contents (in_iv+ivlen) */ 00812 unsigned char *in_offt; /*!< read offset in application data */ 00813 00814 int in_msgtype; /*!< record header: message type */ 00815 size_t in_msglen; /*!< record header: message length */ 00816 size_t in_left; /*!< amount of data read so far */ 00817 00818 size_t in_hslen; /*!< current handshake message length */ 00819 int nb_zero; /*!< # of 0-length encrypted messages */ 00820 int record_read; /*!< record is already present */ 00821 00822 /* 00823 * Record layer (outgoing data) 00824 */ 00825 unsigned char *out_ctr; /*!< 64-bit outgoing message counter */ 00826 unsigned char *out_hdr; /*!< 5-byte record header (out_ctr+8) */ 00827 unsigned char *out_iv; /*!< ivlen-byte IV (out_hdr+5) */ 00828 unsigned char *out_msg; /*!< message contents (out_iv+ivlen) */ 00829 00830 int out_msgtype; /*!< record header: message type */ 00831 size_t out_msglen; /*!< record header: message length */ 00832 size_t out_left; /*!< amount of data not yet written */ 00833 00834 #if defined(POLARSSL_ZLIB_SUPPORT) 00835 unsigned char *compress_buf; /*!< zlib data buffer */ 00836 #endif 00837 #if defined(POLARSSL_SSL_MAX_FRAGMENT_LENGTH) 00838 unsigned char mfl_code; /*!< MaxFragmentLength chosen by us */ 00839 #endif /* POLARSSL_SSL_MAX_FRAGMENT_LENGTH */ 00840 #if defined(POLARSSL_SSL_CBC_RECORD_SPLITTING) 00841 signed char split_done; /*!< flag for record splitting: 00842 -1 disabled, 0 todo, 1 done */ 00843 #endif 00844 00845 /* 00846 * PKI layer 00847 */ 00848 #if defined(POLARSSL_X509_CRT_PARSE_C) 00849 ssl_key_cert *key_cert; /*!< own certificate(s)/key(s) */ 00850 00851 x509_crt *ca_chain; /*!< own trusted CA chain */ 00852 x509_crl *ca_crl; /*!< trusted CA CRLs */ 00853 const char *peer_cn; /*!< expected peer CN */ 00854 #endif /* POLARSSL_X509_CRT_PARSE_C */ 00855 00856 /* 00857 * Support for generating and checking session tickets 00858 */ 00859 #if defined(POLARSSL_SSL_SESSION_TICKETS) 00860 ssl_ticket_keys *ticket_keys; /*!< keys for ticket encryption */ 00861 #endif /* POLARSSL_SSL_SESSION_TICKETS */ 00862 00863 /* 00864 * User settings 00865 */ 00866 int endpoint; /*!< 0: client, 1: server */ 00867 int authmode; /*!< verification mode */ 00868 int client_auth; /*!< flag for client auth. */ 00869 int verify_result; /*!< verification result */ 00870 #if defined(POLARSSL_SSL_RENEGOTIATION) 00871 int disable_renegotiation; /*!< enable/disable renegotiation */ 00872 int renego_max_records; /*!< grace period for renegotiation */ 00873 unsigned char renego_period[8]; /*!< value of the record counters 00874 that triggers renegotiation */ 00875 #endif 00876 int allow_legacy_renegotiation; /*!< allow legacy renegotiation */ 00877 const int *ciphersuite_list[4]; /*!< allowed ciphersuites / version */ 00878 #if defined(POLARSSL_SSL_SET_CURVES) 00879 const ecp_group_id *curve_list; /*!< allowed curves */ 00880 #endif 00881 #if defined(POLARSSL_SSL_TRUNCATED_HMAC) 00882 int trunc_hmac; /*!< negotiate truncated hmac? */ 00883 #endif 00884 #if defined(POLARSSL_SSL_SESSION_TICKETS) 00885 int session_tickets; /*!< use session tickets? */ 00886 int ticket_lifetime; /*!< session ticket lifetime */ 00887 #endif 00888 00889 #if defined(POLARSSL_DHM_C) 00890 mpi dhm_P; /*!< prime modulus for DHM */ 00891 mpi dhm_G; /*!< generator for DHM */ 00892 #endif 00893 00894 #if defined(POLARSSL_KEY_EXCHANGE__SOME__PSK_ENABLED) 00895 /* 00896 * PSK values 00897 */ 00898 unsigned char *psk; 00899 size_t psk_len; 00900 unsigned char *psk_identity; 00901 size_t psk_identity_len; 00902 #endif 00903 00904 #if defined(POLARSSL_SSL_SERVER_NAME_INDICATION) 00905 /* 00906 * SNI extension 00907 */ 00908 unsigned char *hostname; 00909 size_t hostname_len; 00910 #endif 00911 00912 #if defined(POLARSSL_SSL_ALPN) 00913 /* 00914 * ALPN extension 00915 */ 00916 const char **alpn_list; /*!< ordered list of supported protocols */ 00917 const char *alpn_chosen; /*!< negotiated protocol */ 00918 #endif 00919 00920 /* 00921 * Secure renegotiation 00922 */ 00923 int secure_renegotiation; /*!< does peer support legacy or 00924 secure renegotiation */ 00925 #if defined(POLARSSL_SSL_RENEGOTIATION) 00926 size_t verify_data_len; /*!< length of verify data stored */ 00927 char own_verify_data[SSL_VERIFY_DATA_MAX_LEN]; /*!< previous handshake verify data */ 00928 char peer_verify_data[SSL_VERIFY_DATA_MAX_LEN]; /*!< previous handshake verify data */ 00929 #endif 00930 }; 00931 00932 #if defined(POLARSSL_SSL_HW_RECORD_ACCEL) 00933 00934 #define SSL_CHANNEL_OUTBOUND 0 00935 #define SSL_CHANNEL_INBOUND 1 00936 00937 extern int (*ssl_hw_record_init)(ssl_context *ssl, 00938 const unsigned char *key_enc, const unsigned char *key_dec, 00939 size_t keylen, 00940 const unsigned char *iv_enc, const unsigned char *iv_dec, 00941 size_t ivlen, 00942 const unsigned char *mac_enc, const unsigned char *mac_dec, 00943 size_t maclen); 00944 extern int (*ssl_hw_record_activate)(ssl_context *ssl, int direction); 00945 extern int (*ssl_hw_record_reset)(ssl_context *ssl); 00946 extern int (*ssl_hw_record_write)(ssl_context *ssl); 00947 extern int (*ssl_hw_record_read)(ssl_context *ssl); 00948 extern int (*ssl_hw_record_finish)(ssl_context *ssl); 00949 #endif /* POLARSSL_SSL_HW_RECORD_ACCEL */ 00950 00951 /** 00952 * \brief Returns the list of ciphersuites supported by the SSL/TLS module. 00953 * 00954 * \return a statically allocated array of ciphersuites, the last 00955 * entry is 0. 00956 */ 00957 const int *ssl_list_ciphersuites( void ); 00958 00959 /** 00960 * \brief Return the name of the ciphersuite associated with the 00961 * given ID 00962 * 00963 * \param ciphersuite_id SSL ciphersuite ID 00964 * 00965 * \return a string containing the ciphersuite name 00966 */ 00967 const char *ssl_get_ciphersuite_name( const int ciphersuite_id ); 00968 00969 /** 00970 * \brief Return the ID of the ciphersuite associated with the 00971 * given name 00972 * 00973 * \param ciphersuite_name SSL ciphersuite name 00974 * 00975 * \return the ID with the ciphersuite or 0 if not found 00976 */ 00977 int ssl_get_ciphersuite_id( const char *ciphersuite_name ); 00978 00979 /** 00980 * \brief Initialize an SSL context 00981 * (An individual SSL context is not thread-safe) 00982 * 00983 * \param ssl SSL context 00984 * 00985 * \return 0 if successful, or POLARSSL_ERR_SSL_MALLOC_FAILED if 00986 * memory allocation failed 00987 */ 00988 int ssl_init( ssl_context *ssl ); 00989 00990 /** 00991 * \brief Reset an already initialized SSL context for re-use 00992 * while retaining application-set variables, function 00993 * pointers and data. 00994 * 00995 * \param ssl SSL context 00996 * \return 0 if successful, or POLASSL_ERR_SSL_MALLOC_FAILED, 00997 POLARSSL_ERR_SSL_HW_ACCEL_FAILED or 00998 * POLARSSL_ERR_SSL_COMPRESSION_FAILED 00999 */ 01000 int ssl_session_reset( ssl_context *ssl ); 01001 01002 /** 01003 * \brief Set the current endpoint type 01004 * 01005 * \param ssl SSL context 01006 * \param endpoint must be SSL_IS_CLIENT or SSL_IS_SERVER 01007 * 01008 * \note This function should be called right after ssl_init() since 01009 * some other ssl_set_foo() functions depend on it. 01010 */ 01011 void ssl_set_endpoint( ssl_context *ssl, int endpoint ); 01012 01013 /** 01014 * \brief Set the certificate verification mode 01015 * 01016 * \param ssl SSL context 01017 * \param authmode can be: 01018 * 01019 * SSL_VERIFY_NONE: peer certificate is not checked (default), 01020 * this is insecure and SHOULD be avoided. 01021 * 01022 * SSL_VERIFY_OPTIONAL: peer certificate is checked, however the 01023 * handshake continues even if verification failed; 01024 * ssl_get_verify_result() can be called after the 01025 * handshake is complete. 01026 * 01027 * SSL_VERIFY_REQUIRED: peer *must* present a valid certificate, 01028 * handshake is aborted if verification failed. 01029 * 01030 * \note On client, SSL_VERIFY_REQUIRED is the recommended mode. 01031 * With SSL_VERIFY_OPTIONAL, the user needs to call ssl_get_verify_result() at 01032 * the right time(s), which may not be obvious, while REQUIRED always perform 01033 * the verification as soon as possible. For example, REQUIRED was protecting 01034 * against the "triple handshake" attack even before it was found. 01035 */ 01036 void ssl_set_authmode( ssl_context *ssl, int authmode ); 01037 01038 #if defined(POLARSSL_X509_CRT_PARSE_C) 01039 /** 01040 * \brief Set the verification callback (Optional). 01041 * 01042 * If set, the verify callback is called for each 01043 * certificate in the chain. For implementation 01044 * information, please see \c x509parse_verify() 01045 * 01046 * \param ssl SSL context 01047 * \param f_vrfy verification function 01048 * \param p_vrfy verification parameter 01049 */ 01050 void ssl_set_verify( ssl_context *ssl, 01051 int (*f_vrfy)(void *, x509_crt *, int, int *), 01052 void *p_vrfy ); 01053 #endif /* POLARSSL_X509_CRT_PARSE_C */ 01054 01055 /** 01056 * \brief Set the random number generator callback 01057 * 01058 * \param ssl SSL context 01059 * \param f_rng RNG function 01060 * \param p_rng RNG parameter 01061 */ 01062 void ssl_set_rng( ssl_context *ssl, 01063 int (*f_rng)(void *, unsigned char *, size_t), 01064 void *p_rng ); 01065 01066 /** 01067 * \brief Set the debug callback 01068 * 01069 * \param ssl SSL context 01070 * \param f_dbg debug function 01071 * \param p_dbg debug parameter 01072 */ 01073 void ssl_set_dbg( ssl_context *ssl, 01074 void (*f_dbg)(void *, int, const char *), 01075 void *p_dbg ); 01076 01077 /** 01078 * \brief Set the underlying BIO read and write callbacks 01079 * 01080 * \param ssl SSL context 01081 * \param f_recv read callback 01082 * \param p_recv read parameter 01083 * \param f_send write callback 01084 * \param p_send write parameter 01085 */ 01086 void ssl_set_bio( ssl_context *ssl, 01087 int (*f_recv)(void *, unsigned char *, size_t), void *p_recv, 01088 int (*f_send)(void *, const unsigned char *, size_t), void *p_send ); 01089 01090 #if defined(POLARSSL_SSL_SRV_C) 01091 /** 01092 * \brief Set the session cache callbacks (server-side only) 01093 * If not set, no session resuming is done (except if session 01094 * tickets are enabled too). 01095 * 01096 * The session cache has the responsibility to check for stale 01097 * entries based on timeout. See RFC 5246 for recommendations. 01098 * 01099 * Warning: session.peer_cert is cleared by the SSL/TLS layer on 01100 * connection shutdown, so do not cache the pointer! Either set 01101 * it to NULL or make a full copy of the certificate. 01102 * 01103 * The get callback is called once during the initial handshake 01104 * to enable session resuming. The get function has the 01105 * following parameters: (void *parameter, ssl_session *session) 01106 * If a valid entry is found, it should fill the master of 01107 * the session object with the cached values and return 0, 01108 * return 1 otherwise. Optionally peer_cert can be set as well 01109 * if it is properly present in cache entry. 01110 * 01111 * The set callback is called once during the initial handshake 01112 * to enable session resuming after the entire handshake has 01113 * been finished. The set function has the following parameters: 01114 * (void *parameter, const ssl_session *session). The function 01115 * should create a cache entry for future retrieval based on 01116 * the data in the session structure and should keep in mind 01117 * that the ssl_session object presented (and all its referenced 01118 * data) is cleared by the SSL/TLS layer when the connection is 01119 * terminated. It is recommended to add metadata to determine if 01120 * an entry is still valid in the future. Return 0 if 01121 * successfully cached, return 1 otherwise. 01122 * 01123 * \param ssl SSL context 01124 * \param f_get_cache session get callback 01125 * \param p_get_cache session get parameter 01126 * \param f_set_cache session set callback 01127 * \param p_set_cache session set parameter 01128 */ 01129 void ssl_set_session_cache( ssl_context *ssl, 01130 int (*f_get_cache)(void *, ssl_session *), void *p_get_cache, 01131 int (*f_set_cache)(void *, const ssl_session *), void *p_set_cache ); 01132 #endif /* POLARSSL_SSL_SRV_C */ 01133 01134 #if defined(POLARSSL_SSL_CLI_C) 01135 /** 01136 * \brief Request resumption of session (client-side only) 01137 * Session data is copied from presented session structure. 01138 * 01139 * \param ssl SSL context 01140 * \param session session context 01141 * 01142 * \return 0 if successful, 01143 * POLARSSL_ERR_SSL_MALLOC_FAILED if memory allocation failed, 01144 * POLARSSL_ERR_SSL_BAD_INPUT_DATA if used server-side or 01145 * arguments are otherwise invalid 01146 * 01147 * \sa ssl_get_session() 01148 */ 01149 int ssl_set_session( ssl_context *ssl, const ssl_session *session ); 01150 #endif /* POLARSSL_SSL_CLI_C */ 01151 01152 /** 01153 * \brief Set the list of allowed ciphersuites and the preference 01154 * order. First in the list has the highest preference. 01155 * (Overrides all version specific lists) 01156 * 01157 * The ciphersuites array is not copied, and must remain 01158 * valid for the lifetime of the ssl_context. 01159 * 01160 * Note: The server uses its own preferences 01161 * over the preference of the client unless 01162 * POLARSSL_SSL_SRV_RESPECT_CLIENT_PREFERENCE is defined! 01163 * 01164 * \param ssl SSL context 01165 * \param ciphersuites 0-terminated list of allowed ciphersuites 01166 */ 01167 void ssl_set_ciphersuites( ssl_context *ssl, const int *ciphersuites ); 01168 01169 /** 01170 * \brief Set the list of allowed ciphersuites and the 01171 * preference order for a specific version of the protocol. 01172 * (Only useful on the server side) 01173 * 01174 * \param ssl SSL context 01175 * \param ciphersuites 0-terminated list of allowed ciphersuites 01176 * \param major Major version number (only SSL_MAJOR_VERSION_3 01177 * supported) 01178 * \param minor Minor version number (SSL_MINOR_VERSION_0, 01179 * SSL_MINOR_VERSION_1 and SSL_MINOR_VERSION_2, 01180 * SSL_MINOR_VERSION_3 supported) 01181 */ 01182 void ssl_set_ciphersuites_for_version( ssl_context *ssl, 01183 const int *ciphersuites, 01184 int major, int minor ); 01185 01186 #if defined(POLARSSL_X509_CRT_PARSE_C) 01187 /** 01188 * \brief Set the data required to verify peer certificate 01189 * 01190 * \param ssl SSL context 01191 * \param ca_chain trusted CA chain (meaning all fully trusted top-level CAs) 01192 * \param ca_crl trusted CA CRLs 01193 * \param peer_cn expected peer CommonName (or NULL) 01194 */ 01195 void ssl_set_ca_chain( ssl_context *ssl, x509_crt *ca_chain, 01196 x509_crl *ca_crl, const char *peer_cn ); 01197 01198 /** 01199 * \brief Set own certificate chain and private key 01200 * 01201 * \note own_cert should contain in order from the bottom up your 01202 * certificate chain. The top certificate (self-signed) 01203 * can be omitted. 01204 * 01205 * \note This function may be called more than once if you want to 01206 * support multiple certificates (eg, one using RSA and one 01207 * using ECDSA). However, on client, currently only the first 01208 * certificate is used (subsequent calls have no effect). 01209 * 01210 * \param ssl SSL context 01211 * \param own_cert own public certificate chain 01212 * \param pk_key own private key 01213 * 01214 * \return 0 on success or POLARSSL_ERR_SSL_MALLOC_FAILED 01215 */ 01216 int ssl_set_own_cert( ssl_context *ssl, x509_crt *own_cert, 01217 pk_context *pk_key ); 01218 01219 #if ! defined(POLARSSL_DEPRECATED_REMOVED) 01220 #if defined(POLARSSL_DEPRECATED_WARNING) 01221 #define DEPRECATED __attribute__((deprecated)) 01222 #else 01223 #define DEPRECATED 01224 #endif 01225 #if defined(POLARSSL_RSA_C) 01226 /** 01227 * \brief Set own certificate chain and private RSA key 01228 * 01229 * Note: own_cert should contain IN order from the bottom 01230 * up your certificate chain. The top certificate (self-signed) 01231 * can be omitted. 01232 * 01233 * \deprecated Please use \c ssl_set_own_cert() instead. 01234 * 01235 * \param ssl SSL context 01236 * \param own_cert own public certificate chain 01237 * \param rsa_key own private RSA key 01238 * 01239 * \return 0 on success, or a specific error code. 01240 */ 01241 int ssl_set_own_cert_rsa( ssl_context *ssl, x509_crt *own_cert, 01242 rsa_context *rsa_key ) DEPRECATED; 01243 #endif /* POLARSSL_RSA_C */ 01244 01245 /** 01246 * \brief Set own certificate and external RSA private 01247 * key and handling callbacks, such as the PKCS#11 wrappers 01248 * or any other external private key handler. 01249 * (see the respective RSA functions in rsa.h for documentation 01250 * of the callback parameters, with the only change being 01251 * that the rsa_context * is a void * in the callbacks) 01252 * 01253 * Note: own_cert should contain IN order from the bottom 01254 * up your certificate chain. The top certificate (self-signed) 01255 * can be omitted. 01256 * 01257 * \deprecated Please use \c pk_init_ctx_rsa_alt() 01258 * and \c ssl_set_own_cert() instead. 01259 * 01260 * \param ssl SSL context 01261 * \param own_cert own public certificate chain 01262 * \param rsa_key alternate implementation private RSA key 01263 * \param rsa_decrypt alternate implementation of \c rsa_pkcs1_decrypt() 01264 * \param rsa_sign alternate implementation of \c rsa_pkcs1_sign() 01265 * \param rsa_key_len function returning length of RSA key in bytes 01266 * 01267 * \return 0 on success, or a specific error code. 01268 */ 01269 int ssl_set_own_cert_alt( ssl_context *ssl, x509_crt *own_cert, 01270 void *rsa_key, 01271 rsa_decrypt_func rsa_decrypt, 01272 rsa_sign_func rsa_sign, 01273 rsa_key_len_func rsa_key_len ) DEPRECATED; 01274 #undef DEPRECATED 01275 #endif /* POLARSSL_DEPRECATED_REMOVED */ 01276 #endif /* POLARSSL_X509_CRT_PARSE_C */ 01277 01278 #if defined(POLARSSL_KEY_EXCHANGE__SOME__PSK_ENABLED) 01279 /** 01280 * \brief Set the Pre Shared Key (PSK) and the identity name connected 01281 * to it. 01282 * 01283 * \param ssl SSL context 01284 * \param psk pointer to the pre-shared key 01285 * \param psk_len pre-shared key length 01286 * \param psk_identity pointer to the pre-shared key identity 01287 * \param psk_identity_len identity key length 01288 * 01289 * \return 0 if successful or POLARSSL_ERR_SSL_MALLOC_FAILED 01290 */ 01291 int ssl_set_psk( ssl_context *ssl, const unsigned char *psk, size_t psk_len, 01292 const unsigned char *psk_identity, size_t psk_identity_len ); 01293 01294 /** 01295 * \brief Set the PSK callback (server-side only) (Optional). 01296 * 01297 * If set, the PSK callback is called for each 01298 * handshake where a PSK ciphersuite was negotiated. 01299 * The caller provides the identity received and wants to 01300 * receive the actual PSK data and length. 01301 * 01302 * The callback has the following parameters: (void *parameter, 01303 * ssl_context *ssl, const unsigned char *psk_identity, 01304 * size_t identity_len) 01305 * If a valid PSK identity is found, the callback should use 01306 * ssl_set_psk() on the ssl context to set the correct PSK and 01307 * identity and return 0. 01308 * Any other return value will result in a denied PSK identity. 01309 * 01310 * \param ssl SSL context 01311 * \param f_psk PSK identity function 01312 * \param p_psk PSK identity parameter 01313 */ 01314 void ssl_set_psk_cb( ssl_context *ssl, 01315 int (*f_psk)(void *, ssl_context *, const unsigned char *, 01316 size_t), 01317 void *p_psk ); 01318 #endif /* POLARSSL_KEY_EXCHANGE__SOME__PSK_ENABLED */ 01319 01320 #if defined(POLARSSL_DHM_C) 01321 /** 01322 * \brief Set the Diffie-Hellman public P and G values, 01323 * read as hexadecimal strings (server-side only) 01324 * (Default: POLARSSL_DHM_RFC5114_MODP_1024_[PG]) 01325 * 01326 * \param ssl SSL context 01327 * \param dhm_P Diffie-Hellman-Merkle modulus 01328 * \param dhm_G Diffie-Hellman-Merkle generator 01329 * 01330 * \return 0 if successful 01331 */ 01332 int ssl_set_dh_param( ssl_context *ssl, const char *dhm_P, const char *dhm_G ); 01333 01334 /** 01335 * \brief Set the Diffie-Hellman public P and G values, 01336 * read from existing context (server-side only) 01337 * 01338 * \param ssl SSL context 01339 * \param dhm_ctx Diffie-Hellman-Merkle context 01340 * 01341 * \return 0 if successful 01342 */ 01343 int ssl_set_dh_param_ctx( ssl_context *ssl, dhm_context *dhm_ctx ); 01344 #endif /* POLARSSL_DHM_C */ 01345 01346 #if defined(POLARSSL_SSL_SET_CURVES) 01347 /** 01348 * \brief Set the allowed curves in order of preference. 01349 * (Default: all defined curves.) 01350 * 01351 * On server: this only affects selection of the ECDHE curve; 01352 * the curves used for ECDH and ECDSA are determined by the 01353 * list of available certificates instead. 01354 * 01355 * On client: this affects the list of curves offered for any 01356 * use. The server can override our preference order. 01357 * 01358 * Both sides: limits the set of curves used by peer to the 01359 * listed curves for any use (ECDH(E), certificates). 01360 * 01361 * \param ssl SSL context 01362 * \param curves Ordered list of allowed curves, 01363 * terminated by POLARSSL_ECP_DP_NONE. 01364 */ 01365 void ssl_set_curves( ssl_context *ssl, const ecp_group_id *curves ); 01366 #endif /* POLARSSL_SSL_SET_CURVES */ 01367 01368 #if defined(POLARSSL_SSL_SERVER_NAME_INDICATION) 01369 /** 01370 * \brief Set hostname for ServerName TLS extension 01371 * (client-side only) 01372 * 01373 * 01374 * \param ssl SSL context 01375 * \param hostname the server hostname 01376 * 01377 * \return 0 if successful or POLARSSL_ERR_SSL_MALLOC_FAILED 01378 */ 01379 int ssl_set_hostname( ssl_context *ssl, const char *hostname ); 01380 01381 /** 01382 * \brief Set server side ServerName TLS extension callback 01383 * (optional, server-side only). 01384 * 01385 * If set, the ServerName callback is called whenever the 01386 * server receives a ServerName TLS extension from the client 01387 * during a handshake. The ServerName callback has the 01388 * following parameters: (void *parameter, ssl_context *ssl, 01389 * const unsigned char *hostname, size_t len). If a suitable 01390 * certificate is found, the callback should set the 01391 * certificate and key to use with ssl_set_own_cert() (and 01392 * possibly adjust the CA chain as well) and return 0. The 01393 * callback should return -1 to abort the handshake at this 01394 * point. 01395 * 01396 * \param ssl SSL context 01397 * \param f_sni verification function 01398 * \param p_sni verification parameter 01399 */ 01400 void ssl_set_sni( ssl_context *ssl, 01401 int (*f_sni)(void *, ssl_context *, const unsigned char *, 01402 size_t), 01403 void *p_sni ); 01404 #endif /* POLARSSL_SSL_SERVER_NAME_INDICATION */ 01405 01406 #if defined(POLARSSL_SSL_ALPN) 01407 /** 01408 * \brief Set the supported Application Layer Protocols. 01409 * 01410 * \param ssl SSL context 01411 * \param protos NULL-terminated list of supported protocols, 01412 * in decreasing preference order. 01413 * 01414 * \return 0 on success, or POLARSSL_ERR_SSL_BAD_INPUT_DATA. 01415 */ 01416 int ssl_set_alpn_protocols( ssl_context *ssl, const char **protos ); 01417 01418 /** 01419 * \brief Get the name of the negotiated Application Layer Protocol. 01420 * This function should be called after the handshake is 01421 * completed. 01422 * 01423 * \param ssl SSL context 01424 * 01425 * \return Protcol name, or NULL if no protocol was negotiated. 01426 */ 01427 const char *ssl_get_alpn_protocol( const ssl_context *ssl ); 01428 #endif /* POLARSSL_SSL_ALPN */ 01429 01430 /** 01431 * \brief Set the maximum supported version sent from the client side 01432 * and/or accepted at the server side 01433 * (Default: SSL_MAX_MAJOR_VERSION, SSL_MAX_MINOR_VERSION) 01434 * 01435 * Note: This ignores ciphersuites from 'higher' versions. 01436 * Note: Input outside of the SSL_MAX_XXXXX_VERSION and 01437 * SSL_MIN_XXXXX_VERSION range is ignored. 01438 * 01439 * \param ssl SSL context 01440 * \param major Major version number (only SSL_MAJOR_VERSION_3 supported) 01441 * \param minor Minor version number (SSL_MINOR_VERSION_0, 01442 * SSL_MINOR_VERSION_1 and SSL_MINOR_VERSION_2, 01443 * SSL_MINOR_VERSION_3 supported) 01444 */ 01445 void ssl_set_max_version( ssl_context *ssl, int major, int minor ); 01446 01447 /** 01448 * \brief Set the minimum accepted SSL/TLS protocol version 01449 * (Default: SSL_MIN_MAJOR_VERSION, SSL_MIN_MINOR_VERSION) 01450 * 01451 * \note Input outside of the SSL_MAX_XXXXX_VERSION and 01452 * SSL_MIN_XXXXX_VERSION range is ignored. 01453 * 01454 * \note SSL_MINOR_VERSION_0 (SSL v3) should be avoided. 01455 * 01456 * \param ssl SSL context 01457 * \param major Major version number (only SSL_MAJOR_VERSION_3 supported) 01458 * \param minor Minor version number (SSL_MINOR_VERSION_0, 01459 * SSL_MINOR_VERSION_1 and SSL_MINOR_VERSION_2, 01460 * SSL_MINOR_VERSION_3 supported) 01461 */ 01462 void ssl_set_min_version( ssl_context *ssl, int major, int minor ); 01463 01464 #if defined(POLARSSL_SSL_FALLBACK_SCSV) && defined(POLARSSL_SSL_CLI_C) 01465 /** 01466 * \brief Set the fallback flag (client-side only). 01467 * (Default: SSL_IS_NOT_FALLBACK). 01468 * 01469 * \note Set to SSL_IS_FALLBACK when preparing a fallback 01470 * connection, that is a connection with max_version set to a 01471 * lower value than the value you're willing to use. Such 01472 * fallback connections are not recommended but are sometimes 01473 * necessary to interoperate with buggy (version-intolerant) 01474 * servers. 01475 * 01476 * \warning You should NOT set this to SSL_IS_FALLBACK for 01477 * non-fallback connections! This would appear to work for a 01478 * while, then cause failures when the server is upgraded to 01479 * support a newer TLS version. 01480 * 01481 * \param ssl SSL context 01482 * \param fallback SSL_IS_NOT_FALLBACK or SSL_IS_FALLBACK 01483 */ 01484 void ssl_set_fallback( ssl_context *ssl, char fallback ); 01485 #endif /* POLARSSL_SSL_FALLBACK_SCSV && POLARSSL_SSL_CLI_C */ 01486 01487 #if defined(POLARSSL_SSL_ENCRYPT_THEN_MAC) 01488 /** 01489 * \brief Enable or disable Encrypt-then-MAC 01490 * (Default: SSL_ETM_ENABLED) 01491 * 01492 * \note This should always be enabled, it is a security 01493 * improvement, and should not cause any interoperability 01494 * issue (used only if the peer supports it too). 01495 * 01496 * \param ssl SSL context 01497 * \param etm SSL_ETM_ENABLED or SSL_ETM_DISABLED 01498 */ 01499 void ssl_set_encrypt_then_mac( ssl_context *ssl, char etm ); 01500 #endif /* POLARSSL_SSL_ENCRYPT_THEN_MAC */ 01501 01502 #if defined(POLARSSL_SSL_EXTENDED_MASTER_SECRET) 01503 /** 01504 * \brief Enable or disable Extended Master Secret negotiation. 01505 * (Default: SSL_EXTENDED_MS_ENABLED) 01506 * 01507 * \note This should always be enabled, it is a security fix to the 01508 * protocol, and should not cause any interoperability issue 01509 * (used only if the peer supports it too). 01510 * 01511 * \param ssl SSL context 01512 * \param ems SSL_EXTENDED_MS_ENABLED or SSL_EXTENDED_MS_DISABLED 01513 */ 01514 void ssl_set_extended_master_secret( ssl_context *ssl, char ems ); 01515 #endif /* POLARSSL_SSL_EXTENDED_MASTER_SECRET */ 01516 01517 /** 01518 * \brief Disable or enable support for RC4 01519 * (Default: SSL_ARC4_ENABLED) 01520 * 01521 * \note Though the default is RC4 for compatibility reasons in the 01522 * 1.3 branch, the recommended value is SSL_ARC4_DISABLED. 01523 * 01524 * \note This function will likely be removed in future versions as 01525 * RC4 will then be disabled by default at compile time. 01526 * 01527 * \param ssl SSL context 01528 * \param arc4 SSL_ARC4_ENABLED or SSL_ARC4_DISABLED 01529 */ 01530 void ssl_set_arc4_support( ssl_context *ssl, char arc4 ); 01531 01532 #if defined(POLARSSL_SSL_MAX_FRAGMENT_LENGTH) 01533 /** 01534 * \brief Set the maximum fragment length to emit and/or negotiate 01535 * (Default: SSL_MAX_CONTENT_LEN, usually 2^14 bytes) 01536 * (Server: set maximum fragment length to emit, 01537 * usually negotiated by the client during handshake 01538 * (Client: set maximum fragment length to emit *and* 01539 * negotiate with the server during handshake) 01540 * 01541 * \param ssl SSL context 01542 * \param mfl_code Code for maximum fragment length (allowed values: 01543 * SSL_MAX_FRAG_LEN_512, SSL_MAX_FRAG_LEN_1024, 01544 * SSL_MAX_FRAG_LEN_2048, SSL_MAX_FRAG_LEN_4096) 01545 * 01546 * \return 0 if successful or POLARSSL_ERR_SSL_BAD_INPUT_DATA 01547 */ 01548 int ssl_set_max_frag_len( ssl_context *ssl, unsigned char mfl_code ); 01549 #endif /* POLARSSL_SSL_MAX_FRAGMENT_LENGTH */ 01550 01551 #if defined(POLARSSL_SSL_TRUNCATED_HMAC) 01552 /** 01553 * \brief Activate negotiation of truncated HMAC 01554 * (Default: SSL_TRUNC_HMAC_DISABLED on client, 01555 * SSL_TRUNC_HMAC_ENABLED on server.) 01556 * 01557 * \param ssl SSL context 01558 * \param truncate Enable or disable (SSL_TRUNC_HMAC_ENABLED or 01559 * SSL_TRUNC_HMAC_DISABLED) 01560 * 01561 * \return Always 0. 01562 */ 01563 int ssl_set_truncated_hmac( ssl_context *ssl, int truncate ); 01564 #endif /* POLARSSL_SSL_TRUNCATED_HMAC */ 01565 01566 #if defined(POLARSSL_SSL_CBC_RECORD_SPLITTING) 01567 /** 01568 * \brief Enable / Disable 1/n-1 record splitting 01569 * (Default: SSL_CBC_RECORD_SPLITTING_ENABLED) 01570 * 01571 * \note Only affects SSLv3 and TLS 1.0, not higher versions. 01572 * Does not affect non-CBC ciphersuites in any version. 01573 * 01574 * \param ssl SSL context 01575 * \param split SSL_CBC_RECORD_SPLITTING_ENABLED or 01576 * SSL_CBC_RECORD_SPLITTING_DISABLED 01577 */ 01578 void ssl_set_cbc_record_splitting( ssl_context *ssl, char split ); 01579 #endif /* POLARSSL_SSL_CBC_RECORD_SPLITTING */ 01580 01581 #if defined(POLARSSL_SSL_SESSION_TICKETS) 01582 /** 01583 * \brief Enable / Disable session tickets 01584 * (Default: SSL_SESSION_TICKETS_ENABLED on client, 01585 * SSL_SESSION_TICKETS_DISABLED on server) 01586 * 01587 * \note On server, ssl_set_rng() must be called before this function 01588 * to allow generating the ticket encryption and 01589 * authentication keys. 01590 * 01591 * \param ssl SSL context 01592 * \param use_tickets Enable or disable (SSL_SESSION_TICKETS_ENABLED or 01593 * SSL_SESSION_TICKETS_DISABLED) 01594 * 01595 * \return 0 if successful, 01596 * or a specific error code (server only). 01597 */ 01598 int ssl_set_session_tickets( ssl_context *ssl, int use_tickets ); 01599 01600 /** 01601 * \brief Set session ticket lifetime (server only) 01602 * (Default: SSL_DEFAULT_TICKET_LIFETIME (86400 secs / 1 day)) 01603 * 01604 * \param ssl SSL context 01605 * \param lifetime session ticket lifetime 01606 */ 01607 void ssl_set_session_ticket_lifetime( ssl_context *ssl, int lifetime ); 01608 #endif /* POLARSSL_SSL_SESSION_TICKETS */ 01609 01610 #if defined(POLARSSL_SSL_RENEGOTIATION) 01611 /** 01612 * \brief Enable / Disable renegotiation support for connection when 01613 * initiated by peer 01614 * (Default: SSL_RENEGOTIATION_DISABLED) 01615 * 01616 * Note: A server with support enabled is more vulnerable for a 01617 * resource DoS by a malicious client. You should enable this on 01618 * a client to enable server-initiated renegotiation. 01619 * 01620 * \param ssl SSL context 01621 * \param renegotiation Enable or disable (SSL_RENEGOTIATION_ENABLED or 01622 * SSL_RENEGOTIATION_DISABLED) 01623 */ 01624 void ssl_set_renegotiation( ssl_context *ssl, int renegotiation ); 01625 #endif /* POLARSSL_SSL_RENEGOTIATION */ 01626 01627 /** 01628 * \brief Prevent or allow legacy renegotiation. 01629 * (Default: SSL_LEGACY_NO_RENEGOTIATION) 01630 * 01631 * SSL_LEGACY_NO_RENEGOTIATION allows connections to 01632 * be established even if the peer does not support 01633 * secure renegotiation, but does not allow renegotiation 01634 * to take place if not secure. 01635 * (Interoperable and secure option) 01636 * 01637 * SSL_LEGACY_ALLOW_RENEGOTIATION allows renegotiations 01638 * with non-upgraded peers. Allowing legacy renegotiation 01639 * makes the connection vulnerable to specific man in the 01640 * middle attacks. (See RFC 5746) 01641 * (Most interoperable and least secure option) 01642 * 01643 * SSL_LEGACY_BREAK_HANDSHAKE breaks off connections 01644 * if peer does not support secure renegotiation. Results 01645 * in interoperability issues with non-upgraded peers 01646 * that do not support renegotiation altogether. 01647 * (Most secure option, interoperability issues) 01648 * 01649 * \param ssl SSL context 01650 * \param allow_legacy Prevent or allow (SSL_NO_LEGACY_RENEGOTIATION, 01651 * SSL_ALLOW_LEGACY_RENEGOTIATION or 01652 * SSL_LEGACY_BREAK_HANDSHAKE) 01653 */ 01654 void ssl_legacy_renegotiation( ssl_context *ssl, int allow_legacy ); 01655 01656 #if defined(POLARSSL_SSL_RENEGOTIATION) 01657 /** 01658 * \brief Enforce requested renegotiation. 01659 * (Default: enforced, max_records = 16) 01660 * 01661 * When we request a renegotiation, the peer can comply or 01662 * ignore the request. This function allows us to decide 01663 * whether to enforce our renegotiation requests by closing 01664 * the connection if the peer doesn't comply. 01665 * 01666 * However, records could already be in transit from the peer 01667 * when the request is emitted. In order to increase 01668 * reliability, we can accept a number of records before the 01669 * expected handshake records. 01670 * 01671 * The optimal value is highly dependent on the specific usage 01672 * scenario. 01673 * 01674 * \warning On client, the grace period can only happen during 01675 * ssl_read(), as opposed to ssl_write() and ssl_renegotiate() 01676 * which always behave as if max_record was 0. The reason is, 01677 * if we receive application data from the server, we need a 01678 * place to write it, which only happens during ssl_read(). 01679 * 01680 * \param ssl SSL context 01681 * \param max_records Use SSL_RENEGOTIATION_NOT_ENFORCED if you don't want to 01682 * enforce renegotiation, or a non-negative value to enforce 01683 * it but allow for a grace period of max_records records. 01684 */ 01685 void ssl_set_renegotiation_enforced( ssl_context *ssl, int max_records ); 01686 01687 /** 01688 * \brief Set record counter threshold for periodic renegotiation. 01689 * (Default: 2^64 - 256.) 01690 * 01691 * Renegotiation is automatically triggered when a record 01692 * counter (outgoing or ingoing) crosses the defined 01693 * threshold. The default value is meant to prevent the 01694 * connection from being closed when the counter is about to 01695 * reached its maximal value (it is not allowed to wrap). 01696 * 01697 * Lower values can be used to enforce policies such as "keys 01698 * must be refreshed every N packets with cipher X". 01699 * 01700 * \param ssl SSL context 01701 * \param period The threshold value: a big-endian 64-bit number. 01702 * Set to 2^64 - 1 to disable periodic renegotiation 01703 */ 01704 void ssl_set_renegotiation_period( ssl_context *ssl, 01705 const unsigned char period[8] ); 01706 #endif /* POLARSSL_SSL_RENEGOTIATION */ 01707 01708 /** 01709 * \brief Return the number of data bytes available to read 01710 * 01711 * \param ssl SSL context 01712 * 01713 * \return how many bytes are available in the read buffer 01714 */ 01715 size_t ssl_get_bytes_avail( const ssl_context *ssl ); 01716 01717 /** 01718 * \brief Return the result of the certificate verification 01719 * 01720 * \param ssl SSL context 01721 * 01722 * \return 0 if successful, 01723 * -1 if result is not available (eg because the handshake was 01724 * aborted too early), or 01725 * a combination of BADCERT_xxx and BADCRL_xxx flags, see 01726 * x509.h 01727 */ 01728 int ssl_get_verify_result( const ssl_context *ssl ); 01729 01730 /** 01731 * \brief Return the name of the current ciphersuite 01732 * 01733 * \param ssl SSL context 01734 * 01735 * \return a string containing the ciphersuite name 01736 */ 01737 const char *ssl_get_ciphersuite( const ssl_context *ssl ); 01738 01739 /** 01740 * \brief Return the current SSL version (SSLv3/TLSv1/etc) 01741 * 01742 * \param ssl SSL context 01743 * 01744 * \return a string containing the SSL version 01745 */ 01746 const char *ssl_get_version( const ssl_context *ssl ); 01747 01748 #if defined(POLARSSL_X509_CRT_PARSE_C) 01749 /** 01750 * \brief Return the peer certificate from the current connection 01751 * 01752 * Note: Can be NULL in case no certificate was sent during 01753 * the handshake. Different calls for the same connection can 01754 * return the same or different pointers for the same 01755 * certificate and even a different certificate altogether. 01756 * The peer cert CAN change in a single connection if 01757 * renegotiation is performed. 01758 * 01759 * \param ssl SSL context 01760 * 01761 * \return the current peer certificate 01762 */ 01763 const x509_crt *ssl_get_peer_cert( const ssl_context *ssl ); 01764 #endif /* POLARSSL_X509_CRT_PARSE_C */ 01765 01766 #if defined(POLARSSL_SSL_CLI_C) 01767 /** 01768 * \brief Save session in order to resume it later (client-side only) 01769 * Session data is copied to presented session structure. 01770 * 01771 * \warning Currently, peer certificate is lost in the operation. 01772 * 01773 * \param ssl SSL context 01774 * \param session session context 01775 * 01776 * \return 0 if successful, 01777 * POLARSSL_ERR_SSL_MALLOC_FAILED if memory allocation failed, 01778 * POLARSSL_ERR_SSL_BAD_INPUT_DATA if used server-side or 01779 * arguments are otherwise invalid 01780 * 01781 * \sa ssl_set_session() 01782 */ 01783 int ssl_get_session( const ssl_context *ssl, ssl_session *session ); 01784 #endif /* POLARSSL_SSL_CLI_C */ 01785 01786 /** 01787 * \brief Perform the SSL handshake 01788 * 01789 * \param ssl SSL context 01790 * 01791 * \return 0 if successful, POLARSSL_ERR_NET_WANT_READ, 01792 * POLARSSL_ERR_NET_WANT_WRITE, or a specific SSL error code. 01793 */ 01794 int ssl_handshake( ssl_context *ssl ); 01795 01796 /** 01797 * \brief Perform a single step of the SSL handshake 01798 * 01799 * Note: the state of the context (ssl->state) will be at 01800 * the following state after execution of this function. 01801 * Do not call this function if state is SSL_HANDSHAKE_OVER. 01802 * 01803 * \param ssl SSL context 01804 * 01805 * \return 0 if successful, POLARSSL_ERR_NET_WANT_READ, 01806 * POLARSSL_ERR_NET_WANT_WRITE, or a specific SSL error code. 01807 */ 01808 int ssl_handshake_step( ssl_context *ssl ); 01809 01810 #if defined(POLARSSL_SSL_RENEGOTIATION) 01811 /** 01812 * \brief Initiate an SSL renegotiation on the running connection. 01813 * Client: perform the renegotiation right now. 01814 * Server: request renegotiation, which will be performed 01815 * during the next call to ssl_read() if honored by client. 01816 * 01817 * \param ssl SSL context 01818 * 01819 * \return 0 if successful, or any ssl_handshake() return value. 01820 */ 01821 int ssl_renegotiate( ssl_context *ssl ); 01822 #endif /* POLARSSL_SSL_RENEGOTIATION */ 01823 01824 /** 01825 * \brief Read at most 'len' application data bytes 01826 * 01827 * \param ssl SSL context 01828 * \param buf buffer that will hold the data 01829 * \param len maximum number of bytes to read 01830 * 01831 * \return This function returns the number of bytes read, 0 for EOF, 01832 * or a negative error code. 01833 */ 01834 int ssl_read( ssl_context *ssl, unsigned char *buf, size_t len ); 01835 01836 /** 01837 * \brief Write exactly 'len' application data bytes 01838 * 01839 * \param ssl SSL context 01840 * \param buf buffer holding the data 01841 * \param len how many bytes must be written 01842 * 01843 * \return This function returns the number of bytes written, 01844 * or a negative error code. 01845 * 01846 * \note When this function returns POLARSSL_ERR_NET_WANT_WRITE, 01847 * it must be called later with the *same* arguments, 01848 * until it returns a positive value. 01849 * 01850 * \note This function may write less than the number of bytes 01851 * requested if len is greater than the maximum record length. 01852 * For arbitrary-sized messages, it should be called in a loop. 01853 */ 01854 int ssl_write( ssl_context *ssl, const unsigned char *buf, size_t len ); 01855 01856 /** 01857 * \brief Send an alert message 01858 * 01859 * \param ssl SSL context 01860 * \param level The alert level of the message 01861 * (SSL_ALERT_LEVEL_WARNING or SSL_ALERT_LEVEL_FATAL) 01862 * \param message The alert message (SSL_ALERT_MSG_*) 01863 * 01864 * \return 0 if successful, or a specific SSL error code. 01865 */ 01866 int ssl_send_alert_message( ssl_context *ssl, 01867 unsigned char level, 01868 unsigned char message ); 01869 /** 01870 * \brief Notify the peer that the connection is being closed 01871 * 01872 * \param ssl SSL context 01873 */ 01874 int ssl_close_notify( ssl_context *ssl ); 01875 01876 /** 01877 * \brief Free referenced items in an SSL context and clear memory 01878 * 01879 * \param ssl SSL context 01880 */ 01881 void ssl_free( ssl_context *ssl ); 01882 01883 /** 01884 * \brief Initialize SSL session structure 01885 * 01886 * \param session SSL session 01887 */ 01888 void ssl_session_init( ssl_session *session ); 01889 01890 /** 01891 * \brief Free referenced items in an SSL session including the 01892 * peer certificate and clear memory 01893 * 01894 * \param session SSL session 01895 */ 01896 void ssl_session_free( ssl_session *session ); 01897 01898 /** 01899 * \brief Free referenced items in an SSL transform context and clear 01900 * memory 01901 * 01902 * \param transform SSL transform context 01903 */ 01904 void ssl_transform_free( ssl_transform *transform ); 01905 01906 /** 01907 * \brief Free referenced items in an SSL handshake context and clear 01908 * memory 01909 * 01910 * \param handshake SSL handshake context 01911 */ 01912 void ssl_handshake_free( ssl_handshake_params *handshake ); 01913 01914 /* 01915 * Internal functions (do not call directly) 01916 */ 01917 int ssl_handshake_client_step( ssl_context *ssl ); 01918 int ssl_handshake_server_step( ssl_context *ssl ); 01919 void ssl_handshake_wrapup( ssl_context *ssl ); 01920 01921 int ssl_send_fatal_handshake_failure( ssl_context *ssl ); 01922 01923 int ssl_derive_keys( ssl_context *ssl ); 01924 01925 int ssl_read_record( ssl_context *ssl ); 01926 /** 01927 * \return 0 if successful, POLARSSL_ERR_SSL_CONN_EOF on EOF or 01928 * another negative error code. 01929 */ 01930 int ssl_fetch_input ( ssl_context *ssl, size_t nb_want ); 01931 01932 int ssl_write_record( ssl_context *ssl ); 01933 int ssl_flush_output( ssl_context *ssl ); 01934 01935 int ssl_parse_certificate( ssl_context *ssl ); 01936 int ssl_write_certificate( ssl_context *ssl ); 01937 01938 int ssl_parse_change_cipher_spec( ssl_context *ssl ); 01939 int ssl_write_change_cipher_spec( ssl_context *ssl ); 01940 01941 int ssl_parse_finished( ssl_context *ssl ); 01942 int ssl_write_finished( ssl_context *ssl ); 01943 01944 void ssl_optimize_checksum( ssl_context *ssl, 01945 const ssl_ciphersuite_t *ciphersuite_info ); 01946 01947 #if defined(POLARSSL_KEY_EXCHANGE__SOME__PSK_ENABLED) 01948 int ssl_psk_derive_premaster( ssl_context *ssl, key_exchange_type_t key_ex ); 01949 #endif 01950 01951 #if defined(POLARSSL_PK_C) 01952 unsigned char ssl_sig_from_pk( pk_context *pk ); 01953 pk_type_t ssl_pk_alg_from_sig( unsigned char sig ); 01954 #endif 01955 01956 md_type_t ssl_md_alg_from_hash( unsigned char hash ); 01957 01958 #if defined(POLARSSL_SSL_SET_CURVES) 01959 int ssl_curve_is_acceptable( const ssl_context *ssl, ecp_group_id grp_id ); 01960 #endif 01961 01962 #if defined(POLARSSL_X509_CRT_PARSE_C) 01963 static inline pk_context *ssl_own_key( ssl_context *ssl ) 01964 { 01965 return( ssl->handshake->key_cert == NULL ? NULL 01966 : ssl->handshake->key_cert->key ); 01967 } 01968 01969 static inline x509_crt *ssl_own_cert( ssl_context *ssl ) 01970 { 01971 return( ssl->handshake->key_cert == NULL ? NULL 01972 : ssl->handshake->key_cert->cert ); 01973 } 01974 01975 /* 01976 * Check usage of a certificate wrt extensions: 01977 * keyUsage, extendedKeyUsage (later), and nSCertType (later). 01978 * 01979 * Warning: cert_endpoint is the endpoint of the cert (ie, of our peer when we 01980 * check a cert we received from them)! 01981 * 01982 * Return 0 if everything is OK, -1 if not. 01983 */ 01984 int ssl_check_cert_usage( const x509_crt *cert, 01985 const ssl_ciphersuite_t *ciphersuite, 01986 int cert_endpoint, 01987 int *flags ); 01988 #endif /* POLARSSL_X509_CRT_PARSE_C */ 01989 01990 /* constant-time buffer comparison */ 01991 static inline int safer_memcmp( const void *a, const void *b, size_t n ) 01992 { 01993 size_t i; 01994 const unsigned char *A = (const unsigned char *) a; 01995 const unsigned char *B = (const unsigned char *) b; 01996 unsigned char diff = 0; 01997 01998 for( i = 0; i < n; i++ ) 01999 diff |= A[i] ^ B[i]; 02000 02001 return( diff ); 02002 } 02003 02004 #ifdef __cplusplus 02005 } 02006 #endif 02007 02008 #endif /* ssl.h */ 02009
Generated on Tue Jul 12 2022 13:50:38 by
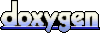