Bug fix release
Dependents: AntiTheftGPS XbeeReceive XbeeSend Superball_Ball2 ... more
MODSERIAL Class Reference
#include <MODSERIAL.h>
Public Types | |
enum | Parity |
A copy of the Serial parity enum. More... | |
enum | IrqType |
A copy of the Serial IrqType enum. More... | |
enum | Result { Ok = 0, NoMemory = -1, NoChar = -1, BufferOversize = -2 } |
Non-blocking functions return code. More... | |
Public Member Functions | |
MODSERIAL (PinName tx, PinName rx, const char *name=NULL) | |
The MODSERIAL constructor is used to initialise the serial object. | |
MODSERIAL (PinName tx, PinName rx, int bufferSize, const char *name=NULL) | |
The MODSERIAL constructor is used to initialise the serial object. | |
MODSERIAL (PinName tx, PinName rx, int txBufferSize, int rxBufferSize, const char *name=NULL) | |
The MODSERIAL constructor is used to initialise the serial object. | |
void | attach (void(*fptr)(MODSERIAL_IRQ_INFO *), IrqType type=RxIrq) |
Function: attach. | |
template<typename T > | |
void | attach (T *tptr, void(T::*mptr)(MODSERIAL_IRQ_INFO *), IrqType type=RxIrq) |
Function: attach. | |
void | connect (void(*fptr)(MODSERIAL_IRQ_INFO *), IrqType type=RxIrq) |
template<typename T > | |
void | connect (T *tptr, void(T::*mptr)(MODSERIAL_IRQ_INFO *), IrqType type=RxIrq) |
int | writeable () |
Function: writeable. | |
int | readable () |
Function: readable. | |
bool | txBufferSane (void) |
Function: txBufferSane. | |
bool | rxBufferSane (void) |
Function: rxBufferSane. | |
int | txBufferGetCount (void) |
Function: txBufferGetCount. | |
int | rxBufferGetCount (void) |
Function: rxBufferGetCount. | |
int | txBufferGetSize (int size) |
Function: txBufferGetSize. | |
int | rxBufferGetSize (int size) |
Function: rxBufferGetSize. | |
bool | txBufferFull (void) |
Function: txBufferFull. | |
bool | rxBufferFull (void) |
Function: rxBufferFull. | |
bool | txBufferEmpty (void) |
Function: txBufferEmpty. | |
bool | rxBufferEmpty (void) |
Function: rxBufferEmpty. | |
int | txBufferSetSize (int size, bool m) |
Function: txBufferSetSize. | |
int | rxBufferSetSize (int size, bool m) |
Function: rxBufferSetSize. | |
int | txBufferSetSize (int size) |
Function: txBufferSetSize. | |
int | rxBufferSetSize (int size) |
Function: rxBufferSetSize. | |
void | txBufferFlush (void) |
Function: txBufferFlush. | |
void | rxBufferFlush (void) |
Function: rxBufferFlush. | |
int | getcNb () |
Function: getcNb. | |
int | getc () |
Function: getc. | |
char | txGetLastChar (void) |
Function: txGetLastChar. | |
char | rxGetLastChar (void) |
Function: rxGetLastChar. | |
bool | txIsBusy (void) |
Function: txIsBusy. | |
void | autoDetectChar (char c) |
Function: autoDetectChar. | |
int | move (char *s, int max, char end) |
Function: move. | |
int | move (char *s, int max) |
Function: move (overloaded) | |
int | putc (int c) |
Function: putc. | |
int | printf (const char *format,...) |
Function: printf. | |
int | scanf (const char *format,...) |
Function: scanf. | |
void | MODDMA (MODDMA *p) |
Set the "void pointer" moddma_p to be a pointer to a MODDMA controller class instance. | |
int | dmaSend (char *buffer, int len, int dmaChannel=7) |
Send a char buffer to the Uarts TX system using DMA. | |
void | attach_dmaSendComplete (void(*fptr)(MODSERIAL_IRQ_INFO *)) |
Attach a callback to the DMA completion. | |
template<typename T > | |
void | attach_dmaSendComplete (T *tptr, void(T::*mptr)(MODSERIAL_IRQ_INFO *)) |
Attach a callback to the DMA completion. | |
Protected Member Functions | |
int | rxDiscardLastChar (void) |
Remove the last char placed into the rx buffer. | |
void | dmaSendCallback (void) |
Callback for dmaSend(). | |
Protected Attributes | |
MODSERIAL_IRQ_INFO | callbackInfo |
Used to pass information to callbacks. |
Detailed Description
- See also:
- http://mbed.org/cookbook/MODSERIAL
- http://mbed.org/handbook/Serial
- example1.cpp
- example2.cpp
- example3a.cpp
- example3b.cpp
- example_dma.cpp
- The MODSERIAL API
MODSERIAL extends the Mbed library Serial to provide fully buffered TX and RX streams. Buffer length is fully customisable.
Before using MODSERIAL users should be familar with Mbed's standard Serial library object. MODSERIAL is a direct "drop in" replacement for Serial. Where previously Serial was used, MODSERIAL can be used as adirect replacement instantly offering standard TX and RX buffering. By default, both TX and RX buffers are 256 bytes in length.
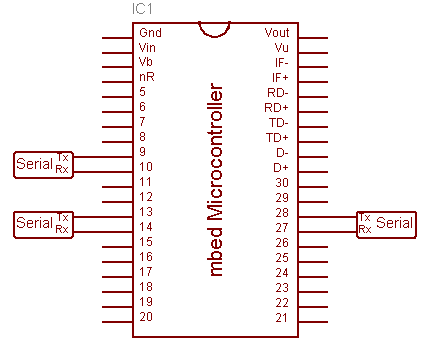
Standard example:
#include "mbed.h" #include "MODSERIAL.h" MODSERIAL pc(USBTX, USBRX); // tx, rx int main() { pc.printf("Hello World!"); while(1) { pc.putc(pc.getc() + 1); } }
Example with alternate buffer length:
#include "mbed.h" #include "MODSERIAL.h" // Make TX and RX buffers 512byes in length MODSERIAL pc(USBTX, USBRX, 512); // tx, rx int main() { pc.printf("Hello World!"); while(1) { pc.putc(pc.getc() + 1); } }
Example with alternate buffer length:
#include "mbed.h" #include "MODSERIAL.h" // Make TX 1024bytes and RX 512byes in length MODSERIAL pc(USBTX, USBRX, 1024, 512); // tx, rx int main() { pc.printf("Hello World!"); while(1) { pc.putc(pc.getc() + 1); } }
Definition at line 245 of file MODSERIAL.h.
Member Enumeration Documentation
enum IrqType |
A copy of the Serial IrqType enum.
Definition at line 263 of file MODSERIAL.h.
enum Parity |
A copy of the Serial parity enum.
Definition at line 254 of file MODSERIAL.h.
enum Result |
Non-blocking functions return code.
- Enumerator:
Ok Ok.
NoMemory Memory allocation failed.
NoChar No character in buffer.
BufferOversize Oversized buffer.
Definition at line 274 of file MODSERIAL.h.
Constructor & Destructor Documentation
MODSERIAL | ( | PinName | tx, |
PinName | rx, | ||
const char * | name = NULL |
||
) |
The MODSERIAL constructor is used to initialise the serial object.
- Parameters:
-
tx PinName of the TX pin. rx PinName of the TX pin.
Definition at line 34 of file MODSERIAL.cpp.
MODSERIAL | ( | PinName | tx, |
PinName | rx, | ||
int | bufferSize, | ||
const char * | name = NULL |
||
) |
The MODSERIAL constructor is used to initialise the serial object.
- Parameters:
-
tx PinName of the TX pin. rx PinName of the TX pin. bufferSize Integer of the TX and RX buffer sizes.
Definition at line 39 of file MODSERIAL.cpp.
MODSERIAL | ( | PinName | tx, |
PinName | rx, | ||
int | txBufferSize, | ||
int | rxBufferSize, | ||
const char * | name = NULL |
||
) |
The MODSERIAL constructor is used to initialise the serial object.
- Parameters:
-
tx PinName of the TX pin. rx PinName of the TX pin. txBufferSize Integer of the TX buffer sizes. rxBufferSize Integer of the RX buffer sizes.
Definition at line 44 of file MODSERIAL.cpp.
Member Function Documentation
int dmaSend | ( | char * | buffer, |
int | len, | ||
int | dmaChannel = 7 |
||
) |
Send a char buffer to the Uarts TX system using DMA.
This blocks regular library sending.
- Parameters:
-
buffer A char buffer of bytes to send. len The length of the buffer to send. dmaChannel The DMA channel to use, defaults to 7
- Returns:
- MODDMA::Status MODDMA::ok if all went ok
Definition at line 998 of file MODSERIAL.h.
void dmaSendCallback | ( | void | ) | [protected] |
Callback for dmaSend().
Definition at line 1066 of file MODSERIAL.h.
Generated on Tue Jul 12 2022 12:20:34 by
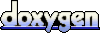