Contains added code for stm32-L432KC compatibility
Dependents: BNO080_stm32_compatible
TMatrix< Rows, Cols, value_type > Class Template Reference
A dimension-templatized class for matrices of values. More...
#include <tmatrix.h>
Inherits BasicIndexMatrix< Rows, Cols, value_type >.
Public Member Functions | |
template<uint16_t RhsCols> | |
TMatrix< Rows, RhsCols, value_type > | operator* (const TMatrix< Cols, RhsCols, value_type > &rhs) const |
Matrix multiplication operator. | |
TMatrix< Rows, Cols, value_type > | operator* (value_type s) const |
Scalar multiplication operator. | |
TMatrix< Rows, Cols, value_type > | operator+ (const TMatrix< Rows, Cols, value_type > &rhs) const |
Element-wise addition operator. | |
TMatrix< Rows, Cols, value_type > | operator- (const TMatrix< Rows, Cols, value_type > &rhs) const |
Element-wise subtraction operator. | |
TMatrix< Rows, Cols, value_type > | operator- (void) const |
Unary negation operator. | |
TMatrix< Rows, Cols, value_type > | operator/ (value_type s) const |
Scalar division operator. | |
TMatrix< Cols, Rows, value_type > | transpose (void) const |
Returns the matrix transpose of this matrix. | |
value_type | sum (void) const |
Returns the sum of the matrix entries. | |
value_type | sumLog (void) const |
Returns the sum of the log of the matrix entries. | |
TMatrix< Rows, Cols, value_type > | recip (void) const |
Returns this vector with its elements replaced by their reciprocals. | |
TMatrix< Rows, Cols, value_type > | pseudoRecip (double epsilon=1e-50) const |
Returns this vector with its elements replaced by their reciprocals, unless a value is less than epsilon, in which case it is left as zero. | |
bool | hasNaN (void) const |
Checks to see if any of this matrix's elements are NaN. | |
Static Public Member Functions | |
static TMatrix< Rows, Cols, value_type > | identity () |
Returns an "identity" matrix with dimensions given by the class's template parameters. | |
static TMatrix< Rows, Cols, value_type > | one () |
Returns a ones matrix with dimensions given by the class's template parameters. | |
static TMatrix< Rows, Cols, value_type > | zero () |
Returns a zero matrix with dimensions given by the class's template parameters. | |
Data Fields | |
TMatrix<(Rows > Cols | Cols:Rows |
Returns the diagonal elements of the matrix. | |
Friends | |
class | BasicMatrix |
Detailed Description
template<uint16_t Rows, uint16_t Cols, typename value_type = double>
class TMatrix< Rows, Cols, value_type >
A dimension-templatized class for matrices of values.
This template class generically supports any constant-sized matrix of values. The Rows
and Cols
template parameters define the size of the matrix at compile-time. Hence, the size of the matrix cannot be chosen at runtime. However, the dimensions are appropriately type-checked at compile-time where possible.
By default, the matrix contains values of type double
. The value_type
template parameter may be selected to allow matrices of integers or floats.
- Note:
- At present, type cohersion between matrices with different
value_type
parameters is not implemented. It is recommended that matrices with value typedouble
be used for all numerical computation.
Note that the special cases of row and column vectors are subsumed by this class.
Definition at line 136 of file tmatrix.h.
Member Function Documentation
bool hasNaN | ( | void | ) | const [inherited] |
Returns an "identity" matrix with dimensions given by the class's template parameters.
In the case that Rows
!= Cols
, this matrix is simply the one where the Aii elements for i < min(Rows, Cols) are 1, and all other elements are 0.
- Returns:
- A TMatrix<Rows, Cols, value_type> with off-diagonal elements set to 0, and diagonal elements set to 1.
value_type sum | ( | void | ) | const [inherited] |
value_type sumLog | ( | void | ) | const [inherited] |
Field Documentation
Generated on Wed Jul 13 2022 01:59:49 by
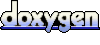