mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
net.c
00001 /* 00002 * TCP networking functions 00003 * 00004 * Copyright (C) 2006-2014, ARM Limited, All Rights Reserved 00005 * 00006 * This file is part of mbed TLS (https://tls.mbed.org) 00007 * 00008 * This program is free software; you can redistribute it and/or modify 00009 * it under the terms of the GNU General Public License as published by 00010 * the Free Software Foundation; either version 2 of the License, or 00011 * (at your option) any later version. 00012 * 00013 * This program is distributed in the hope that it will be useful, 00014 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00015 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00016 * GNU General Public License for more details. 00017 * 00018 * You should have received a copy of the GNU General Public License along 00019 * with this program; if not, write to the Free Software Foundation, Inc., 00020 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00021 */ 00022 00023 #if !defined(POLARSSL_CONFIG_FILE) 00024 #include "polarssl/config.h" 00025 #else 00026 #include POLARSSL_CONFIG_FILE 00027 #endif 00028 00029 #if defined(POLARSSL_NET_C) 00030 00031 #include "polarssl/net.h" 00032 00033 #include <string.h> 00034 00035 #if (defined(_WIN32) || defined(_WIN32_WCE)) && !defined(EFIX64) && \ 00036 !defined(EFI32) 00037 00038 #if defined(POLARSSL_HAVE_IPV6) 00039 #ifdef _WIN32_WINNT 00040 #undef _WIN32_WINNT 00041 #endif 00042 /* Enables getaddrinfo() & Co */ 00043 #define _WIN32_WINNT 0x0501 00044 #include <ws2tcpip.h> 00045 #endif 00046 00047 #include <winsock2.h> 00048 #include <windows.h> 00049 00050 #if defined(_MSC_VER) 00051 #if defined(_WIN32_WCE) 00052 #pragma comment( lib, "ws2.lib" ) 00053 #else 00054 #pragma comment( lib, "ws2_32.lib" ) 00055 #endif 00056 #endif /* _MSC_VER */ 00057 00058 #define read(fd,buf,len) recv(fd,(char*)buf,(int) len,0) 00059 #define write(fd,buf,len) send(fd,(char*)buf,(int) len,0) 00060 #define close(fd) closesocket(fd) 00061 00062 static int wsa_init_done = 0; 00063 00064 #else /* ( _WIN32 || _WIN32_WCE ) && !EFIX64 && !EFI32 */ 00065 00066 #include <sys/types.h> 00067 #include <sys/socket.h> 00068 #include <netinet/in.h> 00069 #include <arpa/inet.h> 00070 #if defined(POLARSSL_HAVE_TIME) 00071 #include <sys/time.h> 00072 #endif 00073 #include <unistd.h> 00074 #include <signal.h> 00075 #include <fcntl.h> 00076 #include <netdb.h> 00077 #include <errno.h> 00078 00079 #if defined(__FreeBSD__) || defined(__OpenBSD__) || defined(__NetBSD__) || \ 00080 defined(__DragonFly__) 00081 #include <sys/endian.h> 00082 #elif defined(__APPLE__) || defined(HAVE_MACHINE_ENDIAN_H) || \ 00083 defined(EFIX64) || defined(EFI32) 00084 #include <machine/endian.h> 00085 #elif defined(sun) 00086 #include <sys/isa_defs.h> 00087 #elif defined(_AIX) || defined(HAVE_ARPA_NAMESER_COMPAT_H) 00088 #include <arpa/nameser_compat.h> 00089 #else 00090 #include <endian.h> 00091 #endif 00092 00093 #endif /* ( _WIN32 || _WIN32_WCE ) && !EFIX64 && !EFI32 */ 00094 00095 #include <stdlib.h> 00096 #include <stdio.h> 00097 00098 #if defined(_MSC_VER) && !defined snprintf && !defined(EFIX64) && \ 00099 !defined(EFI32) 00100 #define snprintf _snprintf 00101 #endif 00102 00103 #if defined(POLARSSL_HAVE_TIME) 00104 #include <time.h> 00105 #endif 00106 00107 #if defined(_MSC_VER) && !defined(EFIX64) && !defined(EFI32) 00108 #include <basetsd.h> 00109 typedef UINT32 uint32_t; 00110 #else 00111 #include <inttypes.h> 00112 #endif 00113 00114 /* 00115 * htons() is not always available. 00116 * By default go for LITTLE_ENDIAN variant. Otherwise hope for _BYTE_ORDER and 00117 * __BIG_ENDIAN to help determine endianness. 00118 */ 00119 #if defined(__BYTE_ORDER) && defined(__BIG_ENDIAN) && \ 00120 __BYTE_ORDER == __BIG_ENDIAN 00121 #define POLARSSL_HTONS(n) (n) 00122 #define POLARSSL_HTONL(n) (n) 00123 #else 00124 #define POLARSSL_HTONS(n) ((((unsigned short)(n) & 0xFF ) << 8 ) | \ 00125 (((unsigned short)(n) & 0xFF00 ) >> 8 )) 00126 #define POLARSSL_HTONL(n) ((((unsigned long )(n) & 0xFF ) << 24) | \ 00127 (((unsigned long )(n) & 0xFF00 ) << 8 ) | \ 00128 (((unsigned long )(n) & 0xFF0000 ) >> 8 ) | \ 00129 (((unsigned long )(n) & 0xFF000000) >> 24)) 00130 #endif 00131 00132 #if defined(POLARSSL_PLATFORM_C) 00133 #include "polarssl/platform.h" 00134 #else 00135 #define polarssl_snprintf snprintf 00136 #endif 00137 00138 unsigned short net_htons( unsigned short n ); 00139 unsigned long net_htonl( unsigned long n ); 00140 #define net_htons(n) POLARSSL_HTONS(n) 00141 #define net_htonl(n) POLARSSL_HTONL(n) 00142 00143 /* 00144 * Prepare for using the sockets interface 00145 */ 00146 static int net_prepare( void ) 00147 { 00148 #if ( defined(_WIN32) || defined(_WIN32_WCE) ) && !defined(EFIX64) && \ 00149 !defined(EFI32) 00150 WSADATA wsaData; 00151 00152 if( wsa_init_done == 0 ) 00153 { 00154 if( WSAStartup( MAKEWORD(2,0), &wsaData ) != 0 ) 00155 return( POLARSSL_ERR_NET_SOCKET_FAILED ); 00156 00157 wsa_init_done = 1; 00158 } 00159 #else 00160 #if !defined(EFIX64) && !defined(EFI32) 00161 signal( SIGPIPE, SIG_IGN ); 00162 #endif 00163 #endif 00164 return( 0 ); 00165 } 00166 00167 /* 00168 * Initiate a TCP connection with host:port 00169 */ 00170 int net_connect( int *fd, const char *host, int port ) 00171 { 00172 #if defined(POLARSSL_HAVE_IPV6) 00173 int ret; 00174 struct addrinfo hints, *addr_list, *cur; 00175 char port_str[6]; 00176 00177 if( ( ret = net_prepare() ) != 0 ) 00178 return( ret ); 00179 00180 /* getaddrinfo expects port as a string */ 00181 memset( port_str, 0, sizeof( port_str ) ); 00182 polarssl_snprintf( port_str, sizeof( port_str ), "%d", port ); 00183 00184 /* Do name resolution with both IPv6 and IPv4, but only TCP */ 00185 memset( &hints, 0, sizeof( hints ) ); 00186 hints.ai_family = AF_UNSPEC; 00187 hints.ai_socktype = SOCK_STREAM; 00188 hints.ai_protocol = IPPROTO_TCP; 00189 00190 if( getaddrinfo( host, port_str, &hints, &addr_list ) != 0 ) 00191 return( POLARSSL_ERR_NET_UNKNOWN_HOST ); 00192 00193 /* Try the sockaddrs until a connection succeeds */ 00194 ret = POLARSSL_ERR_NET_UNKNOWN_HOST; 00195 for( cur = addr_list; cur != NULL; cur = cur->ai_next ) 00196 { 00197 *fd = (int) socket( cur->ai_family, cur->ai_socktype, 00198 cur->ai_protocol ); 00199 if( *fd < 0 ) 00200 { 00201 ret = POLARSSL_ERR_NET_SOCKET_FAILED; 00202 continue; 00203 } 00204 00205 if( connect( *fd, cur->ai_addr, cur->ai_addrlen ) == 0 ) 00206 { 00207 ret = 0; 00208 break; 00209 } 00210 00211 close( *fd ); 00212 ret = POLARSSL_ERR_NET_CONNECT_FAILED; 00213 } 00214 00215 freeaddrinfo( addr_list ); 00216 00217 return( ret ); 00218 00219 #else 00220 /* Legacy IPv4-only version */ 00221 00222 int ret; 00223 struct sockaddr_in server_addr; 00224 struct hostent *server_host; 00225 00226 if( ( ret = net_prepare() ) != 0 ) 00227 return( ret ); 00228 00229 if( ( server_host = gethostbyname( host ) ) == NULL ) 00230 return( POLARSSL_ERR_NET_UNKNOWN_HOST ); 00231 00232 if( ( *fd = (int) socket( AF_INET, SOCK_STREAM, IPPROTO_IP ) ) < 0 ) 00233 return( POLARSSL_ERR_NET_SOCKET_FAILED ); 00234 00235 memcpy( (void *) &server_addr.sin_addr, 00236 (void *) server_host->h_addr, 00237 server_host->h_length ); 00238 00239 server_addr.sin_family = AF_INET; 00240 server_addr.sin_port = net_htons( port ); 00241 00242 if( connect( *fd, (struct sockaddr *) &server_addr, 00243 sizeof( server_addr ) ) < 0 ) 00244 { 00245 close( *fd ); 00246 return( POLARSSL_ERR_NET_CONNECT_FAILED ); 00247 } 00248 00249 return( 0 ); 00250 #endif /* POLARSSL_HAVE_IPV6 */ 00251 } 00252 00253 /* 00254 * Create a listening socket on bind_ip:port 00255 */ 00256 int net_bind( int *fd, const char *bind_ip, int port ) 00257 { 00258 #if defined(POLARSSL_HAVE_IPV6) 00259 int n, ret; 00260 struct addrinfo hints, *addr_list, *cur; 00261 char port_str[6]; 00262 00263 if( ( ret = net_prepare() ) != 0 ) 00264 return( ret ); 00265 00266 /* getaddrinfo expects port as a string */ 00267 memset( port_str, 0, sizeof( port_str ) ); 00268 polarssl_snprintf( port_str, sizeof( port_str ), "%d", port ); 00269 00270 /* Bind to IPv6 and/or IPv4, but only in TCP */ 00271 memset( &hints, 0, sizeof( hints ) ); 00272 hints.ai_family = AF_UNSPEC; 00273 hints.ai_socktype = SOCK_STREAM; 00274 hints.ai_protocol = IPPROTO_TCP; 00275 if( bind_ip == NULL ) 00276 hints.ai_flags = AI_PASSIVE; 00277 00278 if( getaddrinfo( bind_ip, port_str, &hints, &addr_list ) != 0 ) 00279 return( POLARSSL_ERR_NET_UNKNOWN_HOST ); 00280 00281 /* Try the sockaddrs until a binding succeeds */ 00282 ret = POLARSSL_ERR_NET_UNKNOWN_HOST; 00283 for( cur = addr_list; cur != NULL; cur = cur->ai_next ) 00284 { 00285 *fd = (int) socket( cur->ai_family, cur->ai_socktype, 00286 cur->ai_protocol ); 00287 if( *fd < 0 ) 00288 { 00289 ret = POLARSSL_ERR_NET_SOCKET_FAILED; 00290 continue; 00291 } 00292 00293 n = 1; 00294 if( setsockopt( *fd, SOL_SOCKET, SO_REUSEADDR, 00295 (const char *) &n, sizeof( n ) ) != 0 ) 00296 { 00297 close( *fd ); 00298 ret = POLARSSL_ERR_NET_SOCKET_FAILED; 00299 continue; 00300 } 00301 00302 if( bind( *fd, cur->ai_addr, cur->ai_addrlen ) != 0 ) 00303 { 00304 close( *fd ); 00305 ret = POLARSSL_ERR_NET_BIND_FAILED; 00306 continue; 00307 } 00308 00309 if( listen( *fd, POLARSSL_NET_LISTEN_BACKLOG ) != 0 ) 00310 { 00311 close( *fd ); 00312 ret = POLARSSL_ERR_NET_LISTEN_FAILED; 00313 continue; 00314 } 00315 00316 /* I we ever get there, it's a success */ 00317 ret = 0; 00318 break; 00319 } 00320 00321 freeaddrinfo( addr_list ); 00322 00323 return( ret ); 00324 00325 #else 00326 /* Legacy IPv4-only version */ 00327 00328 int ret, n, c[4]; 00329 struct sockaddr_in server_addr; 00330 00331 if( ( ret = net_prepare() ) != 0 ) 00332 return( ret ); 00333 00334 if( ( *fd = (int) socket( AF_INET, SOCK_STREAM, IPPROTO_IP ) ) < 0 ) 00335 return( POLARSSL_ERR_NET_SOCKET_FAILED ); 00336 00337 n = 1; 00338 setsockopt( *fd, SOL_SOCKET, SO_REUSEADDR, 00339 (const char *) &n, sizeof( n ) ); 00340 00341 server_addr.sin_addr.s_addr = net_htonl( INADDR_ANY ); 00342 server_addr.sin_family = AF_INET; 00343 server_addr.sin_port = net_htons( port ); 00344 00345 if( bind_ip != NULL ) 00346 { 00347 memset( c, 0, sizeof( c ) ); 00348 sscanf( bind_ip, "%d.%d.%d.%d", &c[0], &c[1], &c[2], &c[3] ); 00349 00350 for( n = 0; n < 4; n++ ) 00351 if( c[n] < 0 || c[n] > 255 ) 00352 break; 00353 00354 if( n == 4 ) 00355 server_addr.sin_addr.s_addr = net_htonl( 00356 ( (uint32_t) c[0] << 24 ) | 00357 ( (uint32_t) c[1] << 16 ) | 00358 ( (uint32_t) c[2] << 8 ) | 00359 ( (uint32_t) c[3] ) ); 00360 } 00361 00362 if( bind( *fd, (struct sockaddr *) &server_addr, 00363 sizeof( server_addr ) ) < 0 ) 00364 { 00365 close( *fd ); 00366 return( POLARSSL_ERR_NET_BIND_FAILED ); 00367 } 00368 00369 if( listen( *fd, POLARSSL_NET_LISTEN_BACKLOG ) != 0 ) 00370 { 00371 close( *fd ); 00372 return( POLARSSL_ERR_NET_LISTEN_FAILED ); 00373 } 00374 00375 return( 0 ); 00376 #endif /* POLARSSL_HAVE_IPV6 */ 00377 } 00378 00379 #if ( defined(_WIN32) || defined(_WIN32_WCE) ) && !defined(EFIX64) && \ 00380 !defined(EFI32) 00381 /* 00382 * Check if the requested operation would be blocking on a non-blocking socket 00383 * and thus 'failed' with a negative return value. 00384 */ 00385 static int net_would_block( int fd ) 00386 { 00387 ((void) fd); 00388 return( WSAGetLastError() == WSAEWOULDBLOCK ); 00389 } 00390 #else 00391 /* 00392 * Check if the requested operation would be blocking on a non-blocking socket 00393 * and thus 'failed' with a negative return value. 00394 * 00395 * Note: on a blocking socket this function always returns 0! 00396 */ 00397 static int net_would_block( int fd ) 00398 { 00399 /* 00400 * Never return 'WOULD BLOCK' on a non-blocking socket 00401 */ 00402 if( ( fcntl( fd, F_GETFL ) & O_NONBLOCK ) != O_NONBLOCK ) 00403 return( 0 ); 00404 00405 switch( errno ) 00406 { 00407 #if defined EAGAIN 00408 case EAGAIN: 00409 #endif 00410 #if defined EWOULDBLOCK && EWOULDBLOCK != EAGAIN 00411 case EWOULDBLOCK: 00412 #endif 00413 return( 1 ); 00414 } 00415 return( 0 ); 00416 } 00417 #endif /* ( _WIN32 || _WIN32_WCE ) && !EFIX64 && !EFI32 */ 00418 00419 /* 00420 * Accept a connection from a remote client 00421 */ 00422 int net_accept( int bind_fd, int *client_fd, void *client_ip ) 00423 { 00424 #if defined(POLARSSL_HAVE_IPV6) 00425 struct sockaddr_storage client_addr; 00426 #else 00427 struct sockaddr_in client_addr; 00428 #endif 00429 00430 #if defined(__socklen_t_defined) || defined(_SOCKLEN_T) || \ 00431 defined(_SOCKLEN_T_DECLARED) 00432 socklen_t n = (socklen_t) sizeof( client_addr ); 00433 #else 00434 int n = (int) sizeof( client_addr ); 00435 #endif 00436 00437 *client_fd = (int) accept( bind_fd, (struct sockaddr *) 00438 &client_addr, &n ); 00439 00440 if( *client_fd < 0 ) 00441 { 00442 if( net_would_block( bind_fd ) != 0 ) 00443 return( POLARSSL_ERR_NET_WANT_READ ); 00444 00445 return( POLARSSL_ERR_NET_ACCEPT_FAILED ); 00446 } 00447 00448 if( client_ip != NULL ) 00449 { 00450 #if defined(POLARSSL_HAVE_IPV6) 00451 if( client_addr.ss_family == AF_INET ) 00452 { 00453 struct sockaddr_in *addr4 = (struct sockaddr_in *) &client_addr; 00454 memcpy( client_ip, &addr4->sin_addr.s_addr, 00455 sizeof( addr4->sin_addr.s_addr ) ); 00456 } 00457 else 00458 { 00459 struct sockaddr_in6 *addr6 = (struct sockaddr_in6 *) &client_addr; 00460 memcpy( client_ip, &addr6->sin6_addr.s6_addr, 00461 sizeof( addr6->sin6_addr.s6_addr ) ); 00462 } 00463 #else 00464 memcpy( client_ip, &client_addr.sin_addr.s_addr, 00465 sizeof( client_addr.sin_addr.s_addr ) ); 00466 #endif /* POLARSSL_HAVE_IPV6 */ 00467 } 00468 00469 return( 0 ); 00470 } 00471 00472 /* 00473 * Set the socket blocking or non-blocking 00474 */ 00475 int net_set_block( int fd ) 00476 { 00477 #if ( defined(_WIN32) || defined(_WIN32_WCE) ) && !defined(EFIX64) && \ 00478 !defined(EFI32) 00479 u_long n = 0; 00480 return( ioctlsocket( fd, FIONBIO, &n ) ); 00481 #else 00482 return( fcntl( fd, F_SETFL, fcntl( fd, F_GETFL ) & ~O_NONBLOCK ) ); 00483 #endif 00484 } 00485 00486 int net_set_nonblock( int fd ) 00487 { 00488 #if ( defined(_WIN32) || defined(_WIN32_WCE) ) && !defined(EFIX64) && \ 00489 !defined(EFI32) 00490 u_long n = 1; 00491 return( ioctlsocket( fd, FIONBIO, &n ) ); 00492 #else 00493 return( fcntl( fd, F_SETFL, fcntl( fd, F_GETFL ) | O_NONBLOCK ) ); 00494 #endif 00495 } 00496 00497 #if defined(POLARSSL_HAVE_TIME) 00498 /* 00499 * Portable usleep helper 00500 */ 00501 void net_usleep( unsigned long usec ) 00502 { 00503 struct timeval tv; 00504 tv.tv_sec = usec / 1000000; 00505 #if !defined(_WIN32) && ( defined(__unix__) || defined(__unix) || \ 00506 ( defined(__APPLE__) && defined(__MACH__) ) ) 00507 tv.tv_usec = (suseconds_t) usec % 1000000; 00508 #else 00509 tv.tv_usec = usec % 1000000; 00510 #endif 00511 select( 0, NULL, NULL, NULL, &tv ); 00512 } 00513 #endif /* POLARSSL_HAVE_TIME */ 00514 00515 /* 00516 * Read at most 'len' characters 00517 */ 00518 int net_recv( void *ctx, unsigned char *buf, size_t len ) 00519 { 00520 int fd = *((int *) ctx); 00521 int ret = (int) read( fd, buf, len ); 00522 00523 if( ret < 0 ) 00524 { 00525 if( net_would_block( fd ) != 0 ) 00526 return( POLARSSL_ERR_NET_WANT_READ ); 00527 00528 #if ( defined(_WIN32) || defined(_WIN32_WCE) ) && !defined(EFIX64) && \ 00529 !defined(EFI32) 00530 if( WSAGetLastError() == WSAECONNRESET ) 00531 return( POLARSSL_ERR_NET_CONN_RESET ); 00532 #else 00533 if( errno == EPIPE || errno == ECONNRESET ) 00534 return( POLARSSL_ERR_NET_CONN_RESET ); 00535 00536 if( errno == EINTR ) 00537 return( POLARSSL_ERR_NET_WANT_READ ); 00538 #endif 00539 00540 return( POLARSSL_ERR_NET_RECV_FAILED ); 00541 } 00542 00543 return( ret ); 00544 } 00545 00546 /* 00547 * Write at most 'len' characters 00548 */ 00549 int net_send( void *ctx, const unsigned char *buf, size_t len ) 00550 { 00551 int fd = *((int *) ctx); 00552 int ret = (int) write( fd, buf, len ); 00553 00554 if( ret < 0 ) 00555 { 00556 if( net_would_block( fd ) != 0 ) 00557 return( POLARSSL_ERR_NET_WANT_WRITE ); 00558 00559 #if ( defined(_WIN32) || defined(_WIN32_WCE) ) && !defined(EFIX64) && \ 00560 !defined(EFI32) 00561 if( WSAGetLastError() == WSAECONNRESET ) 00562 return( POLARSSL_ERR_NET_CONN_RESET ); 00563 #else 00564 if( errno == EPIPE || errno == ECONNRESET ) 00565 return( POLARSSL_ERR_NET_CONN_RESET ); 00566 00567 if( errno == EINTR ) 00568 return( POLARSSL_ERR_NET_WANT_WRITE ); 00569 #endif 00570 00571 return( POLARSSL_ERR_NET_SEND_FAILED ); 00572 } 00573 00574 return( ret ); 00575 } 00576 00577 /* 00578 * Gracefully close the connection 00579 */ 00580 void net_close( int fd ) 00581 { 00582 shutdown( fd, 2 ); 00583 close( fd ); 00584 } 00585 00586 #endif /* POLARSSL_NET_C */ 00587
Generated on Tue Jul 12 2022 13:50:37 by
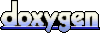