Class Module for MMA845x I2C Accelerometer.
Dependents: mDotEVBM2X MTDOT-EVBDemo-DRH MTDOT-BOX-EVB-Factory-Firmware-LIB-108 MTDOT-UDKDemo_Senet ... more
Fork of MMA845x by
MMA845x.h
00001 /** 00002 * @file MMA845x.h 00003 * @brief Device driver - MMA845x 3-axis accelerometer IC 00004 * @author Tim Barr 00005 * @version 1.0 00006 * @see http://cache.freescale.com/files/sensors/doc/data_sheet/MMA8451Q.pdf 00007 * @see http://cache.freescale.com/files/sensors/doc/data_sheet/MMA8452Q.pdf 00008 * @see http://cache.freescale.com/files/sensors/doc/data_sheet/MMA8453Q.pdf 00009 * 00010 * Copyright (c) 2015 00011 * 00012 * Licensed under the Apache License, Version 2.0 (the "License"); 00013 * you may not use this file except in compliance with the License. 00014 * You may obtain a copy of the License at 00015 * 00016 * http://www.apache.org/licenses/LICENSE-2.0 00017 * 00018 * Unless required by applicable law or agreed to in writing, software 00019 * distributed under the License is distributed on an "AS IS" BASIS, 00020 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00021 * See the License for the specific language governing permissions and 00022 * limitations under the License. 00023 * Forked from 00024 */ 00025 00026 #ifndef MMA845X_H 00027 #define MMA845X_H 00028 00029 #include "mbed.h" 00030 00031 /** Using the Sparkfun SEN-10955 00032 * 00033 * Example: 00034 * @code 00035 * #include "mbed.h" 00036 * #include "MMA845x.h" 00037 * 00038 00039 * 00040 * int main() 00041 * { 00042 00043 * } 00044 * @endcode 00045 */ 00046 00047 00048 /** 00049 * @class MMA845x_DATA 00050 * @brief API abstraction for the MMA845x 3-axis accelerometer IC data 00051 */ 00052 class MMA845x_DATA 00053 { 00054 public: 00055 00056 volatile int16_t _x ; /*!< volatile data variable */ 00057 volatile int16_t _y ; /*!< volatile data variable */ 00058 volatile int16_t _z ; /*!< volatile data variable */ 00059 00060 /** Create the MMA845x_DATA object initialized to the parameter (or 0 if none) 00061 * @param x - the init value of _x 00062 * @param y - the init value of _y 00063 * @param x - the init value of _z 00064 */ 00065 MMA845x_DATA(int16_t x = 0, int16_t y = 0, int16_t z = 0) : _x (x), _y (y), _z (z) {} 00066 00067 /** Overloaded '=' operator to allow shorthand coding, assigning objects to one another 00068 * @param rhs - an object of the same type to assign ourself the same values of 00069 * @return this 00070 */ 00071 MMA845x_DATA &operator= (MMA845x_DATA const &rhs) 00072 { 00073 _x = rhs._x ; 00074 _y = rhs._y ; 00075 _z = rhs._z ; 00076 00077 return *this; 00078 } 00079 00080 /** Overloaded '=' operator to allow shorthand coding, assigning objects to one another 00081 * @param val - Assign each data member (_x, _y, _z) this value 00082 * @return this 00083 */ 00084 MMA845x_DATA &operator= (int16_t const val) 00085 { 00086 _x = _y = _z = val; 00087 00088 return *this; 00089 } 00090 00091 /** Overloaded '==' operator to allow shorthand coding, test objects to one another 00092 * @param rhs - the object to compare against 00093 * @return 1 if the data members are the same and 0 otherwise 00094 */ 00095 bool operator== (MMA845x_DATA const &rhs) const 00096 { 00097 return ((_x == rhs._x )&&(_y == rhs._y )&&(_z == rhs._z )) ? 1 : 0; 00098 } 00099 }; 00100 00101 /** 00102 * @class MMA845x 00103 * @brief API abstraction for the MMA845x 3-axis accelerometer IC 00104 * initial version will be polling only. Interrupt service and rtos support will 00105 * be added at a later point 00106 */ 00107 class MMA845x 00108 { 00109 public: 00110 00111 /** 00112 * @enum SA0 00113 * @brief Possible terminations for the ADDR pin 00114 */ 00115 enum SA0 00116 { 00117 SA0_VSS = 0, /*!< SA0 connected to VSS */ 00118 SA0_VDD /*!< SA0 connected to VDD */ 00119 }; 00120 00121 /** 00122 * @enum WHO_AM_I_VAL 00123 * @brief Device ID's that this class is compatible with 00124 */ 00125 enum WHO_AM_I_VAL 00126 { 00127 MMA8451 = 0x1a, /*!< MMA8451 WHO_AM_I register content */ 00128 MMA8452 = 0x2a, /*!< MMA8452 WHO_AM_I register content */ 00129 MMA8453 = 0x3a, /*!< MMA8453 WHO_AM_I register content */ 00130 }; 00131 00132 /** 00133 * @enum SYS_MODE 00134 * @brief operating mode of MMA845x 00135 */ 00136 enum SYS_MODE 00137 { 00138 STANDBY = 0, 00139 WAKE, SLEEP 00140 }; 00141 00142 /** 00143 * @enum STATUS 00144 * @brief flags for data overwrite and data ready 00145 */ 00146 enum STATUS 00147 { 00148 XDR = 0x01, 00149 YDR = 0x02, 00150 ZDR = 0x04, 00151 XYZDR = 0x08, 00152 XOW = 0x10, 00153 YOW = 0x20, 00154 ZOW = 0x40, 00155 XYZOW = 0x80 00156 }; 00157 00158 /** 00159 * @enum RANGE 00160 * @brief values for measurement range positive and negative 00161 */ 00162 enum RANGE 00163 { 00164 RANGE_2g = 0, 00165 RANGE_4g, RANGE_8g 00166 }; 00167 00168 /** 00169 * @enum RESOLUTION 00170 * @brief selections for resolution of data, 8 bit or maximum 00171 */ 00172 enum RESOLUTION 00173 { 00174 RES_MAX = 0, /* Read back full resolution - normal mode*/ 00175 RES_8BIT = 2 /* Read back 8 bit values only - fast mode*/ 00176 }; 00177 00178 /** 00179 * @enum LOW_NOISE 00180 * @brief Low Noise mode Note: 4g max reading when on 00181 */ 00182 enum LOW_NOISE 00183 { 00184 LN_OFF = 0x00, /* Low Noise mode off */ 00185 LN_ON = 0x02 /* Low Noise mode on, 4g max readings */ 00186 }; 00187 00188 /** 00189 * @enum HPF_MODE 00190 * @brief High Pass Filter mode 00191 */ 00192 enum HPF_MODE 00193 { 00194 HPF_OFF = 0x00, /* High Pass Filter mode off */ 00195 HPF_ON = 0x10 /* High Pass Filter mode on */ 00196 }; 00197 00198 /** 00199 * @enum DATA_RATE 00200 * @brief values for normal output data rate in Hz 00201 */ 00202 enum DATA_RATE 00203 { 00204 DR_800 = 0x00, 00205 DR_400 = 0x08, 00206 DR_200 = 0x10, 00207 DR_100 = 0x18, 00208 DR_50 = 0x20, 00209 DR_12_5 = 0x28, 00210 DR_6_25 = 0x30, 00211 DR_1_56 = 0x38 00212 }; 00213 /** 00214 * @enum ASLP_DATA_RATE 00215 * @brief values for auto_sleep mode data rate in HZ 00216 */ 00217 enum ASLP_DATA_RATE 00218 { 00219 ASLPDR_50 = 0x00, 00220 ALSPDR_12_5 = 0x40, 00221 ASLPDR_6_25 = 0x80, 00222 ASLPDR_1_56 = 0xB0 00223 }; 00224 00225 /** 00226 * @enum OVERSAMPLE_MODE 00227 * @brief sets the oversample mode, Normal, Low power and noise, High resolution, or low power 00228 */ 00229 enum OVERSAMPLE_MODE 00230 { 00231 OS_NORMAL = 0, 00232 OS_LO_PN, OS_HI_RES, OS_LO_POW 00233 }; 00234 00235 /** 00236 * @enum REGISTER 00237 * @brief The device register map 00238 */ 00239 enum REGISTER 00240 { 00241 STATUS = 0x00, 00242 OUT_X_MSB, OUT_X_LSB, OUT_Y_MSB, OUT_Y_LSB, OUT_Z_MSB, OUT_Z_LSB, 00243 00244 F_SETUP = 0x09, TRIG_CFG, // only available on the MMA8451 variant 00245 00246 SYSMOD = 0x0B, 00247 INT_SOURCE, WHO_AM_I, XYZ_DATA_CFG, HP_FILTER_CUTOFF, PL_STATUS, 00248 PL_CFG, PL_COUNT, PL_BF_ZCOMP, P_L_THS_REG, FF_MT_CFG, FF_MT_SRC, 00249 FF_MT_THS, FF_MT_COUNT, 00250 00251 TRANSIENT_CFG = 0x1D, 00252 TRANSIENT_SRC, TRANSIENT_THS, TRANSIENT_COUNT, PULSE_CFG, PULSE_SRC, 00253 PULSE_THSX, PULSE_THSY, PULSE_THSZ, PULSE_TMLT, PULSE_LTCY, PULSE_WIND, 00254 ASLP_COUNT, CTRL_REG1, CTRL_REG2, CTRL_REG3, CTRL_REG4, CTRL_REG5, 00255 OFF_X, OFF_Y, OFF_Z 00256 }; 00257 00258 /** Create the MMA845x object 00259 * @param i2c - A defined I2C object 00260 * @param int1 - A defined InterruptIn object pointer. Default NULL for polling mode 00261 * @param int2 - A defined InterruptIn object pointer. Default NULL for polling mode 00262 * @param i2c_addr - state of pin SA0 00263 * TODO - need to add interrupt support 00264 */ 00265 MMA845x(I2C &i2c, SA0 const i2c_addr = SA0_VSS , InterruptIn* int1 = NULL, InterruptIn* int2 = NULL); 00266 00267 /** Get the X data 00268 * @return The last valid X-axis reading from the accelerometer 00269 */ 00270 int16_t getX(void); 00271 00272 /** Get the Y data 00273 * @return The last valid Y-axis reading from the accelerometer 00274 */ 00275 int16_t getY(void); 00276 00277 /** Get the Z data 00278 * @return The last Z-axis valid reading from the accelerometer 00279 */ 00280 int16_t getZ(void); 00281 00282 /** Get the XYZ data structure 00283 * @return The last valid X, Y, and Z-axis readings from the accelerometer 00284 */ 00285 MMA845x_DATA getXYZ(void); 00286 00287 /** Get the XYZ data structure 00288 * @return accelerometer ID code. Test versus the WHO_AM_I_VAL enum 00289 */ 00290 char getWhoAmI(void) const; 00291 00292 /** Setup the MMA845x for standard accelerometer read mode 00293 * @range - set the measurement range using RANGE enum 00294 * @resolution - set the ADC resolution using the RESOLUTION enum 00295 * @lo_noise - Set the Low-Noise mode using the LOW_NOISE enum 00296 * @data_rate - set the aquisition rate using the DATA_RATE enum 00297 * @os_mode - Set the Over sample mode suing the OVERSAMPLE_MODE enum 00298 * @hpf_mode - Set the Hi pass filter mode using the HPF_MOSE enum 00299 * @return status of command 00300 * 00301 * This sets the resolution, range, data rate, oversample 00302 * mode, hi and lo pass filter. 00303 */ 00304 uint8_t setCommonParameters(RANGE range, RESOLUTION resolution, LOW_NOISE lo_noise, 00305 DATA_RATE data_rate, OVERSAMPLE_MODE os_mode, HPF_MODE hpf_mode ) const; 00306 00307 /** Ebnable Motion detect mode and interrupt handler 00308 * @return status of command 00309 * TODO - need to implement function 00310 */ 00311 uint8_t enableMotionDetect(void) const; 00312 00313 /** Enable Pulse Detect mode and interrupt handler 00314 * @return status of command 00315 * TODO - need to implement function 00316 */ 00317 uint8_t enablePulseDetect(void) const; 00318 00319 /** Enable Orientation mode and interrupt handler 00320 * @return status of command 00321 * TODO - need to implement function 00322 */ 00323 uint8_t enableOrientationDetect(void) const; 00324 00325 /** Enable Transient detect mode and interrupt handler 00326 * @return status of command 00327 * TODO - need to implement function 00328 */ 00329 uint8_t enableTransientDetect(void) const; 00330 00331 /** Enable Autosleep function and interrupt handler 00332 * @return status of command 00333 * TODO - need to implement function 00334 */ 00335 uint8_t enableAutoSleep(void) const; 00336 00337 /** Enbale FIFO function and interrupt handler 00338 * @return status of command 00339 * TODO - need to implement function 00340 */ 00341 uint8_t enableFIFO(void) const; 00342 00343 /** Put the MMA845x in the Standby mode 00344 * @return status of command 00345 */ 00346 uint8_t standbyMode(void) const; 00347 00348 /** Put the MMA845x in the active mode 00349 * @return status of command 00350 */ 00351 uint8_t activeMode(void) const; 00352 00353 /** Check the MMA845x status register 00354 * @return status byte 00355 */ 00356 uint8_t getStatus(void) const; 00357 00358 00359 private: 00360 00361 I2C *_i2c; 00362 InterruptIn *_int1; 00363 InterruptIn *_int2; 00364 uint8_t _i2c_addr; 00365 char _who_am_i; 00366 MMA845x_DATA _data; 00367 bool _polling_mode; 00368 00369 uint8_t init(void); 00370 00371 /** Write to a register (exposed for debugging reasons) 00372 * Note: most writes are only valid in stop mode 00373 * @param reg - The register to be written 00374 * @param data - The data to be written 00375 */ 00376 uint8_t writeRegister(uint8_t const reg, uint8_t const data) const; 00377 00378 /** Read from a register (exposed for debugging reasons) 00379 * @param reg - The register to read from 00380 * @return The register contents 00381 */ 00382 uint8_t readRegister(uint8_t const reg, uint8_t count, char* data) const; 00383 00384 00385 }; 00386 00387 #endif
Generated on Thu Jul 14 2022 17:24:22 by
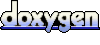