
MAX32620HSP (MAXREFDES100) RPC Example for Graphical User Interface
Dependencies: USBDevice
Fork of HSP_Release by
MAX14720 Class Reference
#include <MAX14720.h>
Public Types | |
enum | registers_t { REG_CHIP_ID = 0x00, REG_CHIP_REV = 0x01, REG_BOOST_CDIV = 0x03, REG_BOOST_ISET = 0x04, REG_BOOST_VSET = 0x05, REG_BOOST_CFG = 0x06, REG_BUCK_VSET = 0x07, REG_BUCK_CFG = 0x08, REG_BUCK_ISET = 0x09, REG_LDO_VSET = 0x0A, REG_LDO_CFG = 0x0B, REG_SWITCH_CFG = 0x0C, REG_BAT_TIME = 0x0D, REG_BAT_CFG = 0x0E, REG_BAT_BCV = 0x0F, REG_BAT_OCV = 0x10, REG_BAT_LCV = 0x11, REG_MON_CFG = 0x19, REG_BOOT_CFG = 0x1A, REG_PIN_STAT = 0x1B, REG_BBB_EXTRA = 0x1C, REG_HANDSHK = 0x1D, REG_UVLO_CFG = 0x1E, REG_PWR_OFF = 0x1F } |
Register Addresses. More... | |
enum | boostISet_t { BOOST_ISET_MIN, BOOST_ISET_50mA, BOOST_ISET_100mA, BOOST_ISET_150mA, BOOST_ISET_200mA, BOOST_ISET_250mA, BOOST_ISET_300mA, BOOST_ISET_350mA } |
Boost Peak Current Settings. More... | |
enum | boostEn_t { BOOST_DISABLED, BOOST_ENABLED, BOOST_EN_MPC } |
Boost Enable Mode. More... | |
enum | buckMd_t { BUCK_BURST, BUCK_FPWM, BUCK_MPC_FPWM } |
Buck Operating Modes. More... | |
enum | buckISet_t { BUCK_ISET_50mA, BUCK_ISET_100mA, BUCK_ISET_150mA, BUCK_ISET_200mA, BUCK_ISET_250mA, BUCK_ISET_300mA, BUCK_ISET_350mA, BUCK_ISET_400mA } |
Buck Peak Current Settings. More... | |
enum | monCfg_t { MON_PULLDOWN = 0x00, MON_HI_Z = 0x08, MON_SWIN = 0x80, MON_SWOUT = 0x81, MON_BIN = 0x82, MON_BOUT = 0x83, MON_HVIN = 0x84, MON_HVOUT = 0x85, MON_LIN = 0x86, MON_LOUT = 0x87 } |
Monitor Configurations. More... | |
enum | uvloIn_t { LIN_UVLO, BIN_UVLO } |
Under-Voltage Lock Out Input. More... | |
Public Member Functions | |
MAX14720 (PinName sda, PinName scl, int slaveAddress) | |
MAX14720 constructor. | |
MAX14720 (I2C *i2c, int slaveAddress) | |
MAX14720 constructor. | |
~MAX14720 () | |
MAX14720 destructor. | |
int | init () |
Initialize MAX14720. | |
int | boostSetVoltage (int mV) |
Set the Boost Voltage. | |
int | boostSetMode (boostEn_t mode) |
Set Boost Enable Mode. | |
int | monSet (monCfg_t monCfg) |
Configure Mon Pin. | |
int | shutdown () |
Shutdown. | |
int | writeReg (registers_t reg, char value) |
Write Register. | |
int | readReg (registers_t reg, char *value) |
Read Register. | |
Data Fields | |
int | clkDivSet |
Boost Clock Divider Enable. | |
boostISet_t | boostISet |
Boost Clock Divider Setting. | |
int | boostMillivolts |
Boost Peak Current Setting. | |
boostEn_t | boostEn |
Boost Voltage in millivolts. | |
bool | boostEMI |
Boost Enable Mode. | |
bool | boostInd |
Boost EMI Setting. | |
bool | boostHysOff |
Boost Inductor Setting. | |
bool | boostPasDsc |
Boost Hysteresis Off. | |
bool | boostActDsc |
Boost Passive Discharge. | |
buckMd_t | buckMd |
Boost Active Discharge. | |
bool | buckFst |
Buck Operating Mode. | |
buckISet_t | buckISet |
Buck Fast Start. | |
bool | buckCfg |
Buck Peak Current Setting. | |
bool | buckInd |
Buck Configuration (Set to 1 when using FPWM mode) | |
bool | buckHysOff |
Buck Inductor Setting. | |
bool | buckMinOT |
Buck Hysteresis Off. | |
bool | buckInteg |
Buck Minimum On Time. | |
bool | buckPasDsc |
Buck Integrate. | |
bool | buckActDsc |
Buck Passive Discharge. | |
bool | buckFScl |
Buck Active Discharge. |
Detailed Description
MAX14720 PMIC Driver.
#include "mbed.h" #include "MAX14720.h" // I2C Master 2 I2C i2c2(I2C2_SDA, I2C2_SCL); #define I2C_ADDR_PMIC (0x54) MAX14720 max14720(&i2c2,I2C_ADDR_PMIC); DigitalOut led(LED1); InterruptIn button(SW1); void turnOff() { max14720.shutdown(); } int main() { int result; button.fall(&turnOff); led = 0; max14720.boostEn = MAX14720::BOOST_ENABLED; result = max14720.init(); if (result == MAX14720_ERROR) printf("Error initializing MAX14720"); wait(1); while(1) { max14720.boostSetMode(MAX14720::BOOST_DISABLED); max14720.boostEn = MAX14720::BOOST_ENABLED; wait(0.5); max14720.boostSetVoltage(2500); wait(0.5); max14720.boostSetVoltage(5000); wait(0.5); } }
Definition at line 88 of file MAX14720.h.
Member Enumeration Documentation
enum boostEn_t |
Boost Enable Mode.
Enumerated enable modes for boost regulator
- Enumerator:
BOOST_DISABLED Boost Disabled.
BOOST_ENABLED Boost Enabled.
BOOST_EN_MPC Boost Enabled by MPC pin.
Definition at line 141 of file MAX14720.h.
enum boostISet_t |
Boost Peak Current Settings.
Enumerated peak current settings for boost regulator
- Enumerator:
Definition at line 126 of file MAX14720.h.
enum buckISet_t |
Buck Peak Current Settings.
Enumerated peak current settings for buck regulator
- Enumerator:
Definition at line 161 of file MAX14720.h.
enum buckMd_t |
Buck Operating Modes.
Enumerated operating modes for buck regulator
- Enumerator:
BUCK_BURST Burst Mode Operation.
BUCK_FPWM Forced PWM Operation.
BUCK_MPC_FPWM MPC activated Forced PWM.
Definition at line 151 of file MAX14720.h.
enum monCfg_t |
Monitor Configurations.
Enumerated configuration modes for monitor multiplexer
- Enumerator:
Definition at line 176 of file MAX14720.h.
enum registers_t |
Register Addresses.
Enumerated MAX14720 register addresses
- Enumerator:
Definition at line 95 of file MAX14720.h.
enum uvloIn_t |
Under-Voltage Lock Out Input.
Enumerated input selection options for UVLO
- Enumerator:
LIN_UVLO LIN used to determine UVLO condition.
BIN_UVLO BIN used to determine UVLO condition.
Definition at line 193 of file MAX14720.h.
Constructor & Destructor Documentation
MAX14720 | ( | PinName | sda, |
PinName | scl, | ||
int | slaveAddress | ||
) |
MAX14720 constructor.
- Parameters:
-
sda mbed pin to use for SDA line of I2C interface. scl mbed pin to use for SCL line of I2C interface. slaveAddress Slave Address of the device.
Definition at line 37 of file MAX14720.cpp.
MAX14720 | ( | I2C * | i2c, |
int | slaveAddress | ||
) |
MAX14720 constructor.
- Parameters:
-
i2c I2C object to use. slaveAddress Slave Address of the device.
Definition at line 64 of file MAX14720.cpp.
~MAX14720 | ( | ) |
MAX14720 destructor.
Definition at line 90 of file MAX14720.cpp.
Member Function Documentation
int boostSetMode | ( | boostEn_t | mode ) |
Set Boost Enable Mode.
Sets the enable mode for the boost regulator
- Parameters:
-
mode The enable mode for the boost regulator
- Returns:
- 0 if no errors, -1 if error.
Definition at line 97 of file MAX14720.cpp.
int boostSetVoltage | ( | int | mV ) |
Set the Boost Voltage.
Sets the voltage for the boost regulator. The voltage is specified in millivoltst. The MAX14720 cannot update the voltage when enabled. This function checks the local boostEn variable and if the regualtor is enabled it will send the disable command before sending the new voltage and re-enable the boost regulator after the new voltage is written.
- Parameters:
-
mV voltage for boost regualtor in millivolts
- Returns:
- 0 if no errors, -1 if error.
Definition at line 110 of file MAX14720.cpp.
int init | ( | void | ) |
Initialize MAX14720.
Applies settings to MAX14720. Settings are stored in public variables. The variables are pre-loaded with the most common configuation. Assign new values to the public variables before calling init.
- Returns:
- 0 if no errors, -1 if error.
Definition at line 141 of file MAX14720.cpp.
int monSet | ( | monCfg_t | monCfg ) |
Configure Mon Pin.
Configures the operating mode of the monitor multiplexer
- Parameters:
-
monCfg The configuration mode for the monitor pin
- Returns:
- 0 if no errors, -1 if error.
Definition at line 185 of file MAX14720.cpp.
int readReg | ( | registers_t | reg, |
char * | value | ||
) |
Read Register.
Reads from the specified register
- Parameters:
-
reg The register to be read value Pointer for where to store the data
- Returns:
- 0 if no errors, -1 if error.
Definition at line 216 of file MAX14720.cpp.
int shutdown | ( | ) |
Shutdown.
Sends the command to turn off all supplies and put the part in battery saving shelf mode.
- Returns:
- 0 if no errors, -1 if error.
Definition at line 195 of file MAX14720.cpp.
int writeReg | ( | registers_t | reg, |
char | value | ||
) |
Write Register.
Writes the given value to the specified register
- Parameters:
-
reg The register to be written value The data to be written
- Returns:
- 0 if no errors, -1 if error.
Definition at line 205 of file MAX14720.cpp.
Field Documentation
bool boostActDsc |
Boost Passive Discharge.
Definition at line 291 of file MAX14720.h.
bool boostEMI |
Boost Enable Mode.
Definition at line 291 of file MAX14720.h.
Boost Voltage in millivolts.
Definition at line 290 of file MAX14720.h.
bool boostHysOff |
Boost Inductor Setting.
Definition at line 291 of file MAX14720.h.
bool boostInd |
Boost EMI Setting.
Definition at line 291 of file MAX14720.h.
Boost Clock Divider Setting.
Definition at line 288 of file MAX14720.h.
int boostMillivolts |
Boost Peak Current Setting.
Definition at line 289 of file MAX14720.h.
bool boostPasDsc |
Boost Hysteresis Off.
Definition at line 291 of file MAX14720.h.
bool buckActDsc |
Buck Passive Discharge.
Definition at line 299 of file MAX14720.h.
bool buckCfg |
Buck Peak Current Setting.
Definition at line 299 of file MAX14720.h.
bool buckFScl |
Buck Active Discharge.
Definition at line 299 of file MAX14720.h.
bool buckFst |
Buck Operating Mode.
Definition at line 297 of file MAX14720.h.
bool buckHysOff |
Buck Inductor Setting.
Definition at line 299 of file MAX14720.h.
bool buckInd |
Buck Configuration (Set to 1 when using FPWM mode)
Definition at line 299 of file MAX14720.h.
bool buckInteg |
Buck Minimum On Time.
Definition at line 299 of file MAX14720.h.
Buck Fast Start.
Definition at line 298 of file MAX14720.h.
Boost Active Discharge.
Definition at line 296 of file MAX14720.h.
bool buckMinOT |
Buck Hysteresis Off.
Definition at line 299 of file MAX14720.h.
bool buckPasDsc |
Buck Integrate.
Definition at line 299 of file MAX14720.h.
int clkDivSet |
Boost Clock Divider Enable.
Definition at line 287 of file MAX14720.h.
Generated on Tue Jul 12 2022 17:59:20 by
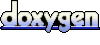