
A board support package for the LPC4088 Display Module.
Dependencies: DM_HttpServer DM_USBHost
Dependents: lpc4088_displaymodule_emwin lpc4088_displaymodule_demo_sphere sampleGUI sampleEmptyGUI ... more
Fork of DMSupport by
Display.h
00001 /* 00002 * Copyright 2014 Embedded Artists AB 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef DISPLAY_H 00018 #define DISPLAY_H 00019 00020 #include "mbed.h" 00021 #include "bios.h" 00022 00023 /** 00024 * Display example 00025 * 00026 * @code 00027 * #include "mbed.h" 00028 * #include "DMBoard.h" 00029 * 00030 * int main(void) { 00031 * // initialize the display 00032 * DMBoard::instance().init(); 00033 * 00034 * // allocate one framebuffer 00035 * Display* disp = DMBoard::instance().display(); 00036 * uint16_t* fb = (uint16_t*)disp->allocateFramebuffer(); 00037 * if (fb == NULL) { 00038 * DMBoard::instance().logger()->printf("Failed to allocate memory for framebuffer\r\n"); 00039 * mbed_die(); 00040 * } 00041 * 00042 * // draw something on the framebuffer 00043 * ... 00044 * 00045 * // turn on the display 00046 * disperr = disp->powerUp(fb); 00047 * if (disperr != Display::Ok) { 00048 * DMBoard::instance().logger()->printf("Failed to initialize the display, got error %d\r\n", disperr); 00049 * mbed_die(); 00050 * } 00051 * 00052 * ... 00053 * } 00054 * @endcode 00055 */ 00056 class Display { 00057 public: 00058 enum DisplayError { 00059 DisplayError_Ok = BiosError_Ok, 00060 DisplayError_ConfigError = BiosError_ConfigError, 00061 DisplayError_WrongBPP = BiosError_WrongBPP, 00062 DisplayError_InvalidParam = BiosError_InvalidParam, 00063 DisplayError_NoInit = BiosError_NoInit, 00064 DisplayError_CalibrationError = BiosError_Calibration, 00065 DisplayError_Timeout = BiosError_Timeout, 00066 DisplayError_TouchNotSupported = BiosError_NotSupported, 00067 DisplayError_MemoryError, 00068 }; 00069 00070 enum Resolution { 00071 Resolution_16bit_rgb565 = Res_16bit_rgb565, 00072 Resolution_18bit_rgb666 = Res_18bit_rgb666, 00073 Resolution_24bit_rgb888 = Res_24bit_rgb888, 00074 }; 00075 00076 /** 00077 * Initialize the display. 00078 */ 00079 virtual DisplayError init() = 0; 00080 00081 /** Turns the display on with the specified framebuffer showing 00082 * 00083 * @param framebuffer the data to show 00084 * @param res the resolution to use 00085 * @param rate the frame rate to use 00086 * 00087 * @returns 00088 * Ok on success 00089 * An error code on failure 00090 */ 00091 virtual DisplayError powerUp(void* framebuffer, Resolution res = Resolution_16bit_rgb565, FrameRate_t rate = FrameRate_Normal) = 0; 00092 00093 /** Turns the display off 00094 * 00095 * @returns 00096 * Ok on success 00097 * An error code on failure 00098 */ 00099 virtual DisplayError powerDown() = 0; 00100 00101 /** Sets the backlight level. 0% is off and 100% is fully on 00102 * 00103 * @param percent backlight in % 00104 * 00105 * @returns 00106 * Ok on success 00107 * An error code on failure 00108 */ 00109 virtual DisplayError backlight(int percent) = 0; 00110 00111 /** Returns the width (in pixels) of the display 00112 * 00113 * @returns the display width 00114 */ 00115 virtual uint16_t width() = 0; 00116 00117 /** Returns the height (in pixels) of the display 00118 * 00119 * @returns the display height 00120 */ 00121 virtual uint16_t height() = 0; 00122 00123 /** Returns the number of bytes used by each pixel 00124 * 00125 * @returns bytes per pixel 00126 */ 00127 virtual uint16_t bytesPerPixel() = 0; 00128 00129 /** Returns the number of bytes used for each frame buffer 00130 * 00131 * @returns width*height*bytesPerPixel 00132 */ 00133 virtual uint32_t fbSize() = 0; 00134 00135 /** Returns the display orientation 00136 * 00137 * @returns the display orientation 00138 */ 00139 virtual bool landscape() = 0; 00140 00141 /** Returns true if the specified resolution can be used 00142 * 00143 * @returns true if supported, false if not 00144 */ 00145 virtual bool isSupported(Resolution res) = 0; 00146 00147 /** Returns the current resolution 00148 * 00149 * @returns the current resolution 00150 */ 00151 virtual Resolution currentResolution() = 0; 00152 00153 /** Replaces the current framebuffer. 00154 * 00155 * Note that this requires the caller or someone else to have a 00156 * reference to the existing framebuffer, otherwise that memory 00157 * is lost. 00158 * 00159 * @param buff the new framebuffer 00160 */ 00161 virtual void setFramebuffer(void* buff) = 0; 00162 00163 /** Replaces the current framebuffer with the specified one. 00164 * 00165 * This function as opposed to the setFramebuffer() one does return 00166 * the old framebuffer. This way the caller can save the old one and 00167 * then swap it back when done. 00168 * 00169 * @param buff the new framebuffer 00170 * @returns the old framebuffer 00171 */ 00172 virtual void* swapFramebuffer(void* buff) = 0; 00173 00174 /** Allocate enough memory for one framebuffer 00175 * 00176 * This function is a to make it easier to allocate memory for framebuffers 00177 * as the number of bytes needed depends on width, height and bytes per pixel. 00178 * 00179 * Free the allocated memory when done using the free() function. 00180 * 00181 * @param res the resolution (default is the one that the display is using) 00182 * @returns a new framebuffer or NULL if out of memory 00183 */ 00184 virtual void* allocateFramebuffer(Resolution res=Resolution_16bit_rgb565) = 0; 00185 00186 /** Allocate enough memory for one or more consequtive framebuffers 00187 * 00188 * This function is a to make it easier to allocate memory for framebuffers 00189 * as the number of bytes needed depends on width, height and bytes per pixel. 00190 * 00191 * Free the allocated memory when done using the free() function. 00192 * 00193 * Use the default parameters to get one framebuffer for the display's current 00194 * resolution. 00195 * 00196 * @param num the number of framebuffers, should be >= 1 00197 * @param res the resolution (default is the one that the display is using) 00198 * @returns new framebuffers or NULL if out of memory 00199 */ 00200 virtual void* allocateFramebuffers(uint32_t num=1, Resolution res=Resolution_16bit_rgb565) = 0; 00201 }; 00202 00203 #endif
Generated on Tue Jul 12 2022 14:18:31 by
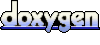