A basic graphics package for the LPC4088 Display Module.
Dependents: lpc4088_displaymodule_demo_sphere sampleGUI sampleEmptyGUI lpc4088_displaymodule_fs_aid ... more
Fork of DMBasicGUI by
ImageButton.h
00001 /* 00002 * Copyright 2014 Embedded Artists AB 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef IMAGEBUTTON_H 00018 #define IMAGEBUTTON_H 00019 00020 #include "Clickable.h" 00021 #include "Image.h" 00022 #include "Resource.h" 00023 00024 /** 00025 * The ImageButton is used in the same way as the Button so see it for an example 00026 */ 00027 class ImageButton : public Clickable { 00028 public: 00029 00030 /** Creates a new button 00031 * 00032 * This button will use a SWIM window to draw on. That window will use 00033 * part of the full size frame buffer to draw on. 00034 * 00035 * @param fb the frame buffer 00036 * @param x the upper left corner of the button 00037 * @param y the upper left corner of the button 00038 * @param width the width of the button 00039 * @param height the height of the button 00040 * @param caption optional text to put below the image 00041 * @param color text color 00042 */ 00043 ImageButton(COLOR_T* fb, uint16_t x, uint16_t y, uint16_t width, uint16_t height, 00044 const char* caption=NULL, COLOR_T color=BLACK); 00045 virtual ~ImageButton(); 00046 00047 /** Loads the mandatory "normal" state image and the optional "pressed" state image 00048 * 00049 * @param imgUp the file with the image for the normal state 00050 * @param imgDown the file with the image for the pressed state (or NULL to use the same) 00051 * 00052 * @returns 00053 * true on success 00054 * false on failure 00055 */ 00056 bool loadImages(const char* imgUp, const char* imgDown = 0); 00057 00058 /** Loads the mandatory "normal" state image and the optional "pressed" state image 00059 * 00060 * @param imgUp the image for the normal state 00061 * @param imgUpSize the size of the imgUp data 00062 * @param imgDown the image for the pressed state (or NULL to use the same) 00063 * @param imgUpSize the size of the imgDown data 00064 * 00065 * @returns 00066 * true on success 00067 * false on failure 00068 */ 00069 bool loadImages(const unsigned char* imgUp, unsigned int imgUpSize, 00070 const unsigned char* imgDown = 0, unsigned int imgDownSize = 0); 00071 00072 /** Loads the mandatory "normal" state image and the optional "pressed" state image 00073 * 00074 * @param resImgUp the resource for the normal state image 00075 * @param resImgDown the resource for the pressed state image (or NULL to use the same) 00076 * 00077 * @returns 00078 * true on success 00079 * false on failure 00080 */ 00081 bool loadImages(Resource* resImgUp, Resource* resImgDown = 0); 00082 00083 /** Specifys a color that will be considered transparent (i.e. will not be drawn) 00084 * @param tColor the transparent color 00085 */ 00086 void setTransparency(COLOR_T tColor); 00087 00088 /** Draws the button (on a new framebuffer if one is specified) 00089 * @param fb the frame buffer 00090 */ 00091 virtual void draw(COLOR_T* fb = 0); 00092 00093 private: 00094 Image::ImageData_t _imgUp; 00095 Image::ImageData_t _imgDown; 00096 char* _caption; 00097 COLOR_T _captionColor; 00098 bool _transparent; 00099 COLOR_T _transparentColor; 00100 }; 00101 00102 #endif /* IMAGEBUTTON_H */
Generated on Tue Jul 12 2022 21:27:03 by
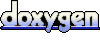