Contains added code for stm32-L432KC compatibility
Dependents: BNO080_stm32_compatible
BNO080 Class Reference
Class to drive the BNO080 9-axis IMU. More...
#include <BNO080.h>
Public Types | |
enum | Report { TOTAL_ACCELERATION = SENSOR_REPORTID_ACCELEROMETER, LINEAR_ACCELERATION = SENSOR_REPORTID_LINEAR_ACCELERATION, GRAVITY_ACCELERATION = SENSOR_REPORTID_GRAVITY, GYROSCOPE = SENSOR_REPORTID_GYROSCOPE_CALIBRATED, MAG_FIELD = SENSOR_REPORTID_MAGNETIC_FIELD_CALIBRATED, MAG_FIELD_UNCALIBRATED = SENSOR_REPORTID_MAGNETIC_FIELD_UNCALIBRATED, ROTATION = SENSOR_REPORTID_ROTATION_VECTOR, GEOMAGNETIC_ROTATION = SENSOR_REPORTID_GEOMAGNETIC_ROTATION_VECTOR, GAME_ROTATION = SENSOR_REPORTID_GAME_ROTATION_VECTOR, TAP_DETECTOR = SENSOR_REPORTID_TAP_DETECTOR, STABILITY_CLASSIFIER = SENSOR_REPORTID_STABILITY_CLASSIFIER, STEP_DETECTOR = SENSOR_REPORTID_STEP_DETECTOR, STEP_COUNTER = SENSOR_REPORTID_STEP_COUNTER, SIGNIFICANT_MOTION = SENSOR_REPORTID_SIGNIFICANT_MOTION, SHAKE_DETECTOR = SENSOR_REPORTID_SHAKE_DETECTOR } |
List of all sensor reports that the IMU supports. More... | |
enum | Stability { UNKNOWN = 0, ON_TABLE = 1, STATIONARY = 2, STABLE = 3, MOTION = 4 } |
Enum to represent the different stability types. More... | |
Public Member Functions | |
BNO080 (Serial *debugPort, PinName user_SDApin, PinName user_SCLpin, PinName user_INTPin, PinName user_RSTPin, uint8_t i2cAddress=0x4a, int i2cPortSpeed=100000) | |
Construct a BNO080, providing pins and parameters. | |
bool | begin () |
Resets and connects to the IMU. | |
void | tare (bool zOnly=false) |
Tells the IMU to use its current rotation vector as the "zero" rotation vector and to reorient all outputs accordingly. | |
bool | enableCalibration (bool calibrateAccel, bool calibrateGyro, bool calibrateMag) |
Tells the IMU to begin a dynamic sensor calibration. | |
bool | saveCalibration () |
Saves the calibration started with startCalibration() and ends the calibration. | |
void | setSensorOrientation (Quaternion orientation) |
Sets the orientation quaternion, telling the sensor how it's mounted in relation to world space. | |
bool | setPermanentOrientation (Quaternion orientation) |
Sets the orientation quaternion, telling the sensor how it's mounted in relation to world space. | |
bool | updateData () |
Checks for new data packets queued on the IMU. | |
uint8_t | getReportStatus (Report report) |
Gets the status of a report as a 2 bit number. | |
const char * | getReportStatusString (Report report) |
Get a string for printout describing the status of a sensor. | |
bool | hasNewData (Report report) |
Checks if a specific report has gotten new data since the last call to this function. | |
void | enableReport (Report report, uint16_t timeBetweenReports) |
Enable a data report from the IMU. | |
void | disableReport (Report report) |
Disable a data report from the IMU. | |
uint32_t | getSerialNumber () |
Gets the serial number (used to uniquely identify each individual device). | |
float | getRange (Report report) |
Gets the range of a report as reported by the IMU. | |
float | getResolution (Report report) |
Gets the resolution of a report as reported by the IMU. | |
float | getPower (Report report) |
Get the power used by a report when it's operating, according to the IMU. | |
float | getMinPeriod (Report report) |
Gets the smallest polling period that a report supports. | |
float | getMaxPeriod (Report report) |
Gets the larges polling period that a report supports. | |
void | printMetadataSummary (Report report) |
Prints a summary of a report's metadata to the debug stream. | |
Data Fields | |
TVector3 | totalAcceleration |
Readout from Accleration report. | |
TVector3 | linearAcceleration |
Readout from Linear Acceleration report. | |
TVector3 | gravityAcceleration |
Readout from Gravity report. | |
TVector3 | gyroRotation |
Readout from Calibrated Gyroscope report Represents the angular velocities of the chip in rad/s in the X, Y, and Z axes. | |
TVector3 | magField |
Readout from the Magnetic Field Calibrated report. | |
TVector3 | magFieldUncalibrated |
Readout from the Magnetic Field Uncalibrated report. | |
TVector3 | hardIronOffset |
Auxiliary readout from the Magnetic Field Uncalibrated report. | |
Quaternion | rotationVector |
Readout from the Rotation Vector report. | |
float | rotationAccuracy |
Auxiliary accuracy readout from the Rotation Vector report. | |
Quaternion | gameRotationVector |
Readout from the Game Rotation Vector report. | |
Quaternion | geomagneticRotationVector |
Readout from the Geomagnetic Rotation Vector report. | |
float | geomagneticRotationAccuracy |
Auxiliary accuracy readout from the Geomagnetic Rotation Vector report. | |
bool | tapDetected |
Tap readout from the Tap Detector report. | |
bool | doubleTap |
Whether the last tap detected was a single or double tap. | |
Stability | stability |
Readout from the stability classifier. | |
bool | stepDetected |
Readout from the Step Detector report. | |
uint16_t | stepCount |
Readout from the Step Counter report. | |
bool | significantMotionDetected |
Readout from the Significant Motion Detector report. | |
bool | shakeDetected |
Readout from the Shake Detector report. | |
uint8_t | majorSoftwareVersion |
Version info read from the IMU when it starts up. | |
bool | xAxisShake |
The axis/axes that shaking was detected in in the latest shaking report. |
Detailed Description
Class to drive the BNO080 9-axis IMU.
There should be one instance of this class per IMU chip. I2C address and pin assignments are passed in the constructor.
Definition at line 38 of file BNO080.h.
Member Enumeration Documentation
enum Report |
List of all sensor reports that the IMU supports.
- Enumerator:
enum Stability |
Enum to represent the different stability types.
See BNO datasheet section 2.4.1 and SH-2 section 6.5.31.2 for details.
- Enumerator:
Constructor & Destructor Documentation
BNO080 | ( | Serial * | debugPort, |
PinName | user_SDApin, | ||
PinName | user_SCLpin, | ||
PinName | user_INTPin, | ||
PinName | user_RSTPin, | ||
uint8_t | i2cAddress = 0x4a , |
||
int | i2cPortSpeed = 100000 |
||
) |
Construct a BNO080, providing pins and parameters.
This doesn't actally initialize the chip, you will need to call begin() for that.
NOTE: while some schematics tell you to connect the BOOTN pin to the processor, this driver does not use or require it. Just tie it to VCC per the datasheet.
- Parameters:
-
debugPort Serial port to write output to. Cannot be nullptr. user_SDApin Hardware I2C SDA pin connected to the IMU user_SCLpin Hardware I2C SCL pin connected to the IMU user_INTPin Input pin connected to HINTN user_RSTPin Output pin connected to NRST i2cAddress I2C address. The BNO defaults to 0x4a, but can also be set to 0x4b via a pin. i2cPortSpeed I2C frequency. The BNO's max is 400kHz.
Definition at line 73 of file BNO080.cpp.
Member Function Documentation
bool begin | ( | ) |
Resets and connects to the IMU.
Verifies that it's connected, and reads out its version info into the class variables above.
If this function is failing, it would be a good idea to turn on BNO_DEBUG in the cpp file to get detailed output.
- Returns:
- whether or not initialization was successful
Definition at line 103 of file BNO080.cpp.
void disableReport | ( | Report | report ) |
Disable a data report from the IMU.
- Parameters:
-
report The report to disable.
Definition at line 407 of file BNO080.cpp.
bool enableCalibration | ( | bool | calibrateAccel, |
bool | calibrateGyro, | ||
bool | calibrateMag | ||
) |
Tells the IMU to begin a dynamic sensor calibration.
To calibrate the IMU, call this function and move the IMU according to the instructions in the "BNO080 Sensor Calibration Procedure" app note (http://www.hillcrestlabs.com/download/59de9014566d0727bd002ae7).
To tell when the calibration is complete, look at the status bits for Game Rotation Vector (for accel and gyro) and Magnetic Field (for the magnetometer).
The gyro and accelerometer should only need to be calibrated once, but the magnetometer will need to be recalibrated every time the orientation of ferrous metals around the IMU changes (e.g. when it is put into a new enclosure).
The new calibration will not be saved in flash until you call saveCalibration().
NOTE: calling this with all false values will cancel any calibration in progress. However, the calibration data being created will remain in use until the next chip reset (I think!)
- Parameters:
-
calibrateAccel Whether to calibrate the accelerometer. calibrateGyro Whether to calibrate the gyro. calibrateMag Whether to calibrate the magnetometer.
- Returns:
- whether the operation succeeded
Definition at line 215 of file BNO080.cpp.
void enableReport | ( | Report | report, |
uint16_t | timeBetweenReports | ||
) |
Enable a data report from the IMU.
Look at the comments above to see what the reports do. This function checks your polling period against the report's max speed in the IMU's metadata, and reports an error if you're trying to poll too fast.
- Parameters:
-
timeBetweenReports time in milliseconds between data updates.
Definition at line 384 of file BNO080.cpp.
float getMaxPeriod | ( | Report | report ) |
Gets the larges polling period that a report supports.
Some reports don't have a max period, in which case this function will return -1.0.
- Returns:
- Period in seconds, or -1.0 on error.
Definition at line 455 of file BNO080.cpp.
float getMinPeriod | ( | Report | report ) |
Gets the smallest polling period that a report supports.
- Returns:
- Period in seconds.
Definition at line 448 of file BNO080.cpp.
float getPower | ( | Report | report ) |
Get the power used by a report when it's operating, according to the IMU.
- Parameters:
-
report
- Returns:
- Power used in mA.
Definition at line 439 of file BNO080.cpp.
float getRange | ( | Report | report ) |
Gets the range of a report as reported by the IMU.
Units are the same as the report's output data.
- Returns:
Definition at line 424 of file BNO080.cpp.
uint8_t getReportStatus | ( | Report | report ) |
Gets the status of a report as a 2 bit number.
per SH-2 section 6.5.1, this is interpreted as:
0 - unreliable
1 - accuracy low
2 - accuracy medium
3 - accuracy high
of course, these are only updated if a given report is enabled.
- Parameters:
-
report
- Returns:
Definition at line 345 of file BNO080.cpp.
const char * getReportStatusString | ( | Report | report ) |
Get a string for printout describing the status of a sensor.
- Returns:
Definition at line 355 of file BNO080.cpp.
float getResolution | ( | Report | report ) |
Gets the resolution of a report as reported by the IMU.
Units are the same as the report's output data.
- Parameters:
-
report
- Returns:
Definition at line 432 of file BNO080.cpp.
uint32_t getSerialNumber | ( | ) |
Gets the serial number (used to uniquely identify each individual device).
NOTE: this function should work according to the datasheet, but the device I was testing with appears to have an empty serial number record as shipped, and I could never get anything out of it. Your mileage may vary.
- Returns:
- The serial number, or 0 on error.
Definition at line 413 of file BNO080.cpp.
bool hasNewData | ( | Report | report ) |
Checks if a specific report has gotten new data since the last call to this function.
- Parameters:
-
report The report to check.
- Returns:
- Whether the report has received new data.
Definition at line 371 of file BNO080.cpp.
void printMetadataSummary | ( | Report | report ) |
Prints a summary of a report's metadata to the debug stream.
Should be useful for debugging and setting up reports since lots of this data isn't given in the datasheets.
Note: to save string constant space, this function is only available when BNO_DEBUG is 1.
Definition at line 467 of file BNO080.cpp.
bool saveCalibration | ( | ) |
Saves the calibration started with startCalibration() and ends the calibration.
You will want to call this once the status bits read as "accuracy high".
WARNING: if you paid for a factory calibrated IMU, then this WILL OVERWRITE THE FACTORY CALIBRATION in whatever sensors are being calibrated. Use with caution!
- Returns:
- whether the operation succeeded
Definition at line 256 of file BNO080.cpp.
bool setPermanentOrientation | ( | Quaternion | orientation ) |
Sets the orientation quaternion, telling the sensor how it's mounted in relation to world space.
See page 40 of the BNO080 datasheet.
Unlike setSensorOrientation(), this setting will persist across sensor restarts. However, it will also take a few hundred milliseconds to write.
- Parameters:
-
orientation quaternion mapping from IMU space to world space.
- Returns:
- true if the operation succeeded, false if it failed.
void setSensorOrientation | ( | Quaternion | orientation ) |
Sets the orientation quaternion, telling the sensor how it's mounted in relation to world space.
See page 40 of the BNO080 datasheet.
NOTE: this driver provides the macro SQRT_2 to help with entering values from that table.
NOTE 2: this setting does not persist and will have to be re-applied every time the chip is reset. Use setPermanentOrientation() for that.
- Parameters:
-
orientation quaternion mapping from IMU space to world space.
Definition at line 289 of file BNO080.cpp.
void tare | ( | bool | zOnly = false ) |
Tells the IMU to use its current rotation vector as the "zero" rotation vector and to reorient all outputs accordingly.
- Parameters:
-
zOnly If true, only the rotation about the Z axis (the heading) will be tared.
Definition at line 197 of file BNO080.cpp.
bool updateData | ( | ) |
Checks for new data packets queued on the IMU.
If there are packets queued, receives all of them and updates the class variables with the results.
- Returns:
- True iff new data packets of any kind were received. If you need more fine-grained data change reporting, check out hasNewData().
Definition at line 324 of file BNO080.cpp.
Field Documentation
bool doubleTap |
Quaternion gameRotationVector |
Quaternion geomagneticRotationVector |
uint8_t majorSoftwareVersion |
float rotationAccuracy |
Quaternion rotationVector |
bool shakeDetected |
uint16_t stepCount |
bool stepDetected |
bool tapDetected |
bool xAxisShake |
Generated on Wed Jul 13 2022 01:59:49 by
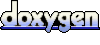