mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
ecdh.h File Reference
Elliptic curve Diffie-Hellman. More...
Go to the source code of this file.
Data Structures | |
struct | ecdh_context |
ECDH context structure. More... | |
Enumerations | |
enum | ecdh_side |
When importing from an EC key, select if it is our key or the peer's key. More... | |
Functions | |
int | ecdh_gen_public (ecp_group *grp, mpi *d, ecp_point *Q, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng) |
Generate a public key. | |
int | ecdh_compute_shared (ecp_group *grp, mpi *z, const ecp_point *Q, const mpi *d, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng) |
Compute shared secret Raw function that only does the core computation. | |
void | ecdh_init (ecdh_context *ctx) |
Initialize context. | |
void | ecdh_free (ecdh_context *ctx) |
Free context. | |
int | ecdh_make_params (ecdh_context *ctx, size_t *olen, unsigned char *buf, size_t blen, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng) |
Generate a public key and a TLS ServerKeyExchange payload. | |
int | ecdh_read_params (ecdh_context *ctx, const unsigned char **buf, const unsigned char *end) |
Parse and procress a TLS ServerKeyExhange payload. | |
int | ecdh_get_params (ecdh_context *ctx, const ecp_keypair *key, ecdh_side side) |
Setup an ECDH context from an EC key. | |
int | ecdh_make_public (ecdh_context *ctx, size_t *olen, unsigned char *buf, size_t blen, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng) |
Generate a public key and a TLS ClientKeyExchange payload. | |
int | ecdh_read_public (ecdh_context *ctx, const unsigned char *buf, size_t blen) |
Parse and process a TLS ClientKeyExchange payload. | |
int | ecdh_calc_secret (ecdh_context *ctx, size_t *olen, unsigned char *buf, size_t blen, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng) |
Derive and export the shared secret. | |
int | ecdh_self_test (int verbose) |
Checkup routine. |
Detailed Description
Elliptic curve Diffie-Hellman.
Copyright (C) 2006-2013, ARM Limited, All Rights Reserved
This file is part of mbed TLS (https://tls.mbed.org)
This program is free software; you can redistribute it and/or modify it under the terms of the GNU General Public License as published by the Free Software Foundation; either version 2 of the License, or (at your option) any later version.
This program is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for more details.
You should have received a copy of the GNU General Public License along with this program; if not, write to the Free Software Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA.
Definition in file ecdh.h.
Enumeration Type Documentation
enum ecdh_side |
Function Documentation
int ecdh_calc_secret | ( | ecdh_context * | ctx, |
size_t * | olen, | ||
unsigned char * | buf, | ||
size_t | blen, | ||
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng | ||
) |
Derive and export the shared secret.
(Last function used by both TLS client en servers.)
- Parameters:
-
ctx ECDH context olen number of bytes written buf destination buffer blen buffer length f_rng RNG function, see notes for ecdh_compute_shared()
p_rng RNG parameter
- Returns:
- 0 if successful, or an POLARSSL_ERR_ECP_XXX error code
int ecdh_compute_shared | ( | ecp_group * | grp, |
mpi * | z, | ||
const ecp_point * | Q, | ||
const mpi * | d, | ||
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng | ||
) |
Compute shared secret Raw function that only does the core computation.
- Parameters:
-
grp ECP group z Destination MPI (shared secret) Q Public key from other party d Our secret exponent (private key) f_rng RNG function (see notes) p_rng RNG parameter
- Returns:
- 0 if successful, or a POLARSSL_ERR_ECP_XXX or POLARSSL_MPI_XXX error code
- Note:
- If f_rng is not NULL, it is used to implement countermeasures against potential elaborate timing attacks, see
ecp_mul()
for details.
void ecdh_free | ( | ecdh_context * | ctx ) |
int ecdh_gen_public | ( | ecp_group * | grp, |
mpi * | d, | ||
ecp_point * | Q, | ||
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng | ||
) |
Generate a public key.
Raw function that only does the core computation.
- Parameters:
-
grp ECP group d Destination MPI (secret exponent, aka private key) Q Destination point (public key) f_rng RNG function p_rng RNG parameter
- Returns:
- 0 if successful, or a POLARSSL_ERR_ECP_XXX or POLARSSL_MPI_XXX error code
int ecdh_get_params | ( | ecdh_context * | ctx, |
const ecp_keypair * | key, | ||
ecdh_side | side | ||
) |
Setup an ECDH context from an EC key.
(Used by clients and servers in place of the ServerKeyEchange for static ECDH: import ECDH parameters from a certificate's EC key information.)
- Parameters:
-
ctx ECDH constext to set key EC key to use side Is it our key (1) or the peer's key (0) ?
- Returns:
- 0 if successful, or an POLARSSL_ERR_ECP_XXX error code
void ecdh_init | ( | ecdh_context * | ctx ) |
int ecdh_make_params | ( | ecdh_context * | ctx, |
size_t * | olen, | ||
unsigned char * | buf, | ||
size_t | blen, | ||
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng | ||
) |
Generate a public key and a TLS ServerKeyExchange payload.
(First function used by a TLS server for ECDHE.)
- Parameters:
-
ctx ECDH context olen number of chars written buf destination buffer blen length of buffer f_rng RNG function p_rng RNG parameter
- Note:
- This function assumes that ctx->grp has already been properly set (for example using ecp_use_known_dp).
- Returns:
- 0 if successful, or an POLARSSL_ERR_ECP_XXX error code
int ecdh_make_public | ( | ecdh_context * | ctx, |
size_t * | olen, | ||
unsigned char * | buf, | ||
size_t | blen, | ||
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng | ||
) |
Generate a public key and a TLS ClientKeyExchange payload.
(Second function used by a TLS client for ECDH(E).)
- Parameters:
-
ctx ECDH context olen number of bytes actually written buf destination buffer blen size of destination buffer f_rng RNG function p_rng RNG parameter
- Returns:
- 0 if successful, or an POLARSSL_ERR_ECP_XXX error code
int ecdh_read_params | ( | ecdh_context * | ctx, |
const unsigned char ** | buf, | ||
const unsigned char * | end | ||
) |
int ecdh_read_public | ( | ecdh_context * | ctx, |
const unsigned char * | buf, | ||
size_t | blen | ||
) |
Generated on Tue Jul 12 2022 13:50:39 by
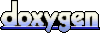