A basic graphics package for the LPC4088 Display Module.
Dependents: lpc4088_displaymodule_demo_sphere sampleGUI sampleEmptyGUI lpc4088_displaymodule_fs_aid ... more
Fork of DMBasicGUI by
AppColorPicker.h
00001 /* 00002 * Copyright 2014 Embedded Artists AB 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef APP_COLORPICKER_H 00018 #define APP_COLORPICKER_H 00019 00020 #include "App.h" 00021 #include "DMBoard.h" 00022 #include "lpc_swim.h" 00023 #include "ImageButton.h" 00024 #include "Resource.h" 00025 00026 /** 00027 * An App example letting the user pick a color from a colored area. 00028 * The hex value in RGB565 format is shown as well as a larger sample 00029 * of the selected color. 00030 * 00031 * The purpose of this example is to show let the user test the touch 00032 * panel and it can be helpful when selecting colors for e.g. backgrounds. 00033 */ 00034 class AppColorPicker : public App { 00035 public: 00036 00037 AppColorPicker(); 00038 virtual ~AppColorPicker(); 00039 00040 virtual bool setup(); 00041 virtual void runToCompletion(); 00042 virtual bool teardown(); 00043 00044 enum Resources { 00045 Resource_Ok_button, 00046 }; 00047 00048 /** Specifies the resource to use 00049 * 00050 * Adds a resource for a specific id. This allows the 00051 * user program to select e.g. which image to use and 00052 * if it should be loaded from a file or an array. 00053 * 00054 * @param id the identifier 00055 * @param res the resource 00056 */ 00057 void addResource(Resources id, Resource* res); 00058 00059 private: 00060 Display* _disp; 00061 SWIM_WINDOW_T* _win; 00062 SWIM_WINDOW_T* _colorwin; 00063 COLOR_T* _fb; 00064 COLOR_T* _fb2; 00065 ImageButton* _btn; 00066 Resource* _resOk; 00067 int _resultX; 00068 int _resultY; 00069 int _resultW; 00070 int _resultH; 00071 00072 void draw(); 00073 }; 00074 00075 #endif
Generated on Tue Jul 12 2022 21:27:03 by
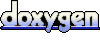