
MAX32620HSP (MAXREFDES100) RPC Example for Graphical User Interface
Dependencies: USBDevice
Fork of HSP_Release by
Peripherals.h
00001 /******************************************************************************* 00002 * Copyright (C) 2016 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 ******************************************************************************* 00032 */ 00033 #ifndef _PERIPHERALS_H_ 00034 #define _PERIPHERALS_H_ 00035 00036 #include "mbed.h" 00037 //#include "USBSerial.h" 00038 #include "MAX30101.h" 00039 #include "HspLed.h" 00040 #include "MAX30205.h" 00041 #include "LIS2DH.h" 00042 #include "MAX30001.h" 00043 #include "BMP280.h" 00044 #include "S25FS512.h" 00045 #include "PushButton.h" 00046 #include "BLE.h" 00047 #include "MAX14720.h" 00048 #include "HspBLE.h" 00049 00050 /** 00051 * This static class is used as a central locatoin for all devices on the HSP platform 00052 * it gives (in-effect) a singleton interface for each device so that anywhere in code 00053 * one can reference on of these devices 00054 */ 00055 class Peripherals { 00056 public: 00057 //static USBSerial *setUSBSerial(USBSerial * device) { mUSBSerial = device; return device; } 00058 //static USBSerial *usbSerial(void) { return mUSBSerial; } 00059 00060 static MAX30101 *max30101(void) { return mMAX30101; } 00061 static MAX30101 *setMAX30101(MAX30101 *device) { mMAX30101 = device; return device; } 00062 00063 static MAX30205 *max30205_top(void) { return mMAX30205_top; } 00064 static MAX30205 *setMAX30205_top(MAX30205 *device) { mMAX30205_top = device; return device; } 00065 00066 static MAX30205 *max30205_bottom(void) { return mMAX30205_bottom; } 00067 static MAX30205 *setMAX30205_bottom(MAX30205 *device) { mMAX30205_bottom = device; return device; } 00068 00069 static HspLed *hspLed(void) { return mHspLed; } 00070 static HspLed *setHspLed(HspLed *device) { mHspLed = device; return device; } 00071 00072 static LIS2DH *lis2dh(void) { return mLIS2DH; } 00073 static LIS2DH *setLIS2DH(LIS2DH *device) { mLIS2DH = device; return device; } 00074 00075 static MAX30001 *max30001(void) { return mMAX30001; } 00076 static MAX30001 *setMAX30001(MAX30001 *device) { mMAX30001 = device; return device; } 00077 00078 static BMP280 *bmp280(void) { return mBMP280; } 00079 static BMP280 *setBMP280(BMP280 *device) { mBMP280 = device; return device; } 00080 00081 static Timer *timestampTimer(void) { return mTimestampTimer; } 00082 static Timer *setTimestampTimer(Timer *timer) { mTimestampTimer = timer; return timer; } 00083 00084 static I2C *i2c1(void) { return mI2c1; } 00085 static I2C *setI2c1(I2C *i2cPort) { mI2c1 = i2cPort; return i2cPort; } 00086 00087 static I2C *i2c2(void) { return mI2c2; } 00088 static I2C *setI2c2(I2C *i2cPort) { mI2c2 = i2cPort; return i2cPort; } 00089 00090 static S25FS512 *s25FS512(void) { return mS25FS512; } 00091 static S25FS512 *setS25FS512(S25FS512 *s25FS512) { mS25FS512 = s25FS512; return s25FS512; } 00092 00093 static PushButton *pushButton(void) { return mPushButton; } 00094 static PushButton *setPushButton(PushButton *pushButton) { mPushButton = pushButton; return pushButton; } 00095 00096 static BLE *ble(void) { return mBLE; } 00097 static BLE *setBLE(BLE *_ble) { mBLE = _ble; return _ble; } 00098 00099 static HspBLE *hspBLE(void) { return mhspBLE; } 00100 static HspBLE *setHspBLE(HspBLE *_hspBLE) { mhspBLE = _hspBLE; return _hspBLE; } 00101 00102 static MAX14720 *max14720(void) { return mMAX14720; } 00103 static MAX14720 *setMAX14720(MAX14720 *_MAX14720) { mMAX14720 = _MAX14720; return _MAX14720; } 00104 00105 private: 00106 static I2C *mI2c1; 00107 static I2C *mI2c2; 00108 //static USBSerial *mUSBSerial; 00109 static MAX30101 *mMAX30101; 00110 static MAX30001 *mMAX30001; 00111 static BMP280 *mBMP280; 00112 static HspLed *mHspLed; 00113 static Serial *mSerial; 00114 static MAX30205 *mMAX30205_top; 00115 static MAX30205 *mMAX30205_bottom; 00116 static LIS2DH *mLIS2DH; 00117 static Timer *mTimestampTimer; 00118 static S25FS512 *mS25FS512; 00119 static PushButton *mPushButton; 00120 static BLE *mBLE; 00121 static MAX14720 *mMAX14720; 00122 static HspBLE *mhspBLE; 00123 }; 00124 00125 #endif // _PERIPHERALS_H_ 00126
Generated on Tue Jul 12 2022 17:59:19 by
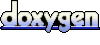