
MAX32620HSP (MAXREFDES100) RPC Example for Graphical User Interface
Dependencies: USBDevice
Fork of HSP_Release by
MAX14720.h
00001 /******************************************************************************* 00002 * Copyright (C) 2016 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 ******************************************************************************* 00032 */ 00033 00034 #ifndef _MAX14720_H_ 00035 #define _MAX14720_H_ 00036 00037 #include "mbed.h" 00038 00039 #define MAX14720_NO_ERROR 0 00040 #define MAX14720_ERROR -1 00041 00042 #define MAX14720_BOOST_MIN_MV 2500 00043 #define MAX14720_BOOST_MAX_MV 5000 00044 #define MAX14720_BOOST_STEP_MV 100 00045 00046 /** 00047 * MAX14720 PMIC Driver 00048 * 00049 * @code 00050 * #include "mbed.h" 00051 * #include "MAX14720.h" 00052 * 00053 * // I2C Master 2 00054 * I2C i2c2(I2C2_SDA, I2C2_SCL); 00055 * 00056 * #define I2C_ADDR_PMIC (0x54) 00057 * MAX14720 max14720(&i2c2,I2C_ADDR_PMIC); 00058 * 00059 * DigitalOut led(LED1); 00060 * InterruptIn button(SW1); 00061 * 00062 * void turnOff() 00063 * { 00064 * max14720.shutdown(); 00065 * } 00066 * 00067 * int main() 00068 * { 00069 * int result; 00070 * button.fall(&turnOff); 00071 * led = 0; 00072 * max14720.boostEn = MAX14720::BOOST_ENABLED; 00073 * result = max14720.init(); 00074 * if (result == MAX14720_ERROR) printf("Error initializing MAX14720"); 00075 * wait(1); 00076 * while(1) { 00077 * max14720.boostSetMode(MAX14720::BOOST_DISABLED); 00078 * max14720.boostEn = MAX14720::BOOST_ENABLED; 00079 * wait(0.5); 00080 * max14720.boostSetVoltage(2500); 00081 * wait(0.5); 00082 * max14720.boostSetVoltage(5000); 00083 * wait(0.5); 00084 * } 00085 * } 00086 * @endcode 00087 */ 00088 class MAX14720 { 00089 00090 public: 00091 /** 00092 *@brief Register Addresses 00093 *@details Enumerated MAX14720 register addresses 00094 */ 00095 typedef enum { 00096 REG_CHIP_ID = 0x00, ///< Chip ID 00097 REG_CHIP_REV = 0x01, ///< Chip Revision 00098 REG_BOOST_CDIV = 0x03, ///< Boost Clock Divider 00099 REG_BOOST_ISET = 0x04, ///< Boost Peak Current 00100 REG_BOOST_VSET = 0x05, ///< Boost Voltage Setting 00101 REG_BOOST_CFG = 0x06, ///< Boost Configuration 00102 REG_BUCK_VSET = 0x07, ///< Buck Voltage Setting 00103 REG_BUCK_CFG = 0x08, ///< Buck Configuration 00104 REG_BUCK_ISET = 0x09, ///< Buck Peak Current and Settings 00105 REG_LDO_VSET = 0x0A, ///< LDO Voltage Setting 00106 REG_LDO_CFG = 0x0B, ///< LDO Configuration 00107 REG_SWITCH_CFG = 0x0C, ///< Switch Configuration 00108 REG_BAT_TIME = 0x0D, ///< Battery Impedance Timing 00109 REG_BAT_CFG = 0x0E, ///< Battery Impedance Configuration 00110 REG_BAT_BCV = 0x0F, ///< Battery Cell Voltage 00111 REG_BAT_OCV = 0x10, ///< Open Cell Voltage 00112 REG_BAT_LCV = 0x11, ///< Loaded Cell Voltage 00113 REG_MON_CFG = 0x19, ///< Monitor Multiplexer Configuration 00114 REG_BOOT_CFG = 0x1A, ///< Boot Configuration 00115 REG_PIN_STAT = 0x1B, ///< Pin Status 00116 REG_BBB_EXTRA = 0x1C, ///< Buck/Buck-Boost Extra 00117 REG_HANDSHK = 0x1D, ///< Power-On Handshake 00118 REG_UVLO_CFG = 0x1E, ///< Under-Voltage Lock Out 00119 REG_PWR_OFF = 0x1F, ///< Power Off Command 00120 } registers_t; 00121 00122 /** 00123 *@brief Boost Peak Current Settings 00124 *@details Enumerated peak current settings for boost regulator 00125 */ 00126 typedef enum { 00127 BOOST_ISET_MIN, ///< Minimum On-Time 00128 BOOST_ISET_50mA, ///< 50mA Peak Current 00129 BOOST_ISET_100mA, ///< 100mA Peak Current 00130 BOOST_ISET_150mA, ///< 150mA Peak Current 00131 BOOST_ISET_200mA, ///< 200mA Peak Current 00132 BOOST_ISET_250mA, ///< 250mA Peak Current 00133 BOOST_ISET_300mA, ///< 300mA Peak Current 00134 BOOST_ISET_350mA, ///< 350mA Peak Current 00135 } boostISet_t; 00136 00137 /** 00138 *@brief Boost Enable Mode 00139 *@details Enumerated enable modes for boost regulator 00140 */ 00141 typedef enum { 00142 BOOST_DISABLED, ///< Boost Disabled 00143 BOOST_ENABLED, ///< Boost Enabled 00144 BOOST_EN_MPC, ///< Boost Enabled by MPC pin 00145 } boostEn_t; 00146 00147 /** 00148 *@brief Buck Operating Modes 00149 *@details Enumerated operating modes for buck regulator 00150 */ 00151 typedef enum { 00152 BUCK_BURST, ///< Burst Mode Operation 00153 BUCK_FPWM, ///< Forced PWM Operation 00154 BUCK_MPC_FPWM, ///< MPC activated Forced PWM 00155 } buckMd_t; 00156 00157 /** 00158 *@brief Buck Peak Current Settings 00159 *@details Enumerated peak current settings for buck regulator 00160 */ 00161 typedef enum { 00162 BUCK_ISET_50mA, ///< 50mA Peak Current 00163 BUCK_ISET_100mA, ///< 100mA Peak Current 00164 BUCK_ISET_150mA, ///< 150mA Peak Current 00165 BUCK_ISET_200mA, ///< 200mA Peak Current 00166 BUCK_ISET_250mA, ///< 250mA Peak Current 00167 BUCK_ISET_300mA, ///< 300mA Peak Current 00168 BUCK_ISET_350mA, ///< 350mA Peak Current 00169 BUCK_ISET_400mA, ///< 400mA Peak Current 00170 } buckISet_t; 00171 00172 /** 00173 *@brief Monitor Configurations 00174 *@details Enumerated configuration modes for monitor multiplexer 00175 */ 00176 typedef enum { 00177 MON_PULLDOWN = 0x00, ///< Pulled down by 100k Ohm 00178 MON_HI_Z = 0x08, ///< High Impedance 00179 MON_SWIN = 0x80, ///< SWIN Selected 00180 MON_SWOUT = 0x81, ///< SWOUT Selected 00181 MON_BIN = 0x82, ///< BIN Selected 00182 MON_BOUT = 0x83, ///< BOUT Selected 00183 MON_HVIN = 0x84, ///< HVIN Selected 00184 MON_HVOUT = 0x85, ///< HVOUT Selected 00185 MON_LIN = 0x86, ///< LIN Selected 00186 MON_LOUT = 0x87, ///< LOUT Selected 00187 } monCfg_t; 00188 00189 /** 00190 *@brief Under-Voltage Lock Out Input 00191 *@details Enumerated input selection options for UVLO 00192 */ 00193 typedef enum { 00194 LIN_UVLO, ///< LIN used to determine UVLO condition 00195 BIN_UVLO, ///< BIN used to determine UVLO condition 00196 } uvloIn_t; 00197 00198 /** 00199 * @brief MAX14720 constructor. 00200 * 00201 * @param sda mbed pin to use for SDA line of I2C interface. 00202 * @param scl mbed pin to use for SCL line of I2C interface. 00203 * @param slaveAddress Slave Address of the device. 00204 */ 00205 MAX14720(PinName sda, PinName scl, int slaveAddress); 00206 00207 /** 00208 * @brief MAX14720 constructor. 00209 * 00210 * @param i2c I2C object to use. 00211 * @param slaveAddress Slave Address of the device. 00212 */ 00213 MAX14720(I2C *i2c, int slaveAddress); 00214 00215 /** 00216 * @brief MAX14720 destructor. 00217 */ 00218 ~MAX14720(); 00219 00220 /** 00221 * @brief Initialize MAX14720 00222 * @details Applies settings to MAX14720. 00223 * Settings are stored in public variables. 00224 * The variables are pre-loaded with the most common configuation. 00225 * Assign new values to the public variables before calling init. 00226 * @returns 0 if no errors, -1 if error. 00227 */ 00228 int init(); 00229 00230 /** 00231 * @brief Set the Boost Voltage 00232 * @details Sets the voltage for the boost regulator. 00233 * The voltage is specified in millivoltst. 00234 * The MAX14720 cannot update the voltage when enabled. 00235 * This function checks the local boostEn variable and if the 00236 * regualtor is enabled it will send the disable command before 00237 * sending the new voltage and re-enable the boost regulator after 00238 * the new voltage is written. 00239 * @param mV voltage for boost regualtor in millivolts 00240 * @returns 0 if no errors, -1 if error. 00241 */ 00242 int boostSetVoltage(int mV); 00243 00244 /** 00245 * @brief Set Boost Enable Mode 00246 * @details Sets the enable mode for the boost regulator 00247 * @param mode The enable mode for the boost regulator 00248 * @returns 0 if no errors, -1 if error. 00249 */ 00250 int boostSetMode(boostEn_t mode); 00251 00252 /** 00253 * @brief Configure Mon Pin 00254 * @details Configures the operating mode of the monitor multiplexer 00255 * @param monCfg The configuration mode for the monitor pin 00256 * @returns 0 if no errors, -1 if error. 00257 */ 00258 int monSet(monCfg_t monCfg); 00259 00260 /** 00261 * @brief Shutdown 00262 * @details Sends the command to turn off all supplies and put the part 00263 * in battery saving shelf mode. 00264 * @returns 0 if no errors, -1 if error. 00265 */ 00266 int shutdown(); 00267 00268 /** 00269 * @brief Write Register 00270 * @details Writes the given value to the specified register 00271 * @param reg The register to be written 00272 * @param value The data to be written 00273 * @returns 0 if no errors, -1 if error. 00274 */ 00275 int writeReg(registers_t reg, char value); 00276 00277 /** 00278 * @brief Read Register 00279 * @details Reads from the specified register 00280 * @param reg The register to be read 00281 * @param value Pointer for where to store the data 00282 * @returns 0 if no errors, -1 if error. 00283 */ 00284 int readReg(registers_t reg, char *value); 00285 00286 bool clkDivEn; /// Boost Clock Divider Enable 00287 int clkDivSet; /// Boost Clock Divider Setting 00288 boostISet_t boostISet; /// Boost Peak Current Setting 00289 int boostMillivolts; /// Boost Voltage in millivolts 00290 boostEn_t boostEn; /// Boost Enable Mode 00291 bool boostEMI, /// Boost EMI Setting 00292 boostInd, /// Boost Inductor Setting 00293 boostHysOff, /// Boost Hysteresis Off 00294 boostPasDsc, /// Boost Passive Discharge 00295 boostActDsc; /// Boost Active Discharge 00296 buckMd_t buckMd; /// Buck Operating Mode 00297 bool buckFst; /// Buck Fast Start 00298 buckISet_t buckISet; /// Buck Peak Current Setting 00299 bool buckCfg, /// Buck Configuration (Set to 1 when using FPWM mode) 00300 buckInd, /// Buck Inductor Setting 00301 buckHysOff, /// Buck Hysteresis Off 00302 buckMinOT, /// Buck Minimum On Time 00303 buckInteg, /// Buck Integrate 00304 buckPasDsc, /// Buck Passive Discharge 00305 buckActDsc, /// Buck Active Discharge 00306 buckFScl; /// Buck Fet Scaling 00307 00308 private: 00309 /// @brief I2C pointer 00310 I2C *i2c; 00311 /// @brief Is this object the owner of the I2C object 00312 bool isOwner; 00313 /// @brief Device slave address 00314 int slaveAddress; 00315 }; 00316 00317 #endif /* _MAX14720_H_ */
Generated on Tue Jul 12 2022 17:59:19 by
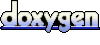