
My modifications/additions to the code
Dependencies: ADXL345 ADXL345_I2C IMUfilter ITG3200 Servo fishgait mbed-rtos mbed pixy_cam
Fork of robotic_fish_ver_4_8 by
PwmIn.cpp
00001 #include "PwmIn.h" 00002 00003 PwmIn::PwmIn(PinName p, float dutyMin, float dutyMax) 00004 : _p(p) { 00005 _p.rise(this, &PwmIn::rise); 00006 _p.fall(this, &PwmIn::fall); 00007 _period = 20000; 00008 _pulsewidth = 0; 00009 _t.start(); 00010 _risen = false; 00011 _dutyMin = dutyMin; 00012 _dutyMax = dutyMax; 00013 _dutyDelta = dutyMax - dutyMin; 00014 00015 } 00016 00017 float PwmIn::period() { 00018 return float(_period)/1000000; 00019 } 00020 00021 float PwmIn::pulsewidth() { 00022 return float(_pulsewidth)/1000000; 00023 } 00024 00025 float PwmIn::dutycycle() { 00026 return float(_pulsewidth) / float(_period); 00027 } 00028 00029 float PwmIn::dutycyclescaledup() { 00030 float duty = float(_pulsewidth) / float(_period); 00031 float dutyAdjusted = (duty > _dutyMin) ? ((duty-_dutyMin)/_dutyDelta) : 0.0; 00032 return (dutyAdjusted < 1.0) ? dutyAdjusted : 1.0; 00033 00034 } 00035 00036 void PwmIn::rise() 00037 { 00038 _tmp = _t.read_us(); 00039 if(_tmp > 19000) { 00040 _period = _tmp; 00041 _risen = true; 00042 _t.reset(); 00043 } 00044 } 00045 00046 void PwmIn::fall() 00047 { 00048 _tmp = _t.read_us(); 00049 if(_tmp > 500 && _tmp < 5000 && _risen == true) { 00050 _pulsewidth = _tmp; 00051 _risen = false; 00052 } 00053 } 00054 00055
Generated on Sun Jul 17 2022 22:28:23 by
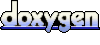