A basic graphics package for the LPC4088 Display Module.
Dependents: lpc4088_displaymodule_demo_sphere sampleGUI sampleEmptyGUI lpc4088_displaymodule_fs_aid ... more
Fork of DMBasicGUI by
Simple Windowing Interface Manager (SWIM)
This package provides the core SWIM capabilities such as Windows initialization and validity checks, Color support for background/primary/fill pens, graphics primatives, and Window deallocation. More...
Data Structures | |
struct | SWIM_WINDOW_T |
Structure used to store information about a specific window. More... | |
Functions | |
void | swim_put_pixel (SWIM_WINDOW_T *win, int32_t x1, int32_t y1) |
Puts a pixel at the virtual X, Y coordinate in the window. | |
void | swim_put_line (SWIM_WINDOW_T *win, int32_t x1, int32_t y1, int32_t x2, int32_t y2) |
Draw a line in the virtual window with clipping. | |
void | swim_put_diamond (SWIM_WINDOW_T *win, int32_t x, int32_t y, int32_t rx, int32_t ry) |
Draw a diamond in the virtual window. | |
void | swim_put_circle (SWIM_WINDOW_T *win, int32_t cx, int32_t cy, int32_t radius, int32_t Filled) |
Draws a circle in the virtual window. | |
void | swim_clear_screen (SWIM_WINDOW_T *win, COLOR_T colr) |
Fills the draw area of the display with the selected color. | |
void | swim_put_box (SWIM_WINDOW_T *win, int32_t x1, int32_t y1, int32_t x2, int32_t y2) |
Place a box with corners (X1, Y1) and (X2, Y2) | |
BOOL_32 | swim_window_open (SWIM_WINDOW_T *win, int32_t xsize, int32_t ysize, COLOR_T *fbaddr, int32_t xwin_min, int32_t ywin_min, int32_t xwin_max, int32_t ywin_max, int32_t border_width, COLOR_T pcolor, COLOR_T bkcolor, COLOR_T fcolor) |
Initializes a window and the default values for the window. | |
BOOL_32 | swim_window_open_noclear (SWIM_WINDOW_T *win, int32_t xsize, int32_t ysize, COLOR_T *fbaddr, int32_t xwin_min, int32_t ywin_min, int32_t xwin_max, int32_t ywin_max, int32_t border_width, COLOR_T pcolor, COLOR_T bkcolor, COLOR_T fcolor) |
Initializes a window without clearing it. | |
void | swim_window_close (SWIM_WINDOW_T *win) |
Deallocates a window. | |
void | swim_set_pen_color (SWIM_WINDOW_T *win, COLOR_T pen_color) |
Sets the pen color. | |
void | swim_set_fill_color (SWIM_WINDOW_T *win, COLOR_T fill_color) |
Sets the fill color. | |
void | swim_set_bkg_color (SWIM_WINDOW_T *win, COLOR_T bkg_color) |
Sets the color used for backgrounds. | |
void | swim_set_default_font (const FONT_T *def_font) |
Sets the font to be used for all new windows. | |
int32_t | swim_get_horizontal_size (SWIM_WINDOW_T *win) |
Get the virtual window horizontal size. | |
int32_t | swim_get_vertical_size (SWIM_WINDOW_T *win) |
Get the virtual window vertical size. | |
STATIC INLINE void | swim_put_pixel_physical (SWIM_WINDOW_T *win, int32_t x1, int32_t y1, COLOR_T color) |
Puts a pixel at the physical X, Y coordinate. | |
STATIC INLINE COLOR_T | swim_get_pixel_physical (SWIM_WINDOW_T *win, int32_t x1, int32_t y1) |
Read value of pixel at the physical X, Y coordinate. |
Detailed Description
This package provides the core SWIM capabilities such as Windows initialization and validity checks, Color support for background/primary/fill pens, graphics primatives, and Window deallocation.
Function Documentation
void swim_clear_screen | ( | SWIM_WINDOW_T * | win, |
COLOR_T | colr | ||
) |
Fills the draw area of the display with the selected color.
- Parameters:
-
win : Pointer to window data structure colr : Color to place in the window
- Returns:
- Nothing
Definition at line 423 of file lpc_swim.c.
int32_t swim_get_horizontal_size | ( | SWIM_WINDOW_T * | win ) |
Get the virtual window horizontal size.
- Parameters:
-
win : Pointer to window data structure
- Returns:
- The virtual window horizontal size
Definition at line 591 of file lpc_swim.c.
STATIC INLINE COLOR_T swim_get_pixel_physical | ( | SWIM_WINDOW_T * | win, |
int32_t | x1, | ||
int32_t | y1 | ||
) |
Read value of pixel at the physical X, Y coordinate.
Read value of pixel at the physical X, Y coordinate from Frame Buffer.
Note that this function must be implemented by the application! If not defined, there will be a link error.
- Parameters:
-
win : Pointer to window data structure x1 : Physical X coordinate of pixel y1 : Physical Y coordinate of pixel color : Value to write to pixel
- Returns:
- Nothing
- Note:
- This function must be implemented out side the swim library (in application).
- Parameters:
-
win : Pointer to window data structure x1 : Physical X coordinate of pixel y1 : Physical Y coordinate of pixel color : Value to write to pixel
- Returns:
- Nothing
Definition at line 326 of file lpc_swim.h.
int32_t swim_get_vertical_size | ( | SWIM_WINDOW_T * | win ) |
Get the virtual window vertical size.
- Parameters:
-
win : Pointer to window data structure
- Returns:
- The virtual window vertical size
Definition at line 597 of file lpc_swim.c.
void swim_put_box | ( | SWIM_WINDOW_T * | win, |
int32_t | x1, | ||
int32_t | y1, | ||
int32_t | x2, | ||
int32_t | y2 | ||
) |
Place a box with corners (X1, Y1) and (X2, Y2)
- Parameters:
-
win : Pointer to window data structure x1 : Virtual left position of box y1 : Virtual upper position of box x2 : Virtual right position of box y2 : Virtual lower position of box
- Returns:
- Nothing
- Note:
- Use pen color for edges and fill color for center.
Definition at line 436 of file lpc_swim.c.
void swim_put_circle | ( | SWIM_WINDOW_T * | win, |
int32_t | cx, | ||
int32_t | cy, | ||
int32_t | radius, | ||
int32_t | Filled | ||
) |
Draws a circle in the virtual window.
- Parameters:
-
win : Pointer to window data structure cx : Virtual center X position of the circle cy : Virtual center Y position of the circle radius : Radius of the circle Filled : Flag to indicate whether the circle should be filled
- Returns:
- Nothing
Definition at line 397 of file lpc_swim.c.
void swim_put_diamond | ( | SWIM_WINDOW_T * | win, |
int32_t | x, | ||
int32_t | y, | ||
int32_t | rx, | ||
int32_t | ry | ||
) |
Draw a diamond in the virtual window.
- Parameters:
-
win : Pointer to window data structure x : Virtual X position of the diamond y : Virtual Y position of the diamond rx : Radius for horizontal ry : Radius for vertical
- Returns:
- Nothing
Definition at line 320 of file lpc_swim.c.
void swim_put_line | ( | SWIM_WINDOW_T * | win, |
int32_t | x1, | ||
int32_t | y1, | ||
int32_t | x2, | ||
int32_t | y2 | ||
) |
Draw a line in the virtual window with clipping.
- Parameters:
-
win : Pointer to window data structure x1 : Virtual X position of X line start y1 : Virtual Y position of Y line start x2 : Virtual X position of X line end y2 : Virtual Y position of Y line end
- Returns:
- Nothing
Definition at line 261 of file lpc_swim.c.
void swim_put_pixel | ( | SWIM_WINDOW_T * | win, |
int32_t | x1, | ||
int32_t | y1 | ||
) |
Puts a pixel at the virtual X, Y coordinate in the window.
- Parameters:
-
win : Pointer to window data structure x1 : Virtual X position of pixel y1 : Virtual Y position of pixel
- Returns:
- Nothing
- Note:
- The pixel will not be displayed if the pixel exceeds the window virtual size. Pixel positions below 0 should not be used with this function.
Definition at line 242 of file lpc_swim.c.
STATIC INLINE void swim_put_pixel_physical | ( | SWIM_WINDOW_T * | win, |
int32_t | x1, | ||
int32_t | y1, | ||
COLOR_T | color | ||
) |
Puts a pixel at the physical X, Y coordinate.
Writes pixel color to Frame buffer at the physical X, Y coordinate.
- Parameters:
-
win : Pointer to window data structure x1 : Physical X coordinate of pixel y1 : Physical Y coordinate of pixel color : Value to write to pixel
- Returns:
- Nothing
- Note:
- This function must be implemented out side the swim library (in application).
- Parameters:
-
win : Pointer to window data structure x1 : Physical X coordinate of pixel y1 : Physical Y coordinate of pixel color : Value to write to pixel
- Returns:
- Nothing
Definition at line 340 of file lpc_swim.h.
void swim_set_bkg_color | ( | SWIM_WINDOW_T * | win, |
COLOR_T | bkg_color | ||
) |
Sets the color used for backgrounds.
- Parameters:
-
win : Pointer to window data structure bkg_color : New background color
- Returns:
- Nothing
Definition at line 578 of file lpc_swim.c.
void swim_set_default_font | ( | const FONT_T * | def_font ) |
Sets the font to be used for all new windows.
- Parameters:
-
def_font : New default font
- Returns:
- Nothing
Definition at line 585 of file lpc_swim.c.
void swim_set_fill_color | ( | SWIM_WINDOW_T * | win, |
COLOR_T | fill_color | ||
) |
Sets the fill color.
- Parameters:
-
win : Pointer to window data structure fill_color : New fill color
- Returns:
- Nothing
Definition at line 571 of file lpc_swim.c.
void swim_set_pen_color | ( | SWIM_WINDOW_T * | win, |
COLOR_T | pen_color | ||
) |
Sets the pen color.
- Parameters:
-
win : Pointer to window data structure pen_color : New pen color
- Returns:
- Nothing
Definition at line 564 of file lpc_swim.c.
void swim_window_close | ( | SWIM_WINDOW_T * | win ) |
Deallocates a window.
- Parameters:
-
win : Pointer to window data structure
- Returns:
- Nothing
- Note:
- This function does nothing.
Definition at line 558 of file lpc_swim.c.
BOOL_32 swim_window_open | ( | SWIM_WINDOW_T * | win, |
int32_t | xsize, | ||
int32_t | ysize, | ||
COLOR_T * | fbaddr, | ||
int32_t | xwin_min, | ||
int32_t | ywin_min, | ||
int32_t | xwin_max, | ||
int32_t | ywin_max, | ||
int32_t | border_width, | ||
COLOR_T | pcolor, | ||
COLOR_T | bkcolor, | ||
COLOR_T | fcolor | ||
) |
Initializes a window and the default values for the window.
- Parameters:
-
win : Pointer to window data structure xsize : Physical horizontal dimension of the display ysize : Physical vertical dimension of the display fbaddr : Address of the display's frame buffer xwin_min : Physical window left coordinate ywin_min : Physical window top coordinate xwin_max : Physical window right coordinate ywin_max : Physical window bottom coordinate border_width : Width of the window border in pixels pcolor : Pen color bkcolor : Background color fcolor : Fill color
- Returns:
- true if the window was initialized correctly, otherwise false
- Note:
- This function must be called prior to any other window function. The window will be drawn in the background color.
Definition at line 506 of file lpc_swim.c.
BOOL_32 swim_window_open_noclear | ( | SWIM_WINDOW_T * | win, |
int32_t | xsize, | ||
int32_t | ysize, | ||
COLOR_T * | fbaddr, | ||
int32_t | xwin_min, | ||
int32_t | ywin_min, | ||
int32_t | xwin_max, | ||
int32_t | ywin_max, | ||
int32_t | border_width, | ||
COLOR_T | pcolor, | ||
COLOR_T | bkcolor, | ||
COLOR_T | fcolor | ||
) |
Initializes a window without clearing it.
- Parameters:
-
win s: Pointer to window data structure xsize : Physical horizontal dimension of the display ysize : Physical vertical dimension of the display fbaddr : Address of the display's frame buffer xwin_min : Physical window left coordinate ywin_min : Physical window top coordinate xwin_max : Physical window right coordinate ywin_max : Physical window bottom coordinate border_width : Width of the window border in pixels pcolor : Pen color bkcolor : Background color fcolor : Fill color
- Returns:
- true if the window was initialized correctly, otherwise false
- Note:
- This function must be called prior to any other window function.
Definition at line 532 of file lpc_swim.c.
Generated on Tue Jul 12 2022 21:27:04 by
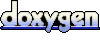