
MAX32620HSP (MAXREFDES100) RPC Example for Graphical User Interface
Dependencies: USBDevice
Fork of HSP_Release by
MAX30101 Class Reference
Maxim Integrated MAX30101 Oximeter chip. More...
#include <MAX30101.h>
Data Structures | |
union | max30101_fifo_configuration_reg |
FIFO_CONFIGURATION (0x08) More... | |
union | max30101_fifo_rd_ptr_reg |
FIFO_READ_PTR (0x06) More... | |
union | max30101_fifo_wr_ptr_reg |
FIFO_WR_PTR (0x04) More... | |
union | max30101_Interrupt_Enable_1_reg |
INTERRUPT_ENABLE1 (0x02) More... | |
union | max30101_Interrupt_Enable_2_reg |
INTERRUPT_ENABLE2 (0x03) More... | |
union | max30101_Interrupt_Status_1_reg |
STATUS1 (0x00) More... | |
union | max30101_Interrupt_Status_2_reg |
STATUS2 (0x01) More... | |
union | max30101_mode_configuration_reg |
MODE_CONFIGURATION (0x09) More... | |
union | max30101_ovf_counter_reg |
OVF_COUNTER (0x05) More... | |
union | max30101_spo2_configuration_reg |
SPO2_CONGIGURATION (0x0A) More... | |
Public Types | |
enum | MAX30101_REG_map_t { REG_INT_STAT_1 = 0x00 , REG_FIFO_DATA = 0x07 , REG_SLT4_SLT3 = 0x12 , REG_TEMP_EN = 0x21 } |
typedef union MAX30101::max30101_Interrupt_Status_1_reg | max30101_Interrupt_Status_1_t |
STATUS1 (0x00) | |
typedef union MAX30101::max30101_Interrupt_Status_2_reg | max30101_Interrupt_Status_2_t |
STATUS2 (0x01) | |
typedef union MAX30101::max30101_Interrupt_Enable_1_reg | max30101_Interrupt_Enable_1_t |
INTERRUPT_ENABLE1 (0x02) | |
typedef union MAX30101::max30101_Interrupt_Enable_2_reg | max30101_Interrupt_Enable_2_t |
INTERRUPT_ENABLE2 (0x03) | |
typedef union MAX30101::max30101_fifo_wr_ptr_reg | max30101_fifo_wr_ptr_t |
FIFO_WR_PTR (0x04) | |
typedef union MAX30101::max30101_ovf_counter_reg | max30101_ovf_counter_reg_t |
OVF_COUNTER (0x05) | |
typedef union MAX30101::max30101_fifo_rd_ptr_reg | max30101_fifo_rd_ptr_t |
FIFO_READ_PTR (0x06) | |
typedef union MAX30101::max30101_fifo_configuration_reg | max30101_fifo_configuration_t |
FIFO_CONFIGURATION (0x08) | |
typedef union MAX30101::max30101_mode_configuration_reg | max30101_mode_configuration_t |
MODE_CONFIGURATION (0x09) | |
typedef union MAX30101::max30101_spo2_configuration_reg | max30101_spo2_configuration_t |
SPO2_CONGIGURATION (0x0A) | |
typedef void(* | DataCallbackFunction )(uint32_t id, uint32_t *buffer, uint32_t length) |
type definition for data interrupt | |
typedef void(* | InterruptFunction )() |
type definition for general interrupt | |
Public Member Functions | |
MAX30101 (PinName sda, PinName scl, int slaveAddress) | |
MAX30101 constructor. | |
MAX30101 (I2C *i2c, int slaveAddress) | |
MAX30101 constructor. | |
~MAX30101 (void) | |
MAX30101 destructor. | |
int | i2c_reg_read (MAX30101_REG_map_t reg, char *value) |
Allows reading from MAX30101 register. | |
int | i2c_reg_write (MAX30101_REG_map_t reg, char value) |
Allows writing to MAX30101 register. | |
int | SpO2mode_init (uint8_t fifo_waterlevel_mark, uint8_t sample_avg, uint8_t sample_rate, uint8_t pulse_width, uint8_t red_led_current, uint8_t ir_led_current) |
This function sets up for the SpO2 mode. | |
int | SpO2mode_stop (void) |
This function will stop the SpO2 mode and turn off all operating LED�s. | |
int | HRmode_init (uint8_t fifo_waterlevel_mark, uint8_t sample_avg, uint8_t sample_rate, uint8_t pulse_width, uint8_t red_led_current) |
This function sets up for the HR mode. | |
int | HRmode_stop (void) |
This function will stop the HR mode and turn off all operating LED’s. | |
int | Multimode_init (uint8_t fifo_waterlevel_mark, uint8_t sample_avg, uint8_t sample_rate, uint8_t pulse_width, uint8_t red_led_current, uint8_t ir_led_current, uint8_t green_led_current, uint8_t slot_1, uint8_t slot_2, uint8_t slot_3, uint8_t slot_4) |
This function sets up for the Multi-mode. | |
int | Multimode_stop (void) |
This function will stop the Multi-mode and turn off all operating LED’s. | |
int | tempread (void) |
This is a function that sets up for temperature read and should be called after one of the mode. | |
int | int_handler (void) |
This is a callback function which collects the data from the FIFO of the MAX30101 in a 32-bit. | |
void | onInterrupt (InterruptFunction _onInterrupt) |
Used to connect a callback for when interrupt data is available. | |
void | onDataAvailable (DataCallbackFunction _onDataAvailable) |
Used to connect a callback for when interrupt data is available. | |
Static Public Member Functions | |
static void | MidIntHandler (void) |
encapsulates the int_handler above | |
Data Fields | |
float | max30101_final_temp |
Global declaration. | |
uint32_t | max30101_buffer [MAX30101_PROC_DATA_SIZE] |
final Processed data | |
char | max30101_rawData [MAX30101_RAW_DATA_SIZE] |
raw data from the chip |
Detailed Description
Maxim Integrated MAX30101 Oximeter chip.
Definition at line 156 of file MAX30101.h.
Member Typedef Documentation
typedef void(* DataCallbackFunction)(uint32_t id, uint32_t *buffer, uint32_t length) |
type definition for data interrupt
Definition at line 487 of file MAX30101.h.
typedef void(* InterruptFunction)() |
type definition for general interrupt
Definition at line 492 of file MAX30101.h.
FIFO_CONFIGURATION (0x08)
typedef union MAX30101::max30101_fifo_rd_ptr_reg max30101_fifo_rd_ptr_t |
FIFO_READ_PTR (0x06)
typedef union MAX30101::max30101_fifo_wr_ptr_reg max30101_fifo_wr_ptr_t |
FIFO_WR_PTR (0x04)
INTERRUPT_ENABLE1 (0x02)
INTERRUPT_ENABLE2 (0x03)
STATUS1 (0x00)
STATUS2 (0x01)
MODE_CONFIGURATION (0x09)
typedef union MAX30101::max30101_ovf_counter_reg max30101_ovf_counter_reg_t |
OVF_COUNTER (0x05)
SPO2_CONGIGURATION (0x0A)
Member Enumeration Documentation
enum MAX30101_REG_map_t |
- Enumerator:
REG_INT_STAT_1 MAX30101 Register addresses.
Status
REG_FIFO_DATA Configuration.
REG_SLT4_SLT3 Die Temp.
REG_TEMP_EN Proximity Func.
Definition at line 162 of file MAX30101.h.
Constructor & Destructor Documentation
MAX30101 | ( | PinName | sda, |
PinName | scl, | ||
int | slaveAddress | ||
) |
MAX30101 constructor.
- Parameters:
-
sda mbed pin to use for SDA line of I2C interface. scl mbed pin to use for SCL line of I2C interface.
Definition at line 40 of file MAX30101.cpp.
MAX30101 | ( | I2C * | i2c, |
int | slaveAddress | ||
) |
~MAX30101 | ( | void | ) |
MAX30101 destructor.
Definition at line 62 of file MAX30101.cpp.
Member Function Documentation
int HRmode_init | ( | uint8_t | fifo_waterlevel_mark, |
uint8_t | sample_avg, | ||
uint8_t | sample_rate, | ||
uint8_t | pulse_width, | ||
uint8_t | red_led_current | ||
) |
This function sets up for the HR mode.
The data is returned in thecallback function max30101_int_handler in global array: buffer[].HR mode handles one LED (Red) data. Hence it can fill up the FIFO up to a maximum of 3bytes/sample x 32 = 96bytes. fifo_waterlevel_mark: corresponds to FIFO_A_FULL, In FIFO Configuration Register (0x08)
- Parameters:
-
sample_avg,: corresponds to SMP_AVE, in FIFO Configuration Register (0x08) sample_rate:corresponds to SPO2_SR, IN SpO2 Configuration Register (0x0A) pulse_width,: corresponds to LED_PW in SpO2 Configuration Register(0x0A) red_led_current,: corresponds to LED1_PA register (0x0C). Please see data sheet for values
- Returns:
- 0-if if no error. A non-zero value indicates an error.
< Reset the device, Mode = don't use...
< Let things settle down a bit
< Sample averaging;
< FIFO Roll over enabled
< Interrupt when certain level is filled
< ADC Range 8192 fullscale
< 100 Samp/sec.
< Pulse Width=411us and ADC Resolution=18
< Clear INT1 by reading the status
< Clear INT2 by reading the status
< Clear FIFO ptr
< Clear FIFO ptr
< Clear FIFO ptr
< HR mode
Definition at line 374 of file MAX30101.cpp.
int HRmode_stop | ( | void | ) |
This function will stop the HR mode and turn off all operating LED’s.
- Returns:
- 0-if if no error. A non-zero value indicates an error.
< Disable FIFO almost full interrupt
< HR mode off
Definition at line 459 of file MAX30101.cpp.
int i2c_reg_read | ( | MAX30101_REG_map_t | reg, |
char * | value | ||
) |
Allows reading from MAX30101 register.
- Parameters:
-
reg,: is the register address, to read from (look at max30101.h and the data sheet for details) value,: is the pointer to the value read from the register
- Returns:
- 0-if no error. A non-zero value indicates an error.
Definition at line 654 of file MAX30101.cpp.
int i2c_reg_write | ( | MAX30101_REG_map_t | reg, |
char | value | ||
) |
Allows writing to MAX30101 register.
- Parameters:
-
reg,: is the register address, to read from (look at max30101.h and the data sheet for details) value,: is the value to write to the register
- Returns:
- 0-if if no error. A non-zero value indicates an error.
Definition at line 642 of file MAX30101.cpp.
int int_handler | ( | void | ) |
This is a callback function which collects the data from the FIFO of the MAX30101 in a 32-bit.
unsigned global array called max30101_buffer[]. Upon every interrupt from the MAX30101, this function is called to service the FIFO of the MAX30101. This callback function also services the interrupt for the temp data. The temp data is collected in a floating point global variable final_temp.
- Parameters:
-
max30101_buffer[],global uint32_t
- Returns:
- 0-if everything is good. A non-zero value indicates an error.
< Read Interrupt flag bits
< Read Interrupt flag bits
< Read the sample(s)
< Heart Rate mode, i.e. 1 led
< SpO2 mode, i.e. 2 led
< Multi-LED mode, i.e. 1-4 led
: The FIFO Size is determined by the Sample size. The number of bytes in a Sample is dictated by number of LED's
LED Selected Bytes in "1" sample 1 3 2 6 3 9 4 12
The I2C API function limits the number of bytes to read, to 256 (i.e. char). Therefore, when set for Multiple LED's and the FIFO size is set to 32. It would mean there is more than 256 bytes. In that case two I2C reads have to be made. However It is important to note that each "Sample" must be read completely and reading only partial number of bytes from a sample will result in erroneous data.
For example: Num of LED selected = 3 and FIFO size is set to 32 (i.e. 0 value in register), then the number of bytes will be 3bytes/Led * 3led's * 32 = 288 bytes in all. Since there are 3 LED's each sample will contain (3 * 3) 9bytes. Therefore Sample 1 = 9bytes, Sample 2 = 18,... Sample 28 = 252. Therefore the first I2C read should be 252 bytes and the second read should be 288-252 = 36.
It turns out that this size issue comes up only when number of LED selected is 3 or 4 and choosing 252bytes for the first I2C read would work for both Number of LED selection.
< Right shift the data based on the LED_PW setting
< Die Temperature Config, Temp disable... after one read...
< Read Interrupt flag bits
Definition at line 69 of file MAX30101.cpp.
void MidIntHandler | ( | void | ) | [static] |
encapsulates the int_handler above
Definition at line 718 of file MAX30101.cpp.
int Multimode_init | ( | uint8_t | fifo_waterlevel_mark, |
uint8_t | sample_avg, | ||
uint8_t | sample_rate, | ||
uint8_t | pulse_width, | ||
uint8_t | red_led_current, | ||
uint8_t | ir_led_current, | ||
uint8_t | green_led_current, | ||
uint8_t | slot_1, | ||
uint8_t | slot_2, | ||
uint8_t | slot_3, | ||
uint8_t | slot_4 | ||
) |
This function sets up for the Multi-mode.
The data is returned in the callback function max30101_int_handler in global array: buffer[]. Multi-LED mode can handle 1 to 4 LED combinations. Hence it can fill up the FIFO up to a maximum of 3bytes/sample x 32 x 4 = 384bytes.
- Parameters:
-
fifo_waterlevel_mark,: corresponds to FIFO_A_FULL, In FIFO Configuration Register (0x08) sample_avg,: corresponds to SMP_AVE, in FIFO Configuration Register (0x08) sample_rate:corresponds to SPO2_SR, IN SpO2 Configuration Register (0x0A) pulse_width,: corresponds to LED_PW in SpO2 Configuration register(0x0A) red_led_current,: corresponds to LED1_PA register (0x0C). Please see data sheet for values ir_led_current,: corresponds to LED2_PA register (0x0D). Please see data sheet for values green_led_current,: corresponds to LED3_PA register (0x0E). Please see data sheet for values slot_1,…,slot_4,: corresponds to Multi-LED Mode control Registers (0x11-0x12).
- Returns:
- 0-if if no error. A non-zero value indicates an error.
< Reset the device, Mode = don't use...
< Let things settle down a bit
< Sample averaging;
< FIFO Roll over enabled
< Interrupt when certain level is filled
< ADC Range 8192 fullscale
< 100 Samp/sec.
< Pulse Width=411us and ADC Resolution=18
< Clear INT1 by reading the status
< Clear INT2 by reading the status
< Clear FIFO ptr
< Clear FIFO ptr
< Clear FIFO ptr
< Enable FIFO almost full interrupt
< Multi-LED mode
Definition at line 484 of file MAX30101.cpp.
int Multimode_stop | ( | void | ) |
This function will stop the Multi-mode and turn off all operating LED’s.
- Returns:
- 0-if if no error. A non-zero value indicates an error.
< Disable FIFO almost full interrupt
< Multi-LED mode off
Definition at line 595 of file MAX30101.cpp.
void onDataAvailable | ( | DataCallbackFunction | _onDataAvailable ) |
Used to connect a callback for when interrupt data is available.
Definition at line 689 of file MAX30101.cpp.
void onInterrupt | ( | InterruptFunction | _onInterrupt ) |
Used to connect a callback for when interrupt data is available.
Definition at line 703 of file MAX30101.cpp.
int SpO2mode_init | ( | uint8_t | fifo_waterlevel_mark, |
uint8_t | sample_avg, | ||
uint8_t | sample_rate, | ||
uint8_t | pulse_width, | ||
uint8_t | red_led_current, | ||
uint8_t | ir_led_current | ||
) |
This function sets up for the SpO2 mode.
The data is returned in the callback function max30101_int_handler in global array: buffer[]. SP mode handles two LED (Red,IR) data. Hence it can fill up the FIFO up to a maximum of 3bytes/sample x 32 x 2 = 192bytes.
- Parameters:
-
fifo_waterlevel_mark,: corresponds to FIFO_A_FULL, In FIFO Configuration Register (0x08) sample_avg,: corresponds to SMP_AVE, in FIFO Configuration Register (0x08) sample_rate,: corresponds to SPO2_SR, IN SpO2 Configuration Register (0x0A) pulse_width,: corresponds to LED_PW in SpO2 Configuration register(0x0A) red_led_current,: corresponds to LED1_PA register (0x0C). Please see data sheet for values ir_led_current,: corresponds to LED2_PA register (0x0D). Please see data sheet for values
- Returns:
- 0-if everything is good. A non-zero value indicates an error.
< Let things settle down a bit
< Sample averaging;
< FIFO Roll over enabled
< Interrupt when certain level is filled
< ADC Range 8192 fullscale
< 100 Samp/sec.
< Pulse Width=411us and ADC Resolution=18
< Clear INT1 by reading the status
< Clear INT2 by reading the status
< Clear FIFO ptr
< Clear FIFO ptr
< Clear FIFO ptr
< Enable FIFO almost full interrupt
< SpO2 mode
Definition at line 253 of file MAX30101.cpp.
int SpO2mode_stop | ( | void | ) |
This function will stop the SpO2 mode and turn off all operating LED�s.
- Returns:
- 0-if if no error. A non-zero value indicates an error.
< Disable FIFO almost full interrupt
< SpO2 mode off
< RED LED current, 0.0
< IR LED current, 0.0
Definition at line 341 of file MAX30101.cpp.
int tempread | ( | void | ) |
This is a function that sets up for temperature read and should be called after one of the mode.
has been setup. The data is returned in the callback function max30101_int_handler. This function needs to be called every time temperature reading is required. Call the temp function after one of the MODES have been started Note that the temp is disabled after one read... also, it is not necessary to read the temp frequently...
- Returns:
- 0-if if no error. A non-zero value indicates an error.
< Interrupt Enable 2, Temperature Interrupt
< Die Temperature Config, Temp enable...
Definition at line 628 of file MAX30101.cpp.
Field Documentation
uint32_t max30101_buffer[MAX30101_PROC_DATA_SIZE] |
final Processed data
Definition at line 159 of file MAX30101.h.
float max30101_final_temp |
Global declaration.
Definition at line 158 of file MAX30101.h.
char max30101_rawData[MAX30101_RAW_DATA_SIZE] |
raw data from the chip
Definition at line 160 of file MAX30101.h.
Generated on Tue Jul 12 2022 17:59:20 by
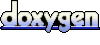