A basic graphics package for the LPC4088 Display Module.
Dependents: lpc4088_displaymodule_demo_sphere sampleGUI sampleEmptyGUI lpc4088_displaymodule_fs_aid ... more
Fork of DMBasicGUI by
ImageButton.cpp
00001 /* 00002 * Copyright 2014 Embedded Artists AB 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "ImageButton.h" 00018 #include "mbed.h" 00019 #include "DMBoard.h" 00020 00021 #include "lpc_swim_image.h" 00022 #include "lpc_swim_font.h" 00023 #include "lpc_colors.h" 00024 00025 ImageButton::ImageButton(COLOR_T* fb, uint16_t x, uint16_t y, uint16_t width, uint16_t height, 00026 const char* caption, COLOR_T color) : 00027 Clickable(fb, x, y, width, height+(caption==NULL?0:20)) 00028 { 00029 _imgUp.pointerToFree = NULL; 00030 _imgUp.pixels = NULL; 00031 _imgDown.pointerToFree = NULL; 00032 _imgDown.pixels = NULL; 00033 _caption = NULL; 00034 _transparent = false; 00035 00036 if (caption != NULL) { 00037 _caption = (char*)malloc(strlen(caption)+1); 00038 if (_caption != NULL) { 00039 strcpy(_caption, caption); 00040 } 00041 _captionColor = color; 00042 } 00043 } 00044 00045 ImageButton::~ImageButton() 00046 { 00047 if (_imgUp.pointerToFree != NULL) { 00048 free(_imgUp.pointerToFree); 00049 _imgUp.pointerToFree = NULL; 00050 } 00051 if (_imgDown.pointerToFree != NULL) { 00052 free(_imgDown.pointerToFree); 00053 _imgDown.pointerToFree = NULL; 00054 } 00055 if (_caption != NULL) { 00056 free(_caption); 00057 _caption = NULL; 00058 } 00059 } 00060 00061 bool ImageButton::loadImages(const char* imgUp, const char* imgDown) 00062 { 00063 if (_imgUp.pointerToFree != NULL) { 00064 free(_imgUp.pointerToFree); 00065 _imgUp.pointerToFree = NULL; 00066 } 00067 if (_imgDown.pointerToFree != NULL) { 00068 free(_imgDown.pointerToFree); 00069 _imgDown.pointerToFree = NULL; 00070 } 00071 if (Image::decode(imgUp, Image::RES_16BIT, &_imgUp) != 0) { 00072 DMBoard::instance().logger()->printf("Failed to load %s\n", imgUp); 00073 return false; 00074 } 00075 if (imgDown != NULL) { 00076 if (Image::decode(imgDown, Image::RES_16BIT, &_imgDown) == 0) { 00077 DMBoard::instance().logger()->printf("Failed to load %s\n", imgDown); 00078 return false; 00079 } 00080 } 00081 return true; 00082 } 00083 00084 bool ImageButton::loadImages(const unsigned char* imgUp, unsigned int imgUpSize, 00085 const unsigned char* imgDown, unsigned int imgDownSize) 00086 { 00087 if (_imgUp.pointerToFree != NULL) { 00088 free(_imgUp.pointerToFree); 00089 _imgUp.pointerToFree = NULL; 00090 } 00091 if (_imgDown.pointerToFree != NULL) { 00092 free(_imgDown.pointerToFree); 00093 _imgDown.pointerToFree = NULL; 00094 } 00095 if (Image::decode(imgUp, imgUpSize, Image::RES_16BIT, &_imgUp) != 0) { 00096 DMBoard::instance().logger()->printf("Failed to load %s\n", imgUp); 00097 return false; 00098 } 00099 if (imgDown != NULL) { 00100 if (Image::decode(imgDown, imgDownSize, Image::RES_16BIT, &_imgDown) == 0) { 00101 DMBoard::instance().logger()->printf("Failed to load %s\n", imgDown); 00102 return false; 00103 } 00104 } 00105 return true; 00106 } 00107 00108 bool ImageButton::loadImages(Resource* resImgUp, Resource* resImgDown) 00109 { 00110 if (_imgUp.pointerToFree != NULL) { 00111 free(_imgUp.pointerToFree); 00112 _imgUp.pointerToFree = NULL; 00113 } 00114 if (_imgDown.pointerToFree != NULL) { 00115 free(_imgDown.pointerToFree); 00116 _imgDown.pointerToFree = NULL; 00117 } 00118 if (Image::decode(resImgUp, Image::RES_16BIT, &_imgUp) != 0) { 00119 DMBoard::instance().logger()->printf("Failed to load \"up\" image\n"); 00120 return false; 00121 } 00122 if (resImgDown != NULL) { 00123 if (Image::decode(resImgDown, Image::RES_16BIT, &_imgDown) == 0) { 00124 DMBoard::instance().logger()->printf("Failed to load \"down\" image\n"); 00125 return false; 00126 } 00127 } 00128 return true; 00129 } 00130 00131 00132 void ImageButton::setTransparency(COLOR_T tColor) 00133 { 00134 _transparent = true; 00135 _transparentColor = tColor; 00136 } 00137 00138 void ImageButton::draw(COLOR_T* fb) 00139 { 00140 if (fb != NULL) { 00141 _win.fb = fb; 00142 } 00143 if (_caption != NULL) { 00144 _win.pen = _captionColor; 00145 swim_put_text_centered_win(&_win, _caption, _imgUp.height+2); 00146 } 00147 if (_pressed) { 00148 if (_imgDown.pixels != NULL) { 00149 if (_transparent) { 00150 swim_put_transparent_image_xy(&_win, _imgDown.pixels, _imgDown.width, _imgDown.height, 0, 0, _transparentColor); 00151 } else { 00152 swim_put_image(&_win, _imgDown.pixels, _imgDown.width, _imgDown.height); 00153 } 00154 } 00155 } else { 00156 if (_imgUp.pixels != NULL) { 00157 if (_transparent) { 00158 swim_put_transparent_image_xy(&_win, _imgUp.pixels, _imgUp.width, _imgUp.height, 0, 0, _transparentColor); 00159 } else { 00160 swim_put_image(&_win, _imgUp.pixels, _imgUp.width, _imgUp.height); 00161 } 00162 } 00163 } 00164 }
Generated on Tue Jul 12 2022 21:27:03 by
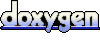