
A board support package for the LPC4088 Display Module.
Dependencies: DM_HttpServer DM_USBHost
Dependents: lpc4088_displaymodule_emwin lpc4088_displaymodule_demo_sphere sampleGUI sampleEmptyGUI ... more
Fork of DMSupport by
BiosDisplay.h
00001 /* 00002 * Copyright 2014 Embedded Artists AB 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef BIOSDISPLAY_H 00018 #define BIOSDISPLAY_H 00019 00020 #include "mbed.h" 00021 #include "TouchPanel.h" 00022 #include "Display.h" 00023 #include "bios.h" 00024 00025 /** 00026 * Glue between the BIOS and the Display interface. 00027 */ 00028 class BiosDisplay : public Display { 00029 public: 00030 00031 /** Get the only instance of the BiosDisplay 00032 * 00033 * @returns The display 00034 */ 00035 static BiosDisplay& instance() 00036 { 00037 static BiosDisplay singleton; 00038 return singleton; 00039 } 00040 00041 00042 /** Initializes the display but does not turn it on 00043 * 00044 * @returns 00045 * Ok on success 00046 * An error code on failure 00047 */ 00048 DisplayError init(); 00049 00050 // From the Display interface 00051 virtual DisplayError powerUp(void* framebuffer, Resolution wanted = Resolution_16bit_rgb565, FrameRate_t rate = FrameRate_Normal); 00052 virtual DisplayError powerDown(); 00053 virtual DisplayError backlight(int percent); 00054 virtual uint16_t width(); 00055 virtual uint16_t height(); 00056 virtual uint16_t bytesPerPixel(); 00057 virtual uint32_t fbSize(); 00058 virtual bool landscape(); 00059 virtual bool isSupported(Resolution res); 00060 virtual Resolution currentResolution(); 00061 virtual void setFramebuffer(void* buff); 00062 virtual void* swapFramebuffer(void* buff); 00063 virtual void* allocateFramebuffer(Resolution res=Resolution_16bit_rgb565); 00064 virtual void* allocateFramebuffers(uint32_t num=1, Resolution res=Resolution_16bit_rgb565); 00065 00066 private: 00067 00068 bool _initialized; 00069 bool _poweredOn; 00070 00071 bios_header_t* _bios; 00072 void* _biosData; 00073 00074 uint16_t _width; 00075 uint16_t _height; 00076 uint16_t _bpp; 00077 uint16_t _supportedRes; 00078 Resolution_t _activeRes; 00079 bool _landscape; 00080 00081 explicit BiosDisplay(); 00082 // hide copy constructor 00083 BiosDisplay(const BiosDisplay&); 00084 // hide assign operator 00085 BiosDisplay& operator=(const BiosDisplay&); 00086 ~BiosDisplay(); 00087 00088 uint32_t bpp(Resolution res) { return ((res == Resolution_16bit_rgb565) ? 2 : 4); } 00089 }; 00090 00091 #endif /* BIOSDISPLAY_H */
Generated on Tue Jul 12 2022 14:18:31 by
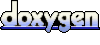