mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
net.h
00001 /** 00002 * \file net.h 00003 * 00004 * \brief Network communication functions 00005 * 00006 * Copyright (C) 2006-2011, ARM Limited, All Rights Reserved 00007 * 00008 * This file is part of mbed TLS (https://tls.mbed.org) 00009 * 00010 * This program is free software; you can redistribute it and/or modify 00011 * it under the terms of the GNU General Public License as published by 00012 * the Free Software Foundation; either version 2 of the License, or 00013 * (at your option) any later version. 00014 * 00015 * This program is distributed in the hope that it will be useful, 00016 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00017 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00018 * GNU General Public License for more details. 00019 * 00020 * You should have received a copy of the GNU General Public License along 00021 * with this program; if not, write to the Free Software Foundation, Inc., 00022 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00023 */ 00024 #ifndef POLARSSL_NET_H 00025 #define POLARSSL_NET_H 00026 00027 #include <stddef.h> 00028 00029 #define POLARSSL_ERR_NET_UNKNOWN_HOST -0x0056 /**< Failed to get an IP address for the given hostname. */ 00030 #define POLARSSL_ERR_NET_SOCKET_FAILED -0x0042 /**< Failed to open a socket. */ 00031 #define POLARSSL_ERR_NET_CONNECT_FAILED -0x0044 /**< The connection to the given server / port failed. */ 00032 #define POLARSSL_ERR_NET_BIND_FAILED -0x0046 /**< Binding of the socket failed. */ 00033 #define POLARSSL_ERR_NET_LISTEN_FAILED -0x0048 /**< Could not listen on the socket. */ 00034 #define POLARSSL_ERR_NET_ACCEPT_FAILED -0x004A /**< Could not accept the incoming connection. */ 00035 #define POLARSSL_ERR_NET_RECV_FAILED -0x004C /**< Reading information from the socket failed. */ 00036 #define POLARSSL_ERR_NET_SEND_FAILED -0x004E /**< Sending information through the socket failed. */ 00037 #define POLARSSL_ERR_NET_CONN_RESET -0x0050 /**< Connection was reset by peer. */ 00038 #define POLARSSL_ERR_NET_WANT_READ -0x0052 /**< Connection requires a read call. */ 00039 #define POLARSSL_ERR_NET_WANT_WRITE -0x0054 /**< Connection requires a write call. */ 00040 00041 #define POLARSSL_NET_LISTEN_BACKLOG 10 /**< The backlog that listen() should use. */ 00042 00043 #ifdef __cplusplus 00044 extern "C" { 00045 #endif 00046 00047 /** 00048 * \brief Initiate a TCP connection with host:port 00049 * 00050 * \param fd Socket to use 00051 * \param host Host to connect to 00052 * \param port Port to connect to 00053 * 00054 * \return 0 if successful, or one of: 00055 * POLARSSL_ERR_NET_SOCKET_FAILED, 00056 * POLARSSL_ERR_NET_UNKNOWN_HOST, 00057 * POLARSSL_ERR_NET_CONNECT_FAILED 00058 */ 00059 int net_connect( int *fd, const char *host, int port ); 00060 00061 /** 00062 * \brief Create a listening socket on bind_ip:port. 00063 * If bind_ip == NULL, all interfaces are binded. 00064 * 00065 * \param fd Socket to use 00066 * \param bind_ip IP to bind to, can be NULL 00067 * \param port Port number to use 00068 * 00069 * \return 0 if successful, or one of: 00070 * POLARSSL_ERR_NET_SOCKET_FAILED, 00071 * POLARSSL_ERR_NET_BIND_FAILED, 00072 * POLARSSL_ERR_NET_LISTEN_FAILED 00073 */ 00074 int net_bind( int *fd, const char *bind_ip, int port ); 00075 00076 /** 00077 * \brief Accept a connection from a remote client 00078 * 00079 * \param bind_fd Relevant socket 00080 * \param client_fd Will contain the connected client socket 00081 * \param client_ip Will contain the client IP address 00082 * Must be at least 4 bytes, or 16 if IPv6 is supported 00083 * 00084 * \return 0 if successful, POLARSSL_ERR_NET_ACCEPT_FAILED, or 00085 * POLARSSL_ERR_NET_WANT_READ is bind_fd was set to 00086 * non-blocking and accept() is blocking. 00087 */ 00088 int net_accept( int bind_fd, int *client_fd, void *client_ip ); 00089 00090 /** 00091 * \brief Set the socket blocking 00092 * 00093 * \param fd Socket to set 00094 * 00095 * \return 0 if successful, or a non-zero error code 00096 */ 00097 int net_set_block( int fd ); 00098 00099 /** 00100 * \brief Set the socket non-blocking 00101 * 00102 * \param fd Socket to set 00103 * 00104 * \return 0 if successful, or a non-zero error code 00105 */ 00106 int net_set_nonblock( int fd ); 00107 00108 /** 00109 * \brief Portable usleep helper 00110 * 00111 * \param usec Amount of microseconds to sleep 00112 * 00113 * \note Real amount of time slept will not be less than 00114 * select()'s timeout granularity (typically, 10ms). 00115 */ 00116 void net_usleep( unsigned long usec ); 00117 00118 /** 00119 * \brief Read at most 'len' characters. If no error occurs, 00120 * the actual amount read is returned. 00121 * 00122 * \param ctx Socket 00123 * \param buf The buffer to write to 00124 * \param len Maximum length of the buffer 00125 * 00126 * \return This function returns the number of bytes received, 00127 * or a non-zero error code; POLARSSL_ERR_NET_WANT_READ 00128 * indicates read() is blocking. 00129 */ 00130 int net_recv( void *ctx, unsigned char *buf, size_t len ); 00131 00132 /** 00133 * \brief Write at most 'len' characters. If no error occurs, 00134 * the actual amount read is returned. 00135 * 00136 * \param ctx Socket 00137 * \param buf The buffer to read from 00138 * \param len The length of the buffer 00139 * 00140 * \return This function returns the number of bytes sent, 00141 * or a non-zero error code; POLARSSL_ERR_NET_WANT_WRITE 00142 * indicates write() is blocking. 00143 */ 00144 int net_send( void *ctx, const unsigned char *buf, size_t len ); 00145 00146 /** 00147 * \brief Gracefully shutdown the connection 00148 * 00149 * \param fd The socket to close 00150 */ 00151 void net_close( int fd ); 00152 00153 #ifdef __cplusplus 00154 } 00155 #endif 00156 00157 #endif /* net.h */ 00158
Generated on Tue Jul 12 2022 13:50:37 by
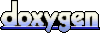