library for MAX14661 16:2 mux
Dependents: ard2pmod MAX14661_Demo
max14661.cpp
00001 /******************************************************************//** 00002 * @file max14661.cpp 00003 * 00004 * @author Justin Jordan 00005 * 00006 * @version 1.0 00007 * 00008 * Started: 11NOV14 00009 * 00010 * Updated: 00011 * 00012 * @brief Source file for MAX14661 class 00013 * 00014 *********************************************************************** 00015 * 00016 * @copyright 00017 * Copyright (C) 2015 Maxim Integrated Products, Inc., All Rights Reserved. 00018 * 00019 * Permission is hereby granted, free of charge, to any person obtaining a 00020 * copy of this software and associated documentation files (the "Software"), 00021 * to deal in the Software without restriction, including without limitation 00022 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00023 * and/or sell copies of the Software, and to permit persons to whom the 00024 * Software is furnished to do so, subject to the following conditions: 00025 * 00026 * The above copyright notice and this permission notice shall be included 00027 * in all copies or substantial portions of the Software. 00028 * 00029 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00030 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00031 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00032 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00033 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00034 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00035 * OTHER DEALINGS IN THE SOFTWARE. 00036 * 00037 * Except as contained in this notice, the name of Maxim Integrated 00038 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00039 * Products, Inc. Branding Policy. 00040 * 00041 * The mere transfer of this software does not imply any licenses 00042 * of trade secrets, proprietary technology, copyrights, patents, 00043 * trademarks, maskwork rights, or any other form of intellectual 00044 * property whatsoever. Maxim Integrated Products, Inc. retains all 00045 * ownership rights. 00046 **********************************************************************/ 00047 00048 00049 #include "max14661.h" 00050 00051 00052 /**********************************************************//** 00053 * Constructor for Max14661 Class 00054 * 00055 * On Entry: 00056 * @param[in] sda - sda pin of I2C bus 00057 * @param[in] scl - scl pin of I2C bus 00058 * @param[in] i2c_adrs - 7-bit slave address of MAX14661 00059 * 00060 * On Exit: 00061 * @return none 00062 * 00063 * Example: 00064 * @code 00065 * 00066 * //declare mux object 00067 * Max14661 mux(D14, D15, MAX14661_I2C_ADRS0); 00068 * 00069 * @endcode 00070 **************************************************************/ 00071 Max14661::Max14661(PinName sda, PinName scl, max14661_i2c_adrs_t i2c_adrs) : 00072 I2C(sda, scl) 00073 { 00074 w_adrs = (i2c_adrs << 1); 00075 r_adrs = (w_adrs | 0x01); 00076 } 00077 00078 00079 /******************************************************************//** 00080 * Writes given commands to CMD_A and CMD_B 00081 * 00082 * On Entry: 00083 * @param[in] cmdA - command for CMD_A 00084 * @param[in] cmdB - command for CMD_B 00085 * 00086 * On Exit: 00087 * @return return value = 0 on success, non-0 on failure 00088 * 00089 * Example: 00090 * @code 00091 * 00092 * //declare mux object 00093 * Max14661 mux(D14, D15, MAX14661_I2C_ADRS0); 00094 * 00095 * uint16_t rtn_val; 00096 * 00097 * rtn_val = mux.wrt_cmd_registers(DISABLE_BANK, DISABLE_BANK); 00098 * 00099 * @endcode 00100 **********************************************************************/ 00101 uint16_t Max14661::wrt_cmd_registers(max14661_cmds_t cmdA, 00102 max14661_cmds_t cmdB) 00103 { 00104 uint8_t data[3]; 00105 uint8_t data_length = 0; 00106 uint16_t rtn_val = 1; 00107 00108 //build packet 00109 data[data_length++] = CMD_A; 00110 data[data_length++] = cmdA; 00111 data[data_length++] = cmdB; 00112 00113 rtn_val = write(w_adrs,(const char*) data, data_length); 00114 00115 return(rtn_val); 00116 } 00117 00118 00119 /******************************************************************//** 00120 * Writes bankA and bankB to coresponding shadow registers 00121 * 00122 * On Entry: 00123 * @param[in] bankA - binary representation of switch states 00124 * @param[in] bankB - binary representation of switch states 00125 * 00126 * On Exit: 00127 * @return return value = 0 on success, non-0 on failure 00128 * 00129 * Example: 00130 * @code 00131 * 00132 * //declare mux object 00133 * Max14661 mux(D14, D15, MAX14661_I2C_ADRS0); 00134 * 00135 * uint16_t bankA = (SW12 | SW02); //example only 00136 * uint16_t bankB = (SW11 | SW01); 00137 * uint16_t rtn_val; 00138 * 00139 * //wite shadow registers 00140 * rtn_val = mux.wrt_shadow_registers(bankA, bankB); 00141 * 00142 * @endcode 00143 **********************************************************************/ 00144 uint16_t Max14661::wrt_shadow_registers(uint16_t bankA, uint16_t bankB) 00145 { 00146 uint8_t data[5]; 00147 uint8_t data_length = 0; 00148 uint16_t rtn_val = 1; 00149 00150 data[data_length++] = SHDW0; 00151 data[data_length++] = (bankA & 0x00FF); 00152 data[data_length++] = ((bankA >> 8) & 0x00FF); 00153 data[data_length++] = (bankB & 0x00FF); 00154 data[data_length++] = ((bankB >> 8) & 0x00FF); 00155 00156 rtn_val = write(w_adrs,(const char*) data, data_length); 00157 00158 return(rtn_val); 00159 } 00160 00161 00162 /******************************************************************//** 00163 * Writes bankA and bankB to coresponding direct access registers 00164 * 00165 * On Entry: 00166 * @param[in] bankA - binary representation of switch states 00167 * @param[in] bankB - binary representation of switch states 00168 * 00169 * On Exit: 00170 * @return return value = 0 on success, non-0 on failure 00171 * 00172 * Example: 00173 * @code 00174 * 00175 * //declare mux object 00176 * Max14661 mux(D14, D15, MAX14661_I2C_ADRS0); 00177 * 00178 * uint16_t bankA = (SW12 | SW02); //example only 00179 * uint16_t bankB = (SW11 | SW01); 00180 * uint16_t rtn_val; 00181 * 00182 * //wite shadow registers 00183 * rtn_val = mux.wrt_dir_registers(bankA, bankB); 00184 * 00185 * @endcode 00186 **********************************************************************/ 00187 uint16_t Max14661::wrt_dir_registers(uint16_t bankA, uint16_t bankB) 00188 { 00189 uint8_t data[5]; 00190 uint8_t data_length = 0; 00191 uint16_t rtn_val = 1; 00192 00193 data[data_length++] = DIR0; 00194 data[data_length++] = (bankA & 0x00FF); 00195 data[data_length++] = ((bankA >> 8) & 0x00FF); 00196 data[data_length++] = (bankB & 0x00FF); 00197 data[data_length++] = ((bankB >> 8) & 0x00FF); 00198 00199 rtn_val = write(w_adrs,(const char*) data, data_length); 00200 00201 return(rtn_val); 00202 } 00203 00204 00205 /******************************************************************//** 00206 * Writes bankA and bankB to coresponding shadow register and then 00207 * issues copy command for both banks 00208 * 00209 * On Entry: 00210 * @param[in] bankA - binary representation of switch states 00211 * @param[in] bankB - binary representation of switch states 00212 * 00213 * On Exit: 00214 * @return return value = 0 on success, non-0 on failure 00215 * 00216 * Example: 00217 * @code 00218 * 00219 * //declare mux object 00220 * Max14661 mux(D14, D15, MAX14661_I2C_ADRS0); 00221 * 00222 * uint16_t bankA = (SW12 | SW02); //example only 00223 * uint16_t bankB = (SW11 | SW01); 00224 * uint16_t rtn_val; 00225 * 00226 * //wite shadow registers 00227 * rtn_val = mux.set_switches(bankA, bankB); 00228 * 00229 * @endcode 00230 **********************************************************************/ 00231 uint16_t Max14661::set_switches(uint16_t bankA, uint16_t bankB) 00232 { 00233 uint8_t data[7]; 00234 uint8_t data_length = 0; 00235 uint16_t rtn_val = 1; 00236 00237 data[data_length++] = SHDW0; 00238 data[data_length++] = (bankA & 0x00FF); 00239 data[data_length++] = ((bankA >> 8) & 0x00FF); 00240 data[data_length++] = (bankB & 0x00FF); 00241 data[data_length++] = ((bankB >> 8) & 0x00FF); 00242 data[data_length++] = COPY_SHADOW; 00243 data[data_length++] = COPY_SHADOW; 00244 00245 rtn_val = write(w_adrs,(const char*) data, data_length); 00246 00247 return(rtn_val); 00248 } 00249 00250 00251 /**********************************************************//** 00252 * Reads data from direct access registers starting at DIR0 and 00253 * stores it in byte array pointed at by 'data' 00254 * 00255 * On Entry: 00256 * @param[in] data - pointer to byte array for storing data 00257 * 00258 * On Exit: 00259 * @param[out] data - data buffer now contains data read 00260 * from dir registers 00261 * @return return value = 0 on success, non-0 on failure 00262 * 00263 * Example: 00264 * @code 00265 * 00266 * //declare mux object 00267 * Max14661 mux(D14, D15, MAX14661_I2C_ADRS0); 00268 * 00269 * uint16_t rtn_val; 00270 * uint8_t data[4]; 00271 * 00272 * //read direct access registers 00273 * rtn_val = mux.rd_dir_registers(data); 00274 * 00275 * @endcode 00276 **********************************************************************/ 00277 uint16_t Max14661::rd_dir_registers(uint8_t* data) 00278 { 00279 uint16_t rtn_val = 1; 00280 00281 data[0] = DIR0; 00282 00283 rtn_val = write(w_adrs,(const char*) data, 1); 00284 00285 if(!rtn_val) 00286 { 00287 rtn_val = read(r_adrs,(char*) data, 4); 00288 } 00289 00290 return(rtn_val); 00291 } 00292 00293 00294 /**********************************************************//** 00295 * Reads data from shadow registers starting at SHDW0 and stores 00296 * it in byte array pointed at by 'data' 00297 * 00298 * On Entry: 00299 * @param[in] data - pointer to byte array for storing data 00300 * 00301 * On Exit: 00302 * @param[out] data - data buffer now contains data read 00303 * from shadow registers 00304 * @return return value = 0 on success, non-0 on failure 00305 * 00306 * Example: 00307 * @code 00308 * 00309 * //declare mux object 00310 * Max14661 mux(D14, D15, MAX14661_I2C_ADRS0); 00311 * 00312 * uint16_t rtn_val; 00313 * uint8_t data[4]; 00314 * 00315 * //read shadow registers 00316 * rtn_val = mux.rd_shadow_registers(data); 00317 * 00318 * @endcode 00319 **************************************************************/ 00320 uint16_t Max14661::rd_shadow_registers(uint8_t* data) 00321 { 00322 uint16_t rtn_val = 1; 00323 00324 data[0] = SHDW0; 00325 00326 rtn_val = write(w_adrs,(const char*) data, 1); 00327 00328 if(!rtn_val) 00329 { 00330 rtn_val = read(r_adrs,(char*) data, 4); 00331 } 00332 00333 return(rtn_val); 00334 }
Generated on Thu Jul 14 2022 13:51:13 by
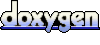