library for MAX14661 16:2 mux
Dependents: ard2pmod MAX14661_Demo
Max14661 Class Reference
#include <max14661.h>
Public Types | |
enum | max14661_i2c_adrs_t |
max14661_i2c_adrs_t - enumerated MAX14661 I2C Addresses More... | |
enum | max14661_regs_t |
max14661_regs_t - enumerated MAX14661 register addresses More... | |
enum | max14661_cmds_t |
max14661_cmds_t - enumerated MAX14661 commands More... | |
enum | max14661_sw_t |
max14661_sw_t - enumerated MAX14661 switch bitmasks More... | |
Public Member Functions | |
Max14661 (PinName sda, PinName scl, max14661_i2c_adrs_t i2c_adrs) | |
Constructor for Max14661 Class. | |
uint16_t | wrt_cmd_registers (max14661_cmds_t cmdA, max14661_cmds_t cmdB) |
Writes given commands to CMD_A and CMD_B. | |
uint16_t | wrt_shadow_registers (uint16_t bankA, uint16_t bankB) |
Writes bankA and bankB to coresponding shadow registers. | |
uint16_t | wrt_dir_registers (uint16_t bankA, uint16_t bankB) |
Writes bankA and bankB to coresponding direct access registers. | |
uint16_t | set_switches (uint16_t bankA, uint16_t bankB) |
Writes bankA and bankB to coresponding shadow register and then issues copy command for both banks. | |
uint16_t | rd_dir_registers (uint8_t *data) |
Reads data from direct access registers starting at DIR0 and stores it in byte array pointed at by 'data'. | |
uint16_t | rd_shadow_registers (uint8_t *data) |
Reads data from shadow registers starting at SHDW0 and stores it in byte array pointed at by 'data'. |
Detailed Description
Max14661 Class.
Definition at line 59 of file max14661.h.
Member Enumeration Documentation
enum max14661_cmds_t |
max14661_cmds_t - enumerated MAX14661 commands
Definition at line 98 of file max14661.h.
enum max14661_i2c_adrs_t |
max14661_i2c_adrs_t - enumerated MAX14661 I2C Addresses
Definition at line 68 of file max14661.h.
enum max14661_regs_t |
max14661_regs_t - enumerated MAX14661 register addresses
Definition at line 80 of file max14661.h.
enum max14661_sw_t |
max14661_sw_t - enumerated MAX14661 switch bitmasks
Definition at line 125 of file max14661.h.
Constructor & Destructor Documentation
Max14661 | ( | PinName | sda, |
PinName | scl, | ||
max14661_i2c_adrs_t | i2c_adrs | ||
) |
Constructor for Max14661 Class.
On Entry:
- Parameters:
-
[in] sda - sda pin of I2C bus [in] scl - scl pin of I2C bus [in] i2c_adrs - 7-bit slave address of MAX14661
On Exit:
- Returns:
- none
Example:
//declare mux object Max14661 mux(D14, D15, MAX14661_I2C_ADRS0);
Definition at line 71 of file max14661.cpp.
Member Function Documentation
uint16_t rd_dir_registers | ( | uint8_t * | data ) |
Reads data from direct access registers starting at DIR0 and stores it in byte array pointed at by 'data'.
On Entry:
- Parameters:
-
[in] data - pointer to byte array for storing data
On Exit:
- Parameters:
-
[out] data - data buffer now contains data read from dir registers
- Returns:
- return value = 0 on success, non-0 on failure
Example:
//declare mux object Max14661 mux(D14, D15, MAX14661_I2C_ADRS0); uint16_t rtn_val; uint8_t data[4]; //read direct access registers rtn_val = mux.rd_dir_registers(data);
Definition at line 277 of file max14661.cpp.
uint16_t rd_shadow_registers | ( | uint8_t * | data ) |
Reads data from shadow registers starting at SHDW0 and stores it in byte array pointed at by 'data'.
On Entry:
- Parameters:
-
[in] data - pointer to byte array for storing data
On Exit:
- Parameters:
-
[out] data - data buffer now contains data read from shadow registers
- Returns:
- return value = 0 on success, non-0 on failure
Example:
//declare mux object Max14661 mux(D14, D15, MAX14661_I2C_ADRS0); uint16_t rtn_val; uint8_t data[4]; //read shadow registers rtn_val = mux.rd_shadow_registers(data);
Definition at line 320 of file max14661.cpp.
uint16_t set_switches | ( | uint16_t | bankA, |
uint16_t | bankB | ||
) |
Writes bankA and bankB to coresponding shadow register and then issues copy command for both banks.
On Entry:
- Parameters:
-
[in] bankA - binary representation of switch states [in] bankB - binary representation of switch states
On Exit:
- Returns:
- return value = 0 on success, non-0 on failure
Example:
//declare mux object Max14661 mux(D14, D15, MAX14661_I2C_ADRS0); uint16_t bankA = (SW12 | SW02); //example only uint16_t bankB = (SW11 | SW01); uint16_t rtn_val; //wite shadow registers rtn_val = mux.set_switches(bankA, bankB);
Definition at line 231 of file max14661.cpp.
uint16_t wrt_cmd_registers | ( | max14661_cmds_t | cmdA, |
max14661_cmds_t | cmdB | ||
) |
Writes given commands to CMD_A and CMD_B.
On Entry:
- Parameters:
-
[in] cmdA - command for CMD_A [in] cmdB - command for CMD_B
On Exit:
- Returns:
- return value = 0 on success, non-0 on failure
Example:
//declare mux object Max14661 mux(D14, D15, MAX14661_I2C_ADRS0); uint16_t rtn_val; rtn_val = mux.wrt_cmd_registers(DISABLE_BANK, DISABLE_BANK);
Definition at line 101 of file max14661.cpp.
uint16_t wrt_dir_registers | ( | uint16_t | bankA, |
uint16_t | bankB | ||
) |
Writes bankA and bankB to coresponding direct access registers.
On Entry:
- Parameters:
-
[in] bankA - binary representation of switch states [in] bankB - binary representation of switch states
On Exit:
- Returns:
- return value = 0 on success, non-0 on failure
Example:
//declare mux object Max14661 mux(D14, D15, MAX14661_I2C_ADRS0); uint16_t bankA = (SW12 | SW02); //example only uint16_t bankB = (SW11 | SW01); uint16_t rtn_val; //wite shadow registers rtn_val = mux.wrt_dir_registers(bankA, bankB);
Definition at line 187 of file max14661.cpp.
uint16_t wrt_shadow_registers | ( | uint16_t | bankA, |
uint16_t | bankB | ||
) |
Writes bankA and bankB to coresponding shadow registers.
On Entry:
- Parameters:
-
[in] bankA - binary representation of switch states [in] bankB - binary representation of switch states
On Exit:
- Returns:
- return value = 0 on success, non-0 on failure
Example:
//declare mux object Max14661 mux(D14, D15, MAX14661_I2C_ADRS0); uint16_t bankA = (SW12 | SW02); //example only uint16_t bankB = (SW11 | SW01); uint16_t rtn_val; //wite shadow registers rtn_val = mux.wrt_shadow_registers(bankA, bankB);
Definition at line 144 of file max14661.cpp.
Generated on Thu Jul 14 2022 13:51:13 by
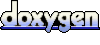