MODDMA GPDMA Controller New features: transfer pins to memory buffer under periodic timer control and send double buffers to DAC
Dependents: FirstTest WaveSim IO-dma-memmem DACDMAfuncgenlib ... more
MODDMA Class Reference
MODDMA GPDMA Controller. More...
#include <MODDMA.h>
Public Types | |
enum | CHANNELS { Channel_0 = 0, Channel_1, Channel_2, Channel_3, Channel_4, Channel_5, Channel_6, Channel_7 } |
Channel definitions. More... | |
enum | IrqType_t { TcIrq = 0, ErrIrq } |
Interrupt callback types. More... | |
enum | Status { Ok = 0, Error = -1, ErrChInUse = -2 } |
Return status codes. More... | |
enum | GPDMA_CONNECTION { SSP0_Tx = 0UL, SSP0_Rx = 1UL, SSP1_Tx = 2UL, SSP1_Rx = 3UL, ADC = 4UL, I2S_Channel_0 = 5UL, I2S_Channel_1 = 6UL, DAC = 7UL, UART0_Tx = 8UL, UART0_Rx = 9UL, UART1_Tx = 10UL, UART1_Rx = 11UL, UART2_Tx = 12UL, UART2_Rx = 13UL, UART3_Tx = 14UL, UART3_Rx = 15UL, MAT0_0 = 16UL, MAT0_1 = 17UL, MAT1_0 = 18UL, MAT1_1 = 19UL, MAT2_0 = 20UL, MAT2_1 = 21UL, MAT3_0 = 22UL, MAT3_1 = 23UL } |
DMA Connection number definitions. More... | |
enum | GPDMA_TRANSFERTYPE { m2m = 0UL, m2p = 1UL, p2m = 2UL, p2p = 3UL, g2m = 4UL, m2g = 5UL } |
GPDMA Transfer type definitions. More... | |
enum | GPDMA_BSIZE { _1 = 0UL, _4 = 1UL, _8 = 2UL, _16 = 3UL, _32 = 4UL, _64 = 5UL, _128 = 6UL, _256 = 7UL } |
Burst size in Source and Destination definitions */. More... | |
enum | GPDMA_WIDTH { byte = 0UL, halfword = 1UL, word = 2UL } |
Width in Src transfer width and Dest transfer width definitions */. More... | |
enum | GPDMA_REQSEL { uart = 0UL, timer = 1UL } |
DMA Request Select Mode definitions. */. More... | |
enum | Config { _E = 1, _M = 2 } |
GPDMA Control register bits. More... | |
enum | CConfig { _CE = (1UL << 0), _IE = (1UL << 14), _ITC = (1UL << 15), _L = (1UL << 16), _A = (1UL << 17), _H = (1UL << 18) } |
GPDMA Channel config register bits. More... | |
Public Member Functions | |
MODDMA () | |
The MODDMA constructor is used to initialise the DMA controller object. | |
~MODDMA () | |
The MODDMA destructor. | |
void | init (bool isConstructorCalling, int Channels=0xFF, int Tc=0xFF, int Err=0xFF) |
Used to setup the DMA controller to prepare for a data transfer. | |
uint32_t | Prepare (MODDMA_Config *c) |
Used to setup and enable the DMA controller. | |
uint32_t | Setup (MODDMA_Config *c) |
Used to setup the DMA controller to prepare for a data transfer. | |
void | Enable (CHANNELS ChannelNumber) |
Enable and begin data transfer. | |
void | Enable (uint32_t ChannelNumber) |
Enable and begin data transfer (overloaded function) | |
void | Enable (MODDMA_Config *config) |
Enable and begin data transfer (overloaded function) | |
void | Disable (CHANNELS ChannelNumber) |
Disable a channel and end data transfer. | |
void | Disable (uint32_t ChannelNumber) |
Disable a channel and end data transfer (overloaded function) | |
bool | Enabled (CHANNELS ChannelNumber) |
Is the specified channel enabled? | |
bool | Enabled (uint32_t ChannelNumber) |
Is the specified channel enabled? (overloaded function) | |
MODDMA_Config * | getConfig (void) |
Get a pointer to the current configuration the ISR is servicing. | |
void | setIrqProcessingChannel (CHANNELS n) |
Set which channel the ISR is currently servicing. | |
CHANNELS | irqProcessingChannel (void) |
Gets which channel the ISR is currently servicing. | |
void | setIrqType (IrqType_t n) |
Sets which type of IRQ the ISR is making a callback for. | |
IrqType_t | irqType (void) |
Get which type of IRQ the ISR is calling you about, terminal count or error. | |
void | clearTcIrq (CHANNELS n) |
Clear the interrupt after handling. | |
void | clearTcIrq (void) |
Clear the interrupt the ISR is currently handing. | |
void | clearErrIrq (CHANNELS n) |
Clear the error interrupt after handling. | |
void | clearErrIrq (void) |
Clear the error interrupt the ISR is currently handing. | |
bool | isActive (CHANNELS ChannelNumber) |
Is the supplied channel currently active? | |
void | haltChannel (CHANNELS ChannelNumber) |
Halt the supplied channel. | |
uint32_t | getControl (CHANNELS ChannelNumber) |
get a channels control register. | |
void | haltAndWaitChannelComplete (CHANNELS n) |
Wait for channel transfer to complete and then halt. | |
void | attach_tc (void(*fptr)(void)) |
Attach a callback to the TC IRQ controller. | |
template<typename T > | |
void | attach_tc (T *tptr, void(T::*mptr)(void)) |
Attach a callback to the TC IRQ controller. | |
void | attach_err (void(*fptr)(void)) |
Attach a callback to the ERR IRQ controller. | |
template<typename T > | |
void | attach_err (T *tptr, void(T::*mptr)(void)) |
Attach a callback to the ERR IRQ controller. | |
uint32_t | lli (CHANNELS ChannelNumber, MODDMA_LLI *set=0) |
Get the Linked List index regsiter for the requested channel. | |
Data Fields | |
MODDMA_Config * | setups [8] |
A store for up to 8 (8 channels) of configurations. | |
FunctionPointer | isrIntTCStat |
The MODDMA controllers terminal count interrupt callback. | |
FunctionPointer | isrIntErrStat |
The MODDMA controllers error interrupt callback. |
Detailed Description
MODDMA GPDMA Controller.
- See also:
- http://mbed.org/cookbook/MODDMA
- example1.cpp
- The MODDMA API
MODDMA defines a GPDMA controller and multiple DMA configurations that allow for DMA transfers from memory to memory, memory to peripheral or peripheral to memory.
At the heart of the library is the MODDMA class that defines a single instance controller that manages all the GPDMA hardware registers and interrupts. The controller can accept multiple configurations that define the channel transfers. Each configuration specifies the source and destination information and other associated parts to maintain the transfer process.
Standard example:
#include "mbed.h" #include "MODDMA.h" DigitalOut led1(LED1); Serial pc(USBTX, USBRX); // tx, rx MODDMA dma; int main() { // Create a string buffer to send directly to a Uart/Serial char s[] = "***DMA*** ABCDEFGHIJKLMNOPQRSTUVWXYZ ***DMA***"; // Create a transfer configuarion MODDMA_Config *config = new MODDMA_Config; // Provide a "minimal" setup for demo purposes. config ->channelNum ( MODDMA::Channel_0 ) // The DMA channel to use. ->srcMemAddr ( (uint32_t) &s ) // A pointer to the buffer to send. ->transferSize ( sizeof(s) ) // The size of that buffer. ->transferType ( MODDMA::m2p ) // Source is memory, destination is peripheral ->dstConn ( MODDMA::UART0_Tx ) // Specifically, peripheral is Uart0 TX (USBTX, USBRX) ; // config end. // Pass the configuration to the MODDMA controller. dma.Setup( config ); // Enable the channel and begin transfer. dma.Enable( config->channelNum() ); while(1) { led1 = !led1; wait(0.25); } }
Definition at line 251 of file MODDMA.h.
Member Enumeration Documentation
enum CConfig |
enum CHANNELS |
enum Config |
enum GPDMA_BSIZE |
enum GPDMA_CONNECTION |
DMA Connection number definitions.
- Enumerator:
enum GPDMA_REQSEL |
enum GPDMA_TRANSFERTYPE |
GPDMA Transfer type definitions.
- Enumerator:
enum GPDMA_WIDTH |
enum IrqType_t |
enum Status |
Constructor & Destructor Documentation
MODDMA | ( | ) |
Member Function Documentation
void clearTcIrq | ( | CHANNELS | n ) |
void clearTcIrq | ( | void | ) |
IrqType_t irqType | ( | void | ) |
uint32_t lli | ( | CHANNELS | ChannelNumber, |
MODDMA_LLI * | set = 0 |
||
) |
void setIrqProcessingChannel | ( | CHANNELS | n ) |
void setIrqType | ( | IrqType_t | n ) |
Field Documentation
FunctionPointer isrIntErrStat |
FunctionPointer isrIntTCStat |
MODDMA_Config* setups[8] |
A store for up to 8 (8 channels) of configurations.
- See also:
- MODDMA_Config
Generated on Tue Jul 12 2022 12:57:52 by
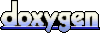