Embedded Artists
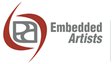
We are the leading providers of products and services around prototyping, evaluation and OEM platforms using NXP's ARM-based microcontrollers.
You are viewing an older revision! See the latest version
LPC4088DM Using Ethernet
The display modules come with 100/10Mbps Ethernet, RJ45 connector and unique MAC address.
The MAC address is unique (read from an onboard EEPROM with EUI-48) between the display modules. The actual address is retrieved by the Ethernet Interface through the mbed_mac_address() function in the mbed SDK, which is handled in the DMSupport library. No special setup needed.
Simple network example¶
The example below shows how to setup a network using DHCP and then retrieve IP addresses:
#include "EthernetInterface.h" ... EthernetInterface eth; ... void foo() { RtosLog* log = DMBoard::instance().logger(); log->printf("EthernetInterface Setting up...\n"); nsapi_error_t net_err = eth.connect(); if (net_err != NSAPI_ERROR_OK) { log->printf(NET_TASK_PREFIX"EthernetInterface connect Error %d\r\n", net_err); } else { log->printf(NET_TASK_PREFIX"IP Address is %s\r\n", eth.get_ip_address()); log->printf(NET_TASK_PREFIX"NetMask is %s\r\n", eth.get_netmask()); log->printf(NET_TASK_PREFIX"Gateway Address is %s\r\n", eth.get_gateway()); log->printf("MAC Address is %s\n", eth.get_mac_address()); log->printf(NET_TASK_PREFIX"Ethernet Setup OK\r\n"); } }
And with a static IP address:
#include "EthernetInterface.h" ... EthernetInterface eth; ... void foo() { nsapi_error_t net_err; RtosLog* log = DMBoard::instance().logger(); log->printf("EthernetInterface Setting up...\n"); do { net_err = eth.set_network("192.168.0.10", "255.255.255.0", "192.168.0.1"); if (net_err != NSAPI_ERROR_OK) { log->printf(NET_TASK_PREFIX"EthernetInterface set_network failed %d\r\n", net_err); break; } net_err = eth.connect(); if (net_err != NSAPI_ERROR_OK) { log->printf(NET_TASK_PREFIX"EthernetInterface connect failed %d\r\n", net_err); break; } log->printf(NET_TASK_PREFIX"EthernetInterface connected\r\n"); } while(false); }
HttpServer¶
The DMSupport library contains a fork of the HttpServer library by Yasushi TAUCHI as well as mbed's official mbed-rpc library as it is needed by the HttpServer.
The reason for forking the HttpServer and not using it directly is to replace all direct calls to printf with the thread safe RtosLog as well as adding some caching to improve performance on slower connections.
The Http server start function will run forever, so the code should be executed in it's own thread like this:
#include "EthernetInterface.h" #include "HTTPServer.h" #include "mbed_rpc.h" void netTask(void const* args) { RtosLog* log = DMBoard::instance().logger(); log->printf("EthernetInterface Setting up...\n"); if(eth.init() != 0) { log->printf("EthernetInterface Initialize Error\n"); mbed_die(); } while (eth.connect() != 0) { Thread::wait(1000); } // Now see what the DHCP server assigned us log->printf("IP Address is %s\n", eth.getIPAddress()); log->printf("NetMask is %s\n", eth.getNetworkMask()); log->printf("Gateway Address is %s\n", eth.getGateway()); log->printf("Ethernet Setup OK\n"); // Allow the server to handle the "/hello" URL by responding with "Hello world !" HTTPServerAddHandler<SimpleHandler>("/hello"); //Default handler // Allow the server to read files from the file system so that the URL "/myfile.txt" // will return the contents of the myfile.txt on the inserted USB memory stick. FSHandler::mount("/usb", "/"); HTTPServerAddHandler<FSHandler>("/"); // Now that all is configured, run the server forever waiting for connections on // port 80 HTTPServerStart(80); } void main() { ... Thread tNetwork(netTask, NULL, osPriorityNormal, 8192); ... }