DMA library for the KL25Z
Dependents: SimpleDMA_HelloWorld RTOS_SPI spiDMAtest Pinscape_Controller_v1 ... more
SimpleDMA.h
00001 #ifndef SIMPLEDMA_H 00002 #define SIMPLEDMA_H 00003 00004 #ifdef RTOS_H 00005 #include "rtos.h" 00006 #endif 00007 00008 #include "mbed.h" 00009 #include "SimpleDMA_KL25.h" 00010 #include "SimpleDMA_KL46.h" 00011 #include "SimpleDMA_LPC1768.h" 00012 00013 00014 /** 00015 * SimpleDMA, DMA made simple! (Okay that was bad) 00016 * 00017 * A class to easily make basic DMA operations happen. Not all features 00018 * of the DMA peripherals are used, but the main ones are: From and to memory 00019 * and peripherals, either continiously or triggered 00020 */ 00021 class SimpleDMA { 00022 public: 00023 /** 00024 * Constructor 00025 * 00026 * @param channel - optional parameter which channel should be used, default is automatic channel selection 00027 */ 00028 SimpleDMA(int channel = -1); 00029 00030 /** 00031 * Set the source of the DMA transfer 00032 * 00033 * Autoincrement increments the pointer after each transfer. If the source 00034 * is an array this should be true, if it is a peripheral or a single memory 00035 * location it should be false. 00036 * 00037 * The source can be any pointer to any memory location. Automatically 00038 * the wordsize is calculated depending on the type, if required you can 00039 * also override this. 00040 * 00041 * @param pointer - pointer to the memory location 00042 * @param autoinc - should the pointer be incremented by the DMA module 00043 * @param size - wordsize in bits (optional, generally can be omitted) 00044 * @return - 0 on success 00045 */ 00046 template<typename Type> 00047 void source(Type* pointer, bool autoinc, int size = sizeof(Type) * 8) { 00048 _source = (uint32_t)pointer; 00049 source_inc = autoinc; 00050 source_size = size; 00051 } 00052 00053 /** 00054 * Set the destination of the DMA transfer 00055 * 00056 * Autoincrement increments the pointer after each transfer. If the source 00057 * is an array this should be true, if it is a peripheral or a single memory 00058 * location it should be false. 00059 * 00060 * The destination can be any pointer to any memory location. Automatically 00061 * the wordsize is calculated depending on the type, if required you can 00062 * also override this. 00063 * 00064 * @param pointer - pointer to the memory location 00065 * @param autoinc - should the pointer be incremented by the DMA module 00066 * @param size - wordsize in bits (optional, generally can be omitted) 00067 * @return - 0 on success 00068 */ 00069 template<typename Type> 00070 void destination(Type* pointer, bool autoinc, int size = sizeof(Type) * 8) { 00071 _destination = (uint32_t)pointer; 00072 destination_inc = autoinc; 00073 destination_size = size; 00074 } 00075 00076 /** 00077 * Set the trigger for the DMA operation 00078 * 00079 * In SimpleDMA_[yourdevice].h you can find the names of the different triggers. 00080 * Trigger_ALWAYS is defined for all devices, it will simply move the data 00081 * as fast as possible. Used for memory-memory transfers. If nothing else is set 00082 * that will be used by default. 00083 * 00084 * @param trig - trigger to use 00085 * @param return - 0 on success 00086 */ 00087 void trigger(SimpleDMA_Trigger trig) { 00088 _trigger = trig; 00089 } 00090 00091 /** 00092 * Set the DMA channel 00093 * 00094 * Generally you will not need to call this function, the constructor does so for you 00095 * 00096 * @param chan - DMA channel to use, -1 = variable channel (highest priority channel which is available) 00097 */ 00098 void channel(int chan); 00099 00100 /** 00101 * Start the transfer 00102 * 00103 * @param length - number of BYTES to be moved by the DMA 00104 */ 00105 int start(uint32_t length); 00106 00107 /** 00108 * Is the DMA channel busy 00109 * 00110 * @param channel - channel to check, -1 = current channel 00111 * @return - true if it is busy 00112 */ 00113 bool isBusy( int channel = -1 ); 00114 00115 /** 00116 * Attach an interrupt upon completion of DMA transfer or error 00117 * 00118 * @param function - function to call upon completion (may be a member function) 00119 */ 00120 void attach(Callback<void(int)> func) { 00121 _callback = func; 00122 } 00123 00124 00125 protected: 00126 int _channel; 00127 SimpleDMA_Trigger _trigger; 00128 uint32_t _source; 00129 uint32_t _destination; 00130 bool source_inc; 00131 bool destination_inc; 00132 uint8_t source_size; 00133 uint8_t destination_size; 00134 00135 bool auto_channel; 00136 00137 //IRQ handlers 00138 Callback<void(int)> _callback; 00139 void irq_handler(void); 00140 00141 static SimpleDMA *irq_owner[DMA_CHANNELS]; 00142 00143 static void irq_handler0( void ); 00144 00145 #if DMA_IRQS > 1 00146 static void irq_handler1( void ); 00147 static void irq_handler2( void ); 00148 static void irq_handler3( void ); 00149 #endif 00150 00151 //Keep searching until we find a non-busy channel, start with lowest channel number 00152 int getFreeChannel(void); 00153 00154 00155 }; 00156 #endif
Generated on Tue Jul 19 2022 07:00:06 by
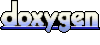