
A board support package for the LPC4088 Display Module.
Dependencies: DM_HttpServer DM_USBHost
Dependents: lpc4088_displaymodule_emwin lpc4088_displaymodule_demo_sphere sampleGUI sampleEmptyGUI ... more
Fork of DMSupport by
BiosDisplay Class Reference
Glue between the BIOS and the Display interface. More...
#include <BiosDisplay.h>
Inherits Display.
Public Member Functions | |
DisplayError | init () |
Initializes the display but does not turn it on. | |
virtual DisplayError | powerUp (void *framebuffer, Resolution wanted=Resolution_16bit_rgb565, FrameRate_t rate=FrameRate_Normal) |
Turns the display on with the specified framebuffer showing. | |
virtual DisplayError | powerDown () |
Turns the display off. | |
virtual DisplayError | backlight (int percent) |
Sets the backlight level. | |
virtual uint16_t | width () |
Returns the width (in pixels) of the display. | |
virtual uint16_t | height () |
Returns the height (in pixels) of the display. | |
virtual uint16_t | bytesPerPixel () |
Returns the number of bytes used by each pixel. | |
virtual uint32_t | fbSize () |
Returns the number of bytes used for each frame buffer. | |
virtual bool | landscape () |
Returns the display orientation. | |
virtual bool | isSupported (Resolution res) |
Returns true if the specified resolution can be used. | |
virtual Resolution | currentResolution () |
Returns the current resolution. | |
virtual void | setFramebuffer (void *buff) |
Replaces the current framebuffer. | |
virtual void * | swapFramebuffer (void *buff) |
Replaces the current framebuffer with the specified one. | |
virtual void * | allocateFramebuffer (Resolution res=Resolution_16bit_rgb565) |
Allocate enough memory for one framebuffer. | |
virtual void * | allocateFramebuffers (uint32_t num=1, Resolution res=Resolution_16bit_rgb565) |
Allocate enough memory for one or more consequtive framebuffers. | |
Static Public Member Functions | |
static BiosDisplay & | instance () |
Get the only instance of the BiosDisplay. |
Detailed Description
Glue between the BIOS and the Display interface.
Definition at line 28 of file BiosDisplay.h.
Member Function Documentation
void * allocateFramebuffer | ( | Resolution | res = Resolution_16bit_rgb565 ) |
[virtual] |
Allocate enough memory for one framebuffer.
This function is a to make it easier to allocate memory for framebuffers as the number of bytes needed depends on width, height and bytes per pixel.
Free the allocated memory when done using the free() function.
- Parameters:
-
res the resolution (default is the one that the display is using)
- Returns:
- a new framebuffer or NULL if out of memory
Implements Display.
Definition at line 169 of file BiosDisplay.cpp.
void * allocateFramebuffers | ( | uint32_t | num = 1 , |
Resolution | res = Resolution_16bit_rgb565 |
||
) | [virtual] |
Allocate enough memory for one or more consequtive framebuffers.
This function is a to make it easier to allocate memory for framebuffers as the number of bytes needed depends on width, height and bytes per pixel.
Free the allocated memory when done using the free() function.
Use the default parameters to get one framebuffer for the display's current resolution.
- Parameters:
-
num the number of framebuffers, should be >= 1 res the resolution (default is the one that the display is using)
- Returns:
- new framebuffers or NULL if out of memory
Implements Display.
Definition at line 177 of file BiosDisplay.cpp.
BiosDisplay::DisplayError backlight | ( | int | percent ) | [virtual] |
Sets the backlight level.
0% is off and 100% is fully on
- Parameters:
-
percent backlight in %
- Returns:
- Ok on success An error code on failure
Implements Display.
Definition at line 133 of file BiosDisplay.cpp.
uint16_t bytesPerPixel | ( | ) | [virtual] |
Returns the number of bytes used by each pixel.
- Returns:
- bytes per pixel
Implements Display.
Definition at line 154 of file BiosDisplay.cpp.
BiosDisplay::Resolution currentResolution | ( | ) | [virtual] |
Returns the current resolution.
- Returns:
- the current resolution
Implements Display.
Definition at line 202 of file BiosDisplay.cpp.
uint32_t fbSize | ( | ) | [virtual] |
Returns the number of bytes used for each frame buffer.
- Returns:
- width*height*bytesPerPixel
Implements Display.
Definition at line 159 of file BiosDisplay.cpp.
uint16_t height | ( | ) | [virtual] |
Returns the height (in pixels) of the display.
- Returns:
- the display height
Implements Display.
Definition at line 149 of file BiosDisplay.cpp.
BiosDisplay::DisplayError init | ( | ) | [virtual] |
Initializes the display but does not turn it on.
- Returns:
- Ok on success An error code on failure
Implements Display.
Definition at line 62 of file BiosDisplay.cpp.
static BiosDisplay& instance | ( | ) | [static] |
Get the only instance of the BiosDisplay.
- Returns:
- The display
Definition at line 35 of file BiosDisplay.h.
bool isSupported | ( | Resolution | res ) | [virtual] |
Returns true if the specified resolution can be used.
- Returns:
- true if supported, false if not
Implements Display.
Definition at line 197 of file BiosDisplay.cpp.
bool landscape | ( | ) | [virtual] |
Returns the display orientation.
- Returns:
- the display orientation
Implements Display.
Definition at line 164 of file BiosDisplay.cpp.
BiosDisplay::DisplayError powerDown | ( | ) | [virtual] |
Turns the display off.
- Returns:
- Ok on success An error code on failure
Implements Display.
Definition at line 123 of file BiosDisplay.cpp.
BiosDisplay::DisplayError powerUp | ( | void * | framebuffer, |
Resolution | res = Resolution_16bit_rgb565 , |
||
FrameRate_t | rate = FrameRate_Normal |
||
) | [virtual] |
Turns the display on with the specified framebuffer showing.
- Parameters:
-
framebuffer the data to show res the resolution to use rate the frame rate to use
- Returns:
- Ok on success An error code on failure
Implements Display.
Definition at line 97 of file BiosDisplay.cpp.
void setFramebuffer | ( | void * | buff ) | [virtual] |
Replaces the current framebuffer.
Note that this requires the caller or someone else to have a reference to the existing framebuffer, otherwise that memory is lost.
- Parameters:
-
buff the new framebuffer
Implements Display.
Definition at line 185 of file BiosDisplay.cpp.
void * swapFramebuffer | ( | void * | buff ) | [virtual] |
Replaces the current framebuffer with the specified one.
This function as opposed to the setFramebuffer() one does return the old framebuffer. This way the caller can save the old one and then swap it back when done.
- Parameters:
-
buff the new framebuffer
- Returns:
- the old framebuffer
Implements Display.
Definition at line 190 of file BiosDisplay.cpp.
uint16_t width | ( | ) | [virtual] |
Returns the width (in pixels) of the display.
- Returns:
- the display width
Implements Display.
Definition at line 144 of file BiosDisplay.cpp.
Generated on Tue Jul 12 2022 14:18:31 by
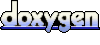