
MAX32620HSP (MAXREFDES100) RPC Example for Graphical User Interface
Dependencies: USBDevice
Fork of HSP_Release by
MAX14720.cpp
00001 /******************************************************************************* 00002 * Copyright (C) 2016 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 ******************************************************************************* 00032 */ 00033 00034 #include "MAX14720.h" 00035 00036 //****************************************************************************** 00037 MAX14720::MAX14720(PinName sda, PinName scl, int slaveAddress) : 00038 slaveAddress(slaveAddress) { 00039 i2c = new I2C(sda, scl); 00040 isOwner = true; 00041 clkDivEn = false; 00042 clkDivSet = 0; 00043 boostISet = BOOST_ISET_100mA; 00044 boostMillivolts = 3300; 00045 boostEn = BOOST_DISABLED; 00046 boostEMI = false; 00047 boostInd = false; 00048 boostHysOff = false; 00049 boostPasDsc = false; 00050 boostActDsc = false; 00051 buckMd = BUCK_BURST; 00052 buckFst = false; 00053 buckISet = BUCK_ISET_300mA; 00054 buckCfg = false; 00055 buckInd = false; 00056 buckHysOff = true; 00057 buckMinOT = true; 00058 buckInteg = true; 00059 buckPasDsc = false; 00060 buckActDsc = false; 00061 buckFScl = false; 00062 } 00063 //****************************************************************************** 00064 MAX14720::MAX14720(I2C *i2c, int slaveAddress) : slaveAddress(slaveAddress) { 00065 this->i2c = i2c; 00066 isOwner = false; 00067 clkDivEn = false; 00068 clkDivSet = 0; 00069 boostISet = BOOST_ISET_100mA; 00070 boostMillivolts = 3300; 00071 boostEn = BOOST_DISABLED; 00072 boostEMI = false; 00073 boostInd = false; 00074 boostHysOff = false; 00075 boostPasDsc = false; 00076 boostActDsc = false; 00077 buckMd = BUCK_BURST; 00078 buckFst = false; 00079 buckISet = BUCK_ISET_300mA; 00080 buckCfg = false; 00081 buckInd = false; 00082 buckHysOff = true; 00083 buckMinOT = true; 00084 buckInteg = true; 00085 buckPasDsc = false; 00086 buckActDsc = false; 00087 buckFScl = false; 00088 } 00089 //****************************************************************************** 00090 MAX14720::~MAX14720() { 00091 if (isOwner == true) { 00092 delete i2c; 00093 } 00094 } 00095 00096 //****************************************************************************** 00097 int MAX14720::boostSetMode(boostEn_t mode) { 00098 int result; 00099 char data; 00100 boostEn = mode; 00101 data = (boostEn << 3) | (boostEMI << 1) | (boostInd); 00102 result = writeReg(REG_BOOST_CFG, data); 00103 if (result == MAX14720_ERROR) { 00104 return result; 00105 } 00106 return 0; 00107 } 00108 00109 //****************************************************************************** 00110 int MAX14720::boostSetVoltage(int mV) { 00111 int result; 00112 char data; 00113 result = MAX14720_NO_ERROR; 00114 if ((MAX14720_BOOST_MIN_MV <= mV) && (mV <= MAX14720_BOOST_MAX_MV)) { 00115 boostMillivolts = mV; 00116 data = (mV - MAX14720_BOOST_MIN_MV) / MAX14720_BOOST_STEP_MV; 00117 } else { 00118 return MAX14720_ERROR; 00119 } 00120 if (boostEn == BOOST_ENABLED) { 00121 result = writeReg(REG_BOOST_CFG, 0x00); 00122 } 00123 if (result == MAX14720_ERROR) { 00124 return result; 00125 } 00126 result = writeReg(REG_BOOST_VSET, data); 00127 if (result == MAX14720_ERROR) { 00128 return result; 00129 } 00130 if (boostEn == BOOST_ENABLED) { 00131 data = (boostEn << 3) | (boostEMI << 1) | (boostInd); 00132 result = writeReg(REG_BOOST_CFG, data); 00133 } 00134 if (result == MAX14720_ERROR) { 00135 return result; 00136 } 00137 return 0; 00138 } 00139 00140 //****************************************************************************** 00141 int MAX14720::init() { 00142 int result; 00143 char data; 00144 data = (clkDivEn << 7) | (clkDivSet); 00145 result = writeReg(REG_BOOST_CDIV, data); 00146 if (result == MAX14720_ERROR) { 00147 return result; 00148 } 00149 data = (boostISet); 00150 result = writeReg(REG_BOOST_ISET, data); 00151 if (result == MAX14720_ERROR) { 00152 return result; 00153 } 00154 if ((MAX14720_BOOST_MIN_MV <= boostMillivolts) && 00155 (boostMillivolts <= MAX14720_BOOST_MAX_MV)) { 00156 data = (boostMillivolts - MAX14720_BOOST_MIN_MV) / MAX14720_BOOST_STEP_MV; 00157 } else { 00158 return MAX14720_ERROR; 00159 } 00160 result = writeReg(REG_BOOST_VSET, data); 00161 if (result == MAX14720_ERROR) { 00162 return result; 00163 } 00164 data = (buckMd << 1) | (buckFst); 00165 result = writeReg(REG_BUCK_CFG, data); 00166 if (result == MAX14720_ERROR) { 00167 return result; 00168 } 00169 data = (boostHysOff << 7) | (boostPasDsc << 6) | (boostActDsc << 5) | 00170 (buckPasDsc << 2) | (buckActDsc << 1) | (buckFScl); 00171 result = writeReg(REG_BBB_EXTRA, data); 00172 if (result == MAX14720_ERROR) { 00173 return result; 00174 } 00175 // Write Boost Enable Register Last 00176 data = (boostEn << 3) | (boostEMI << 1) | (boostInd); 00177 result = writeReg(REG_BOOST_CFG, data); 00178 if (result == MAX14720_ERROR) { 00179 return result; 00180 } 00181 return 0; 00182 } 00183 00184 //****************************************************************************** 00185 int MAX14720::monSet(monCfg_t monCfg) { 00186 int result; 00187 result = writeReg(REG_MON_CFG, monCfg); 00188 if (result == MAX14720_ERROR) { 00189 return result; 00190 } 00191 return 0; 00192 } 00193 00194 //****************************************************************************** 00195 int MAX14720::shutdown() { 00196 int result; 00197 result = writeReg(REG_PWR_OFF, 0xB2); 00198 if (result == MAX14720_ERROR) { 00199 return result; 00200 } 00201 return 0; 00202 } 00203 00204 //****************************************************************************** 00205 int MAX14720::writeReg(registers_t reg, char value) { 00206 int result; 00207 char cmdData[2] = {(char)reg, value}; 00208 result = i2c->write(slaveAddress, cmdData, 2); 00209 if (result != 0) { 00210 return MAX14720_ERROR; 00211 } 00212 return MAX14720_NO_ERROR; 00213 } 00214 00215 //****************************************************************************** 00216 int MAX14720::readReg(registers_t reg, char *value) { 00217 int result; 00218 char cmdData[1] = {(char)reg}; 00219 00220 result = i2c->write(slaveAddress, cmdData, 1); 00221 if (result != 0) { 00222 return MAX14720_ERROR; 00223 } 00224 result = i2c->read(slaveAddress, value, 1); 00225 if (result != 0) { 00226 return MAX14720_ERROR; 00227 } 00228 return MAX14720_NO_ERROR; 00229 }
Generated on Tue Jul 12 2022 17:59:19 by
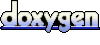