
MAX32620HSP (MAXREFDES100) RPC Example for Graphical User Interface
Dependencies: USBDevice
Fork of HSP_Release by
BMP280 Class Reference
Bosch BMP280 Digital Pressure Sensor. More...
#include <BMP280.h>
Public Types | |
enum | BMP280_REG_map_t { BMP280_READID = 0x58 , BMP280_ID = 0xD0, BMP280_CALIB25 = 0xA1, BMP280_CALIB00 = 0x88 } |
typedef union BMP280::bmp280_config_reg | bmp280_config_t |
CTRL_MEAS (0xF4) | |
typedef union BMP280::bmp280_ctrl_meas | bmp280_ctrl_meas_t |
STATUS (0xF3) | |
typedef union BMP280::bmp280_status | bmp280_status_t |
RESET (0xE0) | |
Public Member Functions | |
BMP280 (PinName sda, PinName scl, int slaveAddress) | |
BMP280 constructor. | |
BMP280 (I2C *i2c, int slaveAddress) | |
BMP280 constructor. | |
int | writeReg (char reg, char value) |
Write a device register. | |
int | readReg (char reg, char *value) |
Read a device register. | |
BMP280 (PinName sda, PinName scl) | |
BMP280 constructor. | |
BMP280 (I2C *i2c) | |
BMP280 constructor. | |
~BMP280 (void) | |
BMP280 destructor. | |
int | init (bmp280_osrs_P_t Osrs_p, bmp280_osrs_T_t Osrs_t, bmp280_FILT_t Filter, bmp280_MODE_t Mode, bmp280_TSB_t T_sb) |
This initializes the BMP280. | |
int | ReadCompData (float *Temp_degC, float *Press_Pa) |
The BMP280 has 2 modes. | |
int | reg_write (BMP280_REG_map_t reg, char value) |
This function allows writing to a register. | |
int | reg_read (BMP280_REG_map_t reg, char *value, char number) |
This function allows writing to a register. | |
void | Reset (void) |
Performs a soft reset on the BMP280. | |
int | Detect (void) |
Detects if the BMP280 is present. | |
float | compensate_T_float (int32_t adc_T) |
Performs calculations on the raw temperature data to convert to. | |
float | compensate_P_float (int32_t adc_P) |
Performs calculations on the raw pressure data to convert to. | |
int | Sleep (void) |
Puts the BMP280 in low power Sleep mode. | |
int | ReadCompDataRaw (char *bmp280_rawData) |
This reads the raw BMP280 data. | |
int | ReadCompDataRaw2 (char *bmp280_rawData) |
This reads the raw BMP280 data uses the Bosch algorithm to get the data. | |
void | ToFloat (char *bmp280_rawData, float *Temp_degC, float *Press_Pa) |
This converts the raw BMP280 data to couble based on Bosch's algorithm. | |
float | ToFahrenheit (float temperature) |
converts to Farenhite from Centigrade | |
int | ReadId (void) |
Reads a unique ID from the register. | |
Data Fields | |
char | bmp280_reset |
ID (0xD0) | |
uint16_t | dig_T1 |
Unique temp coeffs. read from the chip. | |
uint16_t | dig_P1 |
Unique Press. coeffs. read from the chip. | |
int32_t | t_fine |
This is calculated int the temperature to be used by the pressure. |
Detailed Description
Bosch BMP280 Digital Pressure Sensor.
Definition at line 45 of file BMP280.h.
Member Typedef Documentation
typedef union BMP280::bmp280_config_reg bmp280_config_t |
CTRL_MEAS (0xF4)
typedef union BMP280::bmp280_ctrl_meas bmp280_ctrl_meas_t |
STATUS (0xF3)
typedef union BMP280::bmp280_status bmp280_status_t |
RESET (0xE0)
Member Enumeration Documentation
enum BMP280_REG_map_t |
Constructor & Destructor Documentation
BMP280 | ( | PinName | sda, |
PinName | scl, | ||
int | slaveAddress | ||
) |
BMP280 constructor.
- Parameters:
-
sda mbed pin to use for SDA line of I2C interface. scl mbed pin to use for SCL line of I2C interface. slaveAddress Slave Address of the device.
Definition at line 37 of file BMP280.cpp.
BMP280 | ( | I2C * | i2c, |
int | slaveAddress | ||
) |
BMP280 constructor.
- Parameters:
-
i2c I2C object to use. slaveAddress Slave Address of the device.
Definition at line 45 of file BMP280.cpp.
BMP280 | ( | PinName | sda, |
PinName | scl | ||
) |
BMP280 constructor.
- Parameters:
-
sda mbed pin to use for SDA line of I2C interface. scl mbed pin to use for SCL line of I2C interface.
~BMP280 | ( | void | ) |
BMP280 destructor.
Definition at line 57 of file BMP280.cpp.
Member Function Documentation
float compensate_P_float | ( | int32_t | adc_P ) |
Performs calculations on the raw pressure data to convert to.
pressure in Pascal, based on Bosch's algorithm
- Parameters:
-
adc_P Raw Press ADC value, Global dig_P1, dig_P2,..., dig_P9
- Returns:
- The Pressure in Pascals
Definition at line 292 of file BMP280.cpp.
float compensate_T_float | ( | int32_t | adc_T ) |
Performs calculations on the raw temperature data to convert to.
temperature in deg C, based on Bosch's algorithm
- Parameters:
-
Raw Temp ADC value, Global dig_T1, dig_T2, dig_T3
- Returns:
- The Temperature in deg C
Definition at line 274 of file BMP280.cpp.
int Detect | ( | void | ) |
Detects if the BMP280 is present.
- Parameters:
-
none
- Returns:
- 1 for found, 0 for not found, -1 for comm error
Definition at line 252 of file BMP280.cpp.
int init | ( | BMP280::bmp280_osrs_P_t | Osrs_p, |
BMP280::bmp280_osrs_T_t | Osrs_t, | ||
BMP280::bmp280_FILT_t | Filter, | ||
BMP280::bmp280_MODE_t | Mode, | ||
BMP280::bmp280_TSB_t | T_sb | ||
) |
This initializes the BMP280.
The BMP280 has 2 modes. FORCED mode and NORMAL mode. FORCED Mode gives more control to the processor as the processor sends out the Mode to initiate a conversion and a data is sent out then. NORMAL mode is initialized once and it just runs and sends out data at a programmed timed interval. (In this example the main() will set this to Normal function)
- Parameters:
-
Osrs_p- Pressure oversampling Osrs_t- Temperature oversampling Filter- Filter Settings Mode- Power Modes T_sb- Standby time (used with Normal mode) dig_T1,dig_T2,dig_T3- Coeffs used for temp conversion - GLOBAL variables (output) dig_P1,....,dig_P9- Coeffs used for press conversion - GLOBAL variables (output)
- Returns:
- 0-if no error. A non-zero value indicates an error.
Read all the temp coeffecients from the BMP280 memory. It will be used in calculation
Read all the press coeffecients from the BMP280 memory. It will be used in calculation
Definition at line 65 of file BMP280.cpp.
int ReadCompData | ( | float * | Temp_degC, |
float * | Press_Pa | ||
) |
The BMP280 has 2 modes.
FORCED mode and NORMAL mode. FORCED Mode gives more control to the processor as the processor sends out the Mode to initiate a conversion and a data is sent out then. NORMAL mode is initialized once and it just runs and sends out data at a programmed timed interval. (In this example the main() will set this to Normal function)
- Parameters:
-
*Temp_degC - Pointer to temperature (result in deg C) *Press_Pa - Pointer to pressure (resul in Pascal)
- Returns:
- 0-if no error. A non-zero value indicates an error.
Definition at line 189 of file BMP280.cpp.
int ReadCompDataRaw | ( | char * | bmp280_rawData ) |
This reads the raw BMP280 data.
- Parameters:
-
*bmp280_rawData- array of raw output data
- Returns:
- 0-if no error. A non-zero value indicates an error.
Definition at line 167 of file BMP280.cpp.
int ReadCompDataRaw2 | ( | char * | bmp280_rawData ) |
This reads the raw BMP280 data uses the Bosch algorithm to get the data.
in float, then the float gets converted to an String
- Parameters:
-
*bmp280_rawData- array of raw output data
- Returns:
- 0-if no error. A non-zero value indicates an error.
Definition at line 148 of file BMP280.cpp.
int ReadId | ( | void | ) |
Reads a unique ID from the register.
- Parameters:
-
none
- Returns:
- The correct id value which is 0x58
Definition at line 265 of file BMP280.cpp.
int readReg | ( | char | reg, |
char * | value | ||
) |
Read a device register.
CONFIG_REG (0xF5)
int reg_read | ( | BMP280_REG_map_t | reg, |
char * | value, | ||
char | number | ||
) |
This function allows writing to a register.
reg- Address of the register to read from (input) *value- Pointer to the value read from the register (output)
- Returns:
- 0-if no error. A non-zero value indicates an error.
Definition at line 213 of file BMP280.cpp.
int reg_write | ( | BMP280_REG_map_t | reg, |
char | value | ||
) |
This function allows writing to a register.
- Parameters:
-
reg- Address of the register to write to value- Data written to the register
- Returns:
- 0-if no error. A non-zero value indicates an error.
Definition at line 201 of file BMP280.cpp.
void Reset | ( | void | ) |
Performs a soft reset on the BMP280.
- Parameters:
-
none
- Returns:
- none
Definition at line 246 of file BMP280.cpp.
int Sleep | ( | void | ) |
Puts the BMP280 in low power Sleep mode.
- Parameters:
-
none
- Returns:
- 0 if no errors, -1 if error.
Definition at line 230 of file BMP280.cpp.
float ToFahrenheit | ( | float | temperature ) |
converts to Farenhite from Centigrade
- Parameters:
-
temperature in Centigrade
- Returns:
- temperature value in Farenhite
Definition at line 142 of file BMP280.cpp.
void ToFloat | ( | char * | bmp280_rawData, |
float * | Temp_degC, | ||
float * | Press_Pa | ||
) |
This converts the raw BMP280 data to couble based on Bosch's algorithm.
- Parameters:
-
*bmp280_rawData- array of raw input data *Temp_degC- pointer to output, Temp value in deg C *Press_Pa- pointer to output, Press value in Pascals
- Returns:
- 0-if no error. A non-zero value indicates an error.
Definition at line 176 of file BMP280.cpp.
int writeReg | ( | char | reg, |
char | value | ||
) |
Write a device register.
Field Documentation
char bmp280_reset |
Generated on Tue Jul 12 2022 17:59:20 by
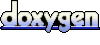