Added support for WNC M14A2A Cellular LTE Data Module.
Dependencies: WNC14A2AInterface
Dependents: http-example-wnc http-example-wnc-modified
Functions | |
void | SpiritAesMode (SpiritFunctionalState xNewState) |
Enables or Disables the AES engine. | |
void | SpiritAesWriteDataIn (uint8_t *pcBufferDataIn, uint8_t cDataLength) |
Writes the data to encrypt or decrypt, or the encryption key for the derive decryption key operation into the AES_DATA_IN registers. | |
void | SpiritAesReadDataOut (uint8_t *pcBufferDataOut, uint8_t cDataLength) |
Returns the encrypted or decrypted data or the decription key from the AES_DATA_OUT register. | |
void | SpiritAesWriteKey (uint8_t *pcKey) |
Writes the encryption key into the AES_KEY_IN register. | |
void | SpiritAesReadKey (uint8_t *pcKey) |
Returns the encryption/decryption key from the AES_KEY_IN register. | |
void | SpiritAesDeriveDecKeyFromEnc (void) |
Derives the decryption key from a given encryption key. | |
void | SpiritAesExecuteEncryption (void) |
Executes the encryption operation. | |
void | SpiritAesExecuteDecryption (void) |
Executes the decryption operation. | |
void | SpiritAesDeriveDecKeyExecuteDec (void) |
Executes the key derivation and the decryption operation. |
Function Documentation
void SpiritAesDeriveDecKeyExecuteDec | ( | void | ) |
Executes the key derivation and the decryption operation.
- Parameters:
-
None.
- Return values:
-
None.
Definition at line 295 of file SPIRIT_Aes.c.
void SpiritAesDeriveDecKeyFromEnc | ( | void | ) |
Derives the decryption key from a given encryption key.
- Parameters:
-
None.
- Return values:
-
None.
Definition at line 256 of file SPIRIT_Aes.c.
void SpiritAesExecuteDecryption | ( | void | ) |
Executes the decryption operation.
- Parameters:
-
None.
- Return values:
-
None.
Definition at line 282 of file SPIRIT_Aes.c.
void SpiritAesExecuteEncryption | ( | void | ) |
Executes the encryption operation.
- Parameters:
-
None.
- Return values:
-
None.
Definition at line 269 of file SPIRIT_Aes.c.
void SpiritAesMode | ( | SpiritFunctionalState | xNewState ) |
Enables or Disables the AES engine.
- Parameters:
-
xNewState new state for AES engine. This parameter can be: S_ENABLE or S_DISABLE.
- Return values:
-
None
Definition at line 118 of file SPIRIT_Aes.c.
void SpiritAesReadDataOut | ( | uint8_t * | pcBufferDataOut, |
uint8_t | cDataLength | ||
) |
Returns the encrypted or decrypted data or the decription key from the AES_DATA_OUT register.
- Parameters:
-
pcBufferDataOut pointer to the user data buffer. The AES_DATA_OUT[0] register value will be put as first element of the buffer (MSB), while the AES_DAT_OUT[cDataLength-1] register value will be put as last element of the buffer (LSB). This parameter is a uint8_t*. cDataLength length of data to read in bytes. This parameter is a uint8_t.
- Return values:
-
None
Definition at line 184 of file SPIRIT_Aes.c.
void SpiritAesReadKey | ( | uint8_t * | pcKey ) |
Returns the encryption/decryption key from the AES_KEY_IN register.
- Parameters:
-
pcKey pointer to the buffer of 4 words (16 bytes) containing the AES key. The first byte of the buffer shall be the most significant byte AES_KEY_0 of the AES key. The last byte of the buffer shall be the less significant byte AES_KEY_15 of the AES key. This parameter is an uint8_t*.
- Return values:
-
None
Definition at line 236 of file SPIRIT_Aes.c.
void SpiritAesWriteDataIn | ( | uint8_t * | pcBufferDataIn, |
uint8_t | cDataLength | ||
) |
Writes the data to encrypt or decrypt, or the encryption key for the derive decryption key operation into the AES_DATA_IN registers.
- Parameters:
-
pcBufferDataIn pointer to the user data buffer. The first byte of the array shall be the MSB byte and it will be put in the AES_DATA_IN[0] register, while the last one shall be the LSB and it will be put in the AES_DATA_IN[cDataLength-1] register. If data to write are less than 16 bytes the remaining AES_DATA_IN registers will be filled with bytes equal to 0. This parameter is an uint8_t*. cDataLength length of data in bytes. This parameter is an uint8_t.
- Return values:
-
None
Definition at line 154 of file SPIRIT_Aes.c.
void SpiritAesWriteKey | ( | uint8_t * | pcKey ) |
Writes the encryption key into the AES_KEY_IN register.
- Parameters:
-
pcKey pointer to the buffer of 4 words containing the AES key. The first byte of the buffer shall be the most significant byte AES_KEY_0 of the AES key. The last byte of the buffer shall be the less significant byte AES_KEY_15 of the AES key. This parameter is an uint8_t*.
- Return values:
-
None
Definition at line 215 of file SPIRIT_Aes.c.
Generated on Tue Jul 12 2022 17:40:26 by
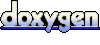