library for MAX14661 16:2 mux
Dependents: ard2pmod MAX14661_Demo
max14661.h
00001 /******************************************************************//** 00002 * @file max14661.h 00003 * 00004 * @author Justin Jordan 00005 * 00006 * @version 1.0 00007 * 00008 * Started: 11NOV14 00009 * 00010 * Updated: 00011 * 00012 * @brief Header file for MAX14661 class 00013 * 00014 *********************************************************************** 00015 * 00016 * @copyright 00017 * Copyright (C) 2015 Maxim Integrated Products, Inc., All Rights Reserved. 00018 * 00019 * Permission is hereby granted, free of charge, to any person obtaining a 00020 * copy of this software and associated documentation files (the "Software"), 00021 * to deal in the Software without restriction, including without limitation 00022 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00023 * and/or sell copies of the Software, and to permit persons to whom the 00024 * Software is furnished to do so, subject to the following conditions: 00025 * 00026 * The above copyright notice and this permission notice shall be included 00027 * in all copies or substantial portions of the Software. 00028 * 00029 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00030 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00031 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00032 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00033 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00034 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00035 * OTHER DEALINGS IN THE SOFTWARE. 00036 * 00037 * Except as contained in this notice, the name of Maxim Integrated 00038 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00039 * Products, Inc. Branding Policy. 00040 * 00041 * The mere transfer of this software does not imply any licenses 00042 * of trade secrets, proprietary technology, copyrights, patents, 00043 * trademarks, maskwork rights, or any other form of intellectual 00044 * property whatsoever. Maxim Integrated Products, Inc. retains all 00045 * ownership rights. 00046 **********************************************************************/ 00047 00048 00049 #ifndef MAX14661_H 00050 #define MAX14661_H 00051 00052 00053 #include "mbed.h" 00054 00055 00056 /******************************************************************//** 00057 * Max14661 Class 00058 **********************************************************************/ 00059 class Max14661 : public I2C 00060 { 00061 uint8_t w_adrs, r_adrs; 00062 00063 public: 00064 00065 /** 00066 * max14661_i2c_adrs_t - enumerated MAX14661 I2C Addresses 00067 */ 00068 typedef enum 00069 { 00070 MAX14661_I2C_ADRS0 = 0x4C, 00071 MAX14661_I2C_ADRS1, 00072 MAX14661_I2C_ADRS2, 00073 MAX14661_I2C_ADRS3 00074 }max14661_i2c_adrs_t; 00075 00076 00077 /** 00078 * max14661_regs_t - enumerated MAX14661 register addresses 00079 */ 00080 typedef enum 00081 { 00082 DIR0, //Switches 8A–1A direct read/write access 00083 DIR1, //Switches 16A–9A direct read/write access 00084 DIR2, //Switches 8B–1B direct read/write access 00085 DIR3, //Switches 16B–9B direct read/write access 00086 SHDW0 = 0x10, //Switches 8A–1A shadow read/write access 00087 SHDW1, //Switches 16A–9A shadow read/write access 00088 SHDW2, //Switches 8B–1B shadow read/write access 00089 SHDW3, //Switches 16B–9B shadow read/write access 00090 CMD_A, //Set mux A command (reads 0x00) 00091 CMD_B //Set mux B command (reads 0x00) 00092 }max14661_regs_t; 00093 00094 00095 /** 00096 * max14661_cmds_t - enumerated MAX14661 commands 00097 */ 00098 typedef enum 00099 { 00100 ENABLE_SW01, //enables sw1 on bank only 00101 ENABLE_SW02, //enables sw2 on bank only 00102 ENABLE_SW03, //enables sw3 on bank only 00103 ENABLE_SW04, //enables sw4 on bank only 00104 ENABLE_SW05, //enables sw5 on bank only 00105 ENABLE_SW06, //enables sw6 on bank only 00106 ENABLE_SW07, //enables sw7 on bank only 00107 ENABLE_SW08, //enables sw8 on bank only 00108 ENABLE_SW09, //enables sw9 on bank only 00109 ENABLE_SW10, //enables sw10 on bank only 00110 ENABLE_SW11, //enables sw11 on bank only 00111 ENABLE_SW12, //enables sw12 on bank only 00112 ENABLE_SW13, //enables sw13 on bank only 00113 ENABLE_SW14, //enables sw14 on bank only 00114 ENABLE_SW15, //enables sw15 on bank only 00115 ENABLE_SW16, //enables sw16 on bank only 00116 DISABLE_BANK, //opens all switches on bank 00117 COPY_SHADOW, //copies both shadow registers for bank 00118 NO_CHANGE, 00119 }max14661_cmds_t; 00120 00121 00122 /** 00123 * max14661_sw_t - enumerated MAX14661 switch bitmasks 00124 */ 00125 typedef enum 00126 { 00127 SW01 = (1 << 0), 00128 SW02 = (1 << 1), 00129 SW03 = (1 << 2), 00130 SW04 = (1 << 3), 00131 SW05 = (1 << 4), 00132 SW06 = (1 << 5), 00133 SW07 = (1 << 6), 00134 SW08 = (1 << 7), 00135 SW09 = (1 << 8), 00136 SW10 = (1 << 9), 00137 SW11 = (1 << 10), 00138 SW12 = (1 << 11), 00139 SW13 = (1 << 12), 00140 SW14 = (1 << 13), 00141 SW15 = (1 << 14), 00142 SW16 = (1 << 15) 00143 }max14661_sw_t; 00144 00145 00146 /**********************************************************//** 00147 * Constructor for Max14661 Class 00148 * 00149 * On Entry: 00150 * @param[in] sda - sda pin of I2C bus 00151 * @param[in] scl - scl pin of I2C bus 00152 * @param[in] i2c_adrs - 7-bit slave address of MAX14661 00153 * 00154 * On Exit: 00155 * @return none 00156 * 00157 * Example: 00158 * @code 00159 * 00160 * //declare mux object 00161 * Max14661 mux(D14, D15, MAX14661_I2C_ADRS0); 00162 * 00163 * @endcode 00164 **************************************************************/ 00165 Max14661(PinName sda, PinName scl, max14661_i2c_adrs_t i2c_adrs); 00166 00167 00168 /******************************************************************//** 00169 * Writes given commands to CMD_A and CMD_B 00170 * 00171 * On Entry: 00172 * @param[in] cmdA - command for CMD_A 00173 * @param[in] cmdB - command for CMD_B 00174 * 00175 * On Exit: 00176 * @return return value = 0 on success, non-0 on failure 00177 * 00178 * Example: 00179 * @code 00180 * 00181 * //declare mux object 00182 * Max14661 mux(D14, D15, MAX14661_I2C_ADRS0); 00183 * 00184 * uint16_t rtn_val; 00185 * 00186 * rtn_val = mux.wrt_cmd_registers(DISABLE_BANK, DISABLE_BANK); 00187 * 00188 * @endcode 00189 **********************************************************************/ 00190 uint16_t wrt_cmd_registers(max14661_cmds_t cmdA, max14661_cmds_t cmdB); 00191 00192 00193 /******************************************************************//** 00194 * Writes bankA and bankB to coresponding shadow registers 00195 * 00196 * On Entry: 00197 * @param[in] bankA - binary representation of switch states 00198 * @param[in] bankB - binary representation of switch states 00199 * 00200 * On Exit: 00201 * @return return value = 0 on success, non-0 on failure 00202 * 00203 * Example: 00204 * @code 00205 * 00206 * //declare mux object 00207 * Max14661 mux(D14, D15, MAX14661_I2C_ADRS0); 00208 * 00209 * uint16_t bankA = (SW12 | SW02); //example only 00210 * uint16_t bankB = (SW11 | SW01); 00211 * uint16_t rtn_val; 00212 * 00213 * //wite shadow registers 00214 * rtn_val = mux.wrt_shadow_registers(bankA, bankB); 00215 * 00216 * @endcode 00217 **********************************************************************/ 00218 uint16_t wrt_shadow_registers(uint16_t bankA, uint16_t bankB); 00219 00220 00221 /******************************************************************//** 00222 * Writes bankA and bankB to coresponding direct access registers 00223 * 00224 * On Entry: 00225 * @param[in] bankA - binary representation of switch states 00226 * @param[in] bankB - binary representation of switch states 00227 * 00228 * On Exit: 00229 * @return return value = 0 on success, non-0 on failure 00230 * 00231 * Example: 00232 * @code 00233 * 00234 * //declare mux object 00235 * Max14661 mux(D14, D15, MAX14661_I2C_ADRS0); 00236 * 00237 * uint16_t bankA = (SW12 | SW02); //example only 00238 * uint16_t bankB = (SW11 | SW01); 00239 * uint16_t rtn_val; 00240 * 00241 * //wite shadow registers 00242 * rtn_val = mux.wrt_dir_registers(bankA, bankB); 00243 * 00244 * @endcode 00245 **********************************************************************/ 00246 uint16_t wrt_dir_registers(uint16_t bankA, uint16_t bankB); 00247 00248 00249 /******************************************************************//** 00250 * Writes bankA and bankB to coresponding shadow register and then 00251 * issues copy command for both banks 00252 * 00253 * On Entry: 00254 * @param[in] bankA - binary representation of switch states 00255 * @param[in] bankB - binary representation of switch states 00256 * 00257 * On Exit: 00258 * @return return value = 0 on success, non-0 on failure 00259 * 00260 * Example: 00261 * @code 00262 * 00263 * //declare mux object 00264 * Max14661 mux(D14, D15, MAX14661_I2C_ADRS0); 00265 * 00266 * uint16_t bankA = (SW12 | SW02); //example only 00267 * uint16_t bankB = (SW11 | SW01); 00268 * uint16_t rtn_val; 00269 * 00270 * //wite shadow registers 00271 * rtn_val = mux.set_switches(bankA, bankB); 00272 * 00273 * @endcode 00274 **********************************************************************/ 00275 uint16_t set_switches(uint16_t bankA, uint16_t bankB); 00276 00277 00278 /**********************************************************//** 00279 * Reads data from direct access registers starting at DIR0 and 00280 * stores it in byte array pointed at by 'data' 00281 * 00282 * On Entry: 00283 * @param[in] data - pointer to byte array for storing data 00284 * 00285 * On Exit: 00286 * @param[out] data - data buffer now contains data read 00287 * from dir registers 00288 * @return return value = 0 on success, non-0 on failure 00289 * 00290 * Example: 00291 * @code 00292 * 00293 * //declare mux object 00294 * Max14661 mux(D14, D15, MAX14661_I2C_ADRS0); 00295 * 00296 * uint16_t rtn_val; 00297 * uint8_t data[4]; 00298 * 00299 * //read direct access registers 00300 * rtn_val = mux.rd_dir_registers(data); 00301 * 00302 * @endcode 00303 **********************************************************************/ 00304 uint16_t rd_dir_registers(uint8_t* data); 00305 00306 00307 /**********************************************************//** 00308 * Reads data from shadow registers starting at SHDW0 and stores 00309 * it in byte array pointed at by 'data' 00310 * 00311 * On Entry: 00312 * @param[in] data - pointer to byte array for storing data 00313 * 00314 * On Exit: 00315 * @param[out] data - data buffer now contains data read 00316 * from shadow registers 00317 * @return return value = 0 on success, non-0 on failure 00318 * 00319 * Example: 00320 * @code 00321 * 00322 * //declare mux object 00323 * Max14661 mux(D14, D15, MAX14661_I2C_ADRS0); 00324 * 00325 * uint16_t rtn_val; 00326 * uint8_t data[4]; 00327 * 00328 * //read shadow registers 00329 * rtn_val = mux.rd_shadow_registers(data); 00330 * 00331 * @endcode 00332 **************************************************************/ 00333 uint16_t rd_shadow_registers(uint8_t* data); 00334 }; 00335 00336 00337 #endif /* MAX14661_H*/
Generated on Thu Jul 14 2022 13:51:13 by
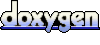