Host library for controlling a WiConnect enabled Wi-Fi module.
Dependents: wiconnect-ota_example wiconnect-web_setup_example wiconnect-test-console wiconnect-tcp_server_example ... more
Wiconnect Class Reference
[Types]
The root WiConnect library class. More...
#include <WiconnectInterface.h>
Inherits wiconnect::NetworkInterface, wiconnect::SocketInterface, wiconnect::FileInterface, and wiconnect::GhmInterface.
Public Member Functions | |
Wiconnect (const SerialConfig &serialConfig, Pin reset=PIN_NC, Pin wake=PIN_NC, bool nonBlocking=WICONNECT_DEFAULT_NONBLOCKING WICONNECT_MALLOC_ARGS) | |
WiConnect class constructor. | |
Wiconnect (const SerialConfig &serialConfig, int internalBufferSize, void *internalBuffer=NULL, Pin reset=PIN_NC, Pin wake=PIN_NC, bool nonBlocking=WICONNECT_DEFAULT_NONBLOCKING WICONNECT_MALLOC_ARGS) | |
WiConnect class constructor. | |
WiconnectResult | init (bool bringNetworkUp=false) |
Initialize library and communication link with WiConnect WiFi module. | |
void | deinit () |
De-initialize library. | |
bool | isInitialized () |
Return TRUE if library is able to communicated with WiConnect WiFi module. | |
bool | updateRequired () |
Return TRUE if the WiFi module's firmware supports the SDK version, FALSE if the WiFi module's firmware needs to be updated. | |
WiconnectResult | reset () |
Toggle the WiConnect WiFi module reset signal. | |
WiconnectResult | wakeup () |
Toggle the WiConnect WiFi moduel wakeup signal. | |
void | flush (int delayMs=500) |
Flush any received data in serial RX buffer and terminate any commands on WiConnect WiFi module. | |
WiconnectResult | getVersion (char *versionBuffer=NULL, int versionBufferSize=0, const Callback &completeCallback=Callback()) |
Return current version of WiConnect WiFi module. | |
WiconnectResult | updateFirmware (bool forced=false, const char *versionStr=NULL, const Callback &completeCallback=Callback()) |
Update the wifi module's internal firmware. | |
WiconnectResult | sendCommand (const Callback &completeCallback, char *responseBuffer, int responseBufferLen, TimerTimeout timeoutMs, const ReaderFunc &reader, void *user, const char *cmd, va_list vaList) |
Send command to WiConnect WiFi module. | |
WiconnectResult | sendCommand (char *responseBuffer, int responseBufferLen, TimerTimeout timeoutMs, const ReaderFunc &reader, void *user, const char *cmd, va_list vaList) |
Send command to WiConnect WiFi module. | |
WiconnectResult | sendCommand (char *responseBuffer, int responseBufferLen, TimerTimeout timeoutMs, const ReaderFunc &reader, void *user, const char *cmd,...) |
Send command to WiConnect WiFi module. | |
WiconnectResult | sendCommand (TimerTimeout timeoutMs, const ReaderFunc &reader, void *user, const char *cmd,...) |
Send command to WiConnect WiFi module. | |
WiconnectResult | sendCommand (const ReaderFunc &reader, void *user, const char *cmd,...) |
Send command to WiConnect WiFi module. | |
WiconnectResult | sendCommand (char *responseBuffer, int responseBufferLen, TimerTimeout timeoutMs, const char *cmd,...) |
Send command to WiConnect WiFi module. | |
WiconnectResult | sendCommand (const Callback &completeCallback, char *responseBuffer, int responseBufferLen, const char *cmd,...) |
Send command to WiConnect WiFi module. | |
WiconnectResult | sendCommand (char *responseBuffer, int responseBufferLen, const char *cmd,...) |
Send command to WiConnect WiFi module. | |
WiconnectResult | sendCommand (const Callback &completeCallback, const char *cmd,...) |
Send command to WiConnect WiFi module. | |
WiconnectResult | sendCommand (const char *cmd,...) |
Send command to WiConnect WiFi module. | |
WiconnectResult | sendCommand (const Callback &completeCallback, TimerTimeout timeoutMs, const char *cmd,...) |
This method uses the library internal buffer. | |
WiconnectResult | sendCommand (TimerTimeout timeoutMs, const char *cmd,...) |
Send command to WiConnect WiFi module. | |
WiconnectResult | sendCommand (const char *cmd, va_list vaList) |
| |
WiconnectResult | checkCurrentCommand () |
Check the status of the currently executing command. | |
void | stopCurrentCommand () |
Stop the currently executing command. | |
const char * | getLastCommandResponseCodeStr () |
When the WiConnect WiFi module returns a response, it contains a response code in the header. | |
uint16_t | getLastCommandResponseLength () |
Return the length in bytes of the previous response. | |
char * | getResponseBuffer () |
Return pointer to internal response buffer. | |
WiconnectResult | responseToUint32 (uint32_t *uint32Ptr) |
Helper method to convert previous response to uint32. | |
WiconnectResult | responseToInt32 (int32_t *int32Ptr) |
Helper method to convert previous response to int32. | |
WiconnectResult | setSetting (const char *settingStr, uint32_t value) |
Set a module setting. | |
WiconnectResult | setSetting (const char *settingStr, const char *value) |
Set a module setting. | |
WiconnectResult | getSetting (const char *settingStr, uint32_t *valuePtr) |
Get a module setting. | |
WiconnectResult | getSetting (const char *settingStr, char **valuePtr) |
Get a module setting. | |
WiconnectResult | getSetting (const char *settingStr, char *valueBuffer, uint16_t valueBufferLen) |
Get a module setting. | |
WiconnectResult | saveSettings () |
Save settings to Non-Volatile Memory. | |
void | setBlockingEnabled (bool blockingEnabled) |
Sets if API calls are blocking or non-blocking. | |
bool | getBlockingEnabled (void) |
Gets if API calls are blocking or non-blocking. | |
void | setCommandDefaultTimeout (TimerTimeout timeoutMs) |
Sets the default maximum time an API method may execute before terminating and return a timeout error code. | |
TimerTimeout | getCommandDefaultTimeout () |
Returns the current default maximum API execution time. | |
void | setPinToGpioMapper (PinToGpioMapper mapper) |
Sets a mapping function used to convert from a host Pin to WiConnect WiFi module GPIO. | |
void | setDebugLogger (LogFunc logFunc) |
Sets callback function used to debug WiConnect WiFi module RX/TX serial data. | |
void | setAssertLogger (LogFunc assertLogFunc) |
Sets callback used when Wiconnect Library hits and internal assertion. | |
WiconnectResult | enqueueCommand (QueuedCommand *command, const Callback &commandCompleteHandler=Callback()) |
Add user command to be executed asynchronously. | |
void | setCommandProcessingPeriod (uint32_t periodMs) |
Set the period at which an asynchronous command should be processed. | |
WiconnectResult | startWebSetup (const char *ssid=NULL, const char *password=NULL, const Callback &completeHandler=Callback()) |
Start the WiConnect WiFi module 'web setup' feature. | |
WiconnectResult | stopWebSetup () |
Stop the WiConnect WiFi module 'web setup' feature. | |
WiconnectResult | isWebSetupRunning (bool *isRunningPtr) |
Return status of WiConnect WiFi module 'web setup' feature. | |
WiconnectResult | join (const char *ssid=NULL, const char *password=NULL, const Callback &completeHandler=Callback()) |
Join a WiFi network. | |
WiconnectResult | leave () |
Leave a WiFi network. | |
WiconnectResult | getNetworkStatus (NetworkStatus *statusPtr) |
Get connection status to WiFi network. | |
WiconnectResult | getNetworkJoinResult (NetworkJoinResult *joinResultPtr) |
Get the result of joining the network. | |
WiconnectResult | getSignalStrength (NetworkSignalStrength *signalStrengthPtr) |
Get NetworkSignalStrength of WiFi network module is connected. | |
WiconnectResult | getRssi (int32_t *rssiPtr) |
Get the RSSI in dBm of WiFi network. | |
WiconnectResult | getMacAddress (MacAddress *macAddress) |
Get MAC address of the WiFi module. | |
WiconnectResult | scan (ScanResultList &resultList, const uint8_t *channelList=NULL, const char *ssid=NULL) |
Scan for available WiFi networks. | |
WiconnectResult | ping (const char *domain=NULL, uint32_t *timeMsPtr=NULL) |
Ping a WiFi network. | |
WiconnectResult | lookup (const char *domain, uint32_t *ipAddressPtr) |
Resolve domain name into IP address. | |
WiconnectResult | setDhcpEnabled (bool enabled) |
Set DHCP enabled. | |
WiconnectResult | getDhcpEnabled (bool *enabledPtr) |
Get if DHCP enabled. | |
WiconnectResult | setIpSettings (uint32_t ip, uint32_t netmask, uint32_t gateway) |
Set static IP settings. | |
WiconnectResult | setIpSettings (const char *ip, const char *netmask, const char *gateway) |
Set static IP settings (with string parameters) | |
WiconnectResult | getIpSettings (uint32_t *ip, uint32_t *netmask, uint32_t *gateway) |
Get network IP settings. | |
WiconnectResult | setDnsAddress (uint32_t dnsAddress) |
Set static DNS Address. | |
WiconnectResult | getDnsAddress (uint32_t *dnsAddress) |
Get the static DNS address. | |
const char * | getIpAddress (char *buffer ALLOW_NULL_STRING_BUFFER) |
Return the current IP address of the module if possible, else return 0.0.0.0. | |
WiconnectResult | closeAllSockets () |
Close all opened sockets. | |
WiconnectResult | registerSocketIrqHandler (Pin irqPin, const Callback &handler) |
Register a host pin as an external interrupt. | |
WiconnectResult | unregisterSocketIrqHandler (Pin irqPin) |
Unregister a previously registered IRQ pin. | |
WiconnectResult | connect (WiconnectSocket &socket, SocketType type, const char *host, uint16_t remortPort, uint16_t localPort, const void *args GPIO_IRQ_ARG) |
Connect to remote server. | |
WiconnectResult | tcpConnect (WiconnectSocket &socket, const char *host, uint16_t remortPort GPIO_IRQ_ARG_NC) |
Connect to remote TCP server. | |
WiconnectResult | tcpListen (uint16_t listeningPort, int maxClients=0 GPIO_IRQ_ARG_NC) |
Start internal TCP server and listen on specified port. | |
WiconnectResult | tcpAccept (WiconnectSocket &socket, uint32_t timeoutMs=WICONNECT_WAIT_FOREVER) |
Wait for next client to connect to TCP server. | |
WiconnectResult | tcpServerStop (void) |
Stop TCP server from listening on port. | |
WiconnectResult | tlsConnect (WiconnectSocket &socket, const char *host, uint16_t remortPort, const char *certFilename=NULL GPIO_IRQ_ARG_NC) |
Connect to remote TLS server. | |
WiconnectResult | udpConnect (WiconnectSocket &socket, const char *host, uint16_t remortPort, uint16_t localPort=SOCKET_ANY_PORT GPIO_IRQ_ARG_NC) |
Connect to remote UDP server. | |
WiconnectResult | udpListen (WiconnectUdpServer &udpServer, uint16_t listeningPort) |
Start a UDP server listening on the given port. | |
WiconnectResult | httpConnect (WiconnectSocket &socket, const char *url, const HttpSocketArgs *args) |
Connect to remote HTTP server. | |
WiconnectResult | httpGet (WiconnectSocket &socket, const char *url, bool openOnly=false, const char *certFilename=NULL) |
Issue HTTP GET Request. | |
WiconnectResult | httpPost (WiconnectSocket &socket, const char *url, const char *contextType, bool openOnly=true, const char *certFilename=NULL) |
Issue HTTP POST Request. | |
WiconnectResult | httpHead (WiconnectSocket &socket, const char *url, const char *certFilename=NULL) |
Issue HTTP HEAD Request. | |
WiconnectResult | httpAddHeader (WiconnectSocket &socket, const char *key, const char *value) |
Add HTTP header key/value pair to opened HTTP request. | |
WiconnectResult | httpGetStatus (WiconnectSocket &socket, uint32_t *statusCodePtr) |
Get the HTTP status code from HTTP request. | |
WiconnectResult | createFile (const ReaderFunc &reader, void *user, const char *name, uint32_t size, uint32_t version=0, FileType type=FILE_TYPE_ANY, bool isEssential=false, int32_t checksum=-1) |
Create a file on the Wiconnect WiFi module filesystem. | |
WiconnectResult | openFile (WiconnectFile &file, const char *name) |
Open a file on the Wiconnect WiFi module filesystem for reading. | |
WiconnectResult | deleteFile (const char *name) |
Delete a file for the Wiconnect WiFi module filesystem. | |
WiconnectResult | deleteFile (const WiconnectFile &file) |
Delete a file for the Wiconnect WiFi module filesystem. | |
WiconnectResult | listFiles (FileList &list, const char *name=NULL, FileType type=FILE_TYPE_ANY, uint32_t version=0) |
List the files on the Wiconnect WiFi module filesystem. | |
WiconnectResult | ghmDownloadCapabilities (const char *capsNameOrCustomUrl=NULL, uint32_t version=0) |
Download a device capabilities file to WiFi module's internal file system. | |
WiconnectResult | ghmActivate (const char *userName, const char *password, const char *capsFilename=NULL) |
Activate WiFi module with http://goHACK.me. | |
WiconnectResult | ghmDeactivate (const char *userName, const char *password) |
Deactivate WiFi module with http://goHACK.me. | |
WiconnectResult | ghmIsActivated (bool *statusPtr) |
Return if WiFi module is activated with http://goHACK.me. | |
WiconnectResult | ghmRead (const char *controlName, const char **valueStrPtr) |
Read control data from http://goHACK.me. | |
WiconnectResult | ghmRead (const char *controlName, uint32_t *valueIntPtr) |
Read control data from http://goHACK.me. | |
WiconnectResult | ghmRead (const char *controlName, char *valueBuffer, uint16_t valueBufferLen) |
Read control data from http://goHACK.me. | |
WiconnectResult | ghmWrite (const char *elementName, const char *strValue) |
Write stream or control data to http://goHACK.me. | |
WiconnectResult | ghmWrite (const char *elementName, uint32_t uintValue) |
Write stream or control data to http://goHACK.me. | |
WiconnectResult | ghmWrite (const char *elementName, int32_t intValue) |
Write stream or control data to http://goHACK.me. | |
WiconnectResult | ghmWrite (const GhmElementArray *elementArray) |
Write stream or control data to http://goHACK.me. | |
WiconnectResult | ghmSynchronize (GhmSyncType type=GHM_SYNC_ALL) |
Synchronize WiFi module with http://goHACK.me. | |
WiconnectResult | ghmPostMessage (WiconnectSocket &socket, bool jsonFormatted=false) |
POST message to http://goHACK.me. | |
WiconnectResult | ghmGetMessage (WiconnectSocket &socket, GhmMessageGetType getType=GHM_MSG_GET_DATA_ONLY) |
GET message from http://goHACK.me. | |
WiconnectResult | ghmGetMessage (WiconnectSocket &socket, uint8_t listIndex, GhmMessageGetType getType=GHM_MSG_GET_DATA_ONLY) |
GET message from http://goHACK.me. | |
WiconnectResult | ghmGetMessage (WiconnectSocket &socket, const char *msgId, GhmMessageGetType getType=GHM_MSG_GET_DATA_ONLY) |
GET message from http://goHACK.me. | |
WiconnectResult | ghmDeleteMessage (uint8_t listIndex) |
Delete message from http://goHACK.me. | |
WiconnectResult | ghmDeleteMessage (const char *msgId) |
Delete message from http://goHACK.me. | |
WiconnectResult | ghmListMessages (GhmMessageList &msgList, uint8_t maxCount=0) |
List available messages for device on http://goHACK.me. | |
Static Public Member Functions | |
static Wiconnect * | getInstance () |
Get instance of previously instantiated Wiconnect Library. | |
static const char * | getWiconnectResultStr (WiconnectResult wiconnectResult) |
Converts a WiconnectResult to string representation. | |
static uint32_t | wiconnectVersionToInt (char *versionStr) |
Converts wiconnect version string to uint32_t representation. | |
static bool | strToIp (const char *str, uint32_t *intPtr) |
Convert string to IP address. | |
static const char * | ipToStr (uint32_t ip, char *ipStrBuffer ALLOW_NULL_STRING_BUFFER) |
Convert IP address to string. | |
static const char * | networkStatusToStr (NetworkStatus status) |
Convert NetworkStatus to string. | |
static const char * | networkJoinResultToStr (NetworkJoinResult joinResult) |
Convert NetworkJoinResult to string. | |
static const char * | signalStrengthToStr (NetworkSignalStrength signalStrenth) |
Convert NetworkSignalStrength to string. | |
static NetworkSignalStrength | rssiToSignalStrength (int rssi) |
Convert RSSI (in dBm) to NetworkSignalStrength. | |
static NetworkSecurity | strToNetworkSecurity (const char *str) |
Convert string to NetworkSecurity. | |
static const char * | networkSecurityToStr (NetworkSecurity security) |
Convert NetworkSecurity to string. | |
static bool | strToSsid (const char *str, Ssid *ssid) |
Convert string Ssid. | |
static const char * | ssidToStr (const Ssid *ssid, char *ssidStrBuffer ALLOW_NULL_STRING_BUFFER) |
Convert Ssid to string. | |
static bool | strToMacAddress (const char *str, MacAddress *macAddress) |
Convert string MacAddress. | |
static const char * | macAddressToStr (const MacAddress *macAddress, char *macStrBuffer ALLOW_NULL_STRING_BUFFER) |
Convert MacAddress to string. | |
static const char * | fileVersionIntToStr (uint32_t version, bool verbose=true, char *buffer=NULL) |
Convert file version uint32 to string. | |
static bool | fileVersionStrToInt (const char *versionStr, uint32_t *versionIntPtr) |
Convert string to file version uint32. | |
static const char * | fileTypeToStr (FileType type) |
Convert FileType to string. | |
static const char * | fileFlagsToStr (FileFlags flags, char *buffer=NULL) |
Convert FileFlags to string. |
Detailed Description
The root WiConnect library class.
This class inheriets all WiConnect functionality.
This class is implemented as a 'singleton'. This means it only needs to be instantiated once. Subsequent class may either use the class instance or the static function: Wiconnect::getInstance()
Definition at line 78 of file WiconnectInterface.h.
Constructor & Destructor Documentation
Wiconnect | ( | const SerialConfig & | serialConfig, |
Pin | reset = PIN_NC , |
||
Pin | wake = PIN_NC , |
||
bool nonBlocking | MALLOC_ARGS = WICONNECT_DEFAULT_NONBLOCKING WICONNECT_MALLOC_ARGS |
||
) |
WiConnect class constructor.
- Note:
- This should only be called once within a program as the WiConnect library is implemented as a singleton.
- If this constructor is used, then all commands must be supplied with an external response buffer. This means most the API functions will not work as they use the internal buffer. It's recommended to use the other constructor that supplies an internal buffer. See Dynamic / Static Allocation
- Parameters:
-
[in] serialConfig The serial (i.e. UART) configuration connected to a WiConnect module. [in] reset Optional, The pin connected to the WiConnect module reset signal. Default: No connection [in] wake Optional, The pin connected to the WiConnect module wake signal. Default: No connection [in] nonBlocking Optional, indicates if the API blocking mode. See Blocking / Non-blocking Modes
Definition at line 109 of file Wiconnect.cpp.
Wiconnect | ( | const SerialConfig & | serialConfig, |
int | internalBufferSize, | ||
void * | internalBuffer = NULL , |
||
Pin | reset = PIN_NC , |
||
Pin | wake = PIN_NC , |
||
bool nonBlocking | MALLOC_ARGS = WICONNECT_DEFAULT_NONBLOCKING WICONNECT_MALLOC_ARGS |
||
) |
WiConnect class constructor.
- Note:
- This should only be called once within a program as the WiConnect library is implemented as a singleton.
- This is the recommended construstor as it supplies the WiConnect library with an internal buffer. Most API calls require the internal buffer.
- Parameters:
-
[in] serialConfig The serial (i.e. UART) configuration connected to a WiConnect module. [in] internalBufferSize The size of the internal buffer. If internalBuffer is NULL, then this size will be dynamically allocated. See Dynamic / Static Allocation [in] internalBuffer Optional, a user allocated buffer. See Dynamic / Static Allocation [in] reset Optional, The pin connected to the WiConnect module reset signal. Default: No connection [in] wake Optional, The pin connected to the WiConnect module wake signal. Default: No connection [in] nonBlocking Optional, indicates if the API blocking mode. See Blocking / Non-blocking Modes
Definition at line 98 of file Wiconnect.cpp.
Generated on Tue Jul 12 2022 17:35:59 by
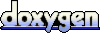