A Ricker is a simple Ticker but using the RIT rather than Timer3
Ricker Class Reference
#include <Ricker.h>
Public Member Functions | |
Ricker () | |
Constructor. | |
~Ricker () | |
Destructor. | |
void | detach (void) |
detach | |
void | attach_ms (void(*fptr)(void), uint32_t u) |
attach_ms | |
template<typename T > | |
void | attach_ms (T *tptr, void(T::*mptr)(void), uint32_t u) |
attach_ms | |
void | attach (void(*fptr)(void), double d) |
attach | |
template<typename T > | |
void | attach (T *tptr, void(T::*mptr)(void), double d) |
attach | |
Protected Member Functions | |
void | tick (void) |
tick |
Detailed Description
A class used to create multiple Rickers. These attach themselves to the RickerSys main controller.
Note, unlike an Mbed Ticker, a Ricker can only make callbacks down to 1ms.
#include "mbed.h" #include "Ricker.h" DigitalOut led1(LED1); Ricker r1; void cb1(void) { led1 = !led1; } int main() { r1.attach(&cb1, 0.25); while(1) { } }
Definition at line 82 of file Ricker.h.
Constructor & Destructor Documentation
Member Function Documentation
void attach | ( | void(*)(void) | fptr, |
double | d | ||
) |
void attach | ( | T * | tptr, |
void(T::*)(void) | mptr, | ||
double | d | ||
) |
void attach_ms | ( | T * | tptr, |
void(T::*)(void) | mptr, | ||
uint32_t | u | ||
) |
void attach_ms | ( | void(*)(void) | fptr, |
uint32_t | u | ||
) |
void detach | ( | void | ) |
Generated on Wed Jul 13 2022 20:31:44 by
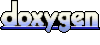