mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
net.h File Reference
Network communication functions. More...
Go to the source code of this file.
Functions | |
int | net_connect (int *fd, const char *host, int port) |
Initiate a TCP connection with host:port. | |
int | net_bind (int *fd, const char *bind_ip, int port) |
Create a listening socket on bind_ip:port. | |
int | net_accept (int bind_fd, int *client_fd, void *client_ip) |
Accept a connection from a remote client. | |
int | net_set_block (int fd) |
Set the socket blocking. | |
int | net_set_nonblock (int fd) |
Set the socket non-blocking. | |
void | net_usleep (unsigned long usec) |
Portable usleep helper. | |
int | net_recv (void *ctx, unsigned char *buf, size_t len) |
Read at most 'len' characters. | |
int | net_send (void *ctx, const unsigned char *buf, size_t len) |
Write at most 'len' characters. | |
void | net_close (int fd) |
Gracefully shutdown the connection. |
Detailed Description
Network communication functions.
Copyright (C) 2006-2011, ARM Limited, All Rights Reserved
This file is part of mbed TLS (https://tls.mbed.org)
This program is free software; you can redistribute it and/or modify it under the terms of the GNU General Public License as published by the Free Software Foundation; either version 2 of the License, or (at your option) any later version.
This program is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for more details.
You should have received a copy of the GNU General Public License along with this program; if not, write to the Free Software Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA.
Definition in file net.h.
Function Documentation
int net_accept | ( | int | bind_fd, |
int * | client_fd, | ||
void * | client_ip | ||
) |
Accept a connection from a remote client.
- Parameters:
-
bind_fd Relevant socket client_fd Will contain the connected client socket client_ip Will contain the client IP address Must be at least 4 bytes, or 16 if IPv6 is supported
- Returns:
- 0 if successful, POLARSSL_ERR_NET_ACCEPT_FAILED, or POLARSSL_ERR_NET_WANT_READ is bind_fd was set to non-blocking and accept() is blocking.
int net_bind | ( | int * | fd, |
const char * | bind_ip, | ||
int | port | ||
) |
Create a listening socket on bind_ip:port.
If bind_ip == NULL, all interfaces are binded.
- Parameters:
-
fd Socket to use bind_ip IP to bind to, can be NULL port Port number to use
- Returns:
- 0 if successful, or one of: POLARSSL_ERR_NET_SOCKET_FAILED, POLARSSL_ERR_NET_BIND_FAILED, POLARSSL_ERR_NET_LISTEN_FAILED
void net_close | ( | int | fd ) |
int net_connect | ( | int * | fd, |
const char * | host, | ||
int | port | ||
) |
int net_recv | ( | void * | ctx, |
unsigned char * | buf, | ||
size_t | len | ||
) |
Read at most 'len' characters.
If no error occurs, the actual amount read is returned.
- Parameters:
-
ctx Socket buf The buffer to write to len Maximum length of the buffer
- Returns:
- This function returns the number of bytes received, or a non-zero error code; POLARSSL_ERR_NET_WANT_READ indicates read() is blocking.
int net_send | ( | void * | ctx, |
const unsigned char * | buf, | ||
size_t | len | ||
) |
Write at most 'len' characters.
If no error occurs, the actual amount read is returned.
- Parameters:
-
ctx Socket buf The buffer to read from len The length of the buffer
- Returns:
- This function returns the number of bytes sent, or a non-zero error code; POLARSSL_ERR_NET_WANT_WRITE indicates write() is blocking.
int net_set_block | ( | int | fd ) |
int net_set_nonblock | ( | int | fd ) |
Generated on Tue Jul 12 2022 13:50:40 by
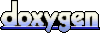