Class Module for MMA845x I2C Accelerometer.
Dependents: mDotEVBM2X MTDOT-EVBDemo-DRH MTDOT-BOX-EVB-Factory-Firmware-LIB-108 MTDOT-UDKDemo_Senet ... more
Fork of MMA845x by
MMA845x Class Reference
API abstraction for the MMA845x 3-axis accelerometer IC initial version will be polling only. More...
#include <MMA845x.h>
Public Types | |
enum | SA0 { SA0_VSS = 0, SA0_VDD } |
Possible terminations for the ADDR pin. More... | |
enum | WHO_AM_I_VAL { MMA8451 = 0x1a, MMA8452 = 0x2a, MMA8453 = 0x3a } |
Device ID's that this class is compatible with. More... | |
enum | SYS_MODE |
operating mode of MMA845x More... | |
enum | STATUS |
flags for data overwrite and data ready More... | |
enum | RANGE |
values for measurement range positive and negative More... | |
enum | RESOLUTION |
selections for resolution of data, 8 bit or maximum More... | |
enum | LOW_NOISE |
Low Noise mode Note: 4g max reading when on. More... | |
enum | HPF_MODE |
High Pass Filter mode. More... | |
enum | DATA_RATE |
values for normal output data rate in Hz More... | |
enum | ASLP_DATA_RATE |
values for auto_sleep mode data rate in HZ More... | |
enum | OVERSAMPLE_MODE |
sets the oversample mode, Normal, Low power and noise, High resolution, or low power More... | |
enum | REGISTER |
The device register map. More... | |
Public Member Functions | |
MMA845x (I2C &i2c, SA0 const i2c_addr=SA0_VSS, InterruptIn *int1=NULL, InterruptIn *int2=NULL) | |
Create the MMA845x object. | |
int16_t | getX (void) |
Get the X data. | |
int16_t | getY (void) |
Get the Y data. | |
int16_t | getZ (void) |
Get the Z data. | |
MMA845x_DATA | getXYZ (void) |
Get the XYZ data structure. | |
char | getWhoAmI (void) const |
Get the XYZ data structure. | |
uint8_t | setCommonParameters (RANGE range, RESOLUTION resolution, LOW_NOISE lo_noise, DATA_RATE data_rate, OVERSAMPLE_MODE os_mode, HPF_MODE hpf_mode) const |
Setup the MMA845x for standard accelerometer read mode - set the measurement range using RANGE enum - set the ADC resolution using the RESOLUTION enum - Set the Low-Noise mode using the LOW_NOISE enum - set the aquisition rate using the DATA_RATE enum - Set the Over sample mode suing the OVERSAMPLE_MODE enum - Set the Hi pass filter mode using the HPF_MOSE enum. | |
uint8_t | enableMotionDetect (void) const |
Ebnable Motion detect mode and interrupt handler. | |
uint8_t | enablePulseDetect (void) const |
Enable Pulse Detect mode and interrupt handler. | |
uint8_t | enableOrientationDetect (void) const |
Enable Orientation mode and interrupt handler. | |
uint8_t | enableTransientDetect (void) const |
Enable Transient detect mode and interrupt handler. | |
uint8_t | enableAutoSleep (void) const |
Enable Autosleep function and interrupt handler. | |
uint8_t | enableFIFO (void) const |
Enbale FIFO function and interrupt handler. | |
uint8_t | standbyMode (void) const |
Put the MMA845x in the Standby mode. | |
uint8_t | activeMode (void) const |
Put the MMA845x in the active mode. | |
uint8_t | getStatus (void) const |
Check the MMA845x status register. |
Detailed Description
API abstraction for the MMA845x 3-axis accelerometer IC initial version will be polling only.
Interrupt service and rtos support will be added at a later point
Definition at line 107 of file MMA845x.h.
Member Enumeration Documentation
enum ASLP_DATA_RATE |
enum LOW_NOISE |
enum OVERSAMPLE_MODE |
enum RANGE |
enum RESOLUTION |
enum SA0 |
enum WHO_AM_I_VAL |
Constructor & Destructor Documentation
MMA845x | ( | I2C & | i2c, |
SA0 const | i2c_addr = SA0_VSS , |
||
InterruptIn * | int1 = NULL , |
||
InterruptIn * | int2 = NULL |
||
) |
Create the MMA845x object.
- Parameters:
-
i2c - A defined I2C object int1 - A defined InterruptIn object pointer. Default NULL for polling mode int2 - A defined InterruptIn object pointer. Default NULL for polling mode i2c_addr - state of pin SA0 TODO - need to add interrupt support
Definition at line 34 of file MMA845x.cpp.
Member Function Documentation
uint8_t activeMode | ( | void | ) | const |
Put the MMA845x in the active mode.
- Returns:
- status of command
Definition at line 181 of file MMA845x.cpp.
uint8_t enableAutoSleep | ( | void | ) | const |
Enable Autosleep function and interrupt handler.
- Returns:
- status of command TODO - need to implement function
Definition at line 163 of file MMA845x.cpp.
uint8_t enableFIFO | ( | void | ) | const |
Enbale FIFO function and interrupt handler.
- Returns:
- status of command TODO - need to implement function
Definition at line 169 of file MMA845x.cpp.
uint8_t enableMotionDetect | ( | void | ) | const |
Ebnable Motion detect mode and interrupt handler.
- Returns:
- status of command TODO - need to implement function
Definition at line 133 of file MMA845x.cpp.
uint8_t enableOrientationDetect | ( | void | ) | const |
Enable Orientation mode and interrupt handler.
- Returns:
- status of command TODO - need to implement function
Definition at line 145 of file MMA845x.cpp.
uint8_t enablePulseDetect | ( | void | ) | const |
Enable Pulse Detect mode and interrupt handler.
- Returns:
- status of command TODO - need to implement function
Definition at line 139 of file MMA845x.cpp.
uint8_t enableTransientDetect | ( | void | ) | const |
Enable Transient detect mode and interrupt handler.
- Returns:
- status of command TODO - need to implement function
Definition at line 157 of file MMA845x.cpp.
uint8_t getStatus | ( | void | ) | const |
char getWhoAmI | ( | void | ) | const |
Get the XYZ data structure.
- Returns:
- accelerometer ID code. Test versus the WHO_AM_I_VAL enum
Definition at line 277 of file MMA845x.cpp.
int16_t getX | ( | void | ) |
Get the X data.
- Returns:
- The last valid X-axis reading from the accelerometer
Definition at line 222 of file MMA845x.cpp.
MMA845x_DATA getXYZ | ( | void | ) |
Get the XYZ data structure.
- Returns:
- The last valid X, Y, and Z-axis readings from the accelerometer
Definition at line 260 of file MMA845x.cpp.
int16_t getY | ( | void | ) |
Get the Y data.
- Returns:
- The last valid Y-axis reading from the accelerometer
Definition at line 235 of file MMA845x.cpp.
int16_t getZ | ( | void | ) |
Get the Z data.
- Returns:
- The last Z-axis valid reading from the accelerometer
Definition at line 247 of file MMA845x.cpp.
uint8_t setCommonParameters | ( | RANGE | range, |
RESOLUTION | resolution, | ||
LOW_NOISE | lo_noise, | ||
DATA_RATE | data_rate, | ||
OVERSAMPLE_MODE | os_mode, | ||
HPF_MODE | hpf_mode | ||
) | const |
Setup the MMA845x for standard accelerometer read mode - set the measurement range using RANGE enum - set the ADC resolution using the RESOLUTION enum - Set the Low-Noise mode using the LOW_NOISE enum - set the aquisition rate using the DATA_RATE enum - Set the Over sample mode suing the OVERSAMPLE_MODE enum - Set the Hi pass filter mode using the HPF_MOSE enum.
- Returns:
- status of command
This sets the resolution, range, data rate, oversample mode, hi and lo pass filter.
Definition at line 95 of file MMA845x.cpp.
uint8_t standbyMode | ( | void | ) | const |
Put the MMA845x in the Standby mode.
- Returns:
- status of command
Definition at line 193 of file MMA845x.cpp.
Generated on Thu Jul 14 2022 17:24:22 by
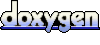