A basic graphics package for the LPC4088 Display Module.
Dependents: lpc4088_displaymodule_demo_sphere sampleGUI sampleEmptyGUI lpc4088_displaymodule_fs_aid ... more
Fork of DMBasicGUI by
AppColorPicker.cpp
00001 /* 00002 * Copyright 2014 Embedded Artists AB 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 00018 #include "mbed.h" 00019 #include "EthernetInterface.h" 00020 #include "AppColorPicker.h" 00021 #include "lpc_swim_font.h" 00022 00023 00024 /****************************************************************************** 00025 * Defines and typedefs 00026 *****************************************************************************/ 00027 00028 #define BOX_SIDE 192 00029 00030 #define BTN_OFF 20 00031 00032 /****************************************************************************** 00033 * Global variables 00034 *****************************************************************************/ 00035 00036 /****************************************************************************** 00037 * Private functions 00038 *****************************************************************************/ 00039 00040 static void buttonClicked(uint32_t x) 00041 { 00042 bool* done = (bool*)x; 00043 *done = true; 00044 } 00045 00046 void AppColorPicker::draw() 00047 { 00048 // Prepare fullscreen 00049 swim_window_open(_win, 00050 _disp->width(), _disp->height(), // full size 00051 _fb, 00052 0,0,_disp->width()-1, _disp->height()-1, // window position and size 00053 1, // border 00054 WHITE, WHITE, BLACK); // colors: pen, backgr, forgr 00055 swim_set_title(_win, "Color Picker", BLACK); 00056 00057 swim_window_open(_colorwin, 00058 _disp->width(), _disp->height(), // full size 00059 _fb, 00060 50,(_disp->height()-BOX_SIDE)/2,50+BOX_SIDE-1, BOX_SIDE+(_disp->height()-BOX_SIDE)/2, // window position and size 00061 0, // border 00062 WHITE, WHITE, BLACK); // colors: pen, backgr, forgr 00063 00064 00065 uint16_t r, g, b; 00066 uint16_t rx = BOX_SIDE/32; 00067 uint16_t bx = BOX_SIDE/32; 00068 uint16_t gy = BOX_SIDE/64; 00069 uint16_t color; 00070 for (int x = 0; x < BOX_SIDE; x++) { 00071 r = (x/rx); 00072 b = 0x1f - (x/bx); 00073 color = ((r & 0x1f) << 11) | ((b & 0x1f) << 0); 00074 for (int y = 0; y < BOX_SIDE; y++) { 00075 g = (y/gy); 00076 _colorwin->pen = color | ((g & 0x3f) << 5); 00077 swim_put_pixel(_colorwin, x, y); 00078 } 00079 } 00080 00081 _btn = new ImageButton(_win->fb, _win->xpmax - BTN_OFF - _resOk->width(), _win->ypmax - BTN_OFF - _resOk->height(), _resOk->width(), _resOk->height()); 00082 _btn->loadImages(_resOk); 00083 _btn->draw(); 00084 00085 // Copy everything onto the back buffer 00086 memcpy(_fb2, _fb, _disp->fbSize()); 00087 } 00088 00089 /****************************************************************************** 00090 * Public functions 00091 *****************************************************************************/ 00092 00093 AppColorPicker::AppColorPicker() : _disp(NULL), _win(NULL), _colorwin(NULL), 00094 _fb(NULL), _fb2(NULL), _btn(NULL), _resOk(NULL) 00095 { 00096 if (DMBoard::instance().display()->width() == 480) { 00097 _resultX = 350; 00098 _resultY = 70; 00099 _resultW = 80; 00100 _resultH = 80; 00101 } else { 00102 _resultW = 80*2; 00103 _resultH = 80*2; 00104 _resultX = 350; 00105 _resultY = (DMBoard::instance().display()->height() - (_resultH + 50))/2; 00106 } 00107 } 00108 00109 AppColorPicker::~AppColorPicker() 00110 { 00111 teardown(); 00112 } 00113 00114 bool AppColorPicker::setup() 00115 { 00116 _disp = DMBoard::instance().display(); 00117 _win = (SWIM_WINDOW_T*)malloc(sizeof(SWIM_WINDOW_T)); 00118 _colorwin = (SWIM_WINDOW_T*)malloc(sizeof(SWIM_WINDOW_T)); 00119 _fb = (COLOR_T*)_disp->allocateFramebuffer(); 00120 _fb2 = (COLOR_T*)_disp->allocateFramebuffer(); 00121 00122 return (_win != NULL && _colorwin != NULL && _fb != NULL && _fb2 != NULL); 00123 } 00124 00125 void AppColorPicker::runToCompletion() 00126 { 00127 bool done = false; 00128 draw(); 00129 _btn->setAction(buttonClicked, (uint32_t)&done); 00130 void* oldFB = _disp->swapFramebuffer(_fb2); 00131 00132 // Wait for touches 00133 TouchPanel* touch = DMBoard::instance().touchPanel(); 00134 touch_coordinate_t coord; 00135 char buf[10]; 00136 swim_set_pen_color(_win, BLACK); 00137 bool showingFB2 = true; 00138 COLOR_T lastColor = WHITE; 00139 while(!done) { 00140 // wait for a new touch signal (signal is sent from AppLauncher, 00141 // which listens for touch events) 00142 ThisThread::flags_wait_any(0x1); 00143 if (touch->read(coord) == TouchPanel::TouchError_Ok) { 00144 if (coord.z > 0 && 00145 coord.x >= _colorwin->xpmin && coord.x <= _colorwin->xpmax && 00146 coord.y >= _colorwin->ypmin && coord.y <= _colorwin->ypmax) { 00147 int x = coord.x - _colorwin->xpmin; 00148 int y = coord.y - _colorwin->ypmin; 00149 COLOR_T c = ((x/(BOX_SIDE/32))<<11) | ((y/(BOX_SIDE/64))<<5) | ((0x1f-(x/(BOX_SIDE/32)))<<0); 00150 if (c != lastColor) { 00151 swim_set_fill_color(_win, c); 00152 swim_put_box(_win, _resultX, _resultY, _resultX+_resultW, _resultY+_resultH); 00153 sprintf(buf, "0x%04x ", c); 00154 swim_put_text_xy(_win, buf, _resultX, _resultY+_resultH+10); 00155 00156 // Swap what is shown and what is drawn on 00157 if (showingFB2) { 00158 _disp->setFramebuffer(_fb); 00159 _win->fb = _fb2; 00160 } else { 00161 _disp->setFramebuffer(_fb2); 00162 _win->fb = _fb; 00163 } 00164 ThisThread::sleep_for(20); 00165 showingFB2 = !showingFB2; 00166 lastColor = c; 00167 } 00168 } 00169 if (_btn->handle(coord.x, coord.y, coord.z > 0)) { 00170 _btn->draw(showingFB2 ? _fb2 : _fb); 00171 } 00172 } 00173 } 00174 00175 // User has clicked the button, restore the original FB 00176 _disp->swapFramebuffer(oldFB); 00177 swim_window_close(_win); 00178 swim_window_close(_colorwin); 00179 } 00180 00181 bool AppColorPicker::teardown() 00182 { 00183 if (_win != NULL) { 00184 free(_win); 00185 _win = NULL; 00186 } 00187 if (_colorwin != NULL) { 00188 free(_colorwin); 00189 _colorwin = NULL; 00190 } 00191 if (_fb != NULL) { 00192 free(_fb); 00193 _fb = NULL; 00194 } 00195 if (_fb2 != NULL) { 00196 free(_fb2); 00197 _fb2 = NULL; 00198 } 00199 if (_btn != NULL) { 00200 delete _btn; 00201 _btn = NULL; 00202 } 00203 return true; 00204 } 00205 00206 void AppColorPicker::addResource(Resources id, Resource* res) 00207 { 00208 _resOk = res; 00209 }
Generated on Tue Jul 12 2022 21:27:03 by
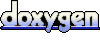