X_NUCLEO_NFC02A1 library for M24LR
Dependencies: ST_INTERFACES
Dependents: HelloWorld_NFC02A1_mbedOS HelloWorld_NFC02A1laatste HelloWorld_NFC02A1
Fork of X_NUCLEO_NFC02A1 by
M24LR.h
00001 /** 00002 ****************************************************************************** 00003 * @file m24lr.h 00004 * @author AMG Central Lab 00005 * @version V2.0.0 00006 * @date 19 May 2017 00007 * @brief header file for M24LR driver . 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 00039 #ifndef __M24LR_H 00040 #define __M24LR_H 00041 00042 #include "DevI2C.h" 00043 #include "Nfc.h" 00044 #include "NDefNfcTag.h" 00045 #include "NDefNfcTagM24LR.h" 00046 00047 //#include "lib_NDEF_URI.h" 00048 /* Exported constants --------------------------------------------------------*/ 00049 /** @defgroup M24LR_Exported_Constants 00050 * @{ 00051 */ 00052 #define I_AM_M24LR04 0x5A 00053 #define I_AM_M24LR16 0x4E 00054 #define I_AM_M24LR64 0x5E 00055 00056 #ifndef NULL 00057 #define NULL (void *) 0 00058 #endif 00059 00060 #define M24LR_PAGEWRITE_NBBYTE 4 00061 00062 #define M24LR_ADDR_DATA_I2C 0xA6 00063 #define M24LR_ADDR_SYST_I2C 0xAE 00064 #define M24LR_I2C_TIMEOUT 200 /* I2C Time out (ms), this is the maximum time needed by M24LR to complete any command */ 00065 00066 #define M24LR_IT_BUSY_MASK 0x01 00067 #define M24LR_IT_WIP_MASK 0x02 00068 00069 /* Registers address */ 00070 #define M24LR_SSS_REG 0x0000 00071 #define M24LR_LOCK_REG 0x0800 00072 #define M24LR_I2C_PWD_REG 0x0900 00073 #define M24LR_CFG_REG 0x0910 00074 #define M24LR_AFI_REG 0x0912 00075 #define M24LR_DSFID_REG 0x0913 00076 #define M24LR_UID_REG 0x0914 00077 #define M24LR_ICREF_REG 0x091C 00078 #define M24LR_MEMSIZE_REG 0x091D 00079 #define M24LR_CTRL_REG 0x0920 00080 00081 /* Registers mask */ 00082 #define M24LR_SSS_LOCK_MASK 0x01 00083 #define M24LR_SSS_RW_MASK 0x06 00084 #define M24LR_SSS_PASSCTRL_MASK 0x18 00085 #define M24LR_LOCK_MASK 0x0F 00086 00087 #define M24LR_CFG_EHCFG0_MASK 0x01 00088 #define M24LR_CFG_EHCFG1_MASK 0x02 00089 #define M24LR_CFG_EHMODE_MASK 0x04 00090 #define M24LR_CFG_WIPBUSY_MASK 0x08 00091 00092 #define M24LR_CTRL_EHEN_MASK 0x01 00093 #define M24LR_CTRL_FIELD_MASK 0x02 00094 #define M24LR_CTRL_TPROG_MASK 0x80 00095 00096 00097 /** 00098 * @brief NFCTAG status enumerator definition 00099 */ 00100 typedef enum 00101 { 00102 NFCTAG_OK = 0, 00103 NFCTAG_ERROR = 1, 00104 NFCTAG_BUSY = 2, 00105 NFCTAG_TIMEOUT = 3 00106 } NFCTAG_StatusTypeDef; 00107 00108 /** 00109 * @brief M24LR VOUT Configuration enumerator definition 00110 */ 00111 typedef enum 00112 { 00113 M24LR_EH_Cfg_6MA = 0, 00114 M24LR_EH_Cfg_3MA, 00115 M24LR_EH_Cfg_1MA, 00116 M24LR_EH_Cfg_300UA 00117 } M24LR_EH_CFG_VOUT; 00118 00119 /** 00120 * @brief M24LR FIELD status enumerator definition 00121 */ 00122 typedef enum 00123 { 00124 M24LR_FIELD_OFF = 0, 00125 M24LR_FIELD_ON 00126 } M24LR_FIELD_STATUS; 00127 00128 /** 00129 * @brief M24LR TT-PROG status enumerator definition 00130 */ 00131 typedef enum 00132 { 00133 M24LR_T_PROG_NO = 0, 00134 M24LR_T_PROG_OK 00135 } M24LR_T_PROG_STATUS; 00136 00137 /** 00138 * @brief M24LR Energy Harvesting status enumerator definition 00139 */ 00140 typedef enum 00141 { 00142 M24LR_EH_DISABLE = 0, 00143 M24LR_EH_ENABLE 00144 } M24LR_EH_STATUS; 00145 00146 /** 00147 * @brief M24LR Energy Harvesting mode enumerator definition 00148 */ 00149 typedef enum 00150 { 00151 M24LR_EH_MODE_ENABLE = 0, 00152 M24LR_EH_MODE_DISABLE 00153 } M24LR_EH_MODE_STATUS; 00154 00155 /** 00156 * @brief M24LR GPO status enumerator definition 00157 */ 00158 typedef enum 00159 { 00160 M24LR_GPO_BUSY = 0, 00161 M24LR_GPO_WIP 00162 } M24LR_GPO_STATUS; 00163 00164 /** 00165 * @brief M24LR Memory information structure definition 00166 */ 00167 typedef struct 00168 { 00169 uint8_t BlockSize; 00170 uint16_t Mem_Size; 00171 } M24LR_Mem_Size; 00172 00173 /** 00174 * @brief M24LR I2C Write Lock register structure definition 00175 */ 00176 typedef struct 00177 { 00178 uint8_t sectors_7_0; 00179 uint8_t sectors_15_8; 00180 uint8_t sectors_23_16; 00181 uint8_t sectors_31_24; 00182 uint8_t sectors_39_32; 00183 uint8_t sectors_47_40; 00184 uint8_t sectors_55_48; 00185 uint8_t sectors_63_56; 00186 } M24LR_Lock_Sectors; 00187 00188 /** 00189 * @brief M24LR UID information structure definition 00190 */ 00191 typedef struct 00192 { 00193 uint32_t MSB_UID; 00194 uint32_t LSB_UID; 00195 } M24LR_UID; 00196 00197 /** 00198 * @brief M24LR Sector Security register structure definition 00199 */ 00200 typedef struct 00201 { 00202 uint8_t SectorLock; 00203 uint8_t RW_Protection; 00204 uint8_t PassCtrl; 00205 } M24LR_SECTOR_SEC; 00206 00207 /** 00208 * @brief M24LR class. 00209 */ 00210 class M24LR : public Nfc { 00211 public: 00212 NFCTAG_StatusTypeDef i2c_init( void ); 00213 NFCTAG_StatusTypeDef i2c_read_id( uint8_t * const pICRef ); 00214 NFCTAG_StatusTypeDef i2c_is_device_ready( const uint32_t Trials ); 00215 NFCTAG_StatusTypeDef i2c_configure_GPO( const uint16_t ITConf ); 00216 NFCTAG_StatusTypeDef i2c_get_GPO_status( uint16_t * const pGPOStatus ); 00217 NFCTAG_StatusTypeDef i2c_read_data( uint8_t * const pData, const uint16_t TarAddr, const uint16_t NbByte ); 00218 NFCTAG_StatusTypeDef i2c_write_data( const uint8_t * const pData, const uint16_t TarAddr, const uint16_t NbByte ); 00219 NFCTAG_StatusTypeDef i2c_read_register( uint8_t * const pData, const uint16_t TarAddr, const uint16_t NbByte ); 00220 NFCTAG_StatusTypeDef i2c_write_register( const uint8_t * const pData, const uint16_t TarAddr, const uint16_t NbByte ); 00221 00222 /* Extended Functions */ 00223 NFCTAG_StatusTypeDef i2c_read_UID( M24LR_UID * const pUid ); 00224 NFCTAG_StatusTypeDef i2c_read_DSFID( uint8_t * const pDsfid ); 00225 NFCTAG_StatusTypeDef i2c_read_AFI( uint8_t * const pAfi ); 00226 NFCTAG_StatusTypeDef i2c_read_I2C_lock_sector( M24LR_Lock_Sectors * const pLock_sector ); 00227 NFCTAG_StatusTypeDef i2c_I2C_lock_sector( const uint8_t Sector ); 00228 NFCTAG_StatusTypeDef i2c_I2C_unlock_sector( const uint8_t Sector ); 00229 NFCTAG_StatusTypeDef i2c_present_I2C_password( const uint32_t PassWord ); 00230 NFCTAG_StatusTypeDef i2c_write_I2C_password( const uint32_t PassWord ); 00231 NFCTAG_StatusTypeDef i2c_read_SSSx( const uint8_t SectorNb, M24LR_SECTOR_SEC * const pData ); 00232 NFCTAG_StatusTypeDef i2c_write_SSSx( const uint8_t SectorNb, const M24LR_SECTOR_SEC * const pData ); 00233 NFCTAG_StatusTypeDef i2c_read_mem_size( M24LR_Mem_Size * const pSizeInfo ); 00234 NFCTAG_StatusTypeDef i2c_get_RF_WIP_busy( M24LR_GPO_STATUS * const pRf_Wip_Busy ); 00235 NFCTAG_StatusTypeDef i2c_setRF_Busy( void ); 00236 NFCTAG_StatusTypeDef i2c_set_RF_WIP( void ); 00237 NFCTAG_StatusTypeDef i2c_read_EH_mode( M24LR_EH_MODE_STATUS * const pEH_mode ); 00238 NFCTAG_StatusTypeDef i2c_enable_EH_mode( void ); 00239 NFCTAG_StatusTypeDef i2c_disable_EH_mode( void ); 00240 NFCTAG_StatusTypeDef i2c_read_EH_cfg( M24LR_EH_CFG_VOUT * const pEH_Cfg ); 00241 NFCTAG_StatusTypeDef i2c_write_EH_cfg( const M24LR_EH_CFG_VOUT EH_Cfg ); 00242 NFCTAG_StatusTypeDef i2c_get_EH( M24LR_EH_STATUS * const pEH_Val ); 00243 NFCTAG_StatusTypeDef i2c_set_EH( void ); 00244 NFCTAG_StatusTypeDef i2c_reset_EH( void ); 00245 NFCTAG_StatusTypeDef i2c_get_RF_field( M24LR_FIELD_STATUS * const pRF_Field ); 00246 NFCTAG_StatusTypeDef i2c_get_TProg( M24LR_T_PROG_STATUS * const pT_Prog ); 00247 00248 void enable_energy_harvesting( void ); 00249 NFCTAG_StatusTypeDef initialization( void ); 00250 00251 virtual int read_binary(uint16_t Offset, uint8_t NbByteToRead, uint8_t *pBufferRead) { 00252 return i2c_read_data( pBufferRead, Offset, NbByteToRead ); 00253 00254 } 00255 00256 virtual int update_binary(uint16_t Offset, uint8_t NbByteToWrite,uint8_t *pDataToWrite) { 00257 return i2c_write_data( pDataToWrite, Offset, NbByteToWrite ); 00258 } 00259 00260 M24LR(uint8_t const address, uint8_t const addressData, DevI2C &devI2C ): 00261 NDefTagUtil(*this), i2c_address_syst(address), i2c_address_data(addressData), dev_I2C(devI2C) {} 00262 00263 NDefLib::NDefNfcTag& get_NDef_tag(){ 00264 return NDefTagUtil; 00265 } 00266 00267 virtual int init(void *ptr) { 00268 (void)ptr; 00269 return i2c_init(); 00270 } 00271 00272 virtual int read_id(uint8_t *id) { 00273 return i2c_read_id(id); 00274 } 00275 00276 private: 00277 static uint8_t NfctagInitialized; 00278 /* Object implementing the interface to use the NDefLib. */ 00279 NDefNfcTagM24LR NDefTagUtil; 00280 00281 uint8_t i2c_address_syst; 00282 uint8_t i2c_address_data; 00283 DevI2C &dev_I2C; 00284 }; 00285 00286 #endif /* __M24LR_H */ 00287 00288 00289 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed Jul 13 2022 03:37:30 by
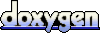