
My modifications/additions to the code
Dependencies: ADXL345 ADXL345_I2C IMUfilter ITG3200 Servo fishgait mbed-rtos mbed pixy_cam
Fork of robotic_fish_ver_4_8 by
guardian.cpp
00001 #include "guardian.h" 00002 00003 Guardian::Guardian(PinName modpin, PinName gainpin) 00004 :mod(modpin), 00005 gain(gainpin) 00006 { 00007 00008 mod.write(0.5); //set autopilot to off 00009 gain.write(1.00); 00010 } 00011 00012 void Guardian::set3D() //set autopilot to 3D 00013 { 00014 mod.write(1.00); 00015 return; 00016 } 00017 00018 void Guardian::set2D() //set autopilot 2D 00019 { 00020 mod.write(0.00); 00021 return; 00022 } 00023 00024 void Guardian::setoff() 00025 { 00026 mod.write(0.5); 00027 return; 00028 } 00029 00030 void Guardian::calibrate() //must be done within 15 sec of power on 00031 { 00032 set2D(); 00033 wait(500); 00034 set3D(); 00035 wait(500); 00036 set2D(); 00037 wait(2000); 00038 //now look for the twitch 00039 return; 00040 } 00041 00042 void Guardian::setmod(float val) 00043 { 00044 mod.write(val); 00045 return; 00046 } 00047 00048 void Guardian::setgain(float val) 00049 { 00050 gain.write(val); 00051 return; 00052 }
Generated on Sun Jul 17 2022 22:28:23 by
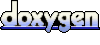