A Ricker is a simple Ticker but using the RIT rather than Timer3
Embed:
(wiki syntax)
Show/hide line numbers
Ricker.h
00001 /* 00002 Copyright (c) 2011 Andy Kirkham 00003 00004 Permission is hereby granted, free of charge, to any person obtaining a copy 00005 of this software and associated documentation files (the "Software"), to deal 00006 in the Software without restriction, including without limitation the rights 00007 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 copies of the Software, and to permit persons to whom the Software is 00009 furnished to do so, subject to the following conditions: 00010 00011 The above copyright notice and this permission notice shall be included in 00012 all copies or substantial portions of the Software. 00013 00014 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 THE SOFTWARE. 00021 */ 00022 00023 #ifndef AJK_RICKER_H 00024 #define AJK_RICKER_H 00025 00026 #include "mbed.h" 00027 #include <list> 00028 00029 namespace AjK { 00030 00031 class Ricker; // forward ref. 00032 00033 // Ricker controller. 00034 /** RickerSys 00035 * 00036 * Base system, only a single instance of this is allowed. It's used to manage 00037 * the RIT IRQ and the chain of "Rickers" attached. 00038 * 00039 * Note, unlike an Mbed Ticker the Ricker uses a 1ms resolution for it's timer. 00040 * Therefore teh smallest unit of time that a Ricker can measure is 1ms. If 00041 * you need sub-millisecond then use an Mbed Ticker. 00042 */ 00043 class RickerSys { 00044 protected: 00045 list<Ricker *> rickers; 00046 public: 00047 void init(void); 00048 RickerSys(); 00049 void isr(void); 00050 void addTicker(Ricker *t); 00051 void delTicker(Ricker *t); 00052 }; 00053 00054 extern RickerSys _rickerSys; 00055 00056 // Ricker instance object. 00057 /** Ricker 00058 * 00059 * A class used to create multiple Rickers. These attach themselves to the RickerSys 00060 * main controller. 00061 * 00062 * <b>Note</b>, unlike an Mbed Ticker, a Ricker can only make callbacks down to 1ms. 00063 * 00064 * @code 00065 * #include "mbed.h" 00066 * #include "Ricker.h" 00067 * 00068 * DigitalOut led1(LED1); 00069 * 00070 * Ricker r1; 00071 * 00072 * void cb1(void) { led1 = !led1; } 00073 * 00074 * int main() { 00075 * 00076 * r1.attach(&cb1, 0.25); 00077 * 00078 * while(1) { } 00079 * } 00080 * @endcode 00081 */ 00082 class Ricker { 00083 protected: 00084 FunctionPointer callback; 00085 uint32_t counter; 00086 uint32_t reload; 00087 00088 /** tick 00089 * @internal 00090 * Called by the RickerSys controller every 1ms. 00091 */ 00092 void tick(void) { 00093 if (counter) { 00094 counter--; 00095 if (counter == 0) { 00096 counter = reload; 00097 callback.call(); 00098 } 00099 } 00100 } 00101 00102 public: 00103 friend class RickerSys; 00104 00105 /** Constructor 00106 */ 00107 Ricker() { 00108 counter = 0; 00109 _rickerSys.addTicker(this); 00110 } 00111 00112 /** Destructor 00113 */ 00114 ~Ricker() { 00115 _rickerSys.delTicker(this); 00116 } 00117 00118 /** detach 00119 * 00120 * Remove the callback from the Ricker. 00121 */ 00122 void detach(void) { 00123 callback.attach(); 00124 } 00125 00126 /** attach_ms 00127 * 00128 * Attach a C style function pointer callback. 00129 * 00130 * @param fptr A C style function pointer. 00131 * @param uint32_t u The number of milliseconds to call at. 00132 */ 00133 void attach_ms(void (*fptr)(void), uint32_t u) { 00134 counter = reload = u; 00135 callback.attach(fptr); 00136 } 00137 00138 /** attach_ms 00139 * 00140 * Attach a C++ style functor object/method callback. 00141 * 00142 * @param tptr A C++ style object pointer. 00143 * @param mptr A C++ style method pointer. 00144 * @param uint32_t u The number of milliseconds to call at. 00145 */ 00146 template<typename T> 00147 void attach_ms(T* tptr, void (T::*mptr)(void), uint32_t u) { 00148 if((mptr != NULL) && (tptr != NULL)) { 00149 counter = reload = u; 00150 callback.attach(tptr, mptr); 00151 } 00152 } 00153 00154 /** attach 00155 * 00156 * Attach a C style function pointer callback. 00157 * 00158 * @param fptr A C style function pointer. 00159 * @param double d The number of seconds to call at. 00160 */ 00161 void attach(void (*fptr)(void), double d) { 00162 counter = reload = (uint32_t)( d * 1000.0 ); 00163 callback.attach(fptr); 00164 } 00165 00166 /** attach 00167 * 00168 * Attach a C++ style functor object/method callback. 00169 * 00170 * @param tptr A C++ style object pointer. 00171 * @param mptr A C++ style method pointer. 00172 * @param double d The number of seconds to call at. 00173 */ 00174 template<typename T> 00175 void attach(T* tptr, void (T::*mptr)(void), double d) { 00176 if((mptr != NULL) && (tptr != NULL)) { 00177 counter = reload = (uint32_t)( d * 1000.0 ); 00178 callback.attach(tptr, mptr); 00179 } 00180 } 00181 00182 }; 00183 00184 }; // namespace AjK ends. 00185 00186 using namespace AjK; 00187 00188 #endif
Generated on Wed Jul 13 2022 20:31:44 by
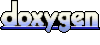