
A board support package for the LPC4088 Display Module.
Dependencies: DM_HttpServer DM_USBHost
Dependents: lpc4088_displaymodule_emwin lpc4088_displaymodule_demo_sphere sampleGUI sampleEmptyGUI ... more
Fork of DMSupport by
Display Class Reference
#include <Display.h>
Inherited by BiosDisplay.
Public Member Functions | |
virtual DisplayError | init ()=0 |
Initialize the display. | |
virtual DisplayError | powerUp (void *framebuffer, Resolution res=Resolution_16bit_rgb565, FrameRate_t rate=FrameRate_Normal)=0 |
Turns the display on with the specified framebuffer showing. | |
virtual DisplayError | powerDown ()=0 |
Turns the display off. | |
virtual DisplayError | backlight (int percent)=0 |
Sets the backlight level. | |
virtual uint16_t | width ()=0 |
Returns the width (in pixels) of the display. | |
virtual uint16_t | height ()=0 |
Returns the height (in pixels) of the display. | |
virtual uint16_t | bytesPerPixel ()=0 |
Returns the number of bytes used by each pixel. | |
virtual uint32_t | fbSize ()=0 |
Returns the number of bytes used for each frame buffer. | |
virtual bool | landscape ()=0 |
Returns the display orientation. | |
virtual bool | isSupported (Resolution res)=0 |
Returns true if the specified resolution can be used. | |
virtual Resolution | currentResolution ()=0 |
Returns the current resolution. | |
virtual void | setFramebuffer (void *buff)=0 |
Replaces the current framebuffer. | |
virtual void * | swapFramebuffer (void *buff)=0 |
Replaces the current framebuffer with the specified one. | |
virtual void * | allocateFramebuffer (Resolution res=Resolution_16bit_rgb565)=0 |
Allocate enough memory for one framebuffer. | |
virtual void * | allocateFramebuffers (uint32_t num=1, Resolution res=Resolution_16bit_rgb565)=0 |
Allocate enough memory for one or more consequtive framebuffers. |
Detailed Description
Display example.
#include "mbed.h" #include "DMBoard.h" int main(void) { // initialize the display DMBoard::instance().init(); // allocate one framebuffer Display* disp = DMBoard::instance().display(); uint16_t* fb = (uint16_t*)disp->allocateFramebuffer(); if (fb == NULL) { DMBoard::instance().logger()->printf("Failed to allocate memory for framebuffer\r\n"); mbed_die(); } // draw something on the framebuffer ... // turn on the display disperr = disp->powerUp(fb); if (disperr != Display::Ok) { DMBoard::instance().logger()->printf("Failed to initialize the display, got error %d\r\n", disperr); mbed_die(); } ... }
Definition at line 56 of file Display.h.
Member Function Documentation
virtual void* allocateFramebuffer | ( | Resolution | res = Resolution_16bit_rgb565 ) |
[pure virtual] |
Allocate enough memory for one framebuffer.
This function is a to make it easier to allocate memory for framebuffers as the number of bytes needed depends on width, height and bytes per pixel.
Free the allocated memory when done using the free() function.
- Parameters:
-
res the resolution (default is the one that the display is using)
- Returns:
- a new framebuffer or NULL if out of memory
Implemented in BiosDisplay.
virtual void* allocateFramebuffers | ( | uint32_t | num = 1 , |
Resolution | res = Resolution_16bit_rgb565 |
||
) | [pure virtual] |
Allocate enough memory for one or more consequtive framebuffers.
This function is a to make it easier to allocate memory for framebuffers as the number of bytes needed depends on width, height and bytes per pixel.
Free the allocated memory when done using the free() function.
Use the default parameters to get one framebuffer for the display's current resolution.
- Parameters:
-
num the number of framebuffers, should be >= 1 res the resolution (default is the one that the display is using)
- Returns:
- new framebuffers or NULL if out of memory
Implemented in BiosDisplay.
virtual DisplayError backlight | ( | int | percent ) | [pure virtual] |
Sets the backlight level.
0% is off and 100% is fully on
- Parameters:
-
percent backlight in %
- Returns:
- Ok on success An error code on failure
Implemented in BiosDisplay.
virtual uint16_t bytesPerPixel | ( | ) | [pure virtual] |
virtual Resolution currentResolution | ( | ) | [pure virtual] |
virtual uint32_t fbSize | ( | ) | [pure virtual] |
Returns the number of bytes used for each frame buffer.
- Returns:
- width*height*bytesPerPixel
Implemented in BiosDisplay.
virtual uint16_t height | ( | ) | [pure virtual] |
Returns the height (in pixels) of the display.
- Returns:
- the display height
Implemented in BiosDisplay.
virtual DisplayError init | ( | ) | [pure virtual] |
Initialize the display.
Implemented in BiosDisplay.
virtual bool isSupported | ( | Resolution | res ) | [pure virtual] |
Returns true if the specified resolution can be used.
- Returns:
- true if supported, false if not
Implemented in BiosDisplay.
virtual bool landscape | ( | ) | [pure virtual] |
virtual DisplayError powerDown | ( | ) | [pure virtual] |
virtual DisplayError powerUp | ( | void * | framebuffer, |
Resolution | res = Resolution_16bit_rgb565 , |
||
FrameRate_t | rate = FrameRate_Normal |
||
) | [pure virtual] |
Turns the display on with the specified framebuffer showing.
- Parameters:
-
framebuffer the data to show res the resolution to use rate the frame rate to use
- Returns:
- Ok on success An error code on failure
Implemented in BiosDisplay.
virtual void setFramebuffer | ( | void * | buff ) | [pure virtual] |
Replaces the current framebuffer.
Note that this requires the caller or someone else to have a reference to the existing framebuffer, otherwise that memory is lost.
- Parameters:
-
buff the new framebuffer
Implemented in BiosDisplay.
virtual void* swapFramebuffer | ( | void * | buff ) | [pure virtual] |
Replaces the current framebuffer with the specified one.
This function as opposed to the setFramebuffer() one does return the old framebuffer. This way the caller can save the old one and then swap it back when done.
- Parameters:
-
buff the new framebuffer
- Returns:
- the old framebuffer
Implemented in BiosDisplay.
virtual uint16_t width | ( | ) | [pure virtual] |
Generated on Tue Jul 12 2022 14:18:31 by
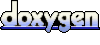