Host library for controlling a WiConnect enabled Wi-Fi module.
Dependents: wiconnect-ota_example wiconnect-web_setup_example wiconnect-test-console wiconnect-tcp_server_example ... more
WiconnectInterface.h
00001 /** 00002 * ACKme WiConnect Host Library is licensed under the BSD licence: 00003 * 00004 * Copyright (c)2014 ACKme Networks. 00005 * All rights reserved. 00006 * 00007 * Redistribution and use in source and binary forms, with or without modification, 00008 * are permitted provided that the following conditions are met: 00009 * 00010 * 1. Redistributions of source code must retain the above copyright notice, 00011 * this list of conditions and the following disclaimer. 00012 * 2. Redistributions in binary form must reproduce the above copyright notice, 00013 * this list of conditions and the following disclaimer in the documentation 00014 * and/or other materials provided with the distribution. 00015 * 3. The name of the author may not be used to endorse or promote products 00016 * derived from this software without specific prior written permission. 00017 * 00018 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS AND ANY EXPRESS OR IMPLIED 00019 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00020 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00021 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00022 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00023 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00024 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00025 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00026 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00027 * OF SUCH DAMAGE. 00028 */ 00029 #pragma once 00030 00031 #include "WiconnectTypes.h" 00032 00033 00034 #include "types/LogFunc.h" 00035 #include "types/ReaderFunc.h" 00036 #include "types/Callback.h" 00037 #include "types/TimeoutTimer.h" 00038 #include "types/PeriodicTimer.h" 00039 #include "types/Gpio.h" 00040 #include "types/WiconnectSerial.h" 00041 00042 #ifdef WICONNECT_ASYNC_TIMER_ENABLED 00043 #include "types/QueuedCommand.h" 00044 #include "types/CommandQueue.h" 00045 #endif 00046 00047 #include "NetworkInterface.h" 00048 #include "SocketInterface.h" 00049 #include "FileInterface.h" 00050 #include "GhmInterface.h" 00051 00052 00053 #ifdef WICONNECT_ENABLE_MALLOC 00054 /// These are optional arguments for host specific malloc/free 00055 #define WICONNECT_MALLOC_ARGS , void* (*malloc_)(size_t) = WICONNECT_DEFAULT_MALLOC, void (*free_)(void*) = WICONNECT_DEFAULT_FREE 00056 #else 00057 #define WICONNECT_MALLOC_ARGS 00058 #endif 00059 00060 00061 /** 00062 * @namespace wiconnect 00063 */ 00064 namespace wiconnect { 00065 00066 00067 /** 00068 * @ingroup api_core_types 00069 * 00070 * @brief The root WiConnect library class. This class 00071 * inheriets all WiConnect functionality. 00072 * 00073 * This class is implemented as a 'singleton'. This means it 00074 * only needs to be instantiated once. Subsequent class may either 00075 * use the class instance or the static function: @ref Wiconnect::getInstance() 00076 * 00077 */ 00078 class Wiconnect : public NetworkInterface, 00079 public SocketInterface, 00080 public FileInterface, 00081 public GhmInterface 00082 { 00083 public: 00084 00085 /** 00086 * @brief WiConnect class constructor 00087 * 00088 * @note This should only be called once within a program as the WiConnect 00089 * library is implemented as a singleton. 00090 * 00091 * @note If this constructor is used, then all commands must be supplied with an external response buffer. 00092 * This means most the API functions will not work as they use the internal buffer. 00093 * It's recommended to use the other constructor that supplies an internal buffer. See @ref setting_alloc 00094 * 00095 * @param[in] serialConfig The serial (i.e. UART) configuration connected to a WiConnect module. 00096 * @param[in] reset Optional, The pin connected to the WiConnect module reset signal. Default: No connection 00097 * @param[in] wake Optional, The pin connected to the WiConnect module wake signal. Default: No connection 00098 * @param[in] nonBlocking Optional, indicates if the API blocking mode. See @ref setting_blocking_modes 00099 */ 00100 Wiconnect(const SerialConfig &serialConfig, Pin reset = PIN_NC, Pin wake = PIN_NC, bool nonBlocking = WICONNECT_DEFAULT_NONBLOCKING WICONNECT_MALLOC_ARGS); 00101 00102 /** 00103 * @brief WiConnect class constructor 00104 * 00105 * @note This should only be called once within a program as the WiConnect 00106 * library is implemented as a singleton. 00107 * 00108 * @note This is the recommended construstor as it supplies the WiConnect library with an 00109 * internal buffer. Most API calls require the internal buffer. 00110 * 00111 * @param[in] serialConfig The serial (i.e. UART) configuration connected to a WiConnect module. 00112 * @param[in] internalBufferSize The size of the internal buffer. If internalBuffer is NULL, then this size will be dynamically allocated. See @ref setting_alloc 00113 * @param[in] internalBuffer Optional, a user allocated buffer. See @ref setting_alloc 00114 * @param[in] reset Optional, The pin connected to the WiConnect module reset signal. Default: No connection 00115 * @param[in] wake Optional, The pin connected to the WiConnect module wake signal. Default: No connection 00116 * @param[in] nonBlocking Optional, indicates if the API blocking mode. See @ref setting_blocking_modes 00117 */ 00118 Wiconnect(const SerialConfig &serialConfig, int internalBufferSize, void *internalBuffer = NULL, Pin reset = PIN_NC, Pin wake = PIN_NC, bool nonBlocking = WICONNECT_DEFAULT_NONBLOCKING WICONNECT_MALLOC_ARGS); 00119 ~Wiconnect(); 00120 00121 00122 // ------------------------------------------------------------------------ 00123 00124 00125 /** 00126 * @ingroup api_core_misc 00127 * 00128 * @brief Get instance of previously instantiated Wiconnect Library 00129 * 00130 * @return Pointer to instance of @ref Wiconnect Library. 00131 */ 00132 static Wiconnect* getInstance(); 00133 00134 /** 00135 * @ingroup api_core_misc 00136 * 00137 * @brief Initialize library and communication link with WiConnect WiFi module. 00138 * 00139 * @note This function is always blocking regardless of configured mode. 00140 * 00141 * @param[in] bringNetworkUp Flag indicating if the module should try to bring the network up upon initialization. 00142 * @return Result of initialization. See @ref WiconnectResult 00143 */ 00144 WiconnectResult init(bool bringNetworkUp=false); 00145 00146 /** 00147 * @ingroup api_core_misc 00148 * 00149 * @brief De-initialize library. 00150 */ 00151 void deinit(); 00152 00153 /** 00154 * @ingroup api_core_misc 00155 * 00156 * @brief Return TRUE if library is able to communicated with WiConnect WiFi module. 00157 * FALSE else. 00158 * 00159 * @return TRUE if library can communicate with WiFi module, FALSE else. 00160 */ 00161 bool isInitialized(); 00162 00163 /** 00164 * @ingroup api_core_misc 00165 * 00166 * @brief Return TRUE if the WiFi module's firmware supports the SDK version, 00167 * FALSE if the WiFi module's firmware needs to be updated. See 00168 * @ref updateFirmware() to update the module's firmware. 00169 * 00170 * @return TRUE WiFi firmware version is supported, FALSE else 00171 */ 00172 bool updateRequired(); 00173 00174 /** 00175 * @ingroup api_core_misc 00176 * 00177 * @brief Toggle the WiConnect WiFi module reset signal. 00178 * 00179 * @note This only resets the module if the library was instantiated with the 'reset' pin 00180 * parameter in the Wiconnect::Wiconnect constructor. 00181 * @note This method is always blocking. A small (1s) delay is added to ensure the module 00182 * has returned from reset and ready. 00183 * 00184 * @return Result of method. See @ref WiconnectResult 00185 */ 00186 WiconnectResult reset(); 00187 00188 /** 00189 * @ingroup api_core_misc 00190 * 00191 * @brief Toggle the WiConnect WiFi moduel wakeup signal. 00192 * 00193 * @note This only wakes the module if the library was instantiated with the 'wake' pin 00194 * parameter in the Wiconnect::Wiconnect constructor. 00195 * @note This method is always blocking. 00196 * 00197 * @return Result of method. See @ref WiconnectResult 00198 */ 00199 WiconnectResult wakeup(); 00200 00201 /** 00202 * @ingroup api_core_misc 00203 * 00204 * @brief Flush any received data in serial RX buffer and terminate any commands on WiConnect WiFi module. 00205 * 00206 * The delayMs parameter is used as the delay between terminating commands on the module and flushing 00207 * the serial RX buffer. This is needed because after terminating commands on the module, the module will 00208 * returns a response. These responses are invalid at this point and should be flushed from the serial RX buffer. 00209 * 00210 * @param[in] delayMs Optional, if not specificed this only flushes the serial RX buffer. 00211 */ 00212 void flush(int delayMs = 500); 00213 00214 /** 00215 * @ingroup api_core_misc 00216 * 00217 * @brief Return current version of WiConnect WiFi module. 00218 * @param[in] versionBuffer Optional, Buffer to hold received version string 00219 * @param[in] versionBufferSize Optional, required if versionBuffer specified. 00220 * @param[in] completeCallback Optional, callback when version is received. arg1 of callback contains version buffer pointer. 00221 * 00222 * @return Result of method. See @ref WiconnectResult 00223 */ 00224 WiconnectResult getVersion(char *versionBuffer = NULL, int versionBufferSize = 0, const Callback &completeCallback = Callback()); 00225 00226 00227 /** 00228 * @ingroup api_core_misc 00229 * 00230 * @brief Update the wifi module's internal firmware. 00231 * @param[in] forced Optional, If true, force update of all firmware files to latest version, else only update out-dated files. 00232 * @param[in] versionStr Optional, If specified, update to specific firmware version, else update to latest version. 00233 * @param[in] completeCallback Optional, callback when update is complete. 'result' callback argument contains result of update. 00234 * 00235 * @return Result of method. See @ref WiconnectResult 00236 */ 00237 WiconnectResult updateFirmware(bool forced = false, const char *versionStr = NULL, const Callback &completeCallback = Callback()); 00238 00239 00240 // ------------------------------------------------------------------------ 00241 00242 00243 /** 00244 * @ingroup api_core_send_command 00245 * 00246 * @brief Send command to WiConnect WiFi module 00247 * 00248 * @note Refer to @ref send_command_desc for more info 00249 * 00250 * @param[in] completeCallback Callback when command completes. arg1 of callback contains responseBuffer pointer, arg2 contains the response length 00251 * @param[in] responseBuffer Buffer to hold command response 00252 * @param[in] responseBufferLen Length of responseBuffer 00253 * @param[in] timeoutMs Maximum time in milliseconds this command should execute 00254 * @param[in] reader Callback for reading data to be read from host and send to module during command 00255 * @param[in] user User data struct used during read Callback. Library doesn't use this. Set NULL if not used. 00256 * @param[in] cmd WiConnect command to send to module 00257 * @param[in] vaList Varaible list of arguments 00258 * @return Result of method. See @ref WiconnectResult 00259 */ 00260 WiconnectResult sendCommand(const Callback &completeCallback, char *responseBuffer, int responseBufferLen, 00261 TimerTimeout timeoutMs, const ReaderFunc &reader, void *user, const char *cmd, va_list vaList); 00262 /** 00263 * @ingroup api_core_send_command 00264 * 00265 * @brief Send command to WiConnect WiFi module 00266 * 00267 * @note Refer to @ref send_command_desc for more info 00268 * 00269 * @param[in] responseBuffer Buffer to hold command response 00270 * @param[in] responseBufferLen Length of responseBuffer 00271 * @param[in] timeoutMs Maximum time in milliseconds this command should execute 00272 * @param[in] reader Callback for reading data to be read from host and send to module during command 00273 * @param[in] user User data struct used during read Callback. Library doesn't use this. Set NULL if not used. 00274 * @param[in] cmd WiConnect command to send to module 00275 * @param[in] vaList Varaible list of arguments 00276 * @return Result of method. See @ref WiconnectResult 00277 */ 00278 WiconnectResult sendCommand(char *responseBuffer, int responseBufferLen, TimerTimeout timeoutMs, const ReaderFunc &reader, 00279 void *user, const char *cmd, va_list vaList); 00280 00281 /** 00282 * @ingroup api_core_send_command 00283 * 00284 * @brief Send command to WiConnect WiFi module 00285 * 00286 * @note Refer to @ref send_command_desc for more info 00287 * @note This method supports variable arguments 00288 * 00289 * @param[in] responseBuffer Buffer to hold command response 00290 * @param[in] responseBufferLen Length of responseBuffer 00291 * @param[in] timeoutMs Maximum time in milliseconds this command should execute 00292 * @param[in] reader Callback for reading data to be read from host and send to module during command 00293 * @param[in] user User data struct used during read Callback. Library doesn't use this. Set NULL if not used. 00294 * @param[in] cmd WiConnect command to send to module 00295 * @return Result of method. See @ref WiconnectResult 00296 */ 00297 WiconnectResult sendCommand(char *responseBuffer, int responseBufferLen, TimerTimeout timeoutMs, const ReaderFunc &reader, 00298 void *user, const char *cmd, ...); 00299 00300 /** 00301 * @ingroup api_core_send_command 00302 * 00303 * @brief Send command to WiConnect WiFi module 00304 * 00305 * This method uses the library internal buffer. 00306 * 00307 * @note Refer to @ref send_command_desc for more info 00308 * @note This method supports variable arguments 00309 * 00310 * @param[in] timeoutMs Maximum time in milliseconds this command should execute 00311 * @param[in] reader Callback for reading data to be read from host and send to module during command 00312 * @param[in] user User data struct used during read Callback. Library doesn't use this. Set NULL if not used. 00313 * @param[in] cmd WiConnect command to send to module 00314 * @return Result of method. See @ref WiconnectResult 00315 */ 00316 WiconnectResult sendCommand(TimerTimeout timeoutMs, const ReaderFunc &reader, void *user, const char *cmd, ...); 00317 00318 /** 00319 * @ingroup api_core_send_command 00320 * 00321 * @brief Send command to WiConnect WiFi module 00322 * 00323 * - This method uses the library internal buffer and 00324 * - default timeout. See setCommandDefaultTimeout() 00325 * 00326 * @note Refer to @ref send_command_desc for more info 00327 * @note This method supports variable arguments 00328 * 00329 * @param[in] reader Callback for reading data to be read from host and send to module during command 00330 * @param[in] user User data struct used during read Callback. Library doesn't use this. Set NULL if not used. 00331 * @param[in] cmd WiConnect command to send to module 00332 * @return Result of method. See @ref WiconnectResult 00333 */ 00334 WiconnectResult sendCommand(const ReaderFunc &reader, void *user, const char *cmd, ...); 00335 00336 /** 00337 * @ingroup api_core_send_command 00338 * 00339 * @brief Send command to WiConnect WiFi module 00340 * 00341 * @note Refer to @ref send_command_desc for more info 00342 * @note This method supports variable arguments 00343 * 00344 * @param[in] responseBuffer Buffer to hold command response 00345 * @param[in] responseBufferLen Length of responseBuffer 00346 * @param[in] timeoutMs Maximum time in milliseconds this command should execute 00347 * @param[in] cmd WiConnect command to send to module 00348 * @return Result of method. See @ref WiconnectResult 00349 */ 00350 WiconnectResult sendCommand(char *responseBuffer, int responseBufferLen, TimerTimeout timeoutMs, const char *cmd, ...); 00351 00352 /** 00353 * @ingroup api_core_send_command 00354 * 00355 * @brief Send command to WiConnect WiFi module 00356 * 00357 * @note Refer to @ref send_command_desc for more info 00358 * @note This method supports variable arguments 00359 * 00360 * @param[in] completeCallback Callback when command completes. arg1 of callback contains responseBuffer pointer, arg2 contains the response length 00361 * @param[in] responseBuffer Buffer to hold command response 00362 * @param[in] responseBufferLen Length of responseBuffer 00363 * @param[in] cmd WiConnect command to send to module 00364 * @return Result of method. See @ref WiconnectResult 00365 */ 00366 WiconnectResult sendCommand(const Callback &completeCallback, char *responseBuffer, int responseBufferLen, const char *cmd, ...); 00367 00368 /** 00369 * @ingroup api_core_send_command 00370 * 00371 * @brief Send command to WiConnect WiFi module 00372 * 00373 * @note Refer to @ref send_command_desc for more info 00374 * @note This method supports variable arguments 00375 * 00376 * @param[in] responseBuffer Buffer to hold command response 00377 * @param[in] responseBufferLen Length of responseBuffer 00378 * @param[in] cmd WiConnect command to send to module 00379 * @return Result of method. See @ref WiconnectResult 00380 */ 00381 WiconnectResult sendCommand(char *responseBuffer, int responseBufferLen, const char *cmd, ...); 00382 00383 /** 00384 * @ingroup api_core_send_command 00385 * 00386 * @brief Send command to WiConnect WiFi module 00387 * 00388 * - This method uses the library internal buffer and 00389 * - default timeout. See setCommandDefaultTimeout() 00390 * 00391 * @note Refer to @ref send_command_desc for more info 00392 * @note This method supports variable arguments 00393 * 00394 * @param[in] completeCallback Callback when command completes. arg1 of callback contains responseBuffer pointer, arg2 contains the response length 00395 * @param[in] cmd WiConnect command to send to module 00396 * @return Result of method. See @ref WiconnectResult 00397 */ 00398 WiconnectResult sendCommand(const Callback &completeCallback, const char *cmd, ...); 00399 00400 /** 00401 * @ingroup api_core_send_command 00402 * 00403 * @brief Send command to WiConnect WiFi module 00404 * 00405 * - This method uses the library internal buffer and 00406 * - default timeout. See setCommandDefaultTimeout() 00407 * 00408 * @note Refer to @ref send_command_desc for more info 00409 * @note This method supports variable arguments 00410 * 00411 * @param[in] cmd WiConnect command to send to module 00412 * @return Result of method. See @ref WiconnectResult 00413 */ 00414 WiconnectResult sendCommand(const char *cmd, ...); 00415 00416 /** 00417 * @ingroup api_core_send_command 00418 * 00419 * This method uses the library internal buffer 00420 * 00421 * @note Refer to @ref send_command_desc for more info 00422 * @note This method supports variable arguments 00423 * 00424 * @param[in] completeCallback Callback when command completes. arg1 of callback contains responseBuffer pointer, arg2 contains the response length 00425 * @param[in] timeoutMs Maximum time in milliseconds this command should execute 00426 * @param[in] cmd WiConnect command to send to module 00427 * @return Result of method. See @ref WiconnectResult 00428 */ 00429 WiconnectResult sendCommand(const Callback &completeCallback, TimerTimeout timeoutMs, const char *cmd, ...); 00430 00431 /** 00432 * @ingroup api_core_send_command 00433 * 00434 * @brief Send command to WiConnect WiFi module 00435 * 00436 * This method uses the library internal buffer 00437 * 00438 * @note Refer to @ref send_command_desc for more info 00439 * @note This method supports variable arguments 00440 * 00441 * @param[in] timeoutMs Maximum time in milliseconds this command should execute 00442 * @param[in] cmd WiConnect command to send to module 00443 * @return Result of method. See @ref WiconnectResult 00444 */ 00445 WiconnectResult sendCommand(TimerTimeout timeoutMs, const char *cmd, ...); 00446 00447 /** 00448 * @ingroup api_core_send_command 00449 * 00450 * - This method uses the library internal buffer and 00451 * - default timeout. See setCommandDefaultTimeout() 00452 * 00453 * @note Refer to @ref send_command_desc for more info 00454 * 00455 * @param[in] cmd WiConnect command to send to module 00456 * @param[in] vaList Varaible list of arguments 00457 * @return Result of method. See @ref WiconnectResult 00458 */ 00459 WiconnectResult sendCommand(const char *cmd, va_list vaList); 00460 00461 /** 00462 * @ingroup api_core_send_command 00463 * 00464 * @brief Check the status of the currently executing command. 00465 * 00466 * Refer to @ref WiconnectResult for more information about the return code. 00467 * 00468 * @note This command is only applicable for non-blocking mode. Refer to @ref setting_blocking_modes. 00469 * 00470 * @return Result of method. See @ref WiconnectResult 00471 */ 00472 WiconnectResult checkCurrentCommand(); 00473 00474 /** 00475 * @ingroup api_core_send_command 00476 * 00477 * @brief Stop the currently executing command. 00478 * 00479 * @note This command is only applicable for non-blocking mode. Refer to @ref setting_blocking_modes. 00480 */ 00481 void stopCurrentCommand(); 00482 00483 00484 // ------------------------------------------------------------------------ 00485 00486 00487 /** 00488 * @ingroup api_core_misc 00489 * 00490 * @brief When the WiConnect WiFi module returns a response, it contains a 00491 * response code in the header. This function converts the previous response code 00492 * to a readable string. 00493 * 00494 * @return string representation of module response code 00495 */ 00496 const char* getLastCommandResponseCodeStr(); 00497 00498 /** 00499 * @ingroup api_core_misc 00500 * 00501 * @brief Return the length in bytes of the previous response. 00502 * 00503 * @return length of previous response 00504 */ 00505 uint16_t getLastCommandResponseLength(); 00506 00507 /** 00508 * @ingroup api_core_misc 00509 * 00510 * @brief Return pointer to internal response buffer. 00511 * 00512 * @return pointer to internal response buffer 00513 */ 00514 char* getResponseBuffer(); 00515 00516 /** 00517 * @ingroup api_core_misc 00518 * 00519 * @brief Helper method to convert previous response to uint32 00520 * 00521 * @note This uses the internal response buffer. 00522 * 00523 * @param[out] uint32Ptr Pointer to hold result of conversion. 00524 * @return Result of conversion. See @ref WiconnectResult 00525 */ 00526 WiconnectResult responseToUint32(uint32_t *uint32Ptr); 00527 00528 /** 00529 * @ingroup api_core_misc 00530 * 00531 * @brief Helper method to convert previous response to int32 00532 * 00533 * @note This uses the internal response buffer. 00534 * 00535 * @param[out] int32Ptr Pointer to hold result of conversion. 00536 * @return Result of conversion. See @ref WiconnectResult 00537 */ 00538 WiconnectResult responseToInt32(int32_t *int32Ptr); 00539 00540 00541 // ------------------------------------------------------------------------ 00542 00543 /** 00544 * @ingroup api_core_settings 00545 * 00546 * @brief Set a module setting 00547 * 00548 * Refer to: http://wiconnect.ack.me/2.0/variables 00549 * for a list of the available settings and descriptions 00550 * 00551 * @param settingStr String module setting name. 00552 * @param value The integer value to set 00553 * 00554 * @return Result of method. See @ref WiconnectResult 00555 */ 00556 WiconnectResult setSetting(const char *settingStr, uint32_t value); 00557 00558 /** 00559 * @ingroup api_core_settings 00560 * 00561 * @brief Set a module setting 00562 * 00563 * Refer to: http://wiconnect.ack.me/2.0/variables 00564 * for a list of the available settings and descriptions 00565 * 00566 * @param settingStr String module setting name. 00567 * @param value The string value to set 00568 * 00569 * @return Result of method. See @ref WiconnectResult 00570 */ 00571 WiconnectResult setSetting(const char *settingStr, const char *value); 00572 00573 /** 00574 * @ingroup api_core_settings 00575 * 00576 * @brief Get a module setting 00577 * 00578 * Refer to: http://wiconnect.ack.me/2.0/variables 00579 * for a list of the available settings and descriptions 00580 * 00581 * @param settingStr String module setting name. 00582 * @param valuePtr Pointer to buffer to contain integer value 00583 * 00584 * @return Result of method. See @ref WiconnectResult 00585 */ 00586 WiconnectResult getSetting(const char *settingStr, uint32_t *valuePtr); 00587 00588 /** 00589 * @ingroup api_core_settings 00590 * 00591 * @brief Get a module setting 00592 * 00593 * Refer to: http://wiconnect.ack.me/2.0/variables 00594 * for a list of the available settings and descriptions 00595 * 00596 * @param settingStr String module setting name. 00597 * @param valuePtr Pointer to hold pointer to internal API buffer containing retrieved setting result 00598 * 00599 * @return Result of method. See @ref WiconnectResult 00600 */ 00601 WiconnectResult getSetting(const char *settingStr, char **valuePtr); 00602 00603 /** 00604 * @ingroup api_core_settings 00605 * 00606 * @brief Get a module setting 00607 * 00608 * Refer to: http://wiconnect.ack.me/2.0/variables 00609 * for a list of the available settings and descriptions 00610 * 00611 * @param settingStr String module setting name. 00612 * @param valueBuffer Buffer to hold retrieved setting result 00613 * @param valueBufferLen The length of the input buffer 00614 * 00615 * @return Result of method. See @ref WiconnectResult 00616 */ 00617 WiconnectResult getSetting(const char *settingStr, char *valueBuffer, uint16_t valueBufferLen); 00618 00619 /** 00620 * @ingroup api_core_settings 00621 * 00622 * @brief Save settings to Non-Volatile Memory 00623 * 00624 * @return Result of method. See @ref WiconnectResult 00625 */ 00626 WiconnectResult saveSettings(); 00627 00628 /** 00629 * @ingroup api_core_settings 00630 * 00631 * @brief Sets if API calls are blocking or non-blocking. 00632 * 00633 * @param[in] blockingEnabled The new blocking value 00634 */ 00635 void setBlockingEnabled(bool blockingEnabled); 00636 00637 /** 00638 * @ingroup api_core_settings 00639 * 00640 * @brief Gets if API calls are blocking or non-blocking. 00641 */ 00642 bool getBlockingEnabled(void); 00643 00644 /** 00645 * @ingroup api_core_settings 00646 * 00647 * @brief Sets the default maximum time an API method may execute before 00648 * terminating and return a timeout error code. 00649 * 00650 * @note All API methods (execpt some sendCommand()) use this default value. 00651 * 00652 * @param[in] timeoutMs Default command timeout in milliseconds 00653 */ 00654 void setCommandDefaultTimeout(TimerTimeout timeoutMs); 00655 00656 /** 00657 * @ingroup api_core_settings 00658 * 00659 * @brief Returns the current default maximum API execution time. 00660 * 00661 * @return Default command timeout in milliseconds 00662 */ 00663 TimerTimeout getCommandDefaultTimeout(); 00664 00665 /** 00666 * @ingroup api_core_settings 00667 * 00668 * @brief Sets a mapping function used to convert from a host Pin to WiConnect WiFi module GPIO. 00669 * 00670 * @param[in] mapper Pin to GPIO mapper function pointer 00671 */ 00672 void setPinToGpioMapper(PinToGpioMapper mapper); 00673 00674 /** 00675 * @ingroup api_core_settings 00676 * 00677 * @brief Sets callback function used to debug WiConnect WiFi module RX/TX serial data. 00678 * 00679 * @param[in] logFunc Logging function pointer 00680 */ 00681 void setDebugLogger(LogFunc logFunc); 00682 00683 /** 00684 * @ingroup api_core_settings 00685 * 00686 * @brief Sets callback used when Wiconnect Library hits and internal assertion. 00687 * 00688 * @note This is mainly for debugging. There's nothing the callback can do to fix the assertion. 00689 * 00690 * @param[in] assertLogFunc Logging function pointer 00691 */ 00692 void setAssertLogger(LogFunc assertLogFunc); 00693 00694 // ------------------------------------------------------------------------ 00695 00696 00697 #ifdef WICONNECT_ASYNC_TIMER_ENABLED 00698 /** 00699 * @ingroup api_core_send_command 00700 * 00701 * @brief Add user command to be executed asynchronously. 00702 * 00703 * Refer to @ref setting_async_processing for more info. 00704 * 00705 * @param[in] command Pointer to QueuedCommand to be executed asynchronously 00706 * @param[in] commandCompleteHandler Callback to be executed when processing is complete. 00707 * @return Result of method. See @ref WiconnectResult 00708 */ 00709 WiconnectResult enqueueCommand(QueuedCommand *command, const Callback &commandCompleteHandler = Callback()); 00710 00711 /** 00712 * @ingroup api_core_settings 00713 * 00714 * @brief Set the period at which an asynchronous command should be processed. 00715 * 00716 * Refer to @ref setting_async_processing for more info. 00717 * 00718 * @param[in] periodMs Processing period in milliseconds 00719 */ 00720 void setCommandProcessingPeriod(uint32_t periodMs); 00721 #endif 00722 00723 00724 /** 00725 * @ingroup conversion_util 00726 * 00727 * @brief Converts a @ref WiconnectResult to string representation. 00728 * 00729 * @param[in] wiconnectResult Result code 00730 * @return String representaion of result code 00731 */ 00732 static const char* getWiconnectResultStr(WiconnectResult wiconnectResult); 00733 00734 /** 00735 * @ingroup conversion_util 00736 * 00737 * @brief Converts wiconnect version string to uint32_t representation. 00738 * 00739 * @param[in] Output of @ref getVersion() API call. 00740 * @return 32bit integer WiConnect firmware version 00741 */ 00742 static uint32_t wiconnectVersionToInt(char *versionStr); 00743 00744 protected: 00745 00746 #ifdef WICONNECT_ENABLE_MALLOC 00747 void* (*_malloc)(size_t); 00748 void (*_free)(void *); 00749 friend class QueuedCommand; 00750 friend class WiconnectSerial; 00751 friend class ScanResult; 00752 #endif 00753 00754 wiconnect::WiconnectSerial serial; 00755 wiconnect::Gpio resetGpio; 00756 wiconnect::Gpio wakeGpio; 00757 00758 volatile bool commandExecuting; 00759 bool initialized; 00760 bool needUpdate; 00761 bool nonBlocking; 00762 00763 PinToGpioMapper pinToGpioMapper; 00764 00765 char *internalBuffer; 00766 int internalBufferSize; 00767 bool internalBufferAlloc; 00768 uint8_t internalProcessingState; 00769 void *currentCommandId; 00770 00771 wiconnect::TimeoutTimer timeoutTimer; 00772 TimerTimeout defaultTimeoutMs; 00773 00774 uint8_t commandHeaderBuffer[32]; 00775 char commandFormatBuffer[WICONNECT_MAX_CMD_SIZE]; 00776 uint8_t commandContext[96]; 00777 00778 void prepare(void *internalBuffer, int internalBufferSize, bool nonBlocking); 00779 bool configureModuleDataBus(void); 00780 WiconnectResult inline receiveResponse(); 00781 WiconnectResult inline receivePacket(); 00782 void issueCommandCallback(WiconnectResult result); 00783 00784 #ifdef WICONNECT_ENABLE_DEBUGGING 00785 LogFunc debugLogger; 00786 #endif 00787 LogFunc assertLogger; 00788 00789 void debugLog(const char *msg, ...); 00790 00791 #ifdef WICONNECT_ASYNC_TIMER_ENABLED 00792 wiconnect::PeriodicTimer commandProcessorTimer; 00793 uint32_t commandProcessingPeriod; 00794 CommandQueue commandQueue; 00795 wiconnect::QueuedCommand *currentQueuedCommand; 00796 00797 void commandProcessingTimerHandler(void); 00798 void processNextQueuedCommand(); 00799 void checkQueuedCommandTimeout(); 00800 #endif 00801 00802 friend class NetworkInterface; 00803 friend class SocketInterface; 00804 friend class FileInterface; 00805 friend class GhmInterface; 00806 friend class GhmMessageList; 00807 friend class GhmMessage; 00808 friend class ScanResultList; 00809 friend class WiconnectFile; 00810 friend class WiconnectSocket; 00811 friend class WiconnectUdpServer; 00812 }; 00813 00814 } 00815 00816 00817 #include "sdkTypes.h" 00818
Generated on Tue Jul 12 2022 17:35:58 by
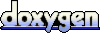